How Can You Effectively Migrate From Postgres Enum To String?
In the world of database management, the choice between using PostgreSQL enums and string types can significantly impact the flexibility and scalability of your application. While enums offer a way to define a fixed set of values, they can become cumbersome as your project evolves. As businesses grow and requirements change, the need to migrate from PostgreSQL enums to strings often arises. This transition, while seemingly straightforward, involves a nuanced understanding of both data types and the implications of such a shift. In this article, we will explore the reasons behind this migration, the challenges it presents, and the best practices to ensure a smooth transition.
As developers and database administrators grapple with the limitations of enums—such as their rigid structure and the difficulties associated with modifying them—many find that switching to string types can provide the flexibility they need. Strings allow for easier updates and additions to the set of possible values without requiring database schema changes. However, this migration is not merely a matter of changing data types; it involves careful planning and execution to maintain data integrity and application functionality.
In the following sections, we will delve into the key considerations for migrating from PostgreSQL enums to strings, including potential pitfalls and strategies for a successful transition. Whether you’re looking to enhance your application’s adaptability or simply streamline your database schema
Migrating Data Types
Migrating from PostgreSQL enums to strings involves several steps to ensure that your data integrity is maintained while transitioning to a more flexible data type. The first step is to identify all the tables and columns that currently use enum types. This is crucial as it will help you plan the migration process and ensure you cover all necessary areas.
Once you have identified the relevant columns, you need to create a new string column to temporarily hold the values. The following SQL command demonstrates how to add a new column to a table:
“`sql
ALTER TABLE your_table_name ADD COLUMN new_column_name VARCHAR;
“`
After creating the new column, you can populate it with the existing enum values. This is achieved with the `UPDATE` statement, which maps the enum values to the new string column:
“`sql
UPDATE your_table_name SET new_column_name = old_enum_column::text;
“`
Once the data has been migrated to the new column, you can safely drop the old enum column. However, before doing so, ensure that all applications and services interacting with the database are updated to use the new string column:
“`sql
ALTER TABLE your_table_name DROP COLUMN old_enum_column;
“`
Finally, you will want to rename the new column to match the original column name for consistency:
“`sql
ALTER TABLE your_table_name RENAME COLUMN new_column_name TO old_enum_column;
“`
Updating Application Logic
It’s essential to update any application logic that interacts with the database to handle the string values instead of enum values. This includes:
- Modifying queries that reference the old enum values to use strings.
- Updating data validation logic to ensure that only valid strings are accepted where enum values were previously used.
- Testing the application thoroughly to ensure that no functionality is broken due to this change.
Consider the following table to identify enum values and their corresponding string representations:
Old Enum Value | New String Value |
---|---|
enum_value_1 | string_value_1 |
enum_value_2 | string_value_2 |
enum_value_3 | string_value_3 |
This table serves as a reference for developers and database administrators to ensure that all instances of enum values are accurately replaced with the corresponding string values.
Testing the Migration
After completing the migration and updating the application logic, it is imperative to conduct thorough testing. The following approaches can be used to validate the migration:
- Unit Tests: Ensure that all new functionalities work as expected by writing unit tests for the updated codebase.
- Integration Tests: Validate that the application interacts properly with the database using the new string values.
- User Acceptance Testing (UAT): Gather feedback from end-users to ensure that the migration meets business requirements.
By following these steps, you can effectively migrate from PostgreSQL enums to string types, ensuring a smoother transition and improved flexibility for future database modifications.
Understanding Postgres Enum Types
PostgreSQL enum types are a user-defined data type that allows you to define a column with a specific set of allowed values. Enums are beneficial for maintaining data integrity but can complicate migrations and application logic.
- Advantages of Enums:
- Enforced value constraints, reducing invalid data entries.
- Improved readability of the database schema.
- Disadvantages of Enums:
- Difficult to alter once defined, requiring a more complex migration process.
- Limited flexibility when new values need to be added.
Reasons for Migration to String Types
Migrating from enum types to string types can enhance flexibility and ease of maintenance. The following factors may drive this decision:
- Application Compatibility: Many programming languages and frameworks handle strings more conveniently than enums.
- Dynamic Value Requirements: If the set of allowed values needs frequent updates, strings simplify this process.
- Database Portability: Moving to a more universally compatible data type can ease migrations between different database systems.
Migration Process Overview
The migration involves several steps to ensure a smooth transition from enum to string types. Here’s an outline of the process:
- Backup Your Database: Always create a backup before making schema changes.
- Create a New Column: Add a new string column to the table.
- Migrate Data: Copy data from the enum column to the new string column.
- Drop the Enum Column: Once verified, remove the old enum column.
- Rename the New Column: Rename the new string column to match the original column name.
