How Do You Run a TypeScript File Effectively?
How to Run a TypeScript File
In the ever-evolving landscape of web development, TypeScript has emerged as a powerful ally for developers seeking to enhance their JavaScript experience. With its static typing and advanced features, TypeScript not only improves code quality but also boosts productivity by catching errors early in the development process. If you’ve ever wondered how to harness the full potential of TypeScript and execute your TypeScript files seamlessly, you’re in the right place. This guide will walk you through the essential steps to get your TypeScript code up and running, whether you’re a seasoned developer or just starting your coding journey.
Running a TypeScript file may seem daunting at first, especially if you’re accustomed to the dynamic nature of JavaScript. However, the process is straightforward once you grasp the basics. You’ll need to set up your environment correctly, which typically involves installing the TypeScript compiler and configuring your project. Understanding the fundamental commands and tools will empower you to execute your TypeScript files effortlessly, allowing you to focus on what truly matters: writing clean, efficient code.
As we delve deeper into this topic, we will explore various methods for running TypeScript files, from using the command line to integrating with build tools and IDEs. Whether you’re looking to compile your code for production or simply
Installing TypeScript
To run a TypeScript file, you first need to have TypeScript installed on your system. You can do this easily through npm (Node Package Manager). Follow these steps:
- Ensure you have Node.js installed. You can verify this by running `node -v` and `npm -v` in your terminal or command prompt.
- Install TypeScript globally by executing the following command:
“`bash
npm install -g typescript
“`
This command downloads and installs the TypeScript compiler globally on your machine, allowing you to run TypeScript commands from anywhere.
Compiling TypeScript Files
Once TypeScript is installed, you can compile your TypeScript files (.ts) into JavaScript (.js) files. The TypeScript compiler (tsc) facilitates this process.
To compile a TypeScript file, follow these steps:
- Open your terminal or command prompt.
- Navigate to the directory containing your TypeScript file.
- Run the following command:
“`bash
tsc yourfile.ts
“`
This will create a JavaScript file named `yourfile.js` in the same directory.
Alternatively, you can compile all TypeScript files in a directory by executing:
“`bash
tsc
“`
This command requires a `tsconfig.json` file in your project directory, which contains configuration settings for the TypeScript compiler.
Running Compiled JavaScript
After compiling your TypeScript code to JavaScript, you can execute the resulting JavaScript file using Node.js. Here’s how:
- In your terminal, run the following command:
“`bash
node yourfile.js
“`
This command will execute the compiled JavaScript file, allowing you to see the output of your TypeScript code.
Using ts-node for Direct Execution
If you prefer to skip the compilation step and run TypeScript files directly, you can use `ts-node`. This is a utility that allows you to run TypeScript files without pre-compiling them.
To use `ts-node`, you first need to install it:
“`bash
npm install -g ts-node
“`
Once installed, you can run your TypeScript file directly by executing:
“`bash
ts-node yourfile.ts
“`
This command compiles and executes the TypeScript file in one step, streamlining the development process.
Common Command-Line Options
When using the TypeScript compiler (tsc), several command-line options can enhance your workflow. Here are a few commonly used options:
Option | Description |
---|---|
-w | Watch for changes and recompile automatically. |
–outDir | Specify an output directory for the compiled files. |
–target | Specify ECMAScript target version (e.g., ES5, ES6). |
–sourceMap | Generate corresponding .map files for debugging. |
These options can be combined to customize the behavior of the TypeScript compiler, making your development process more efficient.
Setting Up Your Environment
To run a TypeScript file, you first need to ensure your development environment is properly configured. This involves installing Node.js and the TypeScript compiler.
- Install Node.js: Download and install Node.js from the [official website](https://nodejs.org/). This installation includes npm (Node Package Manager), which will be used to install TypeScript.
- Install TypeScript: Open your terminal or command prompt and run the following command to install TypeScript globally:
“`bash
npm install -g typescript
“`
- Verify Installation: Confirm that TypeScript is installed by checking the version:
“`bash
tsc -v
“`
Creating a TypeScript File
Once your environment is set up, you can create a TypeScript file.
- Create a new file: Use a code editor (e.g., Visual Studio Code, Sublime Text) and create a new file with a `.ts` extension, for example, `app.ts`.
- Write TypeScript code: Add your TypeScript code in this file. Here’s a simple example:
“`typescript
const greeting: string = “Hello, TypeScript!”;
console.log(greeting);
“`
Compiling TypeScript to JavaScript
TypeScript needs to be compiled into JavaScript before it can be executed. This can be done using the TypeScript compiler (tsc).
- Compile the file: Open your terminal, navigate to the directory containing your `.ts` file, and run:
“`bash
tsc app.ts
“`
- Output: This command will generate a corresponding `app.js` file in the same directory.
