What Can I Do With JavaScript? Exploring Endless Possibilities!
What Can I Do With JavaScript?
In the ever-evolving landscape of web development, JavaScript stands as a cornerstone technology that empowers developers to create dynamic and interactive experiences. Whether you’re a seasoned programmer or a curious beginner, the possibilities with JavaScript are vast and exciting. From enhancing user interfaces to building complex web applications, this versatile language has become indispensable in the toolkit of modern developers. But what exactly can you achieve with JavaScript?
As you delve deeper into the world of JavaScript, you’ll discover its remarkable ability to breathe life into static web pages, transforming them into engaging platforms that respond to user actions in real time. With libraries and frameworks like React, Angular, and Vue.js, developers can streamline their workflow and create robust applications that are both efficient and visually appealing. Moreover, JavaScript’s reach extends beyond the browser; with environments like Node.js, you can harness its power on the server side, enabling full-stack development and opening up new avenues for building scalable applications.
In addition to web development, JavaScript plays a significant role in mobile app development, game design, and even Internet of Things (IoT) projects. Its versatility allows developers to explore a myriad of creative avenues, making it a vital skill in today’s tech landscape. As we journey
Building Interactive Web Applications
JavaScript is integral to developing interactive web applications. It allows developers to create dynamic user interfaces that respond to user inputs in real-time. For instance, by using JavaScript, you can implement features such as:
- Form validation to ensure user input is correct before submission.
- Interactive elements such as sliders, tabs, and modals.
- Real-time updates through AJAX to fetch data without reloading the page.
These functionalities enhance user experience and engagement, making JavaScript a crucial tool for modern web development.
Enhancing User Experience with Animations
Animations can significantly improve the user experience by providing visual feedback and making applications feel more responsive. JavaScript libraries like GSAP and anime.js facilitate creating animations that are smooth and performant. Key aspects of using JavaScript for animations include:
- Controlling timing and sequencing of animations.
- Triggering animations based on user interactions.
- Creating complex animations that react to real-time data.
Here’s a simple example of a JavaScript animation:
javascript
const element = document.getElementById(‘myElement’);
element.style.transition = “transform 0.5s”;
element.style.transform = “translateX(100px)”;
Server-Side Development with Node.js
JavaScript is not limited to client-side development; it can also be employed on the server-side using Node.js. This runtime allows developers to build scalable network applications. Benefits of using Node.js include:
- Non-blocking, event-driven architecture, which is ideal for handling multiple simultaneous connections.
- A vast ecosystem of libraries through npm (Node Package Manager).
- The ability to use JavaScript throughout the entire development stack (both client and server).
A simple Node.js server might look like this:
javascript
const http = require(‘http’);
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader(‘Content-Type’, ‘text/plain’);
res.end(‘Hello, World!\n’);
});
server.listen(3000, () => {
console.log(‘Server running at http://localhost:3000/’);
});
Creating Mobile Applications
JavaScript frameworks such as React Native and Ionic enable developers to create mobile applications using web technologies. This approach allows for:
- Cross-platform compatibility, reducing the need to write separate codebases for iOS and Android.
- Access to native device features like GPS, camera, and notifications through JavaScript APIs.
- A large community and resources for support and development.
The following table summarizes popular frameworks for mobile app development:
Framework | Key Features |
---|---|
React Native | Native components, hot reloading, strong community support |
Ionic | Hybrid apps, extensive UI components, easy to learn |
Flutter | Fast development, expressive UI, single codebase |
Utilizing JavaScript for Game Development
JavaScript can also be leveraged to develop games, with frameworks like Phaser and Three.js supporting 2D and 3D game creation. Key advantages of using JavaScript in game development are:
- Accessibility through web browsers, allowing users to play games without installation.
- Integration with HTML5 for rich graphics and sound.
- A supportive community and numerous resources for game developers.
Developers can utilize the following code snippet to create a simple game loop:
javascript
function gameLoop() {
// Update game state
// Render game state
requestAnimationFrame(gameLoop);
}
gameLoop();
By harnessing JavaScript’s capabilities across various domains, developers can create robust applications that cater to a wide range of user needs and preferences.
Web Development
JavaScript is a cornerstone of modern web development, enabling dynamic interactions on websites. It allows developers to create responsive user interfaces and enhance the user experience. Key areas where JavaScript is utilized include:
- Front-End Development:
- Building interactive web applications using frameworks like React, Angular, or Vue.js.
- Manipulating the DOM to update content, styles, and structure dynamically.
- Back-End Development:
- Utilizing Node.js to build server-side applications and APIs.
- Handling database operations with frameworks like Express.js.
- Full-Stack Development:
- Combining front-end and back-end skills to develop complete web applications.
- Using tools like MERN (MongoDB, Express.js, React, Node.js) stack.
Mobile App Development
JavaScript extends its capabilities to mobile app development through frameworks and libraries that allow developers to create cross-platform applications. Notable tools include:
- React Native: Enables building mobile applications using React.
- Ionic: A framework that allows developers to create hybrid mobile applications using web technologies.
- NativeScript: Allows building native mobile applications using JavaScript.
