How Can You Effectively Comment Out Blocks of Code in Python?
In the world of programming, clarity and organization are paramount. As developers, we often find ourselves needing to temporarily disable sections of code or add annotations to explain our logic without disrupting the flow of execution. This is where the art of commenting comes into play, particularly in Python, a language celebrated for its readability and simplicity. Understanding how to effectively comment out blocks of code not only enhances your coding practices but also fosters collaboration and communication within teams.
Commenting in Python serves multiple purposes, from debugging to documentation. When you comment out a block of code, you create an opportunity to test different functionalities without deleting any existing work. This practice allows for a more flexible coding environment, where experimentation can thrive without the fear of losing valuable lines of code. Moreover, well-placed comments can guide others (and your future self) through complex logic, making it easier to navigate and understand the codebase.
In this article, we will explore the various methods for commenting out blocks of code in Python, including best practices and common pitfalls to avoid. Whether you are a novice programmer or a seasoned developer, mastering this essential skill will undoubtedly enhance your programming toolkit and improve your overall coding experience. Get ready to dive into the world of Python comments and unlock the potential of clearer, more maintainable code!
Using Triple Quotes for Multi-line Comments
In Python, a common method for commenting out blocks of code is to use triple quotes. This approach is not a true comment but rather a multi-line string that is not assigned to any variable. When Python encounters these triple quotes, it ignores the content, effectively treating it as a comment.
Example:
“`python
“””
This is a block of code that will not be executed.
print(“This will not run”)
“””
“`
The triple quotes can be either single (`”’`) or double (`”””`) and can span multiple lines. This method is particularly useful for temporarily disabling large sections of code during debugging.
Using the Hash Symbol for Single-line Comments
For single-line comments, Python uses the hash symbol (“). Any text following the “ on the same line will be treated as a comment and ignored by the interpreter. While this method is effective for individual lines, it can be tedious for larger blocks of code.
Example:
“`python
This line is a comment
print(“This line will not execute”)
“`
Commenting Out Multiple Lines Efficiently
To comment out multiple lines efficiently, you can use the following strategies:
- Select multiple lines in your code editor and apply a block comment shortcut (e.g., `Ctrl + /` in many editors).
- Wrap the lines in triple quotes as previously discussed.
Here’s a table summarizing the methods:
Method | Description | Example |
---|---|---|
Triple Quotes | Used for multi-line comments; not technically a comment. |
""" This is a comment spanning multiple lines. """ |
Hash Symbol | Used for single-line comments; straightforward but not efficient for many lines. |
This is a comment |
Best Practices for Commenting Code
When commenting out blocks of code, it is essential to maintain clarity and readability. Here are some best practices:
- Use comments to explain why certain decisions were made in the code, rather than what the code does.
- Avoid excessive commenting; clean, self-explanatory code is preferable.
- Regularly update comments to reflect any changes in the codebase.
By following these practices, you can ensure that your code remains easy to understand and maintain.
Commenting Out Single Lines of Code
In Python, you can comment out a single line of code by using the hash symbol (“). Any text following this symbol on the same line will be ignored by the Python interpreter.
Example:
“`python
This is a single-line comment
print(“Hello, World!”) This prints a message
“`
It is crucial to ensure that the “ symbol is placed at the beginning of the line or preceding the code you wish to comment out. This method is straightforward and widely used for brief explanations or notes.
Commenting Out Multiple Lines of Code
Python does not have a specific syntax for block comments like some other programming languages. However, there are two common approaches to comment out multiple lines:
- Using Multiple “ Symbols:
Each line that you want to comment out must begin with a “. This method is simple but can be tedious for longer blocks of code.
Example:
“`python
This is the first line of a comment
This is the second line of a comment
This is the third line of a comment
“`
- Using Triple Quotes:
Triple quotes (`”’` or `”””`) can be used to create multi-line strings, which can effectively serve as comments if not assigned to a variable. This method is useful for longer explanations or temporarily disabling blocks of code.
Example:
“`python
”’
This is a multi-line comment.
It spans multiple lines.
”’
print(“This code runs.”)
“`
Note that using triple quotes is often reserved for documentation strings (docstrings) and should be used judiciously to avoid confusion.
Best Practices for Commenting Code
When commenting code, adhere to the following best practices:
- Be Concise: Comments should be brief and to the point. Avoid unnecessary verbosity.
