How Can You Convert a Timestamp to a Date in SQL?
In the world of data management, SQL (Structured Query Language) stands as a cornerstone for interacting with databases. One of the common tasks that database professionals encounter is the need to manipulate and format data for analysis and reporting. Among these tasks, converting timestamps to dates is a fundamental operation that can significantly impact data interpretation and usability. Whether you’re generating reports, conducting analysis, or simply cleaning up your data, understanding how to effectively convert timestamps into a more readable date format is essential.
Timestamps, which include both date and time information, can often be cumbersome when you only need the date component for your analysis. SQL provides various functions and methods to facilitate this conversion, allowing users to extract just the date portion while discarding the time. This process not only simplifies data presentation but also enhances clarity, especially when dealing with large datasets where time granularity may not be necessary.
As we delve deeper into the topic, we will explore the various SQL functions available for converting timestamps to dates across different database systems. We will also discuss practical examples and scenarios where this conversion is particularly useful, equipping you with the knowledge to handle date-related queries with confidence and precision. Whether you are a seasoned database administrator or a newcomer to SQL, mastering this skill will undoubtedly enhance your data manipulation capabilities.
Understanding SQL Timestamp
A timestamp in SQL is a data type that represents a specific point in time. It includes both date and time components, typically formatted as `YYYY-MM-DD HH:MM:SS`. This allows for precise tracking of events down to the second, making it particularly useful in applications that require time-stamped records.
When working with timestamps, it’s essential to understand how to manipulate and format these values to meet various reporting and analytical needs. One common requirement is converting timestamps into date formats, which can simplify data analysis and reporting.
Converting Timestamp to Date
To convert a timestamp to a date in SQL, several functions can be utilized depending on the SQL database system in use. The most commonly used methods include:
- CAST() Function: This is a standard SQL function that can convert a timestamp to a date.
- CONVERT() Function: Specific to SQL Server, this function allows for more control over the format of the output date.
- DATE() Function: In MySQL, this function extracts the date part from a timestamp.
The syntax for each of these methods varies slightly, as shown in the following table:
Database | Function | Syntax | Description |
---|---|---|---|
MySQL | DATE() | SELECT DATE(timestamp_column) FROM table_name; | Extracts the date part from the timestamp. |
SQL Server | CONVERT() | SELECT CONVERT(DATE, timestamp_column) FROM table_name; | Converts timestamp to date with specific format options. |
PostgreSQL | CAST() | SELECT CAST(timestamp_column AS DATE) FROM table_name; | Converts timestamp directly to date. |
The choice of function may depend on the specific requirements of your project as well as the SQL dialect you are using.
Example Usage
Consider a table named `orders` that contains a column `order_timestamp` of type timestamp. To extract the date from this timestamp in various SQL databases, you can use the following queries:
- MySQL:
“`sql
SELECT DATE(order_timestamp) AS order_date FROM orders;
“`
- SQL Server:
“`sql
SELECT CONVERT(DATE, order_timestamp) AS order_date FROM orders;
“`
- PostgreSQL:
“`sql
SELECT CAST(order_timestamp AS DATE) AS order_date FROM orders;
“`
Each of these queries will return only the date part of the `order_timestamp`, facilitating easier analysis and reporting on the dates of orders without the time component.
Considerations
When converting timestamps to dates, be aware of the following:
- Time Zone Differences: Ensure that you account for any time zone differences that may affect the date representation.
- Data Type Compatibility: Verify that the function you use is compatible with the data types in your database to avoid type conversion errors.
- Performance: Frequent conversions may impact performance, especially on large datasets. It may be more efficient to store dates directly in a date column if time data is not needed.
With these methods and considerations, converting timestamps to dates in SQL becomes a straightforward process that enhances your data handling capabilities.
Understanding SQL Timestamp Data Types
In SQL, timestamps are used to store a date and time value. The exact data type may vary depending on the database system (such as MySQL, SQL Server, or PostgreSQL). Here are some common timestamp data types:
- MySQL: `DATETIME`, `TIMESTAMP`
- SQL Server: `DATETIME`, `DATETIME2`, `SMALLDATETIME`
- PostgreSQL: `TIMESTAMP`, `TIMESTAMP WITH TIME ZONE`
Each type has its own characteristics regarding precision and range. For example, the `DATETIME` type in MySQL can represent a range from ‘1000-01-01’ to ‘9999-12-31’.
Methods to Convert Timestamp to Date
Converting a timestamp to a date format is a common requirement in SQL queries. The methods vary by SQL dialect. Below are examples for different SQL systems:
MySQL
In MySQL, use the `DATE()` function to extract the date part from a timestamp:
“`sql
SELECT DATE(your_timestamp_column) AS date_only
FROM your_table;
“`
Alternatively, you can use the `CAST` function:
“`sql
SELECT CAST(your_timestamp_column AS DATE) AS date_only
FROM your_table;
“`
SQL Server
In SQL Server, you can convert a timestamp to a date using the `CONVERT` function or the `CAST` function. Here’s how:
“`sql
SELECT CONVERT(DATE, your_timestamp_column) AS date_only
FROM your_table;
“`
Or using `CAST`:
“`sql
SELECT CAST(your_timestamp_column AS DATE) AS date_only
FROM your_table;
“`
PostgreSQL
In PostgreSQL, the `::` operator or the `DATE()` function can be used for conversion:
“`sql
SELECT your_timestamp_column::DATE AS date_only
FROM your_table;
“`
Or:
“`sql
SELECT DATE(your_timestamp_column) AS date_only
FROM your_table;
“`
Handling Time Zones
When converting timestamps that include time zones, it’s important to consider how the conversion affects the date value. Here are key points for handling time zones:
- MySQL: Use `CONVERT_TZ()` to convert a timestamp from one time zone to another before extracting the date.
