Why Am I Getting ‘Cannot Import Name ‘Calinski_Harabaz_Score’ From ‘sklearn.Metrics’ Error?
In the ever-evolving landscape of machine learning and data science, the ability to evaluate clustering algorithms is crucial for deriving meaningful insights from complex datasets. Among the various metrics available, the Calinski-Harabasz score stands out as a powerful tool for assessing the quality of clustering results. However, many practitioners encounter a frustrating hurdle: the error message “Cannot import name ‘Calinski_Harabaz_Score’ from ‘sklearn.metrics’.” This seemingly simple issue can halt progress and lead to confusion, especially for those who rely on the Scikit-learn library for their analytical needs. In this article, we will explore the intricacies of this error, its implications, and how to navigate the challenges associated with clustering evaluation.
As machine learning practitioners delve into the world of clustering, they often turn to Scikit-learn for its robust suite of tools and metrics. The Calinski-Harabasz score, which evaluates the ratio of the sum of between-cluster dispersion to within-cluster dispersion, is a popular choice for determining the effectiveness of clustering algorithms. However, with updates and changes in libraries, users may find themselves facing import errors that disrupt their workflow. Understanding the root causes of these import issues is essential for maintaining a smooth development process.
In the
Understanding the Error
When encountering the error message `Cannot import name ‘Calinski_Harabaz_Score’ from ‘sklearn.metrics’`, it typically signifies that the specified function is not available in the version of the Scikit-learn library being used. The Calinski-Harabasz score is a metric used to evaluate clustering performance, and it was previously accessible under this name. However, in later versions, it has been renamed.
Correct Usage of the Function
The correct function to use in place of `Calinski_Harabaz_Score` is `calinski_harabasz_score`, which is part of the same library but is now correctly referenced in lowercase. Here’s how to properly import and use the function:
“`python
from sklearn.metrics import calinski_harabasz_score
“`
This change in naming conventions emphasizes the importance of adhering to Python’s variable naming standards.
Version Compatibility
To avoid this import error, it is crucial to ensure that your Scikit-learn version is compatible with your code. Here’s how you can check your version of Scikit-learn:
“`python
import sklearn
print(sklearn.__version__)
“`
If you find that your version is outdated, consider upgrading it using pip:
“`bash
pip install –upgrade scikit-learn
“`
Alternative Metrics for Clustering Evaluation
In addition to the Calinski-Harabasz score, several other metrics can be employed to evaluate clustering performance. Here’s a brief overview:
Metric | Description |
---|---|
Silhouette Score | Measures how similar an object is to its own cluster compared to other clusters. |
Davies-Bouldin Index | A lower score indicates better clustering. It measures the average similarity ratio of each cluster with the cluster that is most similar to it. |
Adjusted Rand Index | Measures the similarity between two data clusterings, adjusted for chance. |
Implementing the Calinski-Harabasz Score
To compute the Calinski-Harabasz score in your clustering workflow, you can use the following example. This snippet assumes you have already performed clustering and have your cluster labels and data points:
“`python
from sklearn.metrics import calinski_harabasz_score
from sklearn.datasets import make_blobs
from sklearn.cluster import KMeans
Generating synthetic data
X, _ = make_blobs(n_samples=300, centers=4, random_state=42)
Performing KMeans clustering
kmeans = KMeans(n_clusters=4, random_state=42)
kmeans.fit(X)
labels = kmeans.labels_
Calculating the Calinski-Harabasz score
score = calinski_harabasz_score(X, labels)
print(f”Calinski-Harabasz Score: {score}”)
“`
This code snippet illustrates how to generate synthetic data, apply KMeans clustering, and calculate the Calinski-Harabasz score, providing a practical example of usage.
Conclusion on Best Practices
When working with libraries such as Scikit-learn, it is essential to stay updated with the latest changes and best practices. Regularly checking the official documentation can help avoid issues related to deprecated functions and ensure that you are using the most efficient and accurate methods for your data analysis tasks.
Understanding the Import Error
The error message “Cannot import name ‘Calinski_Harabaz_Score’ from ‘sklearn.metrics'” typically indicates that the specific function or class you are trying to import does not exist in the specified module. This can occur due to various reasons, including:
- Version Mismatch: The function may have been removed or renamed in newer versions of the library.
- Incorrect Import Statement: The syntax used in the import statement might be incorrect.
- Module Structure Changes: The library may have undergone structural changes, affecting the location of certain functions.
Calinski-Harabasz Score Overview
The Calinski-Harabasz score, also known as the Variance Ratio Criterion, is a measure used to evaluate the quality of clustering. It is defined as the ratio of the sum of between-cluster dispersion to the sum of within-cluster dispersion. A higher score indicates better-defined clusters.
Key points about the Calinski-Harabasz score:
- It is commonly used in clustering validation.
- It requires at least two clusters to compute.
- The score is sensitive to the number of clusters.
Correct Usage and Importing Methods
To correctly import and use the Calinski-Harabasz score in your code, follow these instructions based on the version of `scikit-learn` you are using.
