How Can You Effectively Save Your Python Code and Projects?
How To Save Python: A Guide to Preserving Your Code and Environment
In the ever-evolving landscape of programming, Python stands out as a versatile and powerful language beloved by developers worldwide. Whether you’re a seasoned programmer or just starting your coding journey, knowing how to save your Python projects effectively is crucial. Not only does it ensure that your hard work is safeguarded against unforeseen mishaps, but it also streamlines your workflow, allowing you to focus on creating innovative solutions rather than worrying about data loss. In this article, we will explore essential strategies and best practices for saving your Python code, managing your development environment, and maintaining version control.
As we delve into the intricacies of saving Python, we’ll touch on various methods that cater to different aspects of your coding experience. From utilizing built-in functions to leverage the power of version control systems like Git, you’ll discover how to implement these techniques to enhance your productivity. Additionally, we’ll discuss the importance of creating backups and using virtual environments to keep your projects organized and secure. By the end of this guide, you’ll have a comprehensive understanding of how to protect your Python code and ensure its longevity in an ever-changing digital world.
Using Virtual Environments
Creating and using virtual environments is a crucial practice when working with Python. Virtual environments allow you to manage project-specific dependencies without affecting the global Python installation. This is particularly useful when different projects require different versions of libraries.
To create a virtual environment, you can use the built-in `venv` module. Here’s how to do it:
- Open a terminal or command prompt.
- Navigate to your project directory.
- Run the following command:
“`bash
python -m venv env_name
“`
Replace `env_name` with your preferred name for the virtual environment.
To activate the virtual environment, use the following command:
- On Windows:
“`bash
.\env_name\Scripts\activate
“`
- On macOS and Linux:
“`bash
source env_name/bin/activate
“`
Once activated, any packages you install using `pip` will only be available within this environment.
Managing Dependencies
Managing project dependencies efficiently is vital for maintaining a clean and functional Python environment. You can use the `requirements.txt` file to list all required packages for your project. This file can be generated by running:
“`bash
pip freeze > requirements.txt
“`
To install dependencies from this file in a new environment, use:
“`bash
pip install -r requirements.txt
“`
Consider the following best practices for dependency management:
- Always use a virtual environment for each project.
- Regularly update your dependencies to mitigate security vulnerabilities.
- Utilize tools like `pipenv` or `poetry` for advanced dependency management.
Version Control with Git
Integrating version control into your Python projects enhances collaboration and code management. Git is the most widely used version control system, and it allows you to track changes, revert to previous states, and collaborate with others.
To set up Git for your Python project, follow these steps:
- Initialize a Git repository:
“`bash
git init
“`
- Create a `.gitignore` file to exclude unnecessary files and directories (like `env_name/`, `__pycache__/`, etc.).
- Stage your changes:
“`bash
git add .
“`
- Commit your changes:
“`bash
git commit -m “Initial commit”
“`
By following these steps, you ensure that your Python project is versioned and that collaborators can easily contribute.
Table of Useful Python Commands
Command | Description |
---|---|
python -m venv env_name | Create a new virtual environment |
source env_name/bin/activate | Activate the virtual environment (Linux/Mac) |
.\env_name\Scripts\activate | Activate the virtual environment (Windows) |
pip freeze > requirements.txt | Export current dependencies to a file |
pip install -r requirements.txt | Install dependencies from requirements file |
git init | Initialize a new Git repository |
git add . | Stage all changes for commit |
git commit -m “message” | Commit staged changes with a message |
By adhering to these practices and utilizing the provided commands, you can effectively manage your Python projects, ensuring a clean and organized development process.
Understanding Python Saving Mechanisms
Saving Python data can be achieved through various methods, depending on the type of data and the intended use. The primary methods include saving to files, databases, and using serialization formats.
File-Based Saving Techniques
Python supports numerous file formats for saving data. Common file types include:
- Text Files: Use for simple data storage.
- CSV Files: Ideal for tabular data, easily opened in spreadsheets.
- JSON Files: Suitable for structured data, especially in web applications.
- Binary Files: Efficient storage for complex data structures.
Saving Data to Text and CSV Files
To save data in text or CSV formats, utilize Python’s built-in functions.
Example of saving to a text file:
“`python
with open(‘data.txt’, ‘w’) as file:
file.write(“Hello, World!\n”)
“`
Example of saving to a CSV file:
“`python
import csv
data = [[“Name”, “Age”], [“Alice”, 30], [“Bob”, 25]]
with open(‘data.csv’, ‘w’, newline=”) as file:
writer = csv.writer(file)
writer.writerows(data)
“`
Using JSON for Structured Data
JSON is a versatile format for saving data structures. Python’s `json` module can easily serialize and deserialize data.
Example of saving data as JSON:
“`python
import json
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.json’, ‘w’) as file:
json.dump(data, file)
“`
Database Storage Options
For large datasets or when data integrity is crucial, consider using a database. Popular choices include:
- SQLite: A lightweight, serverless database embedded in Python.
