Why Am I Encountering the ‘Java Illegal Start Of Expression’ Error?
In the world of Java programming, encountering errors is an inevitable part of the development journey. Among the myriad of messages that can appear during compilation, the “Illegal Start of Expression” error stands out as a particularly perplexing one. This cryptic message often leaves both novice and seasoned developers scratching their heads, wondering what went wrong in their code. Understanding this error is crucial for any Java programmer looking to write clean, functional code and avoid common pitfalls. In this article, we will delve into the intricacies of the “Illegal Start of Expression” error, exploring its causes, implications, and how to effectively troubleshoot and resolve it.
Overview
The “Illegal Start of Expression” error typically arises when the Java compiler encounters a line of code that it cannot interpret due to syntax issues. This can occur for a variety of reasons, ranging from misplaced punctuation to incorrect variable declarations. Often, the error is not isolated to the line indicated by the compiler; instead, it may stem from preceding lines that disrupt the logical flow of the code. Understanding the context in which this error occurs is essential for diagnosing and fixing the underlying issues.
As we navigate through the common scenarios that lead to this error, we will also highlight best practices for writing syntactically correct Java code.
Common Causes of Illegal Start of Expression
The “Illegal Start of Expression” error in Java typically arises from issues related to syntax. Identifying the specific cause requires an understanding of common scenarios that lead to this error. Here are some prevalent causes:
- Missing Semicolons: Java statements must end with a semicolon. Forgetting this can lead to confusion for the compiler.
- Improper Method Declaration: Declaring a method without proper syntax, such as missing parentheses or curly braces, can trigger this error.
- Incorrect Variable Declarations: Variables must be declared properly. For instance, trying to use a variable without declaring it first will cause issues.
- Misplaced Operators: Using operators incorrectly or placing them in unexpected locations can lead to syntax errors.
- Using Reserved Keywords: Using Java reserved keywords as variable names or identifiers results in syntax errors.
Examples of the Error
Here are some examples illustrating the “Illegal Start of Expression” error:
“`java
// Example 1: Missing semicolon
int a = 5
int b = 10;
// Example 2: Improper method declaration
void myMethod() {
System.out.println(“Hello World”)
}
// Example 3: Incorrect variable declaration
int 5variable = 10; // Variable name cannot start with a digit
“`
Each of these snippets will cause the Java compiler to throw an “Illegal Start of Expression” error due to the reasons outlined above.
How to Fix the Error
To resolve the “Illegal Start of Expression” error, follow these steps:
- Check for Missing Semicolons: Ensure that every statement ends with a semicolon.
- Review Method Declarations: Verify that all method declarations include parentheses and curly braces where necessary.
- Validate Variable Declarations: Ensure that all variables are declared correctly, adhering to Java naming conventions.
- Examine Operator Placement: Confirm that operators are used correctly and in the right context.
- Avoid Reserved Keywords: Make sure to use variable names that are not reserved keywords in Java.
Cause | Example | Correction |
---|---|---|
Missing Semicolon | int a = 5 | int a = 5; |
Improper Method Declaration | void myMethod() { System.out.println(“Hello World”) } | void myMethod() { System.out.println(“Hello World”); } |
Incorrect Variable Declaration | int 5variable = 10; | int variable5 = 10; |
By following these guidelines, developers can effectively address and resolve “Illegal Start of Expression” errors in their Java code.
Understanding the Java Illegal Start of Expression Error
The “Illegal Start of Expression” error in Java typically occurs during the compilation phase, indicating that the Java compiler has encountered a syntax issue. This error is often related to incorrect placement of code elements or improper syntax usage. Below are common scenarios that can trigger this error:
- Missing Semicolons: Forgetting to terminate statements with a semicolon.
- Incorrect Method Declaration: Issues with method signatures, including missing return types or modifiers.
- Misplaced Braces: Improper opening or closing of curly braces which define code blocks.
- Invalid Variable Declarations: Using reserved keywords or incorrect types.
Common Causes of the Error
The following table lists common coding mistakes that lead to the “Illegal Start of Expression” error, along with examples:
**Cause** | **Example** | **Description** |
---|---|---|
Missing Semicolon | `int a = 10` | Missing semicolon after variable declaration. |
Invalid Method Declaration | `public void myMethod( int a int b) {}` | Missing comma between parameters. |
Misplaced Braces | `if (a > b) { System.out.println(a) } else { System.out.println(b);` | Missing closing brace for the `if` statement. |
Invalid Variable Name | `int 1stNumber = 5;` | Variable names cannot begin with a digit. |
Improper Use of Keywords | `public class className {}` | Using a reserved keyword as an identifier. |
How to Troubleshoot the Error
When faced with the “Illegal Start of Expression” error, follow these troubleshooting steps:
- Check Syntax: Review the line indicated by the compiler and the lines preceding it for any syntax errors.
