How Can You Send an Email Using Python?
In today’s digital age, effective communication is paramount, and email remains one of the most widely used methods for connecting with others. Whether you’re sending a quick update, a formal report, or an automated notification, understanding how to send an email programmatically can save you time and streamline your workflows. Python, with its simplicity and versatility, offers a powerful avenue for automating email tasks, making it an invaluable skill for developers, data analysts, and anyone looking to enhance their productivity.
Sending an email from Python might seem daunting at first, but with the right tools and libraries, it becomes a straightforward process. Python’s built-in libraries, along with third-party packages, provide robust functionalities that allow you to compose, send, and even manage emails effortlessly. From setting up your SMTP server to crafting the perfect message, this article will guide you through the essentials of email automation, ensuring you can communicate effectively without the manual hassle.
As we delve deeper into the intricacies of sending emails from Python, you’ll discover various methods to customize your messages, attach files, and handle errors gracefully. Whether you’re a seasoned programmer or just starting your coding journey, mastering email automation in Python will open up new possibilities for your projects and enhance your overall efficiency. Get ready to unlock the potential of Python as a powerful
Using the smtplib Library
To send an email from Python, the most commonly used library is `smtplib`. This library allows you to establish a connection with an SMTP server to send your email. Here’s a basic example of how to use `smtplib`:
python
import smtplib
from email.mime.text import MIMEText
def send_email(subject, body, to_email):
from_email = “[email protected]”
password = “your_password”
msg = MIMEText(body)
msg[‘Subject’] = subject
msg[‘From’] = from_email
msg[‘To’] = to_email
with smtplib.SMTP(‘smtp.example.com’, 587) as server:
server.starttls() # Upgrade the connection to a secure encrypted SSL/TLS
server.login(from_email, password)
server.send_message(msg)
send_email(“Test Subject”, “This is a test email body.”, “[email protected]”)
In this example, replace `smtp.example.com` with your SMTP server address, and provide your email credentials. The `starttls()` method is used for secure transmission.
Using the Email Message Class
For more complex emails, you can utilize the `EmailMessage` class from the `email` module. This class simplifies the process of creating multipart messages and attachments. Below is an example demonstrating how to send an email with a plain text message:
python
from email.message import EmailMessage
def send_email_with_attachment(subject, body, to_email, attachment_path):
from_email = “[email protected]”
password = “your_password”
msg = EmailMessage()
msg[‘Subject’] = subject
msg[‘From’] = from_email
msg[‘To’] = to_email
msg.set_content(body)
# Adding an attachment
with open(attachment_path, ‘rb’) as attachment:
msg.add_attachment(attachment.read(), maintype=’application’, subtype=’octet-stream’, filename=attachment_path)
with smtplib.SMTP(‘smtp.example.com’, 587) as server:
server.starttls()
server.login(from_email, password)
server.send_message(msg)
send_email_with_attachment(“Subject with Attachment”, “Email body.”, “[email protected]”, “path/to/attachment.txt”)
This code snippet illustrates how to include an attachment when sending an email.
Common SMTP Servers
When sending emails, you often need to know the SMTP server of your email provider. Below is a table that lists some common SMTP servers and their settings:
Provider | SMTP Server | Port | SSL/TLS |
---|---|---|---|
Gmail | smtp.gmail.com | 587 | TLS |
Outlook | smtp.office365.com | 587 | TLS |
Yahoo | smtp.mail.yahoo.com | 587 | TLS |
iCloud | smtp.mail.me.com | 587 | TLS |
Make sure to verify the settings and requirements for your specific email provider, as they may require app-specific passwords or additional security settings.
Handling Errors
When sending emails programmatically, it is essential to handle potential errors gracefully. Common issues include incorrect login credentials, connection failures, and SMTP server errors. You can manage these exceptions using a try-except block:
python
try:
with smtplib.SMTP(‘smtp.example.com’, 587) as server:
server.starttls()
server.login(from_email, password)
server.send_message(msg)
except smtplib.SMTPAuthenticationError:
print(“Authentication error: Check your username/password.”)
except smtplib.SMTPConnectError:
print(“Failed to connect to the server.”)
except Exception as e:
print(f”An error occurred: {e}”)
This practice will help you identify and troubleshoot issues effectively when sending emails from your Python application.
Setting Up Your Environment
To send emails using Python, you need to ensure that your environment is properly set up. This involves installing the necessary libraries and configuring your email account for access.
- Python Installation: Ensure Python is installed on your system. You can download it from [python.org](https://www.python.org/downloads/).
- Required Libraries: The primary library used for sending emails in Python is `smtplib`, which comes pre-installed with Python. However, for more advanced features, you may want to install `email` and `ssl` libraries. If you plan to use third-party services like Gmail or others, consider using the `yagmail` library for easier handling.
To install `yagmail`, run:
bash
pip install yagmail
Using smtplib to Send Emails
The `smtplib` library allows you to send emails using the Simple Mail Transfer Protocol (SMTP). Below is a basic example of how to use it.
python
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
# Email configuration
sender_email = “[email protected]”
receiver_email = “[email protected]”
password = “your_password”
# Create the email content
subject = “Test Email”
body = “This is a test email sent from Python.”
