How Can You Effectively Print in TypeScript?
In the world of web development, TypeScript has emerged as a powerful tool that enhances JavaScript with static typing and robust features. As developers embrace this superset of JavaScript, they often encounter the need to output information to the console or the user interface. Whether you’re debugging your code, displaying data, or simply logging messages for clarity, knowing how to effectively print in TypeScript is essential. This article will guide you through the various methods and best practices for outputting information in TypeScript, ensuring you can communicate effectively with your code and your audience.
When working with TypeScript, printing information can be accomplished through several methods, each serving different purposes. The most common approach is using the `console.log()` function, which allows developers to output messages to the console for debugging and monitoring application behavior. However, TypeScript also provides other methods to display information, such as manipulating the Document Object Model (DOM) to present data directly on the web page. Understanding these techniques not only enhances your coding skills but also improves your ability to troubleshoot and refine your applications.
As we delve deeper into the intricacies of printing in TypeScript, we will explore the nuances of each method, discuss their appropriate use cases, and share tips to optimize your output processes. By mastering these
Using Console for Output
The most common way to print output in TypeScript is through the console. The `console.log()` function is a widely used method to display information in the console, making it easy to debug and monitor the application’s behavior.
Example usage:
“`typescript
console.log(“Hello, TypeScript!”);
“`
This function can also accept multiple arguments, allowing for versatile output formatting. For instance, you can log numbers, strings, objects, and arrays in one go:
“`typescript
const user = { name: “Alice”, age: 30 };
console.log(“User Details:”, user);
“`
Printing to the DOM
If the goal is to display output directly on a webpage, you can manipulate the Document Object Model (DOM) using TypeScript. This involves selecting an HTML element and setting its inner content.
Here’s how to achieve this:
- Select the target element using `document.getElementById()` or other DOM selection methods.
- Set the `innerHTML` or `textContent` property to update the element’s content.
Example code:
“`typescript
const outputElement = document.getElementById(“output”);
if (outputElement) {
outputElement.textContent = “Hello, World!”;
}
“`
Ensure that your HTML includes an element with the corresponding ID:
“`html
“`
Formatting Output
When printing data, especially complex objects or arrays, formatting can enhance readability. You can use `JSON.stringify()` to convert objects or arrays into a readable string format.
Example:
“`typescript
const data = { id: 1, name: “Item”, values: [1, 2, 3] };
console.log(JSON.stringify(data, null, 2)); // Pretty prints the object
“`
This method takes three parameters: the object to stringify, a replacer function (optional), and the number of spaces to use for indentation.
Table of Common Print Methods
Below is a table summarizing various methods to print output in TypeScript along with their use cases.
Method | Description | Example |
---|---|---|
console.log() | Logs output to the console for debugging. | console.log(“Hello!”); |
console.error() | Logs error messages to the console. | console.error(“An error occurred.”); |
document.write() | Writes directly to the HTML document (not recommended). | document.write(“Hello, World!”); |
Element.innerHTML | Sets or gets the HTML content of an element. | element.innerHTML = “Bold Text“; |
Using these methods effectively allows developers to manage output in TypeScript applications, facilitating easier debugging and improved user interaction.
Using Console for Output
Printing in TypeScript can be effectively done using the console object. The console provides several methods to display output in the developer console.
- console.log(): Outputs a message to the console.
- console.error(): Outputs an error message to the console.
- console.warn(): Outputs a warning message to the console.
- console.info(): Outputs an informational message to the console.
Example usage:
“`typescript
console.log(“This is a log message.”);
console.error(“This is an error message.”);
console.warn(“This is a warning message.”);
console.info(“This is an informational message.”);
“`
Displaying Output in the Browser
For web applications, you may want to display output directly on the web page. This can be achieved using the Document Object Model (DOM).
- Select an HTML element where you want to display the output.
- Modify its innerHTML or textContent property.
Example:
“`typescript
const outputElement = document.getElementById(“output”);
if (outputElement) {
outputElement.textContent = “Hello, World!”;
}
“`
Using Template Literals for Complex Outputs
Template literals are a powerful feature in TypeScript that allows for multi-line strings and string interpolation, making it easier to format output.
