Is JavaScript Pass By Reference or Pass By Value? Understanding the Basics
In the world of programming, understanding how data is handled can make all the difference in writing efficient and bug-free code. One of the most debated topics among developers is whether JavaScript uses pass by reference or pass by value. This distinction is crucial because it influences how variables interact with functions, how data is manipulated, and ultimately, how applications behave. As you delve into the nuances of JavaScript’s data handling, you’ll uncover the intricacies that define its approach, shedding light on common misconceptions and enhancing your coding prowess.
When we talk about pass by reference and pass by value, we’re essentially discussing how arguments are passed to functions in programming languages. In JavaScript, this concept can be particularly perplexing due to its unique handling of primitive and object types. While primitive data types like numbers and strings are passed by value—meaning a copy of the data is sent to the function—objects and arrays are treated differently, leading many to believe that they are passed by reference. This duality can create confusion, especially for those new to the language or transitioning from languages with more rigid rules regarding data passing.
As we explore this topic further, we’ll clarify the mechanics behind JavaScript’s argument passing, examining how it affects variable manipulation and function behavior. By demystifying these
Understanding Pass By Value and Pass By Reference
In JavaScript, the concept of passing arguments to functions can be categorized into two primary types: pass by value and pass by reference. Understanding these concepts is crucial for developers as it affects how variables are handled within functions.
Pass by value means that a copy of the variable is passed to the function. Any changes made to the parameter inside the function do not affect the original variable outside the function. In contrast, pass by reference means that a reference to the actual variable is passed, allowing changes made to the parameter to reflect on the original variable.
JavaScript’s Approach
JavaScript utilizes a combination of both methodologies based on the data type being handled:
- Primitive Types: These include numbers, strings, booleans, `null`, “, and `symbol`. When a primitive type is passed to a function, JavaScript creates a copy of the value. Changes to this copy do not affect the original variable.
- Reference Types: These include objects, arrays, and functions. When a reference type is passed to a function, JavaScript passes a reference to the memory location of the object. Changes made to the object within the function will affect the original object.
Examples of Pass By Value
“`javascript
function modifyPrimitive(num) {
num = num + 10;
return num;
}
let originalNum = 5;
let modifiedNum = modifyPrimitive(originalNum);
console.log(originalNum); // 5
console.log(modifiedNum); // 15
“`
In this example, `originalNum` remains unchanged after the function call, demonstrating pass by value.
Examples of Pass By Reference
“`javascript
function modifyObject(obj) {
obj.name = “New Name”;
}
let originalObject = { name: “Old Name” };
modifyObject(originalObject);
console.log(originalObject.name); // “New Name”
“`
Here, the `originalObject` is modified because objects are passed by reference, allowing the function to modify the original object.
Comparison Table
Characteristic | Pass By Value | Pass By Reference |
---|---|---|
Data Types | Primitive types (e.g., number, string) | Reference types (e.g., objects, arrays) |
Effect of Function Changes | Original variable remains unchanged | Original variable can be modified |
Example | Passing a number | Passing an object |
Understanding these distinctions is essential for effective JavaScript programming, as it influences how data is manipulated within functions and how unexpected behaviors can arise from mutating objects. Developers should be mindful of these differences when designing functions and managing data flow in their applications.
Understanding JavaScript’s Parameter Passing
JavaScript utilizes a unique approach to parameter passing that can often lead to confusion regarding whether it is “pass by reference” or “pass by value.”
Pass by Value vs. Pass by Reference
To clarify the concepts of pass by value and pass by reference:
- Pass by Value: This means that a copy of the variable’s value is passed to the function. Changes made to the parameter inside the function do not affect the original variable.
- Pass by Reference: This indicates that a reference to the actual variable is passed to the function. Changes made to the parameter affect the original variable.
JavaScript’s Behavior with Primitive and Non-Primitive Types
JavaScript distinguishes between primitive and non-primitive types when passing arguments:
- Primitive Types (e.g., Number, String, Boolean, Null, , Symbol):
- Passed by value.
- Example:
“`javascript
let a = 5;
function modifyValue(b) {
b = 10;
}
modifyValue(a);
console.log(a); // Output: 5
“`
- Non-Primitive Types (e.g., Object, Array, Function):
- Passed by reference.
- Example:
“`javascript
let obj = { value: 5 };
function modifyObject(o) {
o.value = 10;
}
modifyObject(obj);
console.log(obj.value); // Output: 10
“`
Implications of JavaScript’s Parameter Passing
Understanding how JavaScript handles parameter passing can influence coding practices significantly:
- Mutability:
- Non-primitive types can be mutated, leading to side effects if not handled carefully.
