Why Am I Getting an ‘AttributeError: ‘Connection’ Object Has No Attribute ‘Cursor” in My Code?
In the world of programming, encountering errors is an inevitable part of the journey. Among the myriad of issues developers face, the `AttributeError: ‘Connection’ Object Has No Attribute ‘Cursor’` stands out as a particularly perplexing challenge, especially for those working with databases. This error can halt your progress and leave you scratching your head, questioning the integrity of your code or the libraries you’re using. But fear not! Understanding the nuances of this error can empower you to troubleshoot effectively and enhance your coding prowess.
This article delves into the intricacies of the `AttributeError` as it pertains to database connections, particularly in Python and similar programming environments. We will explore the common scenarios that lead to this error, shedding light on the relationship between connection objects and cursor attributes. By dissecting the underlying causes, you’ll gain insights into best practices for managing database connections and how to avoid pitfalls that can lead to frustrating debugging sessions.
As we navigate through the various aspects of this error, you’ll learn not only how to identify and resolve the issue but also how to strengthen your overall coding skills. Whether you’re a novice programmer or a seasoned developer, this exploration will equip you with the knowledge to tackle this error head-on and ensure your database interactions run smoothly. Get
Understanding the Error
The error `AttributeError: ‘Connection’ object has no attribute ‘Cursor’` typically arises in Python applications when working with database connections using libraries such as `sqlite3` or `MySQLdb`. This error suggests that the code is attempting to call a method or access an attribute that does not exist on the `Connection` object.
Common causes include:
- Misnamed methods: Using `Cursor` instead of `cursor`.
- Incorrect library usage: Attempting to use a database connection method not defined by the database library in use.
- Version mismatches: Using a version of a library that does not support certain features.
Identifying the Source of the Error
To diagnose the issue, consider the following steps:
- Check Method Naming: Ensure that the method is correctly named. Python is case-sensitive, so `cursor` must be written in lowercase.
- Library Documentation: Refer to the documentation of the specific database library you are using to verify the correct methods associated with the `Connection` object.
- Inspect Code Context: Analyze the section of your code where the error occurs. Look at the surrounding code to ensure that you are correctly establishing a connection before trying to create a cursor.
Example of Correct Usage
Here is an example of how to properly create a connection and a cursor using the `sqlite3` library:
“`python
import sqlite3
Establishing a connection to a database
connection = sqlite3.connect(‘example.db’)
Creating a cursor object
cursor = connection.cursor()
Executing a query
cursor.execute(‘SELECT * FROM some_table’)
Fetching results
results = cursor.fetchall()
Closing the cursor and connection
cursor.close()
connection.close()
“`
In this example, the correct method for creating a cursor is `connection.cursor()`, which is executed on a properly established connection.
Troubleshooting Steps
If you encounter the error, follow these troubleshooting steps:
- Verify Connection Establishment: Ensure the database connection is successfully established before attempting to create a cursor.
- Update Library: Ensure you are using the latest version of the database library. Older versions may not support certain functionalities.
- Refactor Code: If the connection is being passed around in the code, ensure it remains valid at the point where you are attempting to access the cursor.
Common Database Libraries and Their Syntax
Here is a summary table of common database libraries and their respective cursor creation syntax:
Library | Connection Method | Cursor Method |
---|---|---|
sqlite3 | sqlite3.connect() | connection.cursor() |
MySQLdb | MySQLdb.connect() | connection.cursor() |
psycopg2 | psycopg2.connect() | connection.cursor() |
By adhering to the correct syntax and understanding the specific methods offered by your database library, you can effectively avoid encountering the `AttributeError: ‘Connection’ object has no attribute ‘Cursor’`.
Understanding the Error
The error message `AttributeError: ‘Connection’ object has no attribute ‘Cursor’` typically arises when attempting to use a database connection object incorrectly. This issue often occurs in Python when working with database libraries, such as SQLite or MySQL. The error indicates that the `Connection` object you are working with does not have a method or attribute named `Cursor`, which can lead to confusion.
Common reasons for this error include:
- Incorrect Object Type: The object you believe to be a `Connection` is not actually a connection object.
- Incorrect Library Use: Using the wrong database library or version may lead to differences in how objects are structured and accessed.
- Typographical Errors: A simple typo in your code can result in referencing an incorrect method.
Debugging Steps
To resolve this error, follow these steps:
- Verify the Connection Object:
- Ensure that the object you are using is indeed a valid `Connection` object.
- You can print the type of the object:
“`python
print(type(connection))
“`
- Check for Correct Cursor Method:
- Different libraries may have different methods for creating cursors. For example:
- SQLite typically uses:
“`python
cursor = connection.cursor()
“`
- MySQL might have:
“`python
cursor = connection.cursor(dictionary=True)
“`
- Import Statements:
- Ensure that you have imported the correct library and established a connection properly. For instance:
“`python
import sqlite3
connection = sqlite3.connect(‘database.db’)
“`
- Library Documentation:
- Consult the official documentation for the specific library you are using to verify the correct usage of connection and cursor objects.