Example:
“`typescript
const name = “John”;
const age = 30;
const message = `Hello, my name is ${name} and I am ${age} years old.`;
console.log(message);
“`
Creating Custom Print Functions
For more complex applications, you may want to encapsulate printing logic in a function. This enhances reusability and maintainability.
Example:
“`typescript
function printMessage(message: string): void {
console.log(message);
}
printMessage(“This is a custom print function.”);
“`
Formatting Output with CSS
When displaying output in the browser, you can enhance the visual appearance by applying CSS styles.
Example:
“`html
“`
This CSS applies a blue color and bold weight to the text displayed in the output div.
Using Third-Party Libraries
For advanced logging and output formatting, consider using libraries such as:
- Winston: A versatile logging library.
- Log4js: A powerful logging framework with various appenders.
Integrating these libraries can provide additional features such as log levels, file output, and more.
Example using Winston:
“`typescript
import { createLogger, format, transports } from ‘winston’;
const logger = createLogger({
level: ‘info’,
format: format.json(),
transports: [new transports.Console()],
});
logger.info(‘This is an information message.’);
“`
Conclusion on Printing in TypeScript
Utilizing the various methods for output in TypeScript allows developers to effectively communicate information within applications. Whether through the console, directly on web pages, or via custom functions, TypeScript offers flexible options for displaying data.
Expert Insights on Printing in TypeScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “When printing in TypeScript, it is essential to understand the underlying JavaScript methods available. Utilizing `console.log()` is the most straightforward approach, but for more complex data structures, consider using `JSON.stringify()` for better readability.”
James Liu (Lead Developer, CodeCraft Solutions). “TypeScript enhances JavaScript’s capabilities, and when it comes to printing, leveraging interfaces and types can significantly improve the clarity of your output. Always define your data structures to ensure that printed results are both informative and type-safe.”
Sarah Thompson (Technical Writer, DevDocs Publishing). “Incorporating print functionality in TypeScript applications should also consider user experience. For instance, using libraries like `print-js` can streamline the process of printing documents directly from the browser, providing a seamless experience for end-users.”
Frequently Asked Questions (FAQs)
How do I print to the console in TypeScript?
To print to the console in TypeScript, use the `console.log()` method. For example, `console.log(‘Hello, World!’);` outputs the string to the console.
Can I print objects in TypeScript?
Yes, you can print objects in TypeScript using `console.log()`. For instance, `console.log({ name: ‘Alice’, age: 30 });` will display the object structure in the console.
Is there a way to format output when printing in TypeScript?
Yes, you can format output using template literals. For example, `console.log(`User: ${user.name}, Age: ${user.age}`);` allows for dynamic string interpolation.
How can I print errors in TypeScript?
To print errors, use `console.error()`. For example, `console.error(‘An error occurred:’, error);` will output the error message to the console in a distinct format.
Can I print messages conditionally in TypeScript?
Yes, you can use conditional statements to print messages. For example, `if (isLoggedIn) { console.log(‘Welcome back!’); }` prints the message only if the condition is true.
What are the differences between console.log and console.warn in TypeScript?
`console.log()` is used for general output, while `console.warn()` is specifically for warning messages. The latter usually appears with a yellow background in the console, indicating a warning rather than standard output.
printing in TypeScript can be accomplished through various methods, depending on the context and requirements of the application. The most common approach is using the `console.log()` function, which allows developers to output information to the console for debugging purposes. This method is particularly useful during the development phase, as it provides immediate feedback on variable values and application flow.
Another method for printing in TypeScript is manipulating the Document Object Model (DOM) to display information on a web page. This can be achieved by selecting an HTML element and updating its inner content using TypeScript. This approach is beneficial for creating dynamic web applications where user interaction and real-time updates are necessary.
Additionally, TypeScript supports the use of third-party libraries and frameworks that can enhance the printing capabilities. For instance, libraries like `print-js` can facilitate printing documents directly from the browser, offering more control over the layout and content. Understanding these various methods allows developers to choose the most suitable approach for their specific use case.
Ultimately, mastering the techniques for printing in TypeScript not only improves debugging efficiency but also enhances user experience by providing clear and relevant information. By leveraging the built-in functions and available libraries, developers can effectively communicate data and application
Author Profile
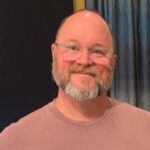
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?