- Function Design:
- Functions that modify input objects can lead to unexpected behaviors if the original objects are referenced elsewhere in the code.
- Avoiding Side Effects:
- To avoid unintended modifications, it is often advisable to create copies of objects or arrays before passing them to functions.
Examples of Common Patterns
Here are common patterns to illustrate the behavior of JavaScript’s parameter passing:
Type | Example Code | Description |
---|---|---|
Primitive (Number) | `function changeValue(n) { n = 20; }` | `n` is a copy; the original variable remains unchanged. |
Primitive (String) | `function changeString(s) { s = “new”; }` | `s` is a copy; the original string remains unchanged. |
Non-Primitive | `function changeArray(arr) { arr.push(4); }` | `arr` references the original array, modifying it directly. |
Non-Primitive | `function changeObj(obj) { obj.key = ‘new’; }` | `obj` references the original object, modifying it directly. |
Conclusion on Parameter Passing in JavaScript
The distinction between how JavaScript handles primitive and non-primitive types is crucial for developers. By understanding these concepts, developers can write more predictable and bug-free code, effectively managing the implications of JavaScript’s parameter passing mechanism.
Understanding JavaScript’s Pass By Reference Mechanism
Dr. Emily Carter (Senior Software Engineer, Code Innovations Inc.). “JavaScript is often misunderstood in terms of its handling of variables. While it utilizes a pass-by-value mechanism for primitive types, objects and arrays are passed by reference. This distinction is crucial for developers to grasp, as it affects how data is manipulated within functions.”
Michael Chen (Lead JavaScript Developer, Tech Solutions Group). “In JavaScript, when you pass an object to a function, you are passing a reference to that object, not the object itself. This means that changes made to the object within the function will reflect outside of it, which can lead to unintended side effects if not properly managed.”
Sarah Johnson (JavaScript Educator, Web Development Academy). “It’s important to clarify that JavaScript’s behavior can lead to confusion, particularly for those coming from languages that strictly enforce pass-by-reference. Understanding that objects are passed by reference while primitives are passed by value is essential for effective coding in JavaScript.”
Frequently Asked Questions (FAQs)
Is JavaScript pass by reference or pass by value?
JavaScript primarily uses pass by value for primitive data types (e.g., numbers, strings, booleans) and pass by reference for objects and arrays. When you pass an object to a function, the reference to that object is passed, allowing modifications to the original object.
What happens when you pass an object to a function in JavaScript?
When an object is passed to a function, the function receives a reference to that object. Any changes made to the object within the function will affect the original object outside the function.
Can you demonstrate pass by reference with an example?
Certainly. For instance, if you have an object `let obj = { key: ‘value’ };` and pass it to a function that modifies `obj.key`, the change will persist outside the function, demonstrating pass by reference.
Are arrays treated differently than objects in JavaScript?
No, arrays are also objects in JavaScript. When you pass an array to a function, you are passing a reference to that array, allowing the function to modify its contents directly.
What is the significance of pass by value in JavaScript?
Pass by value ensures that primitive data types remain unchanged when passed to functions. This behavior prevents unintended side effects, as modifications to the parameter do not affect the original variable.
How can you create a copy of an object to avoid modifying the original?
To create a copy of an object, you can use methods such as `Object.assign({}, originalObject)` or the spread operator `{ …originalObject }`. This creates a shallow copy, preventing changes to the copy from affecting the original object.
In JavaScript, the concept of passing variables to functions can be somewhat misleading due to the language’s handling of data types. JavaScript employs a combination of pass-by-value and pass-by-reference, depending on whether the variable is a primitive type or an object. Primitive types, such as numbers, strings, and booleans, are passed by value, meaning that a copy of the variable is made when it is passed to a function. Any modifications to this copy do not affect the original variable.
On the other hand, objects and arrays in JavaScript are passed by reference. This means that when an object or an array is passed to a function, a reference to the original object is provided, not a copy. Consequently, if the function alters the properties of the object or the contents of the array, those changes will be reflected outside the function, affecting the original data. This duality can lead to confusion, particularly for those new to the language, as the behavior differs based on the type of data being manipulated.
Understanding the distinction between pass-by-value and pass-by-reference is crucial for effective JavaScript programming. Developers must be mindful of how data is passed to functions to avoid unintended side effects, particularly when working with objects
Author Profile
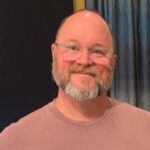
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?