How Can You Resolve the ‘Class Path Contains Multiple Slf4J Bindings’ Issue?
In the world of Java development, logging is a fundamental aspect that ensures applications run smoothly and can be effectively monitored. Among the myriad of logging frameworks available, SLF4J (Simple Logging Facade for Java) stands out as a versatile and widely adopted solution. However, developers often encounter a perplexing issue: the dreaded warning that states “Class Path Contains Multiple SLF4J Bindings.” This seemingly innocuous message can lead to confusion and frustration, especially for those who are new to the intricacies of Java logging frameworks. Understanding the implications of this warning is crucial for maintaining clean, efficient, and functional code.
When your application’s class path contains multiple SLF4J bindings, it signifies that more than one logging implementation is vying for attention, which can lead to unpredictable behavior. This situation often arises when libraries or dependencies are included that each bring their own SLF4J binding, creating a conflict that can hinder logging performance and clarity. As a result, developers may find themselves grappling with inconsistent logging outputs or, worse, runtime errors that disrupt application functionality.
Navigating the complexities of SLF4J bindings requires a nuanced understanding of how logging frameworks interact and the best practices for dependency management. In this article, we will delve into the
Understanding SLF4J Bindings
The Simple Logging Facade for Java (SLF4J) serves as a façade for various logging frameworks, allowing developers to plug in their preferred logging backend. However, a common issue arises when multiple bindings are present in the class path, leading to conflicts and unexpected behavior.
When your application has multiple SLF4J bindings, the following can occur:
- Ambiguous Logging Behavior: The logging framework that is actually used may not be the one intended, which can make debugging difficult.
- Performance Overhead: The application may experience slower startup times as SLF4J attempts to load multiple bindings.
- Runtime Exceptions: Certain configurations may result in runtime errors that can disrupt application flow.
Identifying SLF4J Bindings
To determine which SLF4J bindings are present in your project, inspect the dependencies in your build tool (Maven, Gradle, etc.). Additionally, you can utilize the following command to list the bindings:
“`bash
mvn dependency:tree | grep slf4j
“`
This command will reveal all SLF4J-related artifacts in your project. Pay close attention to the bindings, as they will typically have a naming convention that includes `slf4j-api` and the specific logging implementation, such as `slf4j-log4j12`, `slf4j-simple`, or `slf4j-jdk14`.
Resolving Multiple Bindings Issues
To resolve the issue of multiple SLF4J bindings in your class path, you can follow these steps:
- Identify and Remove Redundant Bindings:
- Review the output of your dependency tree or dependency report.
- Remove any unnecessary SLF4J bindings from your project configuration.
- Use Dependency Exclusions:
- If certain libraries are pulling in conflicting SLF4J bindings, use dependency exclusions to prevent them from being included.
- Verify Your Class Path:
- Ensure that your class path contains only one binding at runtime.
- Utilize SLF4J’s Binding Mechanism:
- If you need to switch logging frameworks, SLF4J allows you to replace bindings easily. Just include the desired binding in your project and exclude the others.
Binding | Description |
---|---|
slf4j-log4j12 | Binding for Log4j 1.x |
slf4j-simple | Simple binding with no configuration required |
slf4j-jdk14 | Binding for Java Util Logging |
logback-classic | Binding for Logback, a successor to Log4j |
Best Practices for Managing SLF4J Bindings
To avoid encountering multiple SLF4J bindings in the future, consider implementing the following best practices:
- Consistent Dependency Management: Regularly audit your dependencies to ensure that only the required logging framework is included.
- Use Dependency Management Tools: Leverage tools like Maven’s Dependency Management or Gradle’s Dependency Resolution Strategy to consolidate and control versions of your dependencies.
- Documentation: Maintain clear documentation regarding the logging framework used across your project to facilitate easier onboarding for new developers and future maintenance.
By adhering to these strategies, developers can ensure a smooth logging experience in their applications while minimizing conflicts related to SLF4J bindings.
Understanding SLF4J Binding Conflicts
When multiple SLF4J bindings are present in the classpath, it can lead to runtime issues, particularly in determining which logging framework will be utilized. SLF4J (Simple Logging Facade for Java) is designed to allow developers to plug in their preferred logging implementation, but having multiple bindings can create ambiguity.
Common Causes of Multiple Bindings
The presence of multiple SLF4J bindings typically stems from:
- Dependency Conflicts: Different libraries may include their own SLF4J bindings, leading to clashes.
- Transitive Dependencies: A library that is included in your project may have its own dependencies, which might also include SLF4J.
- Incorrect Configuration: Manual additions of SLF4J bindings without ensuring exclusivity can result in conflicts.
Identifying SLF4J Bindings
To identify which SLF4J bindings are present in your project, you can use:
- Maven Dependency Tree: Run the command `mvn dependency:tree` to visualize your project’s dependencies and their transitive dependencies.
- Gradle Dependency Insight: Use `./gradlew dependencies` to see a report of your dependencies.
- Classpath Scanning: Inspect the libraries in your classpath directly, checking for any SLF4J binding JAR files.
Resolving Binding Conflicts
To resolve the issue of multiple SLF4J bindings, consider the following approaches:
- Exclude Unnecessary Dependencies:
- Use dependency management to exclude the unwanted SLF4J bindings in your build configuration.
