Why Does the Error ‘Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy” Keep Appearing?
In the ever-evolving landscape of programming and software development, encountering errors can be both a frustrating and enlightening experience. One such error that developers may stumble upon is the perplexing message: “Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’.” This cryptic notification often emerges during the implementation of cryptographic functionalities, particularly when working with libraries that handle secure communications. Understanding the root of this issue not only aids in troubleshooting but also enhances one’s grasp of the underlying libraries and their attributes. In this article, we will delve into the intricacies of this error, exploring its implications and offering insights into effective resolution strategies.
As developers navigate through the complexities of cryptography, they frequently rely on libraries that provide essential tools for secure data transmission. However, the reliance on these modules can sometimes lead to unexpected hurdles, such as the aforementioned error. This issue typically arises when the code references an attribute that is either deprecated or not present in the current version of the library. By examining the context in which this error occurs, we can better appreciate the importance of keeping libraries updated and understanding their documentation.
Moreover, the error highlights the significance of proper error handling and debugging practices in software development. It serves as a reminder that even seasoned developers must
Understanding the Error Message
The error message `Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’` typically arises in Python environments that utilize the `cryptography` library. This issue indicates that the program is attempting to access an attribute or function that does not exist in the current context of the library. Understanding the root causes of this error can help in diagnosing and resolving the underlying issues.
Common reasons for this error include:
- Version Compatibility: The library version installed may not support the attribute in question. This often occurs when using older versions of the library that do not include newer features.
- Incorrect Import Statements: The error may stem from incorrect or missing import statements, leading to the module not being fully initialized.
- Environment Issues: Conflicts between different packages or a corrupted installation can lead to this error.
Resolving the Error
To effectively resolve the `Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’` error, follow these steps:
- Check the Installed Version: Verify the version of the `cryptography` library currently in use. You can do this by running:
“`bash
pip show cryptography
“`
- Upgrade the Library: If the version is outdated, upgrade it using:
“`bash
pip install –upgrade cryptography
“`
- Review Import Statements: Ensure that the import statements in your code are correct. For example, make sure to import the necessary components from the `cryptography` library properly.
- Create a Virtual Environment: To avoid conflicts with other packages, consider creating a virtual environment. This isolates dependencies and can help resolve issues related to package versions.
“`bash
python -m venv myenv
source myenv/bin/activate On Windows use: myenv\Scripts\activate
pip install cryptography
“`
- Consult Documentation: Check the [official cryptography documentation](https://cryptography.io/en/latest/) for updates or changes related to the attribute in question.
Common Attributes in the Cryptography Library
Understanding other attributes and their correct usage within the cryptography library can help developers avoid similar errors. Below is a table listing some common attributes and their descriptions.
Attribute | Description |
---|---|
X509 | Represents X.509 certificates. |
load_pem_x509_certificate | Loads a PEM encoded X.509 certificate. |
EllipticCurvePublicKey | Represents an elliptic curve public key. |
serialization | Contains classes for serializing and deserializing keys and certificates. |
By understanding these attributes, developers can navigate the library more effectively and reduce the likelihood of encountering similar errors in their projects.
Understanding the Error
The error message `Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’` typically indicates that the Python code is attempting to access an attribute or function that does not exist in the specified module. This can arise due to several factors, including:
- Version Mismatch: The attribute may not be available in the version of the library currently installed.
- Incorrect Import: The required module may not have been imported correctly, leading to missing attributes.
- Depricated Features: The attribute may have been removed in recent updates of the library.
Troubleshooting Steps
To resolve this issue, consider the following steps:
- Check Python and Library Versions:
- Ensure that you are using compatible versions of Python and the library (e.g., `cryptography`).
- You can check the installed version of a library using:
“`bash
pip show
- Update Libraries:
- If an older version is found, update the library to the latest version:
“`bash
pip install –upgrade
- Verify Imports:
- Confirm that you are importing the necessary modules correctly. For example:
“`python
from cryptography import x509
“`
- Consult Documentation:
- Review the official documentation for the library to see if the attribute has been moved or renamed.
