How to Fix the ‘ModuleNotFoundError: No Module Named ‘Google.Colab” in Your Python Environment?
In the ever-evolving landscape of data science and machine learning, Google Colab has emerged as a powerful tool, enabling users to write and execute Python code in a collaborative, cloud-based environment. However, as with any software, users occasionally encounter hurdles that can disrupt their workflow. One such challenge is the dreaded `ModuleNotFoundError: No Module Named ‘Google.Colab’`. This error can be a source of frustration, particularly for those who rely on Colab for their projects. Understanding the root causes of this issue is essential for ensuring a smooth coding experience and maximizing the potential of this versatile platform.
As users delve into the world of Google Colab, they often take for granted the seamless integration of libraries and modules that enhance their coding capabilities. However, when the `ModuleNotFoundError` strikes, it serves as a stark reminder of the intricacies of Python’s package management system. This error can arise from various factors, including incorrect imports, missing libraries, or even issues related to the environment setup. By gaining insight into these underlying causes, users can not only resolve the immediate problem but also fortify their understanding of how to effectively manage dependencies in their coding projects.
Navigating the complexities of Google Colab and its associated modules can be daunting,
Understanding the Error
The error message `ModuleNotFoundError: No module named ‘google.colab’` typically arises when a script or notebook is being executed outside of the Google Colab environment. Google Colab is a cloud-based platform that allows users to write and execute Python code in a Jupyter notebook format. It includes specific libraries and modules that are pre-installed and accessible only within that environment.
Reasons for encountering this error include:
- Attempting to run Google Colab-specific code locally on your machine.
- Missing the import statement for the necessary libraries.
- The environment not being properly set up to recognize the Google Colab libraries.
Common Scenarios Leading to the Error
Several common scenarios can lead to encountering this specific error:
- Local Jupyter Notebook: If you attempt to run a notebook that includes `import google.colab`, you will receive this error unless you are in the Google Colab environment.
- Python Scripts: Running scripts that utilize Google Colab functionalities (such as file uploads or specific Colab tools) outside of Colab will trigger this error.
- Code Sharing: Sharing Colab notebooks with others who try to run them in different environments without modification may lead to confusion and errors.
Solutions to Resolve the Error
To address the `ModuleNotFoundError: No module named ‘google.colab’`, consider the following solutions:
- Run in Google Colab: Ensure that you are executing your code within the Google Colab platform. You can access it by navigating to [Google Colab](https://colab.research.google.com).
- Modify the Code: If running locally, you can modify the code to remove or replace Google Colab-specific imports or functionalities with alternatives that work in local environments.
- Use Environment Conditionals: Implement checks in your code to determine if it is running in Google Colab or another environment and import libraries accordingly. For example:
“`python
try:
import google.colab
IN_COLAB = True
except ImportError:
IN_COLAB =
“`
- Utilize Virtual Environments: If you still need to work with similar libraries, you can set up a virtual environment and install packages that mimic some functionalities of Google Colab.
Alternative Libraries and Tools
If you are looking to replicate some of the functionalities of Google Colab in a local environment, consider using the following alternatives:
Functionality | Google Colab | Local Environment Alternatives |
---|---|---|
Jupyter Notebooks | Yes | Yes |
GPU Support | Yes | Local setup with CUDA (NVIDIA GPU) |
File Uploads | `files.upload()` | Use Python’s `tkinter` or `flask` for GUI |
Collaboration | Real-time collaboration | GitHub or other version control systems |
By understanding the context in which the error occurs and applying the appropriate fixes, users can effectively navigate the challenges posed by the `ModuleNotFoundError` when working with Google Colab-specific code.
Common Causes of `ModuleNotFoundError`
The `ModuleNotFoundError: No module named ‘google.colab’` typically arises from several key issues. Understanding these can help in effectively troubleshooting the error.
- Incorrect Environment: The Google Colab module is specifically designed to run in the Google Colab environment. If you are running your code locally or in a different IDE, this error will occur.
- Typographical Errors: Any mistakes in the module name, such as incorrect casing or misspellings, will lead to this error.
- Virtual Environments: If you are using a virtual environment that does not have access to the Google Colab library, the module will not be found.
- Library Not Installed: Although Google Colab comes pre-installed with the necessary libraries, running code outside of Colab requires manual installation.
Troubleshooting Steps
To resolve the `ModuleNotFoundError`, you can follow these steps:
- Check Your Environment: Ensure you are executing your code in Google Colab. If you are on a local machine, consider switching to Colab.
- Verify Module Name: Double-check the spelling and case of the module name. It should be written as `google.colab`.
- Use Virtual Environments Correctly: If you are using a virtual environment, make sure it is activated and includes all the necessary dependencies.
