How Can You Easily Convert a String to a Double in Java?
In the world of programming, data types play a crucial role in how we manipulate and process information. Among these types, strings and doubles are fundamental in Java, each serving distinct purposes. While strings are used to represent textual data, doubles are essential for handling numerical values with decimal points. However, there are times when you need to convert a string representation of a number into a double for calculations or data processing. Understanding how to perform this conversion efficiently can save you from potential errors and enhance your code’s performance. In this article, we will explore the various methods available in Java to convert strings to doubles, ensuring you have the tools you need to tackle this common programming task.
Converting a string to a double in Java is a straightforward process, but it requires a solid understanding of the built-in methods provided by the Java language. The most common approach involves using the `Double.parseDouble()` method, which is both efficient and easy to implement. However, developers should also be aware of potential pitfalls, such as handling invalid input or managing exceptions that may arise during the conversion process. By mastering these techniques, you can ensure that your applications handle numeric data seamlessly, regardless of its original format.
As we delve deeper into the nuances of string-to-double conversion, we will
Using Double.parseDouble()
To convert a string to a double in Java, one of the most common methods is by using the `Double.parseDouble()` method. This static method is straightforward and efficient, taking a string as an argument and returning its double value.
Here is an example of how to use `Double.parseDouble()`:
“`java
String numberString = “3.14”;
double number = Double.parseDouble(numberString);
“`
It is crucial to note that the string must represent a valid double; otherwise, a `NumberFormatException` will be thrown. This method handles both positive and negative numbers, as well as scientific notation.
Using Double.valueOf()
Another approach to convert a string to a double is to utilize the `Double.valueOf()` method. This method also accepts a string and returns a `Double` object, which can be unboxed to a primitive double if needed.
Example:
“`java
String numberString = “42.0”;
Double numberObject = Double.valueOf(numberString);
double number = numberObject; // Unboxing
“`
This method is particularly useful when you want to work with the `Double` object, which can be beneficial in contexts where object references are needed.
Handling Exceptions
When converting strings to doubles, it is essential to handle potential exceptions to maintain robust code. The `NumberFormatException` can occur if the string is not a valid representation of a double. To manage this, you can use a try-catch block.
Example:
“`java
String numberString = “not a number”;
try {
double number = Double.parseDouble(numberString);
} catch (NumberFormatException e) {
System.out.println(“Invalid number format: ” + e.getMessage());
}
“`
This code snippet will catch the exception and prevent the program from crashing, allowing for graceful error handling.
Comparison of Methods
The following table summarizes the differences between `Double.parseDouble()` and `Double.valueOf()`:
Method | Return Type | Throws Exception |
---|---|---|
Double.parseDouble() | double (primitive) | Yes |
Double.valueOf() | Double (object) | Yes |
Both methods are effective for converting strings to doubles; the choice between them depends on whether you need a primitive type or an object.
Using Regular Expressions for Validation
Before conversion, it may be prudent to validate the string format using regular expressions. This can help ensure that the string adheres to a double format.
Example:
“`java
String numberString = “123.45”;
if (numberString.matches(“-?\\d+(\\.\\d+)?”)) {
double number = Double.parseDouble(numberString);
} else {
System.out.println(“Invalid format”);
}
“`
This regex checks for an optional leading negative sign, followed by digits, with an optional decimal part. By validating the string before conversion, you can avoid exceptions and enhance code reliability.
Using Double.parseDouble()
The most straightforward method to convert a string to a double in Java is by using the `Double.parseDouble()` method. This method parses the string argument as a double.
“`java
String numberString = “3.14”;
double number = Double.parseDouble(numberString);
“`
- Input: A string representation of a number.
- Output: A double value.
Key Points:
- If the string does not contain a parsable double, a `NumberFormatException` is thrown.
- This method can handle both decimal numbers and scientific notation.
Using Double.valueOf()
Another approach is to use the `Double.valueOf()` method, which returns a `Double` object instead of a primitive double.
“`java
String numberString = “3.14”;
Double numberObject = Double.valueOf(numberString);
“`
- Input: A string representation of a number.
- Output: A `Double` object.
Differences from `parseDouble()`:
- Returns an object that can be null, which might be useful in certain applications.
- The object can be used in collections that require objects.
Handling Exceptions
When converting strings to doubles, it is essential to handle potential exceptions effectively. Here’s how to manage exceptions in both methods:
“`java
String numberString = “not_a_number”;
try {
double number = Double.parseDouble(numberString);
} catch (NumberFormatException e) {
System.out.println(“Invalid number format: ” + numberString);
}
“`
- Always wrap your conversion logic in a try-catch block.