Game Development
JavaScript is also a popular choice for game development, particularly for web-based games. Tools and libraries that facilitate this include:
- Phaser: A powerful HTML5 game framework for 2D game development.
- Three.js: A library for creating 3D graphics in the browser.
- Babylon.js: A framework for building 3D games with an emphasis on simplicity and performance.
Server-Side Programming
With the advent of Node.js, JavaScript has become a viable option for server-side programming. This environment allows developers to create scalable network applications. Key features include:
- Event-Driven Architecture: Facilitates handling multiple connections simultaneously.
- Non-Blocking I/O: Enhances performance by allowing operations to proceed without waiting for others to finish.
Data Visualization
JavaScript is instrumental in data visualization, allowing developers to create interactive charts and graphs. Libraries that facilitate this include:
- D3.js: A powerful library for producing dynamic, interactive data visualizations.
- Chart.js: A simple yet flexible library for creating various types of charts.
- Plotly.js: A library for making interactive graphs and dashboards.
Desktop Application Development
JavaScript can also be used for building desktop applications. Frameworks that enable this functionality include:
- Electron: Allows developers to create cross-platform desktop applications using web technologies.
- NW.js: Combines Node.js and Chromium to build desktop applications.
Internet of Things (IoT)
JavaScript is increasingly being adopted in the IoT space for developing applications that connect and control devices. Frameworks and platforms include:
- Johnny-Five: A JavaScript Robotics and IoT platform.
- Node-RED: A flow-based development tool for visual programming of IoT applications.
Automation and Scripting
JavaScript can be leveraged for automating tasks and scripting, particularly in web environments. Use cases include:
- Web Scraping: Using libraries like Puppeteer to automate browser tasks.
- Task Automation: Creating scripts that automate repetitive tasks in web applications.
Artificial Intelligence and Machine Learning
JavaScript has made strides into AI and ML, with libraries enabling developers to incorporate intelligent features into applications. Notable libraries include:
- TensorFlow.js: Allows for training and deploying machine learning models in the browser.
- Brain.js: A library that enables neural networks to be created and trained in JavaScript.
Exploring the Versatility of JavaScript in Modern Development
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “JavaScript is not just a web scripting language; it has evolved into a powerful tool for building full-scale applications. With frameworks like Node.js, developers can create server-side applications, making JavaScript a full-stack solution.”
Michael Harris (Lead Front-End Developer, Creative Solutions Agency). “The ability to manipulate the Document Object Model (DOM) in real-time allows JavaScript to create dynamic user interfaces. This interactivity is crucial for enhancing user experience in web applications.”
Linda Patel (JavaScript Educator, Code Academy). “Learning JavaScript opens doors to various career paths, from web development to mobile app development using frameworks like React Native. Its versatility makes it an essential skill for aspiring developers.”
Frequently Asked Questions (FAQs)
What can I do with JavaScript in web development?
JavaScript enables interactive features on websites, such as form validation, dynamic content updates, animations, and user interface enhancements. It is essential for creating responsive web applications.
Can I use JavaScript for server-side programming?
Yes, JavaScript can be used on the server side, primarily through Node.js. This allows developers to build scalable network applications and handle server-side logic, file operations, and database interactions.
Is JavaScript suitable for mobile app development?
Absolutely. JavaScript can be utilized in mobile app development through frameworks like React Native and Ionic, allowing developers to create cross-platform applications that run on both Android and iOS.
What role does JavaScript play in game development?
JavaScript is widely used in game development, particularly for browser-based games. Libraries like Phaser and Three.js facilitate the creation of 2D and 3D games, enabling rich interactive experiences.
Can I use JavaScript for data visualization?
Yes, JavaScript is highly effective for data visualization. Libraries such as D3.js, Chart.js, and Plotly allow developers to create interactive charts and graphs, making data analysis more accessible and engaging.
How does JavaScript enhance user experience on websites?
JavaScript enhances user experience by enabling real-time updates, interactive elements, and personalized content delivery. It allows for smoother navigation and improved engagement through features like AJAX, which loads content without refreshing the page.
JavaScript is an incredibly versatile programming language that plays a crucial role in web development. It enables developers to create interactive and dynamic web pages, enhancing user experience significantly. With JavaScript, one can manipulate the Document Object Model (DOM), allowing for real-time updates and changes to the content displayed on a webpage without requiring a full page reload. This capability is essential for modern web applications, where user engagement and responsiveness are paramount.
Beyond web development, JavaScript has expanded its reach into server-side programming through environments like Node.js. This allows developers to use JavaScript for backend development, enabling full-stack development capabilities with a single language. Additionally, JavaScript can be utilized in mobile app development through frameworks such as React Native, making it possible to build cross-platform applications efficiently. The language’s ecosystem is rich with libraries and frameworks, such as Angular, Vue.js, and jQuery, which further streamline the development process and enhance functionality.
Moreover, JavaScript is not limited to traditional applications; it also plays a significant role in emerging technologies such as Internet of Things (IoT) and machine learning. With libraries like TensorFlow.js, developers can run machine learning models directly in the browser, opening up new possibilities for data-driven applications.
Author Profile
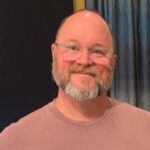
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?