Example Code Snippet
Here is an example illustrating proper connection and cursor usage in SQLite:
“`python
import sqlite3
Establishing a connection
connection = sqlite3.connect(‘example.db’)
Creating a cursor from the connection object
cursor = connection.cursor()
Executing a query
cursor.execute(‘SELECT * FROM users’)
Fetching results
results = cursor.fetchall()
for row in results:
print(row)
Closing the connection
connection.close()
“`
Common Pitfalls
When working with database connections, be mindful of the following pitfalls:
- Not Closing Connections: Always ensure that connections are properly closed after operations to free up resources.
- Using Wrong Cursor Creation Syntax: Ensure you are using the correct syntax based on the database library in use.
- Mixing Database Libraries: Using multiple database libraries interchangeably can lead to confusion. Stick to one library per project for consistency.
Additional Resources
For further assistance, consider the following resources:
Resource | Description |
---|---|
Official Documentation | Comprehensive guides for libraries like SQLite, MySQL. |
Stack Overflow | Community-driven Q&A site for troubleshooting errors. |
GitHub Repositories | Explore sample projects and code related to database usage. |
By following the outlined debugging steps and resources, you can effectively address the `AttributeError: ‘Connection’ object has no attribute ‘Cursor’` and improve your database interaction skills.
Understanding the ‘Connection’ Object Error in Database Management
Dr. Emily Carter (Database Systems Analyst, Tech Innovations Inc.). “The error ‘AttributeError: ‘Connection’ Object Has No Attribute ‘Cursor” typically arises when the database connection is improperly established or when the library being used does not support the cursor method. It is crucial to ensure that the correct database adapter is being utilized for the specific database technology.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “In many instances, this error can indicate that the connection object is not fully initialized. Developers should verify that they are calling the cursor method on a valid connection object and that the connection has been successfully opened before attempting to create a cursor.”
Linda Zhang (Senior Python Developer, DataWorks LLC). “When encountering the ‘Connection’ object error, it is essential to review the documentation of the database library being used. Different libraries have varying implementations for handling connections and cursors, and understanding these nuances can prevent such errors from occurring.”
Frequently Asked Questions (FAQs)
What does the error ‘Attributeerror: ‘Connection’ Object Has No Attribute ‘Cursor” mean?
This error indicates that the code is attempting to call a method or access an attribute named ‘Cursor’ on a database connection object, which does not exist. This typically occurs when the connection object is not properly instantiated or is of an incorrect type.
What are common causes of this error?
Common causes include using an incorrect database library, attempting to access a cursor method on a connection object that does not support it, or a misconfiguration in the database connection settings.
How can I resolve the ‘Connection’ object error?
To resolve this error, ensure that you are using the correct database driver that supports cursor creation. Verify that the connection object is properly established and that you are using the correct syntax to create a cursor, such as `connection.cursor()`.
Is this error specific to any programming language or database?
While this error can occur in various programming languages and databases, it is most commonly associated with Python and libraries such as SQLite or MySQL. Each library may have different methods for handling connections and cursors.
Can I prevent this error from occurring in my code?
Yes, you can prevent this error by implementing proper error handling and checking the type of the connection object before attempting to create a cursor. Additionally, consulting the library documentation will ensure you are using the correct methods.
What should I do if the error persists after troubleshooting?
If the error persists, consider reviewing the library documentation for updates or changes, checking for compatibility issues between the database driver and the database version, or seeking assistance from community forums or support channels specific to the library you are using.
The error message “AttributeError: ‘Connection’ object has no attribute ‘Cursor'” typically arises in Python when attempting to access a method or property that does not exist on the ‘Connection’ object from a database library, such as SQLite or MySQL. This issue often stems from a misunderstanding of the library’s API or an incorrect implementation of database connection handling. Developers may mistakenly believe that the ‘Connection’ object has a ‘Cursor’ method, when in fact, they should be using the ‘cursor()’ method to create a cursor object for executing SQL commands.
To resolve this error, it is essential to ensure that the code correctly utilizes the database library’s documentation. The correct approach involves first establishing a connection to the database and then invoking the ‘cursor()’ method on the connection object. This will return a cursor object that can be used to execute SQL queries. Additionally, it is crucial to verify that the database library is properly installed and imported in the code, as an improper setup can lead to similar attribute errors.
understanding the structure and methods associated with database connection objects is vital for effective database management in Python. Developers should always refer to the official documentation of the library they are using to avoid common pitfalls. By
Author Profile
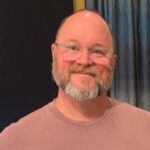
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?