# Set up the MIME
message = MIMEMultipart()
message[“From”] = sender_email
message[“To”] = receiver_email
message[“Subject”] = subject
message.attach(MIMEText(body, “plain”))
# Send the email
with smtplib.SMTP(“smtp.example.com”, 587) as server:
server.starttls() # Secure the connection
server.login(sender_email, password)
server.send_message(message)
Key Points:
- Replace `smtp.example.com` with your email provider’s SMTP server (e.g., `smtp.gmail.com` for Gmail).
- Ensure you use the correct port (587 for TLS, 465 for SSL).
- Use `starttls()` for a secure connection.
Using yagmail for Simplicity
`yagmail` simplifies email sending significantly. After installing `yagmail`, follow these steps:
- Set Up Your Email: Store your email credentials securely. You can set them up using:
bash
yagmail –set [email protected]
- Sending an Email: Use the following code to send an email easily:
python
import yagmail
yag = yagmail.SMTP(“[email protected]”)
yag.send(to=”[email protected]”, subject=”Test Email”, contents=”This is a test email sent from Python using yagmail.”)
Advantages of Using yagmail:
- Simplifies sending emails with attachments and HTML content.
- Automatically handles login and security.
Handling Attachments
When sending emails, you may need to include attachments. Here’s how to do it with both `smtplib` and `yagmail`.
Using smtplib:
python
from email.mime.base import MIMEBase
from email import encoders
# Attach a file
filename = “example.pdf”
with open(filename, “rb”) as attachment:
part = MIMEBase(“application”, “octet-stream”)
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header(“Content-Disposition”, f”attachment; filename={filename}”)
message.attach(part)
Using yagmail:
python
yag.send(to=”[email protected]”, subject=”Test Email with Attachment”, contents=”Please find the attached file.”, attachments=”example.pdf”)
Security Considerations
When dealing with email credentials in your code, keep the following in mind:
- Environment Variables: Store sensitive information such as email passwords in environment variables instead of hardcoding them.
- Two-Factor Authentication: For services like Gmail, enable two-factor authentication and use an app password for SMTP access.
- SSL/TLS: Always use secure connections when sending emails to protect your data.
Expert Insights on Sending Emails from Python
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Utilizing Python’s built-in libraries, such as smtplib, allows developers to seamlessly send emails with minimal code. It is essential to understand the security implications, especially when handling sensitive information.”
Michael Chen (Lead Data Scientist, AI Solutions Group). “When sending emails from Python, consider using third-party services like SendGrid or Mailgun. These platforms not only simplify the process but also provide advanced features such as tracking and analytics.”
Sarah Thompson (Python Developer and Instructor, Code Academy). “For beginners, I recommend starting with simple SMTP configurations. It is crucial to test your email functionality in a controlled environment to avoid issues like spam filtering.”
Frequently Asked Questions (FAQs)
How do I send a simple email using Python?
You can send a simple email using Python’s built-in `smtplib` library. First, set up an SMTP server connection, then create a message using the `email` library, and finally use the `sendmail` method to send the email.
What libraries are commonly used to send emails in Python?
The most commonly used libraries for sending emails in Python are `smtplib` for handling the SMTP protocol and `email` for constructing email messages. Additionally, third-party libraries like `yagmail` and `smtplib` can simplify the process.
Do I need to enable “less secure apps” in my email account to send emails from Python?
Yes, for some email providers like Gmail, you may need to enable “less secure apps” or use an App Password if you have two-factor authentication enabled. This allows external applications to send emails on your behalf.
Can I send HTML emails using Python?
Yes, you can send HTML emails using Python. When constructing your email message, specify the MIME type as `text/html` instead of `text/plain`. This allows you to include HTML content in your emails.
How can I attach files to an email in Python?
To attach files, use the `MIMEBase` class from the `email.mime` module. Create a MIME object for the file, encode it, and attach it to the email message before sending.
Is it possible to send emails asynchronously in Python?
Yes, you can send emails asynchronously using libraries like `asyncio` along with `aiosmtplib`. This allows you to send emails without blocking the execution of your program, improving performance in applications that require high concurrency.
sending an email from Python can be achieved using various libraries, with the most common being the built-in `smtplib`. This library allows users to connect to an SMTP server and send emails programmatically. To effectively send an email, one must set up the SMTP server details, authenticate the sender’s email account, and construct the email message using the `email` module for proper formatting.
Additionally, it is essential to handle exceptions and errors that may arise during the email-sending process, ensuring that the application remains robust and user-friendly. By implementing features such as HTML content, attachments, and CC/BCC options, developers can create versatile email functionalities that cater to diverse communication needs.
Key takeaways include the importance of securing email credentials and considering the use of environment variables or secure vaults for sensitive information. Furthermore, understanding the limitations and policies of the SMTP server being used is crucial to avoid issues related to email sending limits or spam filters. Overall, mastering the process of sending emails from Python can significantly enhance automation and communication capabilities in various applications.
Author Profile
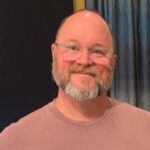
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?