How Can You Get the Maximum Date in SQL?
In the world of data management, the ability to extract meaningful insights from vast datasets is paramount. One common task that database professionals often encounter is the need to identify the maximum date within a dataset. Whether you’re tracking sales performance, monitoring user activity, or analyzing project timelines, determining the latest date can provide critical context and inform decision-making processes. This article delves into the methods and best practices for retrieving the maximum date in SQL, empowering you to harness the full potential of your data.
Understanding how to efficiently query for the maximum date is essential for anyone working with SQL databases. This operation not only aids in reporting and analytics but also enhances data integrity by ensuring that you’re working with the most current information. SQL offers various functions and techniques that can simplify this process, allowing users to navigate through complex datasets with ease.
As we explore the nuances of getting the maximum date in SQL, we’ll cover the different approaches available, from basic queries to more advanced techniques. By mastering these methods, you’ll be equipped to optimize your database queries and elevate your data analysis skills, ultimately leading to more informed business decisions and strategic insights. Join us as we unlock the secrets of SQL date manipulation and discover how to effectively retrieve the maximum date in your datasets.
Using MAX() Function
The `MAX()` function in SQL is a straightforward way to retrieve the maximum value from a specified column, which is particularly useful for finding the maximum date in a dataset. This function can be employed with date columns to determine the latest date in a specified table.
To use the `MAX()` function, the syntax is as follows:
“`sql
SELECT MAX(date_column) AS MaxDate
FROM table_name;
“`
This query will return the most recent date in the `date_column` of the specified `table_name`. The result can be aliased as `MaxDate` for better readability.
Example Query
Consider a table named `orders` with the following structure:
OrderID | OrderDate |
---|---|
1 | 2023-01-15 |
2 | 2023-03-22 |
3 | 2023-05-10 |
4 | 2022-12-01 |
To find the latest order date, you would execute:
“`sql
SELECT MAX(OrderDate) AS LatestOrderDate
FROM orders;
“`
The result will yield:
LatestOrderDate |
---|
2023-05-10 |
GROUP BY Clause with MAX()
The `MAX()` function can also be combined with the `GROUP BY` clause to retrieve the maximum date for each group within a dataset. This is particularly useful when analyzing data categorized by certain attributes.
For example, if the `orders` table also includes a `CustomerID` column, you can find the latest order date for each customer:
“`sql
SELECT CustomerID, MAX(OrderDate) AS LatestOrderDate
FROM orders
GROUP BY CustomerID;
“`
This query will return the most recent order date for each customer, allowing for detailed insights into customer activity.
Handling NULL Values
When using the `MAX()` function, it is important to be aware of how NULL values are handled. In SQL, NULL values are ignored by aggregate functions like `MAX()`. Therefore, if all values in the column are NULL, the result will also be NULL.
To ensure meaningful results, you might want to filter out NULLs before applying the `MAX()` function. This can be done using a `WHERE` clause:
“`sql
SELECT MAX(OrderDate) AS LatestOrderDate
FROM orders
WHERE OrderDate IS NOT NULL;
“`
Performance Considerations
When querying large datasets, the performance of the `MAX()` function can vary based on several factors:
- Indexing: Proper indexing on the date column can significantly enhance the speed of retrieval.
- Data Volume: The larger the dataset, the longer the query may take to execute, especially if it lacks appropriate indexing.
- Database Engine: Different SQL database engines may optimize aggregate functions differently.
By keeping these considerations in mind, you can write efficient SQL queries that effectively utilize the `MAX()` function to retrieve the latest dates in your datasets.
Understanding SQL Date Functions
SQL provides various date functions that can be utilized to extract and manipulate date values from a database. Key functions include:
- MAX(): This function returns the maximum value in a set of values, which can be applied to date columns to find the latest date.
- GROUP BY: Often used in conjunction with MAX() to aggregate results based on specific criteria.
- ORDER BY: This can sort results based on date values to identify the most recent entries.
Using MAX() to Get the Latest Date
To retrieve the maximum date from a specific column in a table, the MAX() function is commonly employed. The basic syntax for this operation is as follows:
“`sql
SELECT MAX(date_column) AS LatestDate
FROM table_name;
“`
Example
Suppose you have a table called `Orders` with a column `OrderDate`. To find the latest order date, you would execute:
“`sql
SELECT MAX(OrderDate) AS LatestOrderDate
FROM Orders;
“`
This query will return the most recent order date from the `Orders` table.
Combining MAX() with GROUP BY
When dealing with multiple groups, you can combine MAX() with GROUP BY to find the latest date per group. This is particularly useful in scenarios where data needs to be categorized.
