Why Does Java Fail to Validate Certificates and How Can You Fix It?
In an increasingly interconnected world, the security of online communications has never been more critical. Java, a widely used programming language, plays a pivotal role in building robust applications that often rely on secure connections. However, developers and users alike can encounter frustrating issues when Java fails to validate certificates, leading to disruptions in functionality and potential security vulnerabilities. Understanding the intricacies of certificate validation in Java is essential for anyone looking to maintain the integrity and reliability of their applications.
At its core, certificate validation is a crucial process that ensures the authenticity and integrity of data exchanged over networks. When Java applications attempt to establish secure connections, they rely on certificates to verify the identity of servers and clients. Unfortunately, various factors can lead to validation failures, including expired certificates, untrusted certificate authorities, or misconfigured security settings. These issues not only hinder application performance but can also expose systems to security risks if left unaddressed.
As we delve deeper into the topic, we will explore the common causes behind Java’s failure to validate certificates and provide insights into effective troubleshooting strategies. By equipping yourself with the knowledge to navigate these challenges, you can enhance your application’s security posture and ensure seamless communication across your network. Whether you’re a seasoned developer or just starting your journey in Java programming, understanding certificate validation is a
Understanding Certificate Validation in Java
Java’s certificate validation process is integral to ensuring secure communications over networks, particularly when using SSL/TLS. The Java Secure Socket Extension (JSSE) handles this process by verifying that the certificates presented by servers are valid and trustworthy. A failure to validate a certificate can stem from several issues, including misconfigured trust stores, expired certificates, or untrusted certificate authorities (CAs).
Key components involved in the certificate validation process include:
- Trust Store: A repository of trusted certificates used by Java applications to validate certificates presented by external entities.
- Keystore: A storage facility for cryptographic keys and certificates, often used in conjunction with the trust store.
- Certificate Authorities (CAs): Trusted entities that issue digital certificates, which are used to establish the identity of the certificate holder.
Common Causes of Certificate Validation Failures
Several common issues can lead to Java failing to validate certificates:
- Expired Certificates: Certificates have a specific validity period. Once expired, they are no longer considered valid.
- Untrusted Certificate Authority: If the certificate is signed by a CA not present in the Java trust store, validation will fail.
- Self-Signed Certificates: These certificates are not signed by a trusted CA and are often rejected unless explicitly added to the trust store.
- Incorrectly Configured Trust Store: If the trust store does not contain the necessary certificates or is not properly referenced in the Java application, validation errors will occur.
- Hostname Mismatch: The certificate’s Common Name (CN) or Subject Alternative Name (SAN) must match the hostname to which the connection is being made.
Configuration Steps for Trust Store
To ensure proper certificate validation, it is essential to configure the trust store correctly. Below are steps to configure the trust store in a Java application:
- Create a Trust Store: Use the `keytool` command to create a new trust store and import necessary certificates.
“`bash
keytool -import -alias
- Specify the Trust Store in Java: When running a Java application, specify the trust store and its password using system properties.
“`bash
java -Djavax.net.ssl.trustStore=
“`
- Verify the Trust Store Contents: Use the `keytool` command to list the contents of the trust store, ensuring all required certificates are present.
“`bash
keytool -list -keystore
Handling Certificate Validation Errors
When encountering certificate validation errors, the following strategies can be employed:
- Review Application Logs: Check application logs for specific error messages related to SSL connections.
- Update Trust Store: Ensure that the trust store is up-to-date with all necessary certificates, including those from CAs.
- Debug SSL Connections: Enable SSL debugging by adding the following JVM argument to get detailed logs about the SSL handshake.
“`bash
-Djavax.net.debug=ssl
“`
- Use a Valid Certificate: If using self-signed certificates, consider transitioning to a CA-signed certificate to avoid trust issues.
Issue | Possible Solution |
---|---|
Expired Certificate | Renew the certificate |
Untrusted CA | Add CA certificate to trust store |
Self-Signed Certificate | Import into trust store |
Hostname Mismatch | Ensure correct hostname is used |
Common Causes of Certificate Validation Failures
Several factors can contribute to Java’s inability to validate a certificate. Understanding these causes can assist in troubleshooting the issue effectively.
- Untrusted Certificate Authority (CA): The root certificate may not be present in the Java truststore.
- Expired Certificates: Certificates that have surpassed their validity period will lead to validation errors.
- Hostname Mismatch: The domain name in the URL must match the Common Name (CN) or Subject Alternative Name (SAN) in the certificate.
- Self-Signed Certificates: These certificates are not validated against a CA and require additional configuration to be trusted.
- Intermediate Certificates Missing: If the server does not send all necessary intermediate certificates, clients may fail to validate the chain.
Resolving Certificate Validation Issues
To address the validation issues, implement the following steps:
- Import the Certificate into the Truststore:
- Obtain the certificate file (e.g., `server.crt`).