- Use Clear Language: Write comments that are easily understandable. Technical jargon should be minimized unless necessary.
- Keep Comments Updated: Ensure comments reflect the current state of the code. Outdated comments can lead to confusion.
- Avoid Obvious Comments: Comments should add value. Avoid stating the obvious, such as commenting “This is a variable” next to a variable declaration.
- Use Comments for Complex Logic: Provide explanations for complex algorithms or logic that may not be immediately clear.
Tools and Features for Commenting in IDEs
Many Integrated Development Environments (IDEs) and code editors provide features that facilitate commenting out code:
Feature | Description |
---|---|
Comment Shortcuts | Most IDEs allow you to comment/uncomment lines quickly using keyboard shortcuts (e.g., `Ctrl + /` or `Cmd + /`). |
Block Commenting | Some IDEs enable users to select multiple lines and comment them out in one action. |
Syntax Highlighting | Comments are usually displayed in a different color, making them easy to distinguish from executable code. |
Utilizing these tools can significantly enhance productivity and maintainability of your code.
Expert Insights on Commenting Out Code in Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Commenting out blocks of code in Python is essential for maintaining code readability and organization. Utilizing the hash symbol () for single-line comments is straightforward, but for multi-line comments, developers often use triple quotes (”’ or “””). This practice not only helps in debugging but also in providing context for future code reviews.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “In Python, the most common method for commenting out blocks of code is by using the hash symbol at the beginning of each line. However, for larger sections, wrapping the code in triple quotes is a practical approach. This method is particularly useful when temporarily disabling code during testing phases without deleting it, ensuring that it can be easily restored later.”
Sarah Thompson (Technical Writer, Python Programming Journal). “Effective commenting practices are crucial for collaborative coding environments. While Python does not have a built-in block comment feature, developers can leverage triple quotes for this purpose. It is important to ensure that comments are clear and informative, as this greatly enhances the maintainability of the codebase.”
Frequently Asked Questions (FAQs)
How do I comment out a single line of code in Python?
To comment out a single line of code in Python, use the hash symbol “ at the beginning of the line. Everything following the “ on that line will be treated as a comment and ignored during execution.
Is there a way to comment out multiple lines of code in Python?
Python does not have a specific syntax for multi-line comments, but you can achieve this by using triple quotes `”’` or `”””`. However, this method creates a string literal and is not technically a comment. The most common practice is to use “ at the beginning of each line.
Can I use block comments in Python?
Python does not support block comments in the traditional sense found in other programming languages. Instead, you can use multiple single-line comments or a multi-line string with triple quotes, although the latter is not a true comment.
What is the purpose of commenting out code?
Commenting out code serves several purposes, including temporarily disabling code for debugging, providing explanations for complex logic, and improving code readability for others or for future reference.
Are comments in Python ignored by the interpreter?
Yes, comments in Python are ignored by the interpreter. They do not affect the execution of the program and are solely for the benefit of the developers who read the code.
Can I use comments to document my functions in Python?
Yes, comments can be used to document functions in Python. However, it is recommended to use docstrings (triple quotes immediately following the function definition) for formal documentation, as they can be accessed through the `help()` function and are more standardized.
In Python, commenting out blocks of code is an essential practice for developers, as it enhances code readability and maintainability. The primary method for commenting in Python is through the use of the hash symbol (), which allows for single-line comments. However, for multi-line comments or block comments, developers often utilize triple quotes (”’ or “””). This approach not only serves to comment out multiple lines of code but can also be employed for docstrings, which provide documentation for functions, classes, and modules.
It is important to understand the context in which comments are used. Effective commenting can clarify complex logic, explain the purpose of specific code sections, and assist others (or oneself) in recalling the reasoning behind certain implementations. Developers should strive to keep comments concise and relevant, ensuring that they add value rather than cluttering the codebase.
In summary, mastering the art of commenting out blocks of code in Python is a vital skill for any programmer. By utilizing the appropriate commenting techniques, developers can create more understandable and maintainable code. Ultimately, thoughtful comments contribute to a collaborative coding environment, fostering better communication and understanding among team members.
Author Profile
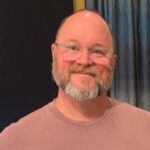
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?