How Can You Effectively Repeat Python Code for Better Efficiency?
In the world of programming, efficiency is key, and one of the most powerful tools at your disposal is the ability to repeat code effortlessly. Whether you’re automating a task, processing data, or creating interactive applications, knowing how to repeat Python code can save you time and effort while enhancing your productivity. This article will guide you through the various methods available in Python to execute code multiple times, empowering you to streamline your coding process and tackle complex problems with ease.
When it comes to repeating code in Python, there are several fundamental techniques that every programmer should master. From simple loops to more advanced constructs, Python offers a variety of ways to execute a block of code multiple times. Understanding these methods not only helps in writing cleaner and more efficient code but also fosters a deeper comprehension of programming logic and flow control.
In this exploration, we will delve into the different approaches to repeating code, including the use of loops, functions, and even recursion. Each method has its unique advantages and applications, making it essential to choose the right one for your specific needs. As we embark on this journey, you’ll discover how these techniques can be applied in real-world scenarios, ultimately enhancing your coding skills and confidence in Python programming.
Using Loops to Repeat Code
Loops are fundamental in Python for executing a block of code multiple times. The two primary types of loops in Python are `for` loops and `while` loops.
A `for` loop iterates over a sequence (like a list or a string) and executes the code block for each item in that sequence. The syntax is as follows:
“`python
for item in sequence:
Code block to execute
“`
A `while` loop continues to execute as long as a specified condition is true. Its syntax is:
“`python
while condition:
Code block to execute
“`
Key Differences Between For and While Loops
Feature | For Loop | While Loop |
---|---|---|
Control | Iterates over a sequence | Continues while condition is true |
Use Case | Best for known iterations | Best for unknown iterations |
Initialization | Automatically handled | Must be initialized manually |
Defining Functions for Code Reusability
Functions are a powerful way to encapsulate code for reuse. By defining a function, you can call it multiple times without rewriting the code. The basic syntax for defining a function is:
“`python
def function_name(parameters):
Code block
return value
“`
When you want to repeat a task, simply call the function:
“`python
function_name(arguments)
“`
Example of a Function
“`python
def greet(name):
print(f”Hello, {name}!”)
greet(“Alice”)
greet(“Bob”)
“`
In this example, the `greet` function can be called multiple times with different names.
Utilizing Recursion
Recursion is another method of repeating code, where a function calls itself to solve smaller instances of the same problem. While powerful, it should be used with caution due to potential stack overflow issues.
Here is a simple example of a recursive function calculating factorial:
“`python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n – 1)
“`
To compute the factorial of 5:
“`python
result = factorial(5) Output will be 120
“`
Advantages and Disadvantages of Recursion
Advantages | Disadvantages |
---|---|
Simplifies code for complex tasks | High memory usage |
Elegant solution for problems | Risk of stack overflow |
Using List Comprehensions for Repetition
List comprehensions provide a concise way to create lists by repeating a code pattern. This method is both efficient and readable. The syntax is:
“`python
[expression for item in iterable]
“`
Example of List Comprehension
“`python
squares = [x**2 for x in range(10)]
“`
This code will generate a list of squares from 0 to 9.
Integrating the Time Module for Delays
Sometimes, you may need to repeat code with a delay. The `time` module in Python allows you to pause the execution. For instance, to repeat a task every few seconds:
“`python
import time
for i in range(5):
print(“This will repeat every 2 seconds.”)
time.sleep(2)
“`
This will print the message five times, with a two-second interval between each print.
By utilizing these various approaches, Python programmers can effectively manage repetition in their code, enhancing both functionality and maintainability.
Using Loops to Repeat Code
Loops are fundamental constructs in Python that allow you to execute a block of code multiple times. The primary types of loops are `for` loops and `while` loops.