- SQL Server: Use `AT TIME ZONE` to specify the time zone during conversion.
- PostgreSQL: You can use `SET TIME ZONE` to adjust the session time zone before performing the conversion.
Examples of Usage
Here are some practical examples demonstrating the conversion of timestamps to dates in different SQL databases:
Database | SQL Statement Example |
---|---|
MySQL | `SELECT DATE(NOW()) AS current_date;` |
SQL Server | `SELECT CONVERT(DATE, GETDATE()) AS current_date;` |
PostgreSQL | `SELECT CURRENT_TIMESTAMP::DATE AS current_date;` |
These examples demonstrate how to retrieve the current date by converting the current timestamp to a date format.
Conclusion on Best Practices
When converting timestamps to dates, consider the following best practices:
- Always verify the data type of your timestamp column.
- Be cautious of time zone effects when dealing with timestamps that include time zone information.
- Test your queries to ensure that the output meets your expectations, especially when dealing with edge cases like daylight saving time changes.
By following these guidelines and utilizing the appropriate SQL functions, you can effectively convert timestamps to date formats across different database systems.
Expert Insights on Converting Timestamps to Dates in SQL
Dr. Emily Chen (Database Architect, Tech Solutions Inc.). “Converting timestamps to dates in SQL is a fundamental skill for database management. It allows for easier data manipulation and reporting, especially when dealing with large datasets that include time-sensitive information.”
Michael Thompson (Senior Data Analyst, Analytics Hub). “Utilizing functions like CAST or CONVERT in SQL not only simplifies the process of transforming timestamps into date formats but also enhances query performance by reducing the amount of data processed in time-based analyses.”
Sarah Patel (SQL Trainer and Consultant, DataWise Academy). “Understanding the nuances of different SQL dialects is crucial when converting timestamps to dates. Each database system may have its own functions and syntax, which can significantly impact the accuracy and efficiency of your data operations.”
Frequently Asked Questions (FAQs)
What is the SQL function to convert a timestamp to a date?
The SQL function commonly used to convert a timestamp to a date is `CAST()` or `CONVERT()`. For example, `CAST(timestamp_column AS DATE)` or `CONVERT(DATE, timestamp_column)` can be used.
How do I format the output date when converting a timestamp?
You can format the output date using the `FORMAT()` function in SQL Server or `TO_CHAR()` in Oracle. For instance, `FORMAT(timestamp_column, ‘yyyy-MM-dd’)` or `TO_CHAR(timestamp_column, ‘YYYY-MM-DD’)` will provide a specific date format.
Can I convert a timestamp to a date in MySQL?
Yes, in MySQL, you can use the `DATE()` function to extract the date from a timestamp. The syntax is `DATE(timestamp_column)`, which returns the date part of the timestamp.
Is it possible to convert a timestamp to a date in PostgreSQL?
Yes, in PostgreSQL, you can use the `::date` operator or the `DATE()` function. For example, `timestamp_column::date` or `DATE(timestamp_column)` will convert the timestamp to a date.
What happens to the time portion when converting a timestamp to a date?
When converting a timestamp to a date, the time portion is discarded, and only the date part is retained. This means that the resulting value will reflect only the year, month, and day.
Are there performance considerations when converting timestamps in SQL?
Yes, frequent conversions can impact performance, especially on large datasets. It is advisable to perform conversions only when necessary and consider indexing strategies to optimize query performance.
In summary, converting a timestamp to a date in SQL is a fundamental operation that allows users to manipulate and format date and time data effectively. SQL provides various functions, such as `CAST()` and `CONVERT()`, which facilitate this conversion. Understanding the specific syntax and options available in different SQL dialects, such as MySQL, PostgreSQL, and SQL Server, is crucial for accurate implementation. Each database management system may have its nuances, requiring users to adapt their queries accordingly.
Moreover, the conversion process is not merely about changing formats; it is essential for data analysis, reporting, and ensuring that date-related queries return the expected results. By converting timestamps to dates, users can streamline their data processing, improve query performance, and enhance the readability of their output. This capability is particularly valuable in scenarios where time precision is less critical than the date itself.
Key takeaways include the importance of selecting the appropriate conversion function based on the SQL environment and understanding the implications of time zones and formats. Additionally, users should be aware of potential pitfalls, such as data truncation or loss of time information, when performing these conversions. Mastery of timestamp to date conversion not only improves data handling but also empowers users to derive meaningful insights from
Author Profile
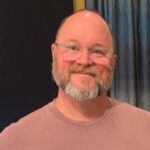
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?