How Can You Add Animation to Dropdowns in React-Bootstrap?
In the world of web development, user experience is paramount, and every interaction counts. One of the most common UI components is the dropdown menu, which provides users with a seamless way to navigate through options. However, with the rise of React and libraries like React-Bootstrap, developers are not just looking to create functional dropdowns; they want to enhance them with captivating animations that elevate the overall aesthetic and usability of their applications. Adding animation to dropdowns can transform a simple interaction into a delightful experience, making your application stand out in a crowded digital landscape.
In this article, we will explore the art of animating dropdown menus using React-Bootstrap, a popular library that combines the power of React with the elegance of Bootstrap. We’ll delve into the various techniques and best practices for integrating animations that not only capture attention but also maintain a smooth user experience. From subtle fades to dynamic slides, the possibilities are endless, and the impact can be significant.
Whether you’re a seasoned developer looking to refine your skills or a newcomer eager to learn the ropes, understanding how to incorporate animations into your dropdowns can greatly enhance your projects. Join us as we uncover the methods and tools available for creating visually engaging dropdowns that leave a lasting impression on your users.
Add Animation to Dropdowns with React-Bootstrap
To enhance user experience in applications, adding animations to dropdown components can be quite effective. React-Bootstrap, a popular library for integrating Bootstrap with React, does not natively support dropdown animations. However, you can achieve this effect using CSS transitions or animation libraries such as `react-transition-group`.
To implement animations for dropdowns, follow these steps:
- **Install the necessary packages** if you haven’t already:
“`bash
npm install react-bootstrap bootstrap react-transition-group
“`
- **Create a custom dropdown component** that wraps the standard React-Bootstrap dropdown and integrates animations.
Here’s an example of how you might set this up:
“`jsx
import React from ‘react’;
import { Dropdown } from ‘react-bootstrap’;
import { CSSTransition } from ‘react-transition-group’;
import ‘./DropdownAnimation.css’; // Import your CSS file for animations
const AnimatedDropdown = () => {
const [show, setShow] = React.useState();
const handleToggle = (nextShow) => {
setShow(nextShow);
};
return (
Dropdown Button
);
};
“`
- Create CSS animations in a separate CSS file (e.g., `DropdownAnimation.css`). Below is an example of how to define fade animations:
“`css
.fade-enter {
opacity: 0;
transform: translateY(-10px);
}
.fade-enter-active {
opacity: 1;
transform: translateY(0);
transition: opacity 300ms, transform 300ms;
}
.fade-exit {
opacity: 1;
transform: translateY(0);
}
.fade-exit-active {
opacity: 0;
transform: translateY(-10px);
transition: opacity 300ms, transform 300ms;
}
“`
- Usage of the component is straightforward. Just import and include `AnimatedDropdown` wherever needed in your application.
Advantages of Using Animation in Dropdowns
Implementing animations in dropdown menus can greatly improve the user interface. Here are several benefits:
- Enhanced User Engagement: Animations draw attention and create a more dynamic experience.
- Visual Feedback: Users receive immediate feedback on their actions (opening or closing the dropdown).
- Improved Perception of Speed: Properly timed animations can make interactions feel faster.
Considerations for Performance
When adding animations, it’s essential to keep performance in mind. Here are some considerations:
Consideration | Explanation |
---|---|
CSS vs. JavaScript | Prefer CSS transitions for smoother performance over JavaScript animations. |
Avoid heavy animations | Keep animations light to prevent jank during user interactions. |
Test on multiple devices | Ensure that animations perform well across different devices and browsers. |
By following these guidelines, you can effectively add animations to dropdowns in a React-Bootstrap application, enriching the overall user experience while maintaining performance.
Implementing Animation in React-Bootstrap Dropdowns
To add animation to dropdowns in React-Bootstrap, you can leverage CSS transitions or animation libraries like React Transition Group. This allows for a smoother user experience when the dropdown appears or disappears.
Using CSS Transitions
CSS transitions can be easily applied to React-Bootstrap dropdowns by customizing the styles. Here’s how you can implement this:
- **Define Your CSS**:
Create a CSS file (e.g., `dropdown.css`) with the following styles:
“`css
.dropdown-enter {
opacity: 0;
transform: translateY(-10px);
}
.dropdown-enter-active {
opacity: 1;
transform: translateY(0);
transition: opacity 300ms, transform 300ms;
}
.dropdown-exit {
opacity: 1;
transform: translateY(0);
}
.dropdown-exit-active {
opacity: 0;
transform: translateY(-10px);
transition: opacity 300ms, transform 300ms;
}
“`
- **Apply CSS in Your Component**:
Use the `Dropdown` component and apply the defined classes to manage the animations.