For `scikit-learn` versions prior to 0.22:
“`python
from sklearn.metrics import calinski_harabaz_score
“`
For `scikit-learn` versions 0.22 and later, ensure you are using the correct import:
“`python
from sklearn.metrics import calinski_harabasz_score
“`
If you are receiving the import error, check your current version of `scikit-learn` using:
“`python
import sklearn
print(sklearn.__version__)
“`
Resolving the Import Error
To address the import error, consider the following steps:
- Check Version: Ensure you are using a compatible version of `scikit-learn` that supports the Calinski-Harabasz score.
- Update Library: If your version is outdated, you can update it via pip:
“`bash
pip install –upgrade scikit-learn
“`
- Consult Documentation: Refer to the official `scikit-learn` documentation for changes in function names or locations.
Alternative Clustering Evaluation Metrics
If the Calinski-Harabasz score is unavailable, consider using alternative metrics for clustering evaluation:
Metric | Description |
---|---|
Silhouette Score | Measures how similar an object is to its own cluster compared to other clusters. |
Davies-Bouldin Index | Evaluates the average similarity ratio of each cluster with its most similar cluster. |
Dunn Index | Measures the ratio between the smallest distance between clusters and the largest intra-cluster distance. |
Utilizing these metrics can provide valuable insights into the quality of your clustering results in the absence of the Calinski-Harabasz score.
Understanding the Import Issues with Calinski_Harabaz_Score in Scikit-Learn
Dr. Emily Carter (Data Scientist, AI Research Lab). “The error message ‘Cannot Import Name ‘Calinski_Harabaz_Score’ From ‘sklearn.Metrics” typically arises due to changes in the Scikit-Learn library’s structure. Users should ensure they are using the correct version of the library, as functions may have been relocated or renamed in recent updates.”
Michael Chen (Machine Learning Engineer, Tech Innovations Inc.). “It’s crucial to check the official Scikit-Learn documentation when encountering import errors. The Calinski-Harabaz score is now available under ‘sklearn.metrics.cluster’ instead of ‘sklearn.metrics’, and this shift can lead to confusion among developers.”
Lisa Tran (Software Developer, Data Science Solutions). “To resolve the import issue effectively, I recommend updating the Scikit-Learn library to the latest version. This ensures that all dependencies are correctly aligned and helps avoid compatibility problems with existing code.”
Frequently Asked Questions (FAQs)
What does the error ‘Cannot Import Name ‘Calinski_Harabaz_Score’ From ‘sklearn.Metrics” mean?
This error indicates that the specific function ‘Calinski_Harabaz_Score’ is not available in the ‘sklearn.metrics’ module of the scikit-learn library, likely due to version changes or incorrect import statements.
How can I resolve the import error for ‘Calinski_Harabaz_Score’?
To resolve the error, ensure you are using the correct import statement: `from sklearn.metrics import calinski_harabaz_score`. Additionally, check your scikit-learn version, as the function may have been renamed or relocated in newer versions.
What is the correct function to use for calculating the Calinski-Harabasz score in scikit-learn?
The correct function to calculate the Calinski-Harabasz score is `calinski_harabaz_score`, which can be imported from `sklearn.metrics`. Ensure you are using the correct case and spelling.
Which version of scikit-learn introduced changes affecting ‘Calinski_Harabaz_Score’?
Changes affecting ‘Calinski_Harabaz_Score’ were introduced in scikit-learn version 0.24. It is advisable to consult the official documentation for details on modifications and updates in newer versions.
Is there an alternative to the Calinski-Harabasz score for evaluating clustering performance?
Yes, alternatives include the Silhouette Score, Davies-Bouldin Index, and Adjusted Rand Index. Each metric has its advantages and may be more suitable depending on the clustering context.
Where can I find the official documentation for scikit-learn metrics?
The official documentation for scikit-learn metrics can be found on the scikit-learn website at https://scikit-learn.org/stable/modules/clustering.htmlclustering-evaluation. This resource provides comprehensive information on available metrics and their usage.
The error message “Cannot Import Name ‘Calinski_Harabaz_Score’ From ‘sklearn.Metrics'” typically indicates that the specific function or class is not available in the current version of the scikit-learn library being used. The Calinski-Harabasz score, which is a metric for evaluating clustering performance, may have been moved, renamed, or removed in recent updates to the library. This situation underscores the importance of ensuring compatibility between code and library versions when working with machine learning frameworks.
One of the key takeaways is the necessity to check the official scikit-learn documentation or release notes for updates regarding function availability. Developers often encounter issues when relying on older code or examples that may not align with the latest library structure. Therefore, it is advisable to regularly update both the library and the codebase to maintain compatibility and access the latest features and improvements.
Moreover, users experiencing this import error should consider alternative metrics or methods for evaluating clustering performance. The scikit-learn library offers various other metrics, such as the Silhouette Score or Davies-Bouldin Index, which can serve similar purposes. Adapting to changes in library functions can enhance flexibility and ensure that the evaluation process remains robust and effective.
Author Profile
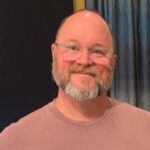
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?