- Install Required Packages: If you are running a local setup and need to use specific Google libraries, install them using pip:
“`bash
pip install google-colab
“`
Alternatives to Google Colab
If you find yourself unable to use Google Colab for certain functionalities, consider these alternatives:
Alternative | Description |
---|---|
Jupyter Notebook | Allows for local execution and customization. |
Kaggle Kernels | Provides a cloud-based environment similar to Colab. |
Microsoft Azure Notebooks | Offers cloud-based Jupyter notebooks with additional integrations. |
Using Google Colab Features Effectively
To maximize your experience with Google Colab, utilize the following features:
- GPU/TPU Support: Enable hardware acceleration via Runtime settings for faster computations.
- Easy Sharing: Share your notebooks easily through Google Drive or via links.
- Integration with Google Drive: Mount your Google Drive to access datasets and save your work seamlessly.
Best Practices for Code Compatibility
To ensure your code runs smoothly across different environments, adhere to these best practices:
- Environment Checks: Implement checks to determine if the code is running in Google Colab.
“`python
try:
import google.colab
IN_COLAB = True
except ImportError:
IN_COLAB =
“`
- Conditional Imports: Use conditional statements to import modules based on the environment.
“`python
if IN_COLAB:
from google.colab import drive
else:
import os
“`
- Documentation: Always document your code to clarify dependencies and environment requirements for future reference.
Understanding the ‘ModuleNotFoundError’ in Google Colab
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “The ‘ModuleNotFoundError: No Module Named ‘Google.Colab” typically arises when users attempt to run code that is not compatible with the Google Colab environment. It’s crucial to ensure that the correct libraries are being referenced and that they are installed within the Colab environment.”
Michael Chen (Software Engineer, Cloud Solutions Group). “This error can often be resolved by checking the spelling of the module name or confirming that the module is indeed available in the Colab environment. Users should also consider using the appropriate import statement that aligns with the library’s documentation.”
Sarah Thompson (Educational Technology Specialist, Learning Tech Advisors). “When encountering this error in Google Colab, it’s essential to remember that some modules may not be pre-installed. Users should leverage the ‘pip install’ command to install any missing libraries directly within their notebook.”
Frequently Asked Questions (FAQs)
What does the error “ModuleNotFoundError: No Module Named ‘Google.Colab'” mean?
This error indicates that the Python interpreter cannot find the specified module ‘Google.Colab’, suggesting that it is either not installed or not accessible in the current environment.
How can I resolve the “ModuleNotFoundError: No Module Named ‘Google.Colab'” error?
To resolve this error, ensure you are running your code in Google Colab. If you are using a local environment, install the required libraries or switch to Google Colab where the module is pre-installed.
Is ‘Google.Colab’ a standard Python library?
No, ‘Google.Colab’ is not a standard Python library. It is a specific module provided by Google Colab for interacting with its features and functionalities.
Can I use Google Colab libraries in a local Jupyter Notebook?
While you cannot directly use Google Colab libraries in a local Jupyter Notebook, you can replicate some functionalities by installing equivalent libraries or using Google Colab’s features through APIs.
What are the common use cases for ‘Google.Colab’?
Common use cases include running machine learning models, data analysis, and sharing code with others, leveraging the cloud-based resources and GPU support provided by Google Colab.
Are there any alternatives to Google Colab for running similar code?
Yes, alternatives include Jupyter Notebook, Kaggle Kernels, and Microsoft Azure Notebooks, which offer similar functionalities for running Python code in a cloud-based environment.
The error message “ModuleNotFoundError: No module named ‘google.colab'” typically arises when a user attempts to execute code that relies on Google Colab-specific libraries outside of the Google Colab environment. This issue often occurs when users try to run scripts in local Python environments, such as Jupyter Notebook or standard Python interpreters, where the Google Colab modules are not available. Understanding the context in which this error occurs is crucial for troubleshooting and ensuring that the necessary libraries are correctly installed and accessible.
One key takeaway is that Google Colab is a cloud-based platform specifically designed for running Python code with integrated support for machine learning and data analysis. It provides a unique environment with pre-installed libraries and tools tailored for these tasks. Therefore, users should ensure they are working within the Google Colab environment when attempting to utilize its specific modules. If users need similar functionality outside of Colab, they must install equivalent libraries locally or adapt their code to work with available alternatives.
Another important insight is the significance of understanding the dependencies of the code being executed. Users should be aware of the libraries and modules their scripts depend on and ensure that these are installed in their local environment if they choose not to use Google Colab. This proactive approach
Author Profile
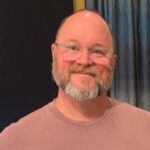
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?