- Catch `NumberFormatException` to handle invalid inputs gracefully.
Using DecimalFormat for Parsing
For more controlled parsing, especially when dealing with formatted strings, the `DecimalFormat` class can be utilized.
“`java
import java.text.DecimalFormat;
import java.text.ParseException;
String numberString = “3,14”;
DecimalFormat df = new DecimalFormat(“.”);
try {
Number number = df.parse(numberString);
double doubleValue = number.doubleValue();
} catch (ParseException e) {
System.out.println(“Parse error: ” + e.getMessage());
}
“`
- Input: A string representation of a formatted number.
- Output: A double value after parsing.
Benefits:
- Allows for custom formatting, such as handling commas or different decimal separators.
- Can manage locale-specific formats.
Comparison of Methods
Method | Returns | Handles Format Exceptions | Use Case |
---|---|---|---|
`Double.parseDouble()` | Primitive `double` | Yes | Quick conversion, simple cases |
`Double.valueOf()` | `Double` object | Yes | When an object is needed |
`DecimalFormat` | `Number` | Yes | Custom formats, locale support |
Each method has its strengths, and choosing the right one depends on the specific requirements of your application.
Expert Insights on Converting Strings to Doubles in Java
Jessica Chen (Senior Software Engineer, Tech Innovations Inc.). “When converting a string to a double in Java, it is essential to use the `Double.parseDouble()` method. This approach is efficient and handles standard numeric formats well, but developers should always anticipate potential `NumberFormatException` errors when the string is not a valid representation of a double.”
Michael Thompson (Java Developer Advocate, Open Source Community). “Utilizing `DecimalFormat` can provide more control over the conversion process, especially when dealing with localized number formats. This method allows developers to define specific patterns and handle various decimal separators, which is crucial for applications with international users.”
Linda Garcia (Lead Software Architect, Enterprise Solutions Corp.). “In scenarios where precision is paramount, consider using `BigDecimal` instead of `double`. Although it requires more overhead, `BigDecimal` provides greater accuracy for financial calculations, making it a better choice when converting strings that represent monetary values.”
Frequently Asked Questions (FAQs)
How can I convert a string to a double in Java?
You can convert a string to a double in Java using the `Double.parseDouble(String s)` method. This method parses the string argument as a double.
What happens if the string cannot be converted to a double?
If the string cannot be parsed as a double, a `NumberFormatException` will be thrown. It is advisable to handle this exception to avoid runtime errors.
Is there a way to convert a string to a double with error handling?
Yes, you can use a try-catch block to handle potential exceptions. This allows you to manage errors gracefully when the string is not a valid double.
Can I convert a string with commas to a double in Java?
No, the `Double.parseDouble()` method does not accept strings with commas. You must remove commas before parsing the string to a double.
What is the difference between `Double.parseDouble()` and `Double.valueOf()`?
`Double.parseDouble()` returns a primitive double, while `Double.valueOf()` returns a Double object. Use `Double.valueOf()` when you need an object reference.
Are there any locale-specific considerations when converting strings to doubles?
Yes, locale-specific number formats, such as different decimal separators, can affect conversion. For locale-aware parsing, consider using `NumberFormat` or `DecimalFormat` classes.
Converting a string to a double in Java is a fundamental task that developers often encounter. The primary method for achieving this is by utilizing the `Double.parseDouble()` method, which efficiently parses a string representation of a number and returns its double equivalent. This method is straightforward and handles standard numeric formats effectively, making it a reliable choice for most applications.
It is essential to be aware of potential exceptions that may arise during the conversion process. Specifically, if the string cannot be parsed into a double due to invalid formatting or non-numeric characters, a `NumberFormatException` will be thrown. Therefore, it is advisable to implement error handling mechanisms, such as try-catch blocks, to manage these exceptions gracefully and ensure that the application remains robust.
In addition to `Double.parseDouble()`, developers can also consider using the `Double.valueOf()` method, which returns a Double object instead of a primitive double. This can be beneficial in scenarios where object references are required. Understanding the nuances between these methods can enhance code clarity and performance, depending on the context of use.
In summary, converting strings to doubles in Java is a straightforward process that requires careful consideration of input validation and error handling. By leveraging the appropriate
Author Profile
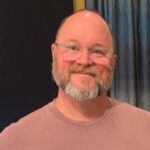
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?