How Can You Convert a String Date to a Date Object in Java?
In the world of programming, working with dates and times can often feel like navigating a labyrinth. One of the most common challenges developers face is converting string representations of dates into actual date objects that can be manipulated and formatted. In Java, this task is not only crucial for ensuring accurate data handling but also essential for creating applications that rely on time-sensitive information. Whether you’re developing a simple application or a complex enterprise system, mastering the art of date conversion will significantly enhance your coding prowess.
Java provides a robust set of tools for date and time manipulation, but understanding how to effectively convert string dates into date objects is a foundational skill every developer should possess. This process involves parsing the string format, which can vary widely, and transforming it into a standardized date object. With the of the `java.time` package in Java 8, the task has become more intuitive, allowing developers to leverage modern APIs that simplify date handling.
As we delve deeper into this topic, we will explore various methods for converting string dates to date objects, including the use of `SimpleDateFormat` and the newer `DateTimeFormatter`. We will also discuss common pitfalls and best practices to ensure that your date conversions are both accurate and efficient. By the end of this article, you will be equipped with
Understanding Date Formats
In Java, converting a string to a date requires an understanding of the date formats used in your string representation. The `SimpleDateFormat` class is typically employed to define the format of the date string. Common date formats include:
- `yyyy-MM-dd` for dates like 2023-10-25
- `MM/dd/yyyy` for dates like 10/25/2023
- `dd-MM-yyyy` for dates like 25-10-2023
It is essential to match the format specified in `SimpleDateFormat` with the actual format of the date string to avoid parsing exceptions.
Using SimpleDateFormat
To convert a string date into a `Date` object in Java, you can follow these steps:
- Create an instance of `SimpleDateFormat` with the desired date format.
- Use the `parse()` method to convert the string into a `Date` object.
Here is an example:
“`java
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateConversion {
public static void main(String[] args) {
String dateString = “2023-10-25”;
SimpleDateFormat formatter = new SimpleDateFormat(“yyyy-MM-dd”);
try {
Date date = formatter.parse(dateString);
System.out.println(“Converted Date: ” + date);
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
This code snippet demonstrates how to parse a string date formatted as `yyyy-MM-dd`.
Error Handling in Date Conversion
When converting string dates, it’s crucial to handle potential errors that may arise, such as:
- ParseException: This occurs when the string does not match the specified date format.
- NullPointerException: This can happen if you try to parse a null string.
Using try-catch blocks can help manage these exceptions effectively.
Example of Date Conversion with Error Handling
Here is a more robust example that includes error handling:
“`java
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Date;
public class SafeDateConversion {
public static void main(String[] args) {
String dateString = “10/25/2023”;
SimpleDateFormat formatter = new SimpleDateFormat(“MM/dd/yyyy”);
try {
Date date = formatter.parse(dateString);
System.out.println(“Converted Date: ” + date);
} catch (ParseException e) {
System.err.println(“Error parsing date: ” + e.getMessage());
}
}
}
“`
This example demonstrates a simple yet effective way to convert a date string while handling parsing errors.
Converting to LocalDate
With the of the `java.time` package in Java 8, you can also convert string dates to `LocalDate` using the `DateTimeFormatter` class. This provides a more modern approach to handling dates.
Example:
“`java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
public class LocalDateConversion {
public static void main(String[] args) {
String dateString = “2023-10-25”;
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(“yyyy-MM-dd”);
LocalDate localDate = LocalDate.parse(dateString, formatter);
System.out.println(“Converted LocalDate: ” + localDate);
}
}
“`
Comparison of Date Classes
The table below summarizes the differences between the older `Date` class and the newer `LocalDate` class:
Feature | Date | LocalDate |
---|---|---|
Package | java.util.Date | java.time.LocalDate |
Thread Safety | Not thread-safe | Immutable and thread-safe |
Time Zone Handling | Includes time zone | Represents a date without time zone |
Using `LocalDate` is generally recommended for date-only representations due to its immutability and thread safety.
Understanding Date Formats
When converting a string date to a Date object in Java, it is crucial to understand the format of the date string. Java uses the `SimpleDateFormat` class, which allows you to define the pattern of the date string.
Common date format patterns include:
Pattern | Description | Example |
---|---|---|
`yyyy` | Year (4 digits) | 2023 |
`MM` | Month (2 digits) | 01 (January) |
`dd` | Day of the month (2 digits) | 09 |
`HH` | Hour in 24-hour format (2 digits) | 14 |
`mm` | Minutes (2 digits) | 30 |
`ss` | Seconds (2 digits) | 45 |
Make sure to match the format in your string exactly with the pattern in your `SimpleDateFormat` instance.
Converting String to Date
To convert a string representation of a date into a `Date` object, you can follow these steps:
- Import necessary classes: You need to import `java.text.SimpleDateFormat` and `java.util.Date`.
- Create an instance of SimpleDateFormat: Define the format pattern that matches your date string.
- Parse the string: Use the `parse()` method to convert the string into a `Date` object.