- Use the `keytool` command to import it:
“`bash
keytool -import -alias mycert -file server.crt -keystore cacerts
“`
- Ensure the correct path to the `cacerts` file (typically found in the JRE’s `lib/security` directory).
- Update the Truststore:
- If the root CA is not present, you will need to add it to the truststore using the same `keytool` command.
- Check Certificate Validity:
- Use the following command to check the certificate’s validity:
“`bash
openssl x509 -in server.crt -noout -dates
“`
- Verify Hostname:
- Ensure that the hostname matches the certificate’s CN or SAN. You can view the certificate details using:
“`bash
openssl x509 -in server.crt -text -noout
“`
- Use a Trusted CA:
- Whenever possible, obtain certificates from trusted Certificate Authorities to avoid self-signed certificate issues.
Java Truststore Management
Java utilizes a truststore to maintain a list of trusted certificates. Proper management of the truststore is essential for smooth SSL/TLS operations.
Action | Command/Description |
---|---|
List Certificates | `keytool -list -keystore cacerts` |
Export Certificate | `keytool -export -alias mycert -file exported.crt -keystore cacerts` |
Delete a Certificate | `keytool -delete -alias mycert -keystore cacerts` |
Change Truststore Location | Use the `-Djavax.net.ssl.trustStore` system property to specify a custom truststore. |
Debugging SSL Issues in Java
To gain insights into SSL-related problems, enable detailed logging by adding the following JVM options:
- `-Djavax.net.debug=ssl:handshake:verbose`
- `-Djavax.net.debug=all`
This will provide comprehensive output regarding the SSL handshake process, which can be invaluable for troubleshooting certificate validation failures.
Expert Insights on Java Certificate Validation Issues
Dr. Emily Carter (Cybersecurity Analyst, SecureTech Solutions). “Java’s failure to validate certificates often stems from misconfigured trust stores or outdated certificate authorities. It is crucial for developers to ensure that their Java environment is up-to-date and that the trust store contains the necessary root certificates.”
Michael Chen (Lead Java Developer, Tech Innovations Inc.). “When encountering certificate validation failures in Java, developers should first check the certificate chain for any missing intermediate certificates. Implementing proper error handling can also provide insights into where the validation process is breaking down.”
Lisa Thompson (Software Security Consultant, CodeGuardians). “Understanding the Java Secure Socket Extension (JSSE) is essential for troubleshooting certificate validation issues. Developers must be aware of how to configure SSL contexts correctly to prevent these errors from occurring.”
Frequently Asked Questions (FAQs)
What does “Java Failed To Validate Certificate” mean?
This error indicates that Java encountered an issue when attempting to validate a digital certificate, often due to an untrusted certificate authority or an expired certificate.
What are common causes of certificate validation failures in Java?
Common causes include using self-signed certificates, expired certificates, missing root or intermediate certificates in the trust store, or incorrect certificate chain configurations.
How can I resolve the “Java Failed To Validate Certificate” error?
To resolve this error, ensure that the certificate is valid, install the necessary root or intermediate certificates in the Java trust store, or configure your application to trust the specific certificate.
Where can I find the Java trust store?
The default Java trust store is usually located in the `lib/security/cacerts` file within the Java installation directory. You can also specify a custom trust store using the `-Djavax.net.ssl.trustStore` system property.
How do I import a certificate into the Java trust store?
You can import a certificate using the `keytool` command-line utility. The command typically looks like this: `keytool -import -alias
What should I do if I encounter this error in a production environment?
In a production environment, ensure that all certificates are properly signed by a trusted certificate authority, regularly update your trust store, and monitor for certificate expiration to prevent service interruptions.
The issue of “Java Failed To Validate Certificate” typically arises when Java applications attempt to establish secure connections over HTTPS but encounter problems with SSL/TLS certificates. This failure can stem from various factors, including expired certificates, untrusted certificate authorities, or improperly configured Java keystores. Understanding the underlying causes is crucial for developers and system administrators to ensure secure communications in their applications.
One of the primary reasons for certificate validation failures is the use of self-signed certificates or certificates from untrusted sources. Java maintains a truststore that contains a list of trusted certificate authorities. If the certificate presented by the server is not in this truststore, Java will reject the connection. Therefore, it is essential to ensure that all certificates are properly signed by a trusted authority or to manually import self-signed certificates into the Java truststore when necessary.
Another common issue is the mismatch between the hostname and the certificate’s subject name. When a certificate is issued, it is tied to a specific domain name. If the application attempts to connect to a different domain, the validation will fail. Developers should ensure that the certificate matches the domain being accessed to avoid this pitfall.
addressing the “Java Failed To Validate Certificate” error
Author Profile
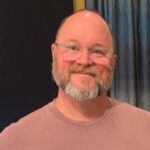
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?