Should You Process JSON One by One or All in One? Exploring the Best Approach
In the world of data interchange, JSON (JavaScript Object Notation) has emerged as a fundamental format due to its simplicity and versatility. Whether you’re developing web applications, mobile apps, or server-side solutions, the way you process JSON can significantly impact performance and user experience. The debate between processing JSON one by one or all in one batch is a crucial consideration for developers looking to optimize their applications. This article delves into the nuances of these two approaches, guiding you through their advantages and potential pitfalls, and helping you make informed decisions for your projects.
When it comes to handling JSON data, the method you choose can affect everything from speed to resource management. Processing JSON one by one allows for real-time handling of data, which can be particularly beneficial in scenarios where immediate feedback is necessary. On the other hand, processing all JSON data in one go can lead to improved efficiency and reduced overhead, especially when dealing with large datasets. Each approach has its own set of trade-offs that can influence the overall architecture of your application.
As we explore the intricacies of these processing methods, we will examine key factors such as performance, scalability, and ease of implementation. By understanding the implications of each method, you can better tailor your JSON processing strategy to meet the specific
Processing JSON One By One
Processing JSON data one by one refers to the method of handling each data record individually. This approach is particularly useful when dealing with large datasets or when processing time needs to be minimized. By focusing on one record at a time, developers can ensure that errors are caught and handled immediately, maintaining overall system stability.
Key advantages of processing JSON one by one include:
- Error Handling: Issues can be addressed on a per-record basis without affecting the entire dataset.
- Resource Management: Lower memory usage since only a single record is loaded at any time.
- Sequential Processing: Allows for ordered execution, which may be necessary for certain applications.
The process typically involves the following steps:
- Parsing: Each JSON object is parsed to extract relevant information.
- Processing: Business logic is applied to the data.
- Storing/Outputting: The result is either stored in a database or outputted for further use.
This method is often implemented in loops, iterating over each JSON entry.
Processing JSON All In One
Processing JSON all in one involves handling the entire dataset at once. This method is efficient for smaller datasets or when speed is a priority, as it allows for bulk operations. However, this approach may require more memory and could lead to performance bottlenecks if the dataset is too large.
Advantages of processing JSON all in one include:
- Performance: Faster execution since all data is loaded and processed in a single operation.
- Simplicity: Easier to write and maintain code when dealing with bulk data.
- Batch Operations: Allows for operations that affect multiple records simultaneously, such as bulk inserts or updates in databases.
The processing steps for this method typically include:
- Bulk Parsing: The entire JSON array is parsed in one go.
- Batch Processing: Business logic is applied to all records simultaneously.
- Outputting/Storage: The results are saved or returned as a single operation.
A comparison of the two methods can be illustrated in the following table:
Feature | One By One | All In One |
---|---|---|
Error Handling | Immediate for each record | Requires post-processing analysis |
Memory Usage | Low | High |
Performance | Slower for large datasets | Faster for small to medium datasets |
Complexity | More complex error checking | Simpler implementation |
Ultimately, the choice between processing JSON one by one or all in one depends on the specific use case, dataset size, and performance requirements of the application. Each method has its strengths and can be applied effectively in different scenarios.
Processing JSON One By One
Processing JSON data individually can be particularly useful when handling large datasets or when specific records need to be manipulated or analyzed. This method allows for a focused approach, where each JSON object is processed in isolation.
Key advantages of processing JSON one by one include:
- Memory Efficiency: Loading only one record at a time minimizes memory usage, which is crucial for large datasets.
- Targeted Manipulation: Allows for focused operations on specific records without affecting the entire dataset.
- Error Handling: Easier to identify and manage errors associated with individual records.
Example of Processing JSON One By One
Consider a JSON array of user objects:
“`json
[
{“id”: 1, “name”: “Alice”},
{“id”: 2, “name”: “Bob”},
{“id”: 3, “name”: “Charlie”}
]
“`
The following example demonstrates how to process each user object in Python:
“`python
import json
Sample JSON data
data = ”’
[
{“id”: 1, “name”: “Alice”},
{“id”: 2, “name”: “Bob”},
{“id”: 3, “name”: “Charlie”}
]
”’
Load JSON data
users = json.loads(data)
Process each user one by one
for user in users:
print(f”Processing user ID: {user[‘id’]}, Name: {user[‘name’]}”)
Additional processing can be done here
“`
Processing JSON All In One
Processing JSON data all at once is advantageous when the entire dataset needs to be analyzed or transformed collectively. This approach is effective for operations that require a comprehensive understanding of the dataset as a whole.