Running the Compiled JavaScript File
After compiling your TypeScript file, you can run the resulting JavaScript file using Node.js.
- Run the JavaScript file: In your terminal, execute the following command:
“`bash
node app.js
“`
- Expected Output: If everything is set up correctly, you should see the output:
“`
Hello, TypeScript!
“`
Using ts-node for Direct Execution
For convenience, you can use `ts-node`, a TypeScript execution engine for Node.js, which allows you to run TypeScript files directly without compiling them first.
- Install ts-node: Run the following command to install `ts-node` globally:
“`bash
npm install -g ts-node
“`
- Run TypeScript file: Now, you can execute your TypeScript file directly by running:
“`bash
ts-node app.ts
“`
This method simplifies the process, especially during development.
Common Errors and Troubleshooting
When working with TypeScript, you may encounter some common errors. Here are a few troubleshooting tips:
Error Type | Description | Solution |
---|---|---|
`Cannot find module` | The specified module does not exist. | Ensure the module is installed. |
`Type ‘X’ is not assignable` | Type mismatch error. | Check variable types and assignments. |
`Unexpected token` | Syntax error in TypeScript code. | Review the code for syntax issues. |
By following these guidelines, you can efficiently run TypeScript files and troubleshoot common issues that may arise during the development process.
Expert Insights on Running TypeScript Files
Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “To run a TypeScript file, it is essential to first ensure that TypeScript is installed globally on your machine. You can do this using the command `npm install -g typescript`. Once installed, you can compile your TypeScript file using `tsc yourfile.ts`, which will generate a corresponding JavaScript file. Finally, run the compiled JavaScript file with Node.js using `node yourfile.js`.”
James Patel (Lead Developer Advocate, CodeCraft). “Utilizing TypeScript’s built-in features can significantly enhance your development experience. After installing TypeScript, consider using `ts-node` for a more streamlined approach. This allows you to run TypeScript files directly without the need for manual compilation. Simply execute `npx ts-node yourfile.ts` to see your code in action immediately.”
Sarah Johnson (Technical Writer, Dev Insights). “Understanding how to run TypeScript files is crucial for developers transitioning from JavaScript. I recommend setting up a TypeScript configuration file (`tsconfig.json`) to manage compiler options effectively. This file allows you to define how TypeScript files are handled, making it easier to run your projects with `tsc` or `ts-node` as your needs evolve.”
Frequently Asked Questions (FAQs)
How do I compile a TypeScript file?
To compile a TypeScript file, use the TypeScript compiler (tsc). Run the command `tsc filename.ts` in your terminal, where `filename.ts` is the name of your TypeScript file. This will generate a corresponding JavaScript file.
What command do I use to run a TypeScript file directly?
You can run a TypeScript file directly using the `ts-node` package. First, install it using `npm install -g ts-node`, then execute your TypeScript file with the command `ts-node filename.ts`.
Is it necessary to install TypeScript globally to run a TypeScript file?
No, it is not necessary to install TypeScript globally. You can install it locally in your project using `npm install typescript`, and then run it using npx with the command `npx tsc filename.ts`.
Can I run TypeScript files in a browser?
TypeScript files cannot be run directly in a browser. They must be compiled into JavaScript first. After compilation, you can include the generated JavaScript file in your HTML.
What is the difference between using `tsc` and `ts-node`?
`tsc` compiles TypeScript files into JavaScript files, which can then be executed. `ts-node`, on the other hand, allows you to run TypeScript files directly without the need for prior compilation, making it useful for development and testing.
How can I configure TypeScript to watch for changes in files?
You can configure TypeScript to watch for changes by using the `–watch` flag with the `tsc` command, like so: `tsc –watch`. This will automatically recompile your TypeScript files whenever they are modified.
In summary, running a TypeScript file involves several straightforward steps that ensure the code is executed correctly. First, it is essential to have TypeScript installed on your system, which can be done using npm. After installation, you can compile TypeScript files into JavaScript using the TypeScript compiler (tsc). This compilation step is crucial as browsers and Node.js cannot directly execute TypeScript code.
Once the TypeScript file is compiled, you can run the resulting JavaScript file using Node.js or any web browser. For Node.js, the command is simply ‘node filename.js’, where ‘filename.js’ is the output JavaScript file. In a browser environment, you can include the compiled JavaScript file in your HTML document, allowing it to run as part of your web application.
Additionally, utilizing tools like ts-node can streamline the process by allowing you to run TypeScript files directly without the need for separate compilation. This approach is particularly useful during development, as it saves time and simplifies the workflow. Understanding these steps and tools is vital for effective TypeScript development and execution.
Author Profile
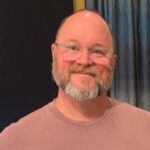
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?