Does Python Have Arrays? Exploring Array Options in Python Programming
When diving into the world of programming, one of the first questions that often arises is whether a particular language supports arrays. For Python enthusiasts and newcomers alike, understanding the data structures available in Python is crucial for efficient coding and problem-solving. While many programming languages have a dedicated array type, Python takes a different approach, offering a variety of options that cater to different needs. This article will explore the question: Does Python have arrays?
At first glance, Python may not have a traditional array type like some other languages, but it provides powerful alternatives that serve similar purposes. The most commonly used data structure in Python is the list, which allows for dynamic sizing and versatile operations. Additionally, Python’s standard library and third-party packages introduce specialized array-like structures, such as the `array` module and NumPy arrays, which are tailored for numerical computations and performance optimization.
As we delve deeper into this topic, we will uncover the nuances of these data structures, their functionalities, and the scenarios in which each is best utilized. Whether you’re a seasoned developer or just starting your programming journey, understanding how Python handles arrays and similar constructs will enhance your coding toolkit and enable you to write more effective and efficient code.
Understanding Python Arrays
In Python, the term “array” can be somewhat misleading as it is often confused with lists, which are the primary data structure for storing sequences of elements. However, Python does provide a formal array structure through the `array` module, which offers a more efficient way to handle homogeneous data types compared to lists.
The `array` module allows for the creation of arrays that can store data types such as integers, floats, and characters. These arrays are mutable, meaning their contents can be changed after creation. Here are some key points regarding Python arrays:
- Homogeneous Data: Unlike lists that can hold mixed data types, arrays are designed to hold data of a single type.
- Performance: Arrays can lead to better performance in terms of memory usage and speed when dealing with large datasets compared to lists.
- Methods: The array module provides a variety of methods for manipulating arrays, including adding, removing, and accessing elements.
To create an array in Python, one can use the following syntax:
“`python
import array
Creating an array of integers
arr = array.array(‘i’, [1, 2, 3, 4, 5])
“`
In this example, the first argument `’i’` specifies the type of the array, which in this case is an integer.
Comparison of Lists and Arrays
While both lists and arrays serve similar purposes in Python, they have distinct differences that affect their use cases. The following table summarizes these differences:
Feature | List | Array |
---|---|---|
Data Types | Heterogeneous | Homogeneous |
Memory Efficiency | Less efficient | More efficient |
Performance | Slower with large datasets | Faster with large datasets |
Methods | Extensive methods available | Limited methods |
When to Use Arrays
Choosing between lists and arrays largely depends on the specific requirements of the application. Here are scenarios where using arrays is more advantageous:
- Numerical Data Processing: When performing operations on large datasets of numerical data, arrays can provide better performance due to lower memory overhead.
- Data Interfacing: When interfacing with C libraries or when working with systems requiring fixed data types, arrays are often preferred.
- Scientific Computing: Libraries such as NumPy provide powerful array functionalities for mathematical operations and are widely used in scientific computing.
In summary, while Python lists are versatile and widely used, the `array` module offers a specialized tool for scenarios where type consistency and performance are critical. Understanding when to leverage each structure is essential for efficient programming in Python.
Understanding Arrays in Python
Python does not have a built-in array data type like some other programming languages, such as Java or C++. However, Python provides several alternatives that can be used to achieve similar functionality, the most notable being lists and the `array` module.
Lists vs. Arrays
- Lists:
- Dynamic and can hold items of different data types.
- Syntax: `my_list = [1, ‘two’, 3.0]`
- Common methods: `append()`, `remove()`, `extend()`, `insert()`, etc.
- Arrays:
- Provided by the `array` module, which requires all elements to be of the same type.
- More memory efficient than lists for large datasets.
- Syntax:
“`python
import array
my_array = array.array(‘i’, [1, 2, 3])
“`
- Type codes determine the type of elements, e.g., ‘i’ for integers, ‘f’ for floats.
Using the Array Module
The `array` module allows you to create arrays in Python. It provides a more compact representation compared to lists. Here are some key features:
Feature | Description |
---|---|
Type Codes | Defines the data type of the array elements (e.g., ‘i’, ‘f’, ‘d’). |
Memory Efficiency | Uses less memory than lists for large data sets with uniform data types. |
Basic Operations | Supports operations such as indexing, slicing, and basic element manipulation. |
NumPy Arrays
For more advanced array manipulation, the NumPy library is widely used in scientific computing. It offers:
- Homogeneous Data: All elements must be of the same type.