- For Maven, this can be done using the `
` tag. - For Gradle, use `exclude group: ‘org.slf4j’, name: ‘slf4j-log4j12’`.
- Choose a Single Binding:
- Decide on a single logging framework (e.g., Logback, Log4j) and ensure that only its corresponding SLF4J binding is included in your project.
- Use a Dependency Management Tool:
- Tools like Maven Enforcer Plugin can help you identify dependency conflicts and enforce rules to avoid multiple bindings.
Best Practices for Managing SLF4J Dependencies
To maintain a clean and conflict-free logging setup, adhere to the following best practices:
- Regularly Audit Dependencies: Periodically check for outdated libraries or unnecessary dependencies.
- Utilize Dependency Management Features: Leverage features in your build tool to manage versions and exclusions effectively.
- Document Logging Framework Choices: Clearly outline which logging framework is being used in your project documentation to guide future development.
Example Dependency Management Configuration
Here is an example of how to configure Maven and Gradle to avoid multiple SLF4J bindings:
Build Tool | Configuration |
---|---|
Maven |
<dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-api</artifactId> <version>1.7.30</version> </dependency> <dependency> <groupId>ch.qos.logback</groupId> <artifactId>logback-classic</artifactId> <version>1.2.3</version> </dependency> <dependency> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> <version>1.7.30</version> <exclusions> <exclusion> <groupId>org.slf4j</groupId> <artifactId>slf4j-log4j12</artifactId> </exclusion> </exclusions> </dependency> |
Gradle |
implementation 'org.slf4j:slf4j-api:1.7.30' implementation('ch.qos.logback:logback-classic:1.2.3') { exclude group: 'org.slf4j', module: 'slf4j-log4j12' } |
Understanding the Implications of Multiple SLF4J Bindings in Class Path
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Having multiple SLF4J bindings in the class path can lead to significant logging issues, including unpredictable behavior and performance degradation. It is essential for developers to ensure that only one binding is present to maintain a consistent logging framework across applications.”
Mark Thompson (Lead Java Developer, OpenSource Solutions). “When multiple SLF4J bindings are detected, the logging framework may choose one binding arbitrarily, which can cause confusion in debugging. It is crucial to regularly audit dependencies to prevent this situation and to ensure that the intended logging implementation is being utilized.”
Lisa Ramirez (DevOps Consultant, CloudTech Advisors). “The presence of multiple SLF4J bindings can complicate the deployment process and lead to runtime exceptions. To mitigate these risks, teams should adopt a strict dependency management policy and utilize tools that can analyze and resolve conflicts in the class path effectively.”
Frequently Asked Questions (FAQs)
What does it mean when the class path contains multiple SLF4J bindings?
When the class path contains multiple SLF4J bindings, it indicates that multiple implementations of the SLF4J API are present, which can lead to conflicts and unexpected behavior in logging.
How can I identify the SLF4J bindings in my class path?
You can identify SLF4J bindings in your class path by examining the dependencies in your build configuration file (like Maven’s `pom.xml` or Gradle’s `build.gradle`) or by using tools like `mvn dependency:tree` for Maven projects.
What issues may arise from having multiple SLF4J bindings?
Having multiple SLF4J bindings can result in runtime exceptions, inconsistent logging behavior, and difficulty in determining which logging implementation is being used, leading to potential confusion during debugging.
How can I resolve the issue of multiple SLF4J bindings?
To resolve this issue, you should ensure that only one SLF4J binding is included in your project dependencies. You can exclude unwanted bindings or adjust your dependency management to include only the required one.
What are some common SLF4J bindings that developers might encounter?
Common SLF4J bindings include Logback, Log4j, and java.util.logging. Each of these provides a different logging implementation that can be used with the SLF4J API.
Is it safe to ignore warnings about multiple SLF4J bindings?
It is not safe to ignore warnings about multiple SLF4J bindings. Ignoring these warnings can lead to unpredictable logging behavior and complicate troubleshooting efforts in your application.
The presence of multiple SLF4J bindings in the class path can lead to significant issues in Java applications, primarily due to the ambiguity it creates regarding which logging implementation to utilize. SLF4J (Simple Logging Facade for Java) is designed to provide a simple abstraction for various logging frameworks. However, when multiple bindings are available, it results in a conflict that can cause unexpected behavior, such as logging messages being sent to the wrong destination or not appearing at all. This situation often manifests as warnings during application startup, indicating that multiple bindings have been found.
To mitigate the problems associated with multiple SLF4J bindings, it is crucial to ensure that only one binding is included in the class path at any given time. This can be achieved by reviewing project dependencies and removing any unnecessary or conflicting bindings. Tools such as Maven or Gradle can assist in managing dependencies effectively, allowing developers to identify and exclude redundant libraries. By maintaining a clean class path, developers can ensure that their logging configuration behaves as expected, leading to more reliable application performance and easier debugging.
In summary, addressing the issue of multiple SLF4J bindings is essential for maintaining the integrity of logging within Java applications. By adhering to best practices in dependency management and
Author Profile
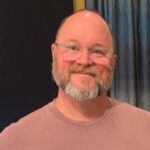
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?