How Can You Effectively Squash All Commits in a Git Branch?
In the world of version control, particularly when using Git, the ability to manage your commit history is crucial for maintaining a clean and organized project. One powerful technique that developers often turn to is squashing commits. Whether you’re tidying up your commit history before merging a feature branch into the main branch or simply looking to streamline your project’s development timeline, knowing how to squash all commits in a branch can be a game-changer. This process not only enhances readability but also simplifies the history for future collaborators, making it easier to understand the evolution of the codebase.
Squashing commits involves combining multiple commits into a single, cohesive commit. This is particularly useful for branches that have accumulated a series of incremental changes that may clutter the project history. By learning how to squash commits effectively, you can ensure that your project’s history reflects meaningful milestones rather than a series of minor adjustments. The process can seem daunting at first, especially for those new to Git, but with a clear understanding of the steps involved, you can master this technique and elevate your version control skills.
In this article, we will explore the various methods for squashing commits, the best practices to follow, and the potential pitfalls to avoid. Whether you’re a seasoned developer or just starting out, this guide
Understanding the Rebase Command
Rebasing is a powerful Git feature that allows you to integrate changes from one branch into another. When squashing commits, rebase is often the method of choice as it allows for a cleaner history. The command `git rebase` can be used in several ways, but in this context, we focus on the interactive mode that enables you to edit commit messages and squash multiple commits into a single one.
To initiate an interactive rebase, you will use the following command:
“`
git rebase -i HEAD~n
“`
Here, `n` is the number of commits you want to consider for squashing. For example, if you want to squash the last 5 commits, you would replace `n` with `5`.
Steps to Squash Commits
Follow these steps to effectively squash commits in a branch:
- Start Interactive Rebase: Execute the rebase command with the desired number of commits.
- Select Commits to Squash: An editor will open, displaying a list of the last `n` commits. The commits are prefixed by the word `pick`.
- Modify the List: Change the word `pick` to `squash` (or simply `s`) for the commits you want to combine with the first commit in the list.
- Save and Exit: After adjusting the list, save the changes and exit the editor.
- Edit Commit Message: Another editor will open, allowing you to write a new commit message for the squashed commit. This can be a combination of the previous messages or a completely new one.
- Finalize the Rebase: Save and exit again to complete the rebase process.
Here is a basic example of what the commit list may look like in the editor:
“`
pick a1b2c3d Commit message 1
squash a2b3c4e Commit message 2
squash a3b4c5f Commit message 3
“`
Handling Conflicts During Rebase
During the rebase process, you might encounter merge conflicts. If this happens, Git will pause the rebase and allow you to resolve the conflicts. Follow these steps to manage conflicts:
- Identify the files with conflicts.
- Open the conflicting files and resolve the conflicts manually.
- After resolving, stage the changes using:
“`
git add
“`
- Continue the rebase process with:
“`
git rebase –continue
“`
If you wish to abort the rebase at any point, you can use:
“`
git rebase –abort
“`
Best Practices
When squashing commits, consider the following best practices to maintain a clean commit history:
- Squash Related Commits: Only squash commits that are closely related to the same feature or bug fix.
- Use Meaningful Commit Messages: Ensure the final commit message conveys the purpose of the changes succinctly.
- Test After Rebase: Always run tests to ensure that the code remains functional after squashing commits.
Action | Command |
---|---|
Start Interactive Rebase | git rebase -i HEAD~n |
Continue After Conflict | git rebase –continue |
Abort Rebase | git rebase –abort |
Understanding Git Rebase and Squashing Commits
To effectively squash all commits in a branch, it is essential to understand how Git rebase functions. Git rebase allows you to rewrite commit history by moving or combining commits. This is particularly useful for cleaning up a branch before merging it into the main branch.
- Rebase vs. Merge:
- Rebase: Applies commits from one branch onto another, creating a linear history.
- Merge: Combines branches and creates a merge commit, which can lead to a more complex history.
Steps to Squash All Commits in a Branch
Follow these steps to squash all commits in a specific branch:
- Checkout the Branch: Start by checking out the branch you want to squash.
“`bash
git checkout
“`
- Initiate Interactive Rebase: Use the interactive rebase command to start the process.
“`bash
git rebase -i HEAD~
“`
Replace `
- Edit the Rebase File: An editor will open displaying the list of commits. The format typically looks like this:
“`
pick
pick
pick
“`
- Change the word `pick` to `squash` (or `s`) for all commits except the first one. This means you want to combine these commits into the first commit.
- Save and Close the Editor: After making changes, save the file and close the editor.
- Edit the Commit Message: Another editor will open allowing you to edit the combined commit message. You can retain the messages of the squashed commits or write a new one.