- PostgreSQL: An advanced, open-source relational database.
- MongoDB: A NoSQL database, ideal for unstructured data.
Example of saving data to SQLite:
“`python
import sqlite3
connection = sqlite3.connect(‘example.db’)
cursor = connection.cursor()
cursor.execute(‘CREATE TABLE IF NOT EXISTS users (name TEXT, age INTEGER)’)
cursor.execute(‘INSERT INTO users (name, age) VALUES (?, ?)’, (‘Alice’, 30))
connection.commit()
connection.close()
“`
Serialization with Pickle
Python’s `pickle` module allows you to serialize and deserialize Python objects efficiently. This method is particularly useful for saving complex data types.
Example of using Pickle:
“`python
import pickle
data = {‘name’: ‘Alice’, ‘age’: 30}
with open(‘data.pkl’, ‘wb’) as file:
pickle.dump(data, file)
with open(‘data.pkl’, ‘rb’) as file:
loaded_data = pickle.load(file)
“`
Best Practices for Saving Data
When saving data, adhere to the following best practices:
- Choose the Right Format: Select a file format that suits your data structure and accessibility needs.
- Use Context Managers: Always utilize `with` statements for file operations to ensure files are properly closed.
- Error Handling: Implement try-except blocks to handle potential errors during file I/O operations.
- Data Validation: Validate data before saving to prevent corruption or loss.
Conclusion and Recommendations
Each method of saving Python data serves different purposes, and the choice should align with the specific requirements of your application. For lightweight applications, file-based saving is often sufficient, while larger, more complex applications benefit from database integration or serialization through Pickle.
Strategies for Efficient Python Resource Management
Dr. Emily Tran (Senior Software Engineer, Tech Innovations Inc.). “To save Python effectively, developers should focus on optimizing their code by utilizing built-in functions and libraries. This not only enhances performance but also reduces memory consumption, ensuring that applications run smoothly even under heavy loads.”
Michael Chen (Data Scientist, Analytics Hub). “Implementing efficient data structures is crucial in Python. By using generators instead of lists for large datasets, one can significantly reduce memory usage, leading to a more efficient processing of data without compromising on speed.”
Sarah Patel (Python Developer Advocate, CodeCraft Academy). “Regularly profiling and monitoring your Python applications can reveal bottlenecks and areas for improvement. Tools like cProfile and memory_profiler are invaluable for identifying inefficient code segments that can be optimized to save both time and resources.”
Frequently Asked Questions (FAQs)
How do I save a Python script?
To save a Python script, open your preferred text editor or Integrated Development Environment (IDE), write your code, and then select “File” followed by “Save As.” Choose a location, enter a filename with a `.py` extension, and click “Save.”
What is the best way to save data in Python?
The best way to save data in Python depends on the data type. For structured data, consider using the `pickle` module for serialization, or the `json` module for JSON format. For databases, use libraries like `sqlite3` or `SQLAlchemy` for relational data storage.
Can I save Python variables to a file?
Yes, you can save Python variables to a file using the `pickle` module, which serializes Python objects. Alternatively, you can write variables to a text or CSV file using the `open()` function and appropriate file handling methods.
How can I save output from a Python program to a text file?
To save output from a Python program to a text file, use the `open()` function in write mode. Redirect output using `file.write()` or by using `with open(‘filename.txt’, ‘w’) as file:` to ensure proper file handling.
Is there a way to save a Python environment?
Yes, you can save a Python environment by creating a requirements file using `pip freeze > requirements.txt`. This file lists all installed packages and their versions, allowing you to replicate the environment using `pip install -r requirements.txt`.
How do I save plots created with Matplotlib in Python?
To save plots created with Matplotlib, use the `savefig()` function. Specify the filename and format (e.g., PNG, PDF) by calling `plt.savefig(‘plot.png’)` before `plt.show()`. This ensures the plot is saved to the specified file.
In summary, saving a Python program involves understanding various methods to ensure that your code is stored securely and can be easily accessed for future use. The most common way to save a Python script is by using a text editor or an Integrated Development Environment (IDE) to write the code and then saving it with a .py file extension. This allows Python to recognize the file as a script that can be executed in the Python interpreter.
Additionally, it is essential to utilize version control systems like Git to manage changes and collaborate effectively with others. By committing changes regularly, developers can track the evolution of their code, revert to previous versions if necessary, and maintain a history of their work. This practice not only enhances code management but also fosters better collaboration within teams.
Another important aspect of saving Python code is ensuring that dependencies and environment configurations are properly documented. Using tools such as virtual environments and requirements.txt files can help in preserving the necessary libraries and packages needed for the script to run successfully. This practice is crucial for maintaining the integrity of the code when sharing it with others or deploying it in different environments.
saving Python effectively requires a combination of proper file management, version control, and environment configuration. By adopting these
Author Profile
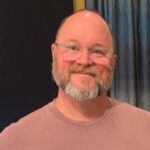
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?