Which of the Following Methods Outputs Data in a Python Program?
In the world of programming, the ability to communicate effectively with users is paramount. For Python developers, this communication often takes the form of outputting data—whether it’s displaying results, logging information, or providing feedback. Understanding the various methods available for outputting data in a Python program is essential for creating effective and user-friendly applications. In this article, we will explore the different techniques and tools that Python offers for data output, helping you enhance your coding skills and improve your programs’ interactivity.
When we talk about outputting data in Python, we are essentially discussing how to present information in a way that is meaningful and accessible to users. Python provides several built-in functions and libraries that facilitate this process, allowing developers to choose the most suitable method based on their specific needs. From simple console prints to more complex graphical user interfaces, the options are as diverse as the applications themselves.
Moreover, understanding the nuances of data output can significantly impact the user experience. Whether you are debugging a program or creating a polished final product, knowing how to effectively convey information can make all the difference. In the following sections, we will delve into the various output methods available in Python, equipping you with the knowledge to make informed choices in your programming journey.
Output Functions in Python
In Python, several functions and methods can be utilized to output data to the console or other output streams. The most commonly used function for this purpose is `print()`, but there are other methods and techniques that also serve to display or write data.
The Print Function
The `print()` function is the cornerstone of output in Python. It converts the specified objects into a string format and writes them to the standard output device (usually the console). The function can take multiple arguments and has various parameters that allow customization of the output.
- Basic Usage:
“`python
print(“Hello, World!”)
“`
- Multiple Arguments:
“`python
name = “Alice”
age = 30
print(“Name:”, name, “Age:”, age)
“`
- Custom Separator:
You can change the default separator (which is a space) by using the `sep` parameter.
“`python
print(“Hello”, “World”, sep=”-“)
“`
- Custom End Character:
The `end` parameter allows you to specify what to print at the end of the output.
“`python
print(“Hello”, end=”!”)
print(“World”)
“`
Writing to Files
In addition to console output, Python allows writing data to files, which can be beneficial for logging or storing information. The built-in `open()` function, combined with the file object’s `write()` method, facilitates this process.
- Example of Writing to a File:
“`python
with open(‘output.txt’, ‘w’) as file:
file.write(“This is a line of text.\n”)
“`
Here, the `with` statement is used to ensure that the file is properly closed after its suite finishes, even if an error is raised.
Formatted Output
For more complex output requirements, Python offers formatted string literals (f-strings) and the `format()` method.
- Using F-strings:
F-strings are a concise way to embed expressions inside string literals.
“`python
name = “Alice”
age = 30
print(f”{name} is {age} years old.”)
“`
- Using Format Method:
The `format()` method can also be used for formatting strings.
“`python
print(“{} is {} years old.”.format(name, age))
“`
Outputting Data Structures
When outputting complex data structures like lists and dictionaries, the `pprint` module can enhance readability. This module provides a capability to “pretty-print” arbitrary Python data structures.
- Example:
“`python
import pprint
data = {‘name’: ‘Alice’, ‘age’: 30, ‘hobbies’: [‘reading’, ‘hiking’]}
pprint.pprint(data)
“`
Output Comparison Table
The following table summarizes different output methods in Python:
Method | Description | Example |
---|---|---|
print() | Outputs data to console | print(“Hello, World!”) |
write() | Writes data to a file | file.write(“Hello”) |
pprint.pprint() | Pretty-prints data structures | pprint.pprint(data) |
f-strings | Formatted string literals | f”{name} is {age}” |
format() | Formats strings | “{} is {}”.format(name, age) |
These output methods provide a robust toolkit for displaying data in Python, allowing developers to choose the most appropriate method based on their specific needs.
Common Methods to Output Data in Python
In Python, there are several built-in functions and methods to output data. The most commonly used methods include:
- print() Function: This is the most straightforward way to output data to the console. It can take multiple arguments and format them as needed.
“`python
print(“Hello, World!”)
print(“The value is:”, 42)
“`
- Formatted Strings (f-strings): Introduced in Python 3.6, f-strings allow for inline expression evaluation and make string formatting simpler and more readable.
“`python
name = “Alice”
age = 30
print(f”{name} is {age} years old.”)
“`
- str.format() Method: This method provides a way to format strings using placeholders.
“`python
name = “Bob”
score = 95
print(“{} scored {} points”.format(name, score))
“`
- % Formatting: An older method of string formatting that uses the `%` operator. While less common in recent code, it is still found in legacy systems.
