Why Am I Seeing ‘Objects Are Not Valid As A React Child’ in My React App?
In the dynamic world of web development, React has emerged as a powerhouse for building user interfaces, allowing developers to create seamless, interactive experiences. However, even the most seasoned React developers can encounter perplexing errors that disrupt their workflow. One such common issue is the dreaded “Objects Are Not Valid As A React Child” error. This cryptic message can leave developers scratching their heads, unsure of what went wrong in their code. Understanding this error is crucial for anyone looking to master React and enhance their debugging skills.
At its core, the “Objects Are Not Valid As A React Child” error stems from a misunderstanding of how React handles rendering. When React attempts to render components, it expects certain data types, and when it encounters an unexpected object, it throws this error. This can happen in various scenarios, such as when trying to display complex data structures directly in the JSX or when passing props that are not formatted correctly. As developers dive deeper into the React ecosystem, recognizing the nuances of data types and their impact on rendering becomes essential.
Navigating this error requires a blend of understanding React’s rendering logic and a keen eye for data manipulation. By unraveling the common pitfalls that lead to this error, developers can not only troubleshoot their current projects more effectively but also build
Understanding the Error Message
The error message “Objects are not valid as a React child” typically occurs when a React component attempts to render an object directly. In React, the render method can only return primitives like strings or numbers, arrays, or valid React elements. When an object is inadvertently included in the render output, React cannot process it, resulting in this error.
Common scenarios that lead to this error include:
- Directly rendering an object: Attempting to use an object as a child in JSX.
- Incorrect data structures: Passing an object instead of a string or number where expected.
- Improperly formatted state or props: Using state or props that contain objects in a way that React cannot interpret.
Common Causes
Understanding the common causes of this error can help developers avoid it. Here are some frequent pitfalls:
- Rendering non-primitive values: For instance, trying to display a JavaScript object or an array directly in JSX.
- Improper data mapping: When mapping through arrays, if the callback returns an object instead of a valid element or string.
- State mismanagement: Using complex objects in state without proper handling can lead to this issue.
Examples of the Error
Here are a few examples that illustrate how this error might occur:
“`jsx
// Example 1: Directly rendering an object
const user = { name: “John”, age: 30 };
return
; // Error: Objects are not valid as a React child
// Example 2: Mapping over an array incorrectly
const items = [{ id: 1, name: “Item 1” }, { id: 2, name: “Item 2” }];
return (
-
{items.map(item => item)} // Error: Objects are not valid as a React child
);
“`
How to Fix the Error
To resolve the “Objects are not valid as a React child” error, you can follow these guidelines:
- Convert objects to strings: If you need to display an object, convert it to a string using `JSON.stringify()`.
- Access specific properties: Instead of rendering the entire object, access and render specific properties.
- Ensure proper mapping: When mapping over arrays, ensure the callback function returns a valid React element.
Here’s how you can implement these fixes:
“`jsx
// Fix for Example 1
return
; // Correctly displays the user object as a string
// Fix for Example 2
return (
-
{items.map(item =>
- {item.name}
)} // Correctly returns a list item
);
“`
Debugging Tips
When encountering this error, follow these debugging tips:
- Check render outputs: Print out the values being rendered to ensure they are valid types.
- Use PropTypes: Implement PropTypes in your components to validate the expected data types.
- Inspect component state: Use React Developer Tools to inspect component state and props to identify any unexpected objects.
Issue | Solution |
---|---|
Rendering an object | Use JSON.stringify() to convert to a string |
Improper array mapping | Ensure map returns valid React elements |
Invalid state or props | Validate data types and structure |
Understanding the Error
The error message “Objects are not valid as a React child” occurs when a React component attempts to render an object directly into the JSX. React can only render primitive types such as strings and numbers, as well as other React components.
Common scenarios that lead to this error include:
- Returning an object from a render method.
- Attempting to interpolate an object in JSX.
- Passing an object as a child to a React element.
Common Causes
This error typically arises from:
- Incorrect Data Structures: When the data being rendered is a complex object rather than a string or number.
- API Responses: When data fetched from an API is not properly processed or destructured before rendering.
- State Management: When state variables hold object references instead of renderable values.
Examples of the Error
Consider the following examples where this error might occur:
“`jsx
// Example of rendering an object directly
const user = { name: “John”, age: 30 };
return
; // Error: Objects are not valid as a React child
“`
“`jsx
// Example of incorrect JSX interpolation
const data = { title: “Hello”, content: “World” };
return
; // Error: Objects are not valid as a React child
“`
How to Fix the Error
To resolve the “Objects are not valid as a React child” error, consider the following strategies:
- Destructure Objects: Ensure you are extracting the values you want to render.
