How Can You Import Functions from Another File in Python?
In the world of programming, efficiency and organization are key to writing clean, maintainable code. As projects grow in complexity, the need to structure your code into manageable segments becomes paramount. One of the most effective ways to achieve this in Python is by importing functions from other files. This not only helps in keeping your code DRY (Don’t Repeat Yourself) but also enhances readability and collaboration among developers. Whether you’re building a small script or a large application, mastering the art of importing functions can significantly streamline your workflow and elevate your coding skills.
When you import functions from another file in Python, you’re essentially leveraging the power of modular programming. This approach allows you to break down your code into distinct components, each responsible for a specific task. By doing so, you can easily reuse code across different projects, making your development process more efficient. Additionally, it fosters a collaborative environment where multiple developers can work on different parts of a project simultaneously without stepping on each other’s toes.
Understanding how to import functions effectively opens up a world of possibilities. You can create libraries of reusable code, share your functions with others, and even utilize third-party modules to enhance your projects. In this article, we will delve into the various methods of importing functions in Python, explore best practices, and
Using the `import` Statement
To import functions from another file in Python, you primarily use the `import` statement. This allows you to access functions, classes, and variables defined in another module. The basic syntax is as follows:
“`python
import module_name
“`
After importing, you can call functions using the module name as a prefix:
“`python
module_name.function_name()
“`
Importing Specific Functions
If you want to import specific functions from a module, you can use the `from` keyword. This approach makes the functions directly accessible without needing to prefix them with the module name.
“`python
from module_name import function_name
“`
This method allows you to call the function directly:
“`python
function_name()
“`
You can also import multiple functions by separating them with commas:
“`python
from module_name import function_one, function_two
“`
Using Aliases
Sometimes, it is beneficial to use aliases to avoid name conflicts or to simplify function calls. You can create an alias for a module or a specific function using the `as` keyword. For example:
“`python
import module_name as alias_name
“`
To create an alias for a specific function:
“`python
from module_name import function_name as alias_function
“`
This allows you to call the function using the alias:
“`python
alias_function()
“`
Example Structure of Python Files
Consider the following example where you have two Python files: `math_functions.py` and `main.py`.
math_functions.py
“`python
def add(a, b):
return a + b
def subtract(a, b):
return a – b
“`
main.py
“`python
from math_functions import add
result = add(5, 3)
print(result) Outputs: 8
“`
Table of Import Styles
Import Style | Example | Usage |
---|---|---|
Import whole module | import math | Access all functions with module prefix |
Import specific function | from math import sqrt | Direct access to function |
Import with alias | import math as m | Shorter prefix for convenience |
Import multiple functions | from math import sin, cos | Access multiple functions directly |
Best Practices for Importing
- Organize Imports: Keep your import statements at the top of your file for clarity.
- Avoid Circular Imports: Be cautious of circular imports, as they can lead to errors.
- Use Absolute Imports: Prefer absolute imports over relative imports for better readability.
- Limit Imports: Only import the functions or classes you need to keep your namespace clean.
By following these guidelines, you can effectively manage and utilize functions across different Python files, enhancing the modularity and maintainability of your code.
Using the Import Statement
In Python, the primary method for importing functions from another file is by using the `import` statement. This allows for modular programming, enabling code reuse and better organization.
- Basic Syntax:
“`python
import module_name
“`
In this case, `module_name` is the name of the Python file (without the `.py` extension) that contains the functions you want to import.
Importing Specific Functions
If you only need specific functions from a module, you can import them directly using the following syntax:
- Specific Import:
“`python
from module_name import function_name
“`
This method allows you to call the function directly without prefixing it with the module name.
Importing Multiple Functions
To import multiple functions from a module, you can list them in parentheses or separate them with commas.
- Multiple Imports:
“`python
from module_name import function1, function2
“`
- Using Parentheses:
“`python
from module_name import (
function1,
function2,
function3
)
“`
Renaming Imports
You can also rename functions or modules during import to avoid naming conflicts or for convenience.
- Renaming a Function:
“`python
from module_name import function_name as fn
“`
This allows you to call the function with the alias `fn`.
Importing All Functions
To import all functions from a module, the asterisk (`*`) can be used. However, use this approach sparingly as it can lead to unclear code.