Example
For instance, if you want to find the latest order date for each customer in the `Orders` table, the query would look like this:
“`sql
SELECT CustomerID, MAX(OrderDate) AS LatestOrderDate
FROM Orders
GROUP BY CustomerID;
“`
This query provides the latest order date for each customer.
Filtering Results with WHERE Clause
To refine your search for the maximum date, you can apply a WHERE clause to filter data before calculating the maximum.
Example
If you want to find the latest order date for a specific customer, you would write:
“`sql
SELECT MAX(OrderDate) AS LatestOrderDate
FROM Orders
WHERE CustomerID = ‘12345’;
“`
This will return the most recent order date only for the customer with ID ‘12345’.
Handling NULL Values
When working with date columns, it is essential to consider NULL values, as they can affect the results of the MAX() function. By default, NULL values are ignored in calculations. However, if all values in the column are NULL, the result of MAX() will also be NULL.
To ensure you are accounting for NULL values, you can use the COALESCE function to substitute a default date when necessary:
Example
“`sql
SELECT COALESCE(MAX(OrderDate), ‘No Orders’) AS LatestOrderDate
FROM Orders;
“`
This query will return ‘No Orders’ if there are no entries in the `OrderDate` column.
Performance Considerations
When querying large datasets for maximum dates, consider the following:
- Indexing: Ensure the date column is indexed to improve query performance.
- Database Design: Proper normalization can reduce the volume of data and enhance performance.
- Query Optimization: Analyze execution plans to identify potential bottlenecks.
By understanding these concepts, you can efficiently retrieve maximum date values in SQL while maintaining optimal performance and accuracy.
Expert Insights on Retrieving Maximum Dates in SQL
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “When working with SQL databases, the MAX() function is essential for retrieving the latest date in a dataset. It is crucial to ensure that the column being queried is indexed properly to optimize performance, especially in large datasets.”
James Patel (Senior Data Analyst, Insight Analytics Group). “Using MAX() in conjunction with GROUP BY can yield powerful insights. For example, retrieving the maximum order date per customer allows businesses to understand their most recent interactions, enhancing customer relationship management.”
Linda Martinez (SQL Consultant, Data Solutions LLC). “While the MAX() function is straightforward, it is important to handle NULL values appropriately. Utilizing COALESCE can ensure that your queries return meaningful results even when some records are missing date entries.”
Frequently Asked Questions (FAQs)
What is the SQL query to get the maximum date from a column?
To retrieve the maximum date from a date column in SQL, you can use the following query:
“`sql
SELECT MAX(date_column) AS MaxDate
FROM table_name;
“`
Can I use the MAX function with other data types in SQL?
The MAX function is primarily used with numeric, date, and string data types. It returns the highest value among the provided data types, but its behavior varies depending on the data type.
Does the MAX function consider NULL values in SQL?
The MAX function ignores NULL values when calculating the maximum. If all values are NULL, it will return NULL as the result.
How can I get the maximum date for a specific condition in SQL?
To obtain the maximum date based on specific conditions, you can use the WHERE clause in conjunction with the MAX function. For example:
“`sql
SELECT MAX(date_column) AS MaxDate
FROM table_name
WHERE condition;
“`
Is it possible to get the maximum date grouped by another column?
Yes, you can use the GROUP BY clause to get the maximum date for each group. For example:
“`sql
SELECT group_column, MAX(date_column) AS MaxDate
FROM table_name
GROUP BY group_column;
“`
What happens if there are duplicate maximum dates in SQL?
If there are duplicate maximum dates, the MAX function will still return that maximum date once. To see all occurrences, you may need to use a different approach, such as a subquery or a common table expression (CTE).
In SQL, retrieving the maximum date from a dataset is a common requirement for various analytical and reporting tasks. The primary function used for this purpose is the `MAX()` aggregate function, which efficiently identifies the highest value in a specified column. This function can be applied in conjunction with the `SELECT` statement to extract the maximum date from a table, ensuring that users can quickly access the most recent entry in their datasets.
Additionally, it is essential to consider the context in which the maximum date is being retrieved. For instance, filtering the results using the `WHERE` clause can help narrow down the dataset to specific conditions, allowing for more targeted analysis. Furthermore, when working with grouped data, the `GROUP BY` clause can be utilized alongside `MAX()` to obtain the maximum date for each group, providing deeper insights into trends over time.
In summary, mastering the use of the `MAX()` function in SQL is crucial for effective data analysis. By understanding how to implement this function within various query structures, users can enhance their ability to derive meaningful insights from their data. This knowledge not only improves data retrieval efficiency but also supports informed decision-making based on the most current information available.
Author Profile
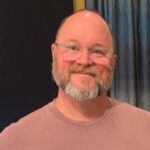
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?