“`python
name = “Charlie”
balance = 200.50
print(“%s has a balance of $%.2f” % (name, balance))
“`
Outputting Data to Files
In addition to console output, Python can also write data to files. Common methods for file output include:
- Writing with open(): The `open()` function, combined with methods like `.write()` or `.writelines()`, allows for writing data to files.
“`python
with open(‘output.txt’, ‘w’) as f:
f.write(“This is a line of text.\n”)
f.writelines([“Line 1\n”, “Line 2\n”])
“`
- Using JSON: The `json` module can be used to output data in JSON format, which is useful for data interchange.
“`python
import json
data = {‘name’: ‘David’, ‘age’: 25}
with open(‘data.json’, ‘w’) as f:
json.dump(data, f)
“`
Outputting Data to Other Formats
Python provides libraries for outputting data in various formats, including CSV and XML.
- CSV Output: The `csv` module facilitates writing data to CSV files.
“`python
import csv
data = [[‘Name’, ‘Age’], [‘Eve’, 22], [‘Frank’, 29]]
with open(‘data.csv’, ‘w’, newline=”) as f:
writer = csv.writer(f)
writer.writerows(data)
“`
- XML Output: The `xml.etree.ElementTree` module allows for creating XML documents.
“`python
import xml.etree.ElementTree as ET
root = ET.Element(“people”)
person = ET.SubElement(root, “person”)
person.set(“name”, “Grace”)
person.set(“age”, “28”)
tree = ET.ElementTree(root)
tree.write(“data.xml”)
“`
Using Logging for Output
For more advanced output requirements, especially in production code, the `logging` module is often employed. It provides a flexible framework for emitting log messages from Python programs.
- Basic Logging Example:
“`python
import logging
logging.basicConfig(level=logging.INFO)
logging.info(“This is an info message.”)
logging.error(“This is an error message.”)
“`
This method allows for outputting to different destinations (console, files) and supports varying levels of importance (DEBUG, INFO, WARNING, ERROR, CRITICAL).
Understanding Data Output Methods in Python Programming
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, the primary method for outputting data is through the built-in `print()` function, which allows developers to display strings, numbers, and other data types in the console. Mastery of this function is essential for effective debugging and user interaction.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Beyond `print()`, Python also supports outputting data to files using the `write()` method of file objects. This is crucial for applications that require data persistence, enabling developers to store results for future access.”
Sarah Patel (Data Scientist, Analytics Hub). “For more complex data visualization, libraries such as Matplotlib and Seaborn provide functions to output graphical representations of data. This capability is vital for data analysis and presentation, allowing insights to be communicated effectively.”
Frequently Asked Questions (FAQs)
Which of the following functions outputs data in a Python program?
The `print()` function is the primary method used to output data to the console in a Python program.
Can I output data to a file in Python?
Yes, you can output data to a file using the `write()` method of file objects after opening a file in write mode.
What is the difference between `print()` and `return` in Python?
The `print()` function displays data to the console, while `return` sends a value back to the caller of a function, terminating that function’s execution.
How can I format the output of data in Python?
You can format output using f-strings, the `format()` method, or the `%` operator to control the appearance of the data being printed.
Is it possible to output data to a graphical user interface (GUI) in Python?
Yes, you can output data to a GUI using libraries such as Tkinter, PyQt, or Kivy, which provide widgets for displaying information.
Can I output data in different formats, like JSON or CSV, in Python?
Yes, Python has built-in libraries like `json` and `csv` that allow you to output data in JSON and CSV formats, respectively.
In Python programming, outputting data is a fundamental aspect that allows developers to communicate results, display information, and facilitate user interaction. Various methods exist for outputting data, with the most common being the `print()` function. This built-in function is versatile and can handle multiple data types, making it an essential tool for debugging and presenting results in a readable format.
Additionally, Python provides other mechanisms for outputting data, such as writing to files using the `write()` method or utilizing libraries like `pandas` for data visualization. Each method serves different purposes and can be chosen based on the specific requirements of the program. Understanding these options is crucial for effective data presentation and manipulation in Python.
Overall, mastering the various ways to output data in Python enhances a programmer’s ability to create user-friendly applications and effectively convey information. By leveraging these output techniques, developers can ensure that their programs are not only functional but also accessible and informative for end-users.
Author Profile
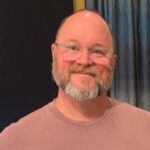
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?