Here’s a code example demonstrating these steps:
“`java
import java.text.SimpleDateFormat;
import java.util.Date;
public class DateConverter {
public static void main(String[] args) {
String dateString = “2023-01-09 14:30:45”; // Example date string
String format = “yyyy-MM-dd HH:mm:ss”; // Corresponding format
try {
SimpleDateFormat sdf = new SimpleDateFormat(format);
Date date = sdf.parse(dateString);
System.out.println(“Converted Date: ” + date);
} catch (Exception e) {
e.printStackTrace();
}
}
}
“`
Handling Exceptions
While parsing a date string, it is essential to handle potential exceptions that may arise, such as:
- ParseException: This occurs when the string does not match the expected format.
To properly handle exceptions, wrap your parsing code in a try-catch block, as shown in the above example. This ensures that your application does not crash due to improperly formatted input.
Using java.time Package
Since Java 8, the `java.time` package provides a more modern approach to date and time handling. You can use the `LocalDateTime` and `DateTimeFormatter` classes for this purpose.
Here’s how to perform the conversion using the newer API:
“`java
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
public class DateConverter {
public static void main(String[] args) {
String dateString = “2023-01-09 14:30:45”; // Example date string
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(“yyyy-MM-dd HH:mm:ss”);
LocalDateTime dateTime = LocalDateTime.parse(dateString, formatter);
System.out.println(“Converted LocalDateTime: ” + dateTime);
}
}
“`
This approach provides a more robust and thread-safe way to handle date and time conversions in Java. The `LocalDateTime` class represents date-time without a time zone, making it suitable for many applications.
Expert Insights on Converting String Dates to Date Objects in Java
Emily Carter (Senior Java Developer, Tech Innovations Inc.). “When converting string dates to date objects in Java, it is crucial to use the `SimpleDateFormat` class effectively. This class allows you to define the format of the date string, ensuring accurate parsing and conversion. Always handle potential exceptions, such as `ParseException`, to maintain robust code.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “Utilizing the `LocalDate` and `DateTimeFormatter` classes from the `java.time` package is highly recommended for modern Java applications. This approach not only simplifies the conversion process but also enhances code readability and maintainability. It is essential to match the date format string with the input string for successful conversion.”
Sarah Johnson (Java Programming Instructor, University of Technology). “In my experience teaching Java, I emphasize the importance of understanding the underlying date formats when converting strings. Developers should familiarize themselves with ISO 8601 formats, as they are widely used and can prevent common pitfalls associated with date parsing. Additionally, leveraging `DateTimeFormatter` provides a more type-safe and flexible way to handle date conversions.”
Frequently Asked Questions (FAQs)
How do I convert a string date to a date object in Java?
To convert a string date to a date object in Java, you can use the `SimpleDateFormat` class. Create an instance of `SimpleDateFormat` with the desired date format, then call the `parse()` method with the string date to obtain a `Date` object.
What format should I use with SimpleDateFormat?
The format you use with `SimpleDateFormat` should match the format of the string date. For example, if your string date is “2023-10-15”, you should use the format “yyyy-MM-dd”.
What exceptions should I handle when converting string dates?
When converting string dates, you should handle `ParseException`, which is thrown if the string cannot be parsed into a valid date. Additionally, consider handling `NullPointerException` if the input string is null.
Can I convert string dates in different time zones?
Yes, you can convert string dates in different time zones by using `SimpleDateFormat` along with `TimeZone`. Set the desired time zone using the `setTimeZone()` method before parsing the string date.
Is there an alternative to SimpleDateFormat for date conversion in Java?
Yes, starting from Java 8, you can use the `java.time` package, specifically `LocalDate`, `LocalDateTime`, or `ZonedDateTime` classes along with `DateTimeFormatter` for more modern and thread-safe date conversions.
What should I do if the string date format is inconsistent?
If the string date format is inconsistent, consider normalizing the input by using regular expressions to identify and convert various formats to a standard format before parsing with `SimpleDateFormat` or `DateTimeFormatter`.
Converting a string date to a date object in Java is a fundamental task that developers often encounter. The process typically involves using the `SimpleDateFormat` class or the `DateTimeFormatter` class introduced in Java 8. Both classes provide robust methods to parse string representations of dates into actual date objects, allowing for further manipulation and formatting as required by the application.
When using `SimpleDateFormat`, it is essential to define the pattern that matches the string date format. This ensures accurate parsing. For example, if the string date is in the format “dd-MM-yyyy”, the corresponding pattern must be specified. Similarly, `DateTimeFormatter` offers a more modern and thread-safe approach for date-time manipulation, allowing developers to utilize the `LocalDate`, `LocalDateTime`, and `ZonedDateTime` classes for more complex date operations.
Key takeaways include the importance of understanding the format of the input string and the corresponding pattern needed for conversion. Additionally, developers should be cautious of potential `ParseException` when using `SimpleDateFormat` and consider using `DateTimeFormatter` for new developments to leverage its advantages in terms of immutability and thread safety. Overall, mastering these techniques is crucial for effective
Author Profile
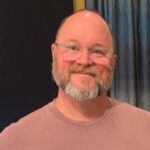
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?