“`jsx
const user = { name: “John”, age: 30 };
return
; // Correct: Renders “John”
“`
- Use JSON.stringify: For debugging purposes, if you want to display the object itself.
“`jsx
const data = { title: “Hello”, content: “World” };
return
; // Renders: {“title”:”Hello”,”content”:”World”}
“`
– **Conditional Rendering**: Check if the data is an object before rendering.
“`jsx
const renderContent = (content) => {
if (typeof content === ‘object’) {
return
;
}
return
;
};
“`
Best Practices
To avoid encountering this error in the future, adhere to the following best practices:
- Data Validation: Ensure that the data being passed to components is in the expected format.
- Use PropTypes: Implement PropTypes for type-checking props, which can help catch potential issues early.
- Destructure Props: Always destructure props in your components to ensure you are using the correct data types.
Best Practice | Description |
---|---|
Data Validation | Verify data types before rendering. |
Use PropTypes | Utilize PropTypes to enforce prop types. |
Destructure Props | Extract necessary values from props explicitly. |
By following these practices and understanding the underlying causes of the error, developers can effectively prevent and troubleshoot instances of the “Objects are not valid as a React child” message in their applications.
Understanding the ‘Objects Are Not Valid As A React Child’ Error
Dr. Emily Chen (Senior Software Engineer, React Development Team). “The error ‘Objects Are Not Valid As A React Child’ typically occurs when a developer attempts to render a JavaScript object directly within a React component. This is a common mistake, especially for those new to React, as it requires a clear understanding of how JSX handles expressions.”
Michael Johnson (Lead Frontend Developer, CodeCraft Solutions). “To resolve this error, developers should ensure that they are passing primitive data types such as strings or numbers to JSX. If an object needs to be displayed, it should be converted to a string using methods like JSON.stringify() or by extracting specific properties that can be rendered.”
Sarah Patel (Technical Writer, React Insights). “Understanding the context of the error is crucial for debugging. Often, this issue arises when mapping over arrays of objects without properly accessing their properties. A solid grasp of JavaScript fundamentals is essential for effective React development.”
Frequently Asked Questions (FAQs)
What does the error “Objects Are Not Valid As A React Child” mean?
This error indicates that you are attempting to render an object directly in your React component, which is not valid. React can only render primitives like strings, numbers, or valid React elements.
How can I fix the “Objects Are Not Valid As A React Child” error?
To resolve this error, ensure that you are not passing an object directly to the JSX. Instead, convert the object to a string using `JSON.stringify()` or render its properties individually.
What types of data can be rendered as React children?
React children can be strings, numbers, arrays of valid React elements, or valid React elements themselves. Objects must be transformed into one of these types before rendering.
Can this error occur when using state or props in React?
Yes, this error can occur if state or props contain objects that are mistakenly rendered in JSX. Always verify the data type before rendering to avoid this issue.
Are there any common scenarios that lead to this error?
Common scenarios include attempting to render an object returned from an API directly, using an object in a map function without extracting its properties, or mistakenly passing an object as a child to a component.
How can I debug the “Objects Are Not Valid As A React Child” error?
To debug, check the component’s render method and inspect the data being passed as children. Use console logging to identify the type of the data and ensure it is a valid type for rendering.
The error message “Objects are not valid as a React child” typically arises in React applications when an attempt is made to render a JavaScript object directly within a component’s JSX. React components can only render certain types of data, including strings, numbers, arrays, and other React elements. When an object is mistakenly passed into the render method, React throws this error to indicate that it cannot process the object as a valid child element.
One common scenario leading to this error is when developers try to display complex data structures, such as objects returned from APIs, without properly extracting the necessary values. To resolve this issue, developers should ensure that they are rendering only primitive data types or React components. Utilizing methods like `JSON.stringify()` can help visualize objects for debugging purposes, but it is not a solution for rendering them in the UI.
Another important takeaway is the significance of validating the data being passed to components. Implementing PropTypes or TypeScript can help catch these errors during development, ensuring that the data types align with the expected structure. Additionally, error boundaries can be employed to gracefully handle such errors and improve the user experience by preventing the entire application from crashing due to a rendering issue.
Author Profile
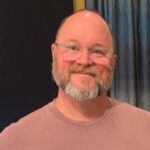
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?