Benefits of processing JSON all at once include:
- Batch Operations: Enables operations that affect multiple records simultaneously.
- Simplified Logic: Reduces the complexity of handling individual records by addressing the dataset in a unified manner.
- Performance: Can be faster for small to medium datasets, as it reduces the overhead of multiple function calls.
Example of Processing JSON All In One
Using the same user data, processing all records simultaneously can be done as follows:
“`python
import json
Sample JSON data
data = ”’
[
{“id”: 1, “name”: “Alice”},
{“id”: 2, “name”: “Bob”},
{“id”: 3, “name”: “Charlie”}
]
”’
Load JSON data
users = json.loads(data)
Process all users at once
for user in users:
user[‘active’] = True Mark all users as active
“`
Comparison of Approaches
Feature | One By One | All In One |
---|---|---|
Memory Usage | Lower, as only one record loaded | Higher, as all records loaded |
Error Handling | Easier to isolate errors | Harder to pinpoint issues |
Speed | Slower for large datasets | Faster for small to medium datasets |
Complexity | Higher complexity for logic | Simpler logic overall |
Both methods serve distinct purposes depending on the requirements of the task at hand. The choice between processing JSON one by one or all at once should align with the specific goals of the application or data manipulation task.
Evaluating JSON Processing Strategies: One by One or All at Once
Dr. Emily Carter (Data Architect, Tech Innovations Inc.). “Processing JSON one by one allows for greater control over data integrity and error handling. This method is particularly beneficial when dealing with large datasets where individual records may vary significantly in structure.”
Michael Chen (Senior Software Engineer, Cloud Solutions Corp.). “Batch processing JSON data all at once can lead to significant performance improvements, especially when the data is uniform. This approach minimizes overhead and can leverage parallel processing capabilities effectively.”
Jessica Patel (Lead Data Scientist, Analytics Hub). “The choice between processing JSON one by one or all at once should be guided by the specific use case. For real-time applications, one by one may be preferable, while for analytics and reporting, batch processing can yield faster results.”
Frequently Asked Questions (FAQs)
What does “process JSON one by one” mean?
Processing JSON one by one refers to handling individual JSON objects sequentially, allowing for focused manipulation or analysis of each object separately.
What is the advantage of processing JSON all in one?
Processing JSON all in one allows for batch operations, which can enhance performance by reducing overhead and enabling simultaneous handling of multiple objects, thereby improving efficiency.
When should I choose to process JSON one by one instead of all in one?
You should opt for processing JSON one by one when dealing with large datasets that require specific handling, such as validation or transformation of individual records, which may not be feasible in bulk.
Can processing JSON all in one lead to data loss or errors?
Yes, processing JSON all in one can lead to data loss or errors if a single object fails during processing, as it may affect the entire batch unless proper error handling mechanisms are implemented.
What tools or libraries can assist in processing JSON one by one or all in one?
Various programming languages offer libraries for JSON processing, such as Python’s `json` module, JavaScript’s `JSON` object, and Java’s Jackson library, which support both one-by-one and batch processing.
Is there a performance difference between processing JSON one by one and all in one?
Yes, processing JSON all in one typically offers better performance due to reduced function call overhead and optimized memory usage, while one-by-one processing may incur additional time for context switching and resource allocation.
In the realm of data processing, particularly when dealing with JSON (JavaScript Object Notation) formats, the choice between processing data one by one or all at once is a critical consideration. Each approach has its own set of advantages and drawbacks that can significantly impact the efficiency and performance of applications. Processing JSON data one by one allows for greater control and flexibility, especially in scenarios where individual records require distinct handling or validation. This method can enhance error handling, as developers can isolate issues with specific entries without affecting the entire dataset.
Conversely, processing all JSON data in one go can lead to improved performance, particularly when dealing with large volumes of data. This batch processing approach minimizes the overhead associated with multiple function calls and can leverage optimized algorithms for bulk operations. However, this method may introduce challenges in error management, as a single failure could compromise the integrity of the entire dataset. Therefore, careful consideration must be given to the nature of the data and the specific requirements of the application when choosing between these two methods.
Ultimately, the decision to process JSON data one by one or all at once should be informed by the specific use case, performance requirements, and the complexity of the data involved. By weighing the pros and cons of each approach
Author Profile
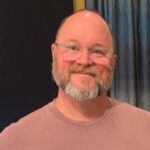
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?