- Finalize the Rebase: Save and close the editor again to complete the rebase process.
Handling Conflicts During Rebase
If conflicts arise during the rebase, Git will pause the process and prompt you to resolve them. Here’s how to handle conflicts:
- Identify Conflicts: Check the files with conflicts, indicated by the conflict markers (e.g., `<<<<<<<`, `=======`, `>>>>>>>`).
- Resolve Conflicts: Edit the files to resolve the issues, then mark them as resolved.
“`bash
git add
“`
- Continue the Rebase: After resolving all conflicts, continue the rebase process.
“`bash
git rebase –continue
“`
- Abort the Rebase (if necessary): If you encounter issues and wish to cancel the rebase, you can do so with:
“`bash
git rebase –abort
“`
Verifying the Squashed Commit
Once the rebase is complete, it is crucial to verify the changes:
- Check Commit History: Use the following command to view the new commit history.
“`bash
git log –oneline
“`
- Inspect Changes: Review the changes to ensure everything is as expected.
“`bash
git diff HEAD~1
“`
Force Pushing Changes to Remote
If the branch has been pushed to a remote repository, you will need to force push the changes due to the rewritten history.
- Force Push Command:
“`bash
git push origin
“`
This command replaces the remote branch with your squashed commits, ensuring that your local changes are reflected remotely.
Expert Insights on Squashing Commits in Version Control
Dr. Emily Chen (Senior Software Engineer, CodeCraft Solutions). “Squashing commits is a powerful technique that helps maintain a clean and readable project history. It is particularly beneficial when preparing for a pull request, as it consolidates changes and makes it easier for reviewers to understand the evolution of the code.”
Michael Thompson (DevOps Specialist, Agile Innovations). “When squashing commits, it is crucial to ensure that the commit messages are meaningful and descriptive. This practice not only enhances clarity but also aids in tracking the project’s progress over time, making it easier for new team members to get up to speed.”
Sarah Patel (Git Trainer and Author, Version Control Academy). “While squashing commits can simplify the commit history, it is essential to communicate with your team before doing so. This ensures that everyone is aware of the changes and can adjust their workflows accordingly, preventing confusion and potential merge conflicts.”
Frequently Asked Questions (FAQs)
What does it mean to squash commits in a branch?
Squashing commits refers to the process of combining multiple commit entries into a single commit. This is often done to create a cleaner project history by reducing clutter from minor commits.
How do I squash all commits in a branch using Git?
To squash all commits in a branch, you can use the command `git rebase -i HEAD~n`, where `n` is the number of commits you want to squash. In the interactive rebase interface, change the word “pick” to “squash” for the commits you wish to combine.
Will squashing commits affect the commit history?
Yes, squashing commits will rewrite the commit history of the branch. This can make it difficult to track changes made in the individual commits, but it provides a cleaner and more concise history.
Can I squash commits that have already been pushed to a remote repository?
Yes, you can squash commits that have been pushed to a remote repository, but you will need to force push the changes using `git push origin branch-name –force`. This can overwrite the history on the remote, so it should be done with caution.
What are the risks of squashing commits?
The primary risk of squashing commits is the potential loss of detailed commit history, which may include important context for changes. Additionally, if others are collaborating on the same branch, force pushing after a squash can lead to conflicts.
Is there a way to squash commits without losing the commit messages?
Yes, during the interactive rebase, you can choose to keep the commit messages by editing them in the rebase interface. You can also use the `–squash` option with `git merge` to combine commits while preserving messages.
Squashing all commits in a branch is a powerful technique in version control systems, particularly in Git. This process involves combining multiple commit entries into a single cohesive commit. It is often used to clean up a branch’s history before merging into the main branch, ensuring that the commit log remains concise and meaningful. By squashing commits, developers can eliminate unnecessary noise in the commit history, making it easier for others to understand the evolution of the codebase.
To squash commits, developers typically use the interactive rebase feature in Git. This allows users to select a range of commits and choose the option to squash them into one. The result is a single commit that encapsulates all the changes made in the squashed commits, along with a new commit message that can summarize the overall changes. This process not only simplifies the commit history but also enhances collaboration by providing a clearer narrative of the changes made in a feature branch.
It is essential to note that squashing commits rewrites the commit history, which can lead to complications if the branch has already been shared with others. Therefore, it is advisable to squash commits before pushing changes to a shared repository. Additionally, developers should communicate with their team to avoid conflicts and ensure that everyone is aligned on
Author Profile
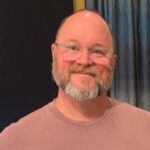
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?