How to Effectively Use Laravel and Tailwind to Determine If a Link is Active?
In the world of web development, creating a seamless and visually appealing user experience is paramount. Laravel, a powerful PHP framework, combined with Tailwind CSS, a utility-first CSS framework, provides developers with the tools necessary to build stunning applications efficiently. One common challenge developers face is managing navigation links, particularly when it comes to indicating which link is currently active. This is where the concept of “if link active” comes into play, allowing developers to enhance their navigation menus dynamically.
Understanding how to effectively highlight active links in your Laravel application using Tailwind CSS can significantly improve user engagement and navigation clarity. By leveraging Laravel’s robust routing capabilities alongside Tailwind’s flexible styling options, you can create a navigation system that not only looks great but also communicates context to users effortlessly. This article will delve into the best practices for implementing active link states, ensuring that your application is both functional and aesthetically pleasing.
As we explore this topic, we’ll cover techniques for conditionally applying classes based on the current route, making your navigation responsive to user interactions. With the right approach, you can elevate your Laravel projects and provide users with an intuitive experience that keeps them oriented within your application. So, let’s dive into the world of Laravel and Tailwind, and discover how to make your navigation links come alive
Implementing Active Links in Laravel with Tailwind CSS
To effectively manage the active states of navigation links in a Laravel application using Tailwind CSS, you’ll typically leverage Laravel’s routing capabilities alongside Blade’s templating engine. This allows you to dynamically determine which link should be marked as active based on the current route.
The basic approach involves checking the current route against the routes defined in your navigation. Here’s a simple example of how you might implement this:
“`blade
“`
This refactoring not only enhances readability but also promotes reusability across your application.
Handling Nested Routes
For applications with nested routes, the logic may become slightly more complex. You can modify the `active_link` function to accommodate parent routes. Here’s an updated version:
“`php
if (!function_exists(‘active_link’)) {
function active_link($routeNames)
{
foreach ((array)$routeNames as $routeName) {
if (request()->routeIs($routeName)) {
return ‘text-blue-500’;
}
}
return ‘text-gray-800’;
}
}
“`
With this implementation, you can pass an array of route names:
“`blade
“`
This flexibility allows you to maintain a visually coherent navigation experience even with more complex route structures.
Route Name | Active Class | Usage Example |
---|---|---|
home | text-blue-500 | {{ active_link(‘home’) }} |
about | text-blue-500 | {{ active_link(‘about’) }} |
dashboard.* | text-blue-500 | {{ active_link([‘dashboard.index’, ‘dashboard.*’]) }} |
Creating Active Link States in Laravel with Tailwind CSS
To manage active link states in a Laravel application using Tailwind CSS, you need to leverage Laravel’s built-in routing capabilities along with conditional classes in Blade templates. This allows you to dynamically apply styles to links based on the current route.
Blade Syntax for Active Links
In your Blade templates, you can utilize the `request()` helper or the `Route` facade to check the current route. Here’s how to implement it:
This approach simplifies the link rendering and keeps your templates clean.
Handling Nested Routes
For applications with nested routes, you might want to highlight parent links based on active child links. Utilize `request()->is()` to check for route patterns:
This example demonstrates how to hide certain links on smaller screens while maintaining the active state styling.
Final Thoughts on Active Link States
Implementing active link states in Laravel with Tailwind CSS requires a blend of Laravel’s routing features and Tailwind’s utility classes. By utilizing Blade’s capabilities, you can create a clean, maintainable navigation system that enhances user experience.
Best Practices for Implementing Active Links in Laravel with Tailwind CSS
Emily Carter (Senior Web Developer, Tech Innovations Inc.). “Incorporating Tailwind CSS with Laravel requires a clear understanding of how to manage active links. I recommend using the `request()->is()` method to dynamically add classes to your links, ensuring that the active state is visually distinct and consistent across your application.”
Michael Thompson (Frontend Architect, Creative Solutions). “Utilizing Tailwind CSS for styling active links in a Laravel application can enhance user experience significantly. It is essential to apply conditional classes based on the current route, and I suggest leveraging Blade directives for cleaner and more maintainable code.”
Sarah Lee (Full Stack Developer, Web Masters Co.). “To effectively manage active links in a Laravel application styled with Tailwind, I advocate for using the `Route::currentRouteName()` method. This approach allows developers to easily check the current route and apply the appropriate Tailwind classes, creating a seamless navigation experience.”
Frequently Asked Questions (FAQs)
How can I determine if a link is active in Laravel when using Tailwind CSS?
To determine if a link is active in Laravel with Tailwind CSS, you can use the `request()->is()` method to compare the current URL with the link’s URL. If they match, you can apply an active class to the link.
What is the recommended way to apply Tailwind CSS classes conditionally in Laravel?
The recommended way is to use Blade’s conditional directives. You can use the `@class` directive or inline PHP to append the active class based on the current route or URL.
Can I use route names to check for active links in Laravel?
Yes, you can use route names with the `route()` helper function in conjunction with the `request()->routeIs()` method to check if the current route matches a specific named route.
How do I style an active link differently in Tailwind CSS?
To style an active link differently, you can conditionally apply Tailwind CSS classes within your Blade template. For example, you can add `text-blue-500` for active links and `text-gray-500` for inactive ones.
Is it possible to manage active states for nested routes in Laravel?
Yes, you can manage active states for nested routes by using the `request()->is()` method with wildcard patterns. This allows you to match parent routes and apply the active class accordingly.
What should I do if my active link styling is not working as expected?
If your active link styling is not working, ensure that the URL or route name being checked accurately matches the current request. Additionally, verify that the Tailwind CSS classes are correctly applied and that there are no conflicting styles.
In summary, managing active links in a Laravel application styled with Tailwind CSS is a fundamental aspect of creating a user-friendly navigation experience. By utilizing Laravel’s built-in route helpers and conditional classes, developers can easily determine which links should appear active based on the current route. This enhances usability by providing clear visual cues to users, indicating their current location within the application.
Furthermore, integrating Tailwind CSS with Laravel allows for a streamlined approach to styling active links. By leveraging Tailwind’s utility-first classes, developers can quickly apply styles that reflect the active state of a link. This not only simplifies the styling process but also ensures consistency across the application, as Tailwind promotes reusability and modular design.
Ultimately, the combination of Laravel’s routing capabilities and Tailwind’s styling framework empowers developers to create intuitive and visually appealing navigation systems. By implementing these techniques, applications can achieve a professional look while enhancing user engagement and satisfaction. The result is a seamless experience that encourages users to explore the application further.
Author Profile
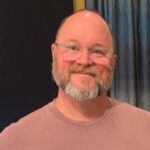
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?