“`jsx
import React from ‘react’;
import { Dropdown } from ‘react-bootstrap’;
import ‘./dropdown.css’;
const AnimatedDropdown = () => {
return (
Dropdown Button
);
};
export default AnimatedDropdown;
“`
Using React Transition Group
For more complex animations, consider using React Transition Group. This library allows you to manage the lifecycle of transitions more effectively.
- **Install React Transition Group**:
Use npm or yarn to install the library.
“`bash
npm install react-transition-group
“`
- **Implementing the Transition**:
Wrap the `Dropdown.Menu` with `CSSTransition` from React Transition Group.
“`jsx
import React, { useState } from ‘react’;
import { Dropdown } from ‘react-bootstrap’;
import { CSSTransition } from ‘react-transition-group’;
import ‘./dropdown.css’;
const TransitionDropdown = () => {
const [show, setShow] = useState();
return (
Dropdown Button
);
};
export default TransitionDropdown;
“`
Animation Customization Options
You can customize your animations further by adjusting the CSS properties:
CSS Property | Description |
---|---|
`opacity` | Controls the transparency of the dropdown. |
`transform` | Allows for movement effects (e.g., slide). |
`transition` | Specifies the duration and timing function for the transitions. |
By adjusting these properties, you can create a variety of effects, such as fading, sliding, or bouncing animations, tailored to your design requirements.
Expert Insights on Adding Animation to Dropdowns in React-Bootstrap
Lisa Chen (Front-End Developer, CodeCraft). “Incorporating animations into dropdowns using React-Bootstrap enhances user experience by providing visual feedback. Utilizing CSS transitions alongside React’s state management can create smooth and engaging dropdown animations that delight users.”
James Patel (UI/UX Designer, Digital Innovations). “Animations in dropdown menus can significantly improve usability. It is essential to balance aesthetics with performance; therefore, lightweight animations should be prioritized to ensure they do not hinder the application’s responsiveness.”
Sarah Thompson (React Specialist, Tech Trends). “When adding animations to dropdowns in React-Bootstrap, leveraging libraries like React Spring or Framer Motion can simplify the process. These libraries offer powerful tools to create fluid animations that can be easily integrated into existing components.”
Frequently Asked Questions (FAQs)
How can I add animation to a dropdown in React-Bootstrap?
You can add animation to a dropdown in React-Bootstrap by using the `Transition` component. Wrap the dropdown items with the `Transition` component and specify the animation properties you want to apply.
What types of animations can be applied to dropdowns in React-Bootstrap?
You can apply various animations such as fade, slide, or custom CSS animations. Utilize CSS transitions or animations in conjunction with React-Bootstrap’s `Transition` component to achieve the desired effect.
Is it necessary to use CSS for dropdown animations in React-Bootstrap?
While React-Bootstrap provides basic transition capabilities, using CSS is recommended for more complex animations. Custom CSS can enhance the visual appeal and provide greater control over the animation effects.
Can I use third-party animation libraries with React-Bootstrap dropdowns?
Yes, you can integrate third-party animation libraries such as Framer Motion or React Spring with React-Bootstrap dropdowns. Ensure that the animation library is compatible with React components and properly manage the dropdown state.
Are there any performance considerations when adding animations to dropdowns?
Yes, excessive animations can impact performance, especially on lower-end devices. It’s advisable to keep animations simple and test performance across different devices to ensure a smooth user experience.
How do I control the timing of the dropdown animations in React-Bootstrap?
You can control the timing of animations by adjusting the `timeout` property in the `Transition` component. Additionally, you can use CSS to define the duration and easing of the animations for finer control.
Incorporating animation into dropdowns in React-Bootstrap can significantly enhance the user experience by providing visual feedback and making interactions more engaging. By utilizing CSS transitions or libraries like React Transition Group, developers can create smooth animations that draw attention to dropdown elements. This not only improves aesthetics but also helps users understand the state changes of the dropdown, whether it is opening or closing.
Key takeaways include the importance of selecting the right animation techniques that align with the overall design of the application. Simple fade or slide animations are often effective, as they do not overwhelm the user while still providing a noticeable effect. Additionally, ensuring that animations are performant and do not hinder usability is crucial, particularly on mobile devices where responsiveness is paramount.
Furthermore, developers should consider accessibility when adding animations. Providing options to disable animations for users who may be sensitive to motion can enhance inclusivity. Overall, adding animation to dropdowns in React-Bootstrap is a valuable enhancement that, when implemented thoughtfully, can lead to a more polished and user-friendly interface.
Author Profile
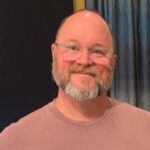
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?