- Look for Missing Elements: Ensure that all statements end with a semicolon, and verify that method signatures are correctly formatted.
- Review Braces: Confirm that all opening braces `{` have corresponding closing braces `}`.
- Variable Names: Ensure that variable names adhere to Java naming conventions and do not start with numbers or use reserved keywords.
- Use IDE Features: Utilize the features of your Integrated Development Environment (IDE) that highlight syntax errors in real-time.
Example Illustrating the Error
Consider the following code snippet that generates the “Illegal Start of Expression” error:
“`java
public class Example {
public static void main(String[] args) {
int number = 5
System.out.println(“Number: ” + number);
}
}
“`
In this case, the error arises from the missing semicolon at the end of the line where `number` is declared. Correcting the code would involve adding the missing semicolon:
“`java
public class Example {
public static void main(String[] args) {
int number = 5; // Semicolon added here
System.out.println(“Number: ” + number);
}
}
“`
By addressing the issues outlined, developers can effectively resolve the “Illegal Start of Expression” error and ensure that their Java code compiles successfully.
Understanding Java’s Illegal Start Of Expression Error
Dr. Emily Carter (Senior Java Developer, CodeCraft Solutions). “The ‘Illegal Start Of Expression’ error in Java typically arises from syntax issues, such as misplaced brackets or incorrect use of keywords. Developers should carefully review their code for these common pitfalls to resolve the error effectively.”
Michael Chen (Java Language Expert, Tech Innovations). “This error can often confuse new programmers. It is crucial to remember that Java is sensitive to syntax and structure. A missing semicolon or an incorrectly placed variable declaration can trigger this error, so a thorough code review is essential.”
Lisa Tran (Software Engineer, DevOps Insights). “When encountering the ‘Illegal Start Of Expression’ error, I recommend using an Integrated Development Environment (IDE) that highlights syntax errors in real-time. This can significantly reduce debugging time and help developers identify the root cause of the issue more quickly.”
Frequently Asked Questions (FAQs)
What does “Illegal Start of Expression” mean in Java?
The “Illegal Start of Expression” error in Java indicates that the compiler has encountered a syntax issue, typically due to misplaced symbols, incorrect keywords, or improper variable declarations.
What are common causes of the “Illegal Start of Expression” error?
Common causes include missing semicolons, incorrect use of parentheses, misplaced curly braces, or using reserved keywords incorrectly in variable names or method declarations.
How can I fix the “Illegal Start of Expression” error?
To fix this error, carefully review the line indicated by the compiler, ensuring that all syntax rules are followed, such as proper punctuation, correct use of operators, and valid variable names.
Can this error occur in both method and class declarations?
Yes, the “Illegal Start of Expression” error can occur in both method and class declarations if there are syntax violations, such as missing access modifiers or incorrect method signatures.
Is the “Illegal Start of Expression” error specific to certain Java versions?
No, this error is not specific to any Java version; it is a general syntax error that can occur in any version of Java when the code does not conform to the language’s syntax rules.
How can I identify the exact location of the error in my code?
The Java compiler provides a line number where the error occurs, but the actual issue may be on the previous line. Carefully examine the surrounding code for any syntax mistakes.
The “Illegal Start of Expression” error in Java is a common compilation issue that typically arises from syntax errors in the code. This error message indicates that the Java compiler has encountered an unexpected token or an improper structure in the code, which prevents it from correctly interpreting the intended expression. Understanding the causes of this error is essential for effective debugging and ensuring that Java programs compile successfully.
Common triggers for the “Illegal Start of Expression” error include missing semicolons, misplaced curly braces, incorrect use of keywords, and improper variable declarations. Developers must pay close attention to the syntax rules of Java, as even minor mistakes can lead to this error. Additionally, the error may occur when trying to declare a variable or method in an inappropriate context, such as outside of a class or method body.
To resolve this error, it is crucial to carefully review the code for any syntax issues and ensure that all expressions are correctly formed. Utilizing an Integrated Development Environment (IDE) can help identify these errors more easily, as many IDEs provide real-time syntax checking and suggestions. By adhering to Java’s syntax rules and best practices, developers can minimize the occurrence of such errors and enhance the overall quality of their code.
Author Profile
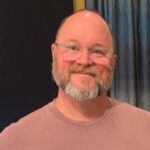
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?