Common Causes
The following are common reasons for encountering this error:
Cause | Description |
---|---|
Missing Dependency | The library may not be installed or not available in the current environment. |
Attribute Removal | The attribute could have been removed in a recent update of the library. |
Typographical Error | There might be a typo in the attribute name or import statement. |
Usage of Different APIs | The code might be referencing a different library or API that does not include the attribute. |
Example of Correct Usage
Here is an example illustrating the correct usage of an X.509 certificate in Python using the `cryptography` library:
“`python
from cryptography import x509
from cryptography.hazmat.backends import default_backend
Create a new X.509 certificate
cert = x509.CertificateBuilder().subject_name(
x509.Name([x509.NameAttribute(x509.oid.NameOID.COMMON_NAME, u”example.com”)])
).issuer_name(
x509.Name([x509.NameAttribute(x509.oid.NameOID.COMMON_NAME, u”example.com”)])
).public_key(
Add public key here
).serial_number(
x509.random_serial_number()
).not_valid_before(
Set valid from date
).not_valid_after(
Set valid until date
).sign(
Sign the certificate
private_key, x509.hashes.SHA256(), default_backend()
)
“`
Ensure that all required components are correctly defined and that the environment is correctly set up.
Understanding the ‘Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy’ Error
Dr. Emily Carter (Cryptography Specialist, SecureTech Solutions). “The error message ‘Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy” typically indicates a version mismatch in the libraries being used. It is crucial to ensure that the OpenSSL version is compatible with the Python library versions, as discrepancies can lead to such attribute errors.”
Mark Thompson (Senior Software Engineer, CyberSecure Inc.). “This specific error often arises when developers attempt to access attributes that may have been deprecated or removed in newer versions of the library. It is advisable to consult the library’s documentation for the latest updates and to verify that the correct attributes are being utilized.”
Lisa Chen (Open Source Advocate, Tech Innovators Group). “In many cases, the ‘X509_V_Flag_Notify_Policy’ attribute is not present in certain builds of the library. Users should consider installing a different build or version that includes this attribute, or alternatively, refactor their code to avoid reliance on it.”
Frequently Asked Questions (FAQs)
What does the error “Module ‘Lib’ has no attribute ‘X509_V_Flag_Notify_Policy’ mean?
This error indicates that the Python environment is attempting to access an attribute named `X509_V_Flag_Notify_Policy` within the `Lib` module, which does not exist in the current version of the library being used.
Which library is associated with the ‘X509_V_Flag_Notify_Policy’ attribute?
The attribute is related to the OpenSSL library, specifically within the context of X.509 certificate verification flags. It is typically found in the `cryptography` or `OpenSSL` Python libraries.
How can I resolve the “Module ‘Lib’ has no attribute ‘X509_V_Flag_Notify_Policy'” error?
To resolve this error, ensure that you are using the correct version of the OpenSSL library that supports the `X509_V_Flag_Notify_Policy` attribute. Updating the library or checking for compatibility with your Python version may help.
Is ‘X509_V_Flag_Notify_Policy’ available in all versions of the OpenSSL library?
No, the `X509_V_Flag_Notify_Policy` attribute is not available in all versions. It was introduced in later versions of OpenSSL, so using an outdated version may lead to this error.
What should I do if updating the library does not fix the issue?
If updating the library does not resolve the issue, consider checking your code for any compatibility issues or conflicts with other libraries. Additionally, reviewing the library’s documentation for changes in attributes or methods may provide further insights.
Where can I find more information about the OpenSSL library and its attributes?
More information can be found in the official OpenSSL documentation, as well as the documentation for the Python `cryptography` library. These resources provide detailed descriptions of available attributes and their usage.
The error message “Module ‘Lib’ Has No Attribute ‘X509_V_Flag_Notify_Policy” typically indicates an issue related to the OpenSSL library in Python, particularly when using the cryptography module. This error arises when the code attempts to access a specific attribute that is not available in the current version of the library being used. It is essential to ensure that the correct version of the cryptography library is installed and that it is compatible with the version of Python in use.
To resolve this issue, users should first verify their current library versions by using package management tools such as pip. Upgrading the cryptography library to the latest version may resolve the attribute error, as newer versions often include bug fixes and additional features. Additionally, checking the official documentation for both Python and the cryptography library can provide insights into any changes or deprecations that may have occurred in recent updates.
Furthermore, it is advisable to review the code for any potential typos or incorrect references to the X509_V_Flag_Notify_Policy attribute. Ensuring that the code adheres to the latest standards and practices can prevent similar issues in the future. In cases where the problem persists, consulting community forums or seeking assistance from experienced developers can
Author Profile
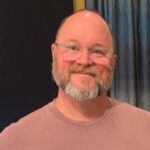
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?