- Import All:
“`python
from module_name import *
“`
While convenient, this can cause namespace pollution, making it difficult to track which functions belong to which module.
Handling Import Errors
When importing functions, errors may arise if the module or function does not exist. It is good practice to handle such exceptions.
- Try-Except Block:
“`python
try:
from module_name import function_name
except ImportError:
print(“Module or function does not exist.”)
“`
This ensures that your program does not crash and can handle the error gracefully.
Relative Imports
In a package structure, relative imports can be used to import functions from sibling or parent modules. This is particularly useful in larger projects.
- Relative Import Example:
“`python
from . import sibling_module
from ..parent_module import function_name
“`
Using a dot (`.`) signifies the current package level, while double dots (`..`) indicate moving up one level.
Best Practices for Importing Functions
To maintain clean and efficient code, consider the following best practices:
- Import only what you need.
- Use absolute imports for clarity.
- Organize imports at the top of your files.
- Avoid wildcard imports to prevent namespace conflicts.
- Keep related imports grouped together for readability.
By following these guidelines, you can enhance code maintainability and readability across your projects.
Expert Insights on Importing Functions in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “Importing functions from another file in Python is a fundamental skill that enhances code modularity and reusability. Utilizing the `import` statement correctly can streamline your workflow and make your codebase more maintainable.”
James Liu (Software Engineer, Open Source Advocate). “When importing functions, it is crucial to understand the difference between absolute and relative imports. This knowledge will help you avoid common pitfalls, especially in larger projects where module paths can become complex.”
Sarah Thompson (Python Educator, Code Academy). “For beginners, I recommend starting with simple import statements like `from module_name import function_name`. This approach not only clarifies the source of each function but also encourages a deeper understanding of Python’s module system.”
Frequently Asked Questions (FAQs)
How do I import a function from another Python file?
To import a function from another Python file, use the `import` statement followed by the filename (without the `.py` extension) and the function name. For example, if you have a file named `my_functions.py` with a function `my_function`, you can import it using `from my_functions import my_function`.
Can I import multiple functions from the same file?
Yes, you can import multiple functions from the same file by separating the function names with commas. For instance, `from my_functions import function_one, function_two` allows you to use both functions in your current file.
What if the file I want to import is in a different directory?
If the file is in a different directory, you need to ensure that the directory is included in your Python path. You can do this by modifying the `sys.path` list or by using relative imports if the files are part of a package.
Is it possible to import all functions from a file?
Yes, you can import all functions from a file using the asterisk (`*`) syntax. For example, `from my_functions import *` imports all public functions and variables from `my_functions.py`. However, this practice is generally discouraged due to potential naming conflicts.
What are the differences between `import` and `from … import`?
Using `import` imports the entire module, requiring you to prefix functions with the module name (e.g., `my_functions.my_function()`). In contrast, `from … import` allows direct access to the function without the module prefix, making the code cleaner but potentially leading to naming conflicts.
Can I import functions conditionally in Python?
Yes, you can import functions conditionally by placing the import statement inside a function or a conditional block. This allows for dynamic imports based on certain conditions, which can be useful for optimizing performance or managing dependencies.
In Python, importing functions from another file is a fundamental practice that enhances code organization and reusability. By utilizing the `import` statement, developers can access functions defined in separate modules, allowing for cleaner and more manageable codebases. This practice not only streamlines development but also fosters collaboration among team members, as functions can be shared and reused across different projects.
There are several methods to import functions, including using the `import` statement to bring in an entire module or employing `from module import function` to import specific functions directly. Each method has its use cases and can be chosen based on the needs of the project. Additionally, understanding the importance of module paths and the Python search path can greatly aid in effectively managing imports, especially in larger applications.
Key takeaways from the discussion include the significance of maintaining a modular structure in programming, which leads to improved code readability and maintenance. It is also essential to be mindful of naming conflicts and to utilize aliases when necessary to avoid ambiguity. Overall, mastering the import functionality in Python is crucial for any developer aiming to write efficient and scalable code.
Author Profile
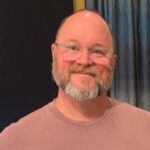
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?