Step-by-Step Migration Example
Consider a table named `orders` with an enum column `status` defined as follows:
“`sql
CREATE TYPE order_status AS ENUM (‘pending’, ‘shipped’, ‘delivered’);
CREATE TABLE orders (
id SERIAL PRIMARY KEY,
status order_status NOT NULL
);
“`
To migrate from `order_status` to a string representation, follow these steps:
- Backup the Orders Table:
“`sql
CREATE TABLE orders_backup AS SELECT * FROM orders;
“`
- Add a New String Column:
“`sql
ALTER TABLE orders ADD COLUMN status_string VARCHAR(20);
“`
- Migrate Data to the New Column:
“`sql
UPDATE orders SET status_string = status::text;
“`
- Drop the Enum Column:
“`sql
ALTER TABLE orders DROP COLUMN status;
“`
- Rename the New Column:
“`sql
ALTER TABLE orders RENAME COLUMN status_string TO status;
“`
Considerations During Migration
When migrating from enums to strings, keep the following points in mind:
- Data Integrity: Validate that all enum values are correctly converted to strings.
- Application Changes: Update any application logic that interacts with the status column to handle string values instead of enums.
- Testing: Conduct thorough testing to ensure that the migration does not introduce any issues in the application.
Post-Migration Cleanup
After migration, it is essential to conduct the following cleanup activities:
- Remove Unused Enum Types: If the enum type is no longer used, drop it from the database.
- Optimize the Database: Consider running `VACUUM` and `ANALYZE` to optimize the database performance post-migration.
By following these steps and considerations, the migration from PostgreSQL enum types to string types can be executed efficiently, minimizing disruptions and maximizing flexibility for future changes.
Expert Insights on Migrating from Postgres Enum to String
Dr. Emily Chen (Database Architect, Tech Innovations Inc.). “Migrating from Postgres Enum to String can simplify application logic and enhance flexibility. Enums can be rigid and require database changes for new values, while strings allow for dynamic updates without the need for schema alterations.”
James Patel (Senior Software Engineer, Cloud Solutions Corp.). “When transitioning from enums to strings, it is crucial to implement a robust validation layer in your application. This ensures that only acceptable string values are stored, maintaining data integrity and preventing issues down the line.”
Lisa Tran (Data Migration Specialist, DataWise Consulting). “A well-planned migration strategy is essential. It is advisable to conduct thorough testing and consider the impact on existing queries and indexes. Additionally, using a migration script can help automate the process and reduce human error.”
Frequently Asked Questions (FAQs)
What are the reasons to migrate from Postgres Enum to String?
Migrating from Postgres Enum to String can enhance flexibility, improve compatibility with various applications, and simplify data management. Strings allow for easier updates and modifications without needing to alter the database schema.
How can I identify columns using Enum types in my Postgres database?
You can identify Enum columns by querying the `pg_type` and `pg_enum` system catalogs. Use the following SQL command:
“`sql
SELECT a.attname, t.typname
FROM pg_attribute a
JOIN pg_type t ON a.atttypid = t.oid
WHERE t.typtype = ‘e’;
“`
What steps are involved in migrating Enum fields to String?
The migration process typically involves the following steps:
- Identify all Enum columns in your database.
- Create a new String column to hold the data.
- Update the new column with values from the Enum column.
- Drop the Enum column after ensuring data integrity.
- Rename the new String column to match the original Enum column name.
Are there any risks associated with migrating Enum to String?
Yes, potential risks include data loss if the migration is not handled properly, possible inconsistencies if the Enum values are not mapped correctly to strings, and application compatibility issues if the application expects Enum types.
How can I ensure data integrity during the migration?
To ensure data integrity, perform the migration in a transaction, validate the data after the migration, and consider creating backups before starting the process. Additionally, thoroughly test the application with the new String columns before finalizing the migration.
What tools or methods can assist in the migration process?
Various tools can assist in the migration process, including database migration frameworks like Liquibase or Flyway. Additionally, custom scripts can be written in SQL or a programming language like Python to automate the migration tasks.
Migrating from PostgreSQL enums to strings is a significant undertaking that can enhance flexibility and maintainability in database design. Enums, while useful for defining a set of allowed values, can introduce challenges when changes are required, such as adding or removing values. Transitioning to strings allows for greater adaptability, as new values can be added without the need for database migrations or schema changes, thus streamlining the development process.
During the migration process, it is crucial to carefully plan and execute the transition to avoid data loss or inconsistencies. This involves updating the database schema, modifying application code to handle string values instead of enums, and ensuring that existing data is converted accurately. Testing the migration thoroughly in a staging environment before deploying changes to production is essential to identify any potential issues early on.
Key takeaways from this discussion include the importance of considering future scalability when designing database schemas. While enums may seem convenient for enforcing data integrity, their rigidity can become a hindrance as application requirements evolve. Embracing string values can provide the necessary flexibility to accommodate changing business needs without extensive rework. Overall, a thoughtful migration strategy can lead to a more robust and adaptable database system.
Author Profile
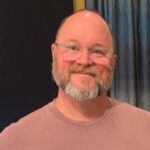
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?