- Multi-dimensional Arrays: Supports n-dimensional arrays.
- Performance: Optimized for performance with large data sets.
Example of creating a NumPy array:
“`python
import numpy as np
my_numpy_array = np.array([1, 2, 3])
“`
Comparison of Data Structures
Data Structure | Type Homogeneity | Dynamic Sizing | Performance | Use Cases |
---|---|---|---|---|
List | No | Yes | Moderate | General-purpose storage |
Array (array) | Yes | Limited | High for large data | Numeric computations |
NumPy Array | Yes | No | Very high | Scientific and mathematical operations |
In summary, while Python does not have traditional arrays, it provides lists and the `array` module for array-like functionality. For more complex needs, NumPy offers powerful capabilities that make it the standard for numerical computations in Python. Each option has its use cases and advantages, making Python a versatile language for various programming tasks.
Understanding Arrays in Python: Expert Insights
Dr. Emily Carter (Computer Science Professor, Tech University). “While Python does not have a built-in array data type like some other programming languages, it provides a powerful list structure that can serve similar purposes. For more specialized array functionalities, the NumPy library is often recommended, as it offers efficient multi-dimensional arrays and a wide range of mathematical operations.”
Michael Chen (Senior Software Engineer, Data Solutions Inc.). “Many developers mistakenly believe that Python lacks arrays. In reality, Python lists can be used as dynamic arrays. However, for numerical computations, using NumPy arrays is essential due to their performance benefits and the rich set of features they offer.”
Sarah Thompson (Data Scientist, Analytics Hub). “In Python, the choice between lists and arrays often depends on the specific use case. Lists are versatile and easy to use for general purposes, while arrays from libraries like NumPy are optimized for numerical data processing, making them indispensable in data science.”
Frequently Asked Questions (FAQs)
Does Python have arrays?
Yes, Python has arrays, but they are not as commonly used as lists. The `array` module provides a basic array type that is more efficient for numerical data than lists.
What is the difference between Python lists and arrays?
Python lists can hold elements of different data types, while arrays from the `array` module are restricted to a single data type. This makes arrays more memory efficient for large datasets.
How do you create an array in Python?
You can create an array in Python using the `array` module by importing it and specifying the type code. For example:
“`python
import array
my_array = array.array(‘i’, [1, 2, 3, 4]) ‘i’ indicates an array of integers
“`
When should I use arrays instead of lists in Python?
Use arrays when you need to store large amounts of numerical data and require efficient memory usage. For general-purpose programming, lists are often more convenient due to their flexibility.
Are there any third-party libraries for arrays in Python?
Yes, libraries such as NumPy provide powerful array structures that support multidimensional arrays and a wide range of mathematical operations. NumPy arrays are preferred for scientific computing.
Can you perform mathematical operations on Python arrays?
Basic mathematical operations can be performed on arrays created with the `array` module, but for advanced operations, it is recommended to use NumPy arrays, which support vectorized operations and broadcasting.
Python does not have a built-in array data structure in the same way that languages like C or Java do. Instead, it provides a more versatile and powerful data structure known as a list, which can be used similarly to arrays. Lists in Python are dynamic, allowing for the storage of elements of various data types, and they come with a rich set of built-in methods for manipulation.
For scenarios that require true array functionality, Python offers the array module, which provides a more efficient storage option for homogeneous data types. However, the array module is less commonly used than lists due to its limitations in terms of flexibility and the need for type specification. Additionally, libraries such as NumPy provide robust array-like structures that support multi-dimensional arrays and a wide array of mathematical operations, making them ideal for scientific computing and data analysis.
In summary, while Python does not have traditional arrays, it offers several alternatives that fulfill similar purposes. The choice between lists, the array module, and third-party libraries like NumPy depends on the specific requirements of the application, such as the need for performance, data type uniformity, or advanced mathematical capabilities.
Author Profile
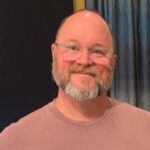
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?