For Loops
A `for` loop iterates over a sequence (such as a list, tuple, string, or range). The syntax is straightforward:
“`python
for item in iterable:
Code to execute
“`
Example:
“`python
for i in range(5):
print(“This is repetition number:”, i)
“`
This code will print the message five times, with the index printed each time.
While Loops
A `while` loop continues to execute as long as a specified condition is true. The syntax is as follows:
“`python
while condition:
Code to execute
“`
Example:
“`python
count = 0
while count < 5:
print("This is repetition number:", count)
count += 1
```
This example will also print the message five times, incrementing the `count` variable each iteration.
Defining Functions for Repeated Code
Functions encapsulate code into reusable blocks, allowing you to invoke the same code multiple times with different parameters.
Function Definition
To define a function, use the `def` keyword followed by the function name and parentheses.
“`python
def function_name(parameters):
Code to execute
“`
Example:
“`python
def repeat_message(message, times):
for i in range(times):
print(message)
repeat_message(“Hello, World!”, 3)
“`
This function prints “Hello, World!” three times, demonstrating how functions can simplify repetitive tasks.
Using List Comprehensions for Concise Repetition
List comprehensions provide a concise way to create lists based on existing lists. They can also be used to perform repeated actions in a compact format.
Syntax:
“`python
[expression for item in iterable]
“`
Example:
“`python
messages = [“Repeat this message” for _ in range(4)]
for msg in messages:
print(msg)
“`
This will print “Repeat this message” four times by creating a list of messages first.
Utilizing the `time` Module for Delayed Repetition
When repeated actions require timing control, the `time` module can be beneficial. The `sleep` function introduces a delay between repetitions.
Example:
“`python
import time
for i in range(3):
print(“This will repeat with a delay.”)
time.sleep(2) Wait for 2 seconds
“`
This code will print the message three times, with a two-second delay between each print.
Recursion as a Method of Code Repetition
Recursion is a programming technique where a function calls itself to solve smaller instances of a problem. Although not a traditional loop, it effectively repeats code.
**Example:**
“`python
def recursive_repeat(message, times):
if times > 0:
print(message)
recursive_repeat(message, times – 1)
recursive_repeat(“This is a recursive repetition.”, 3)
“`
This function will print the message three times recursively.
Using the `itertools` Module for Advanced Repetition
The `itertools` module provides powerful tools for creating iterators for efficient looping. The `cycle` function can be used for infinite repetition of a sequence.
Example:
“`python
import itertools
for message in itertools.cycle([“Repeat this”, “Again”, “One more time”]):
print(message)
Use break or another condition to stop the loop
“`
This code will repeat the messages indefinitely until a break condition is introduced.
Strategies for Efficiently Repeating Python Code
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “When considering how to repeat Python code efficiently, using functions is paramount. Functions encapsulate reusable code, allowing for cleaner and more maintainable scripts. Additionally, leveraging loops such as ‘for’ and ‘while’ can automate repetitive tasks effectively.”
Mark Thompson (Lead Software Engineer, CodeMaster Solutions). “In Python, the ‘timeit’ module is an invaluable tool for measuring the execution time of code snippets. This can help developers identify which methods of repetition—whether through loops or recursion—are the most efficient for their specific use cases.”
Linda Garcia (Python Instructor, Online Coding Academy). “Understanding the principles of DRY (Don’t Repeat Yourself) is crucial when repeating code in Python. By utilizing decorators and higher-order functions, developers can enhance their code’s reusability and reduce redundancy, which ultimately leads to better performance.”
Frequently Asked Questions (FAQs)
How can I repeat a block of code in Python?
You can repeat a block of code in Python using loops. The most common types are `for` loops and `while` loops. A `for` loop iterates over a sequence, while a `while` loop continues until a specified condition is .
What is the syntax for a for loop in Python?
The syntax for a `for` loop in Python is as follows:
“`python
for variable in iterable:
code to execute
“`
This structure allows you to execute the indented code block for each item in the iterable.
How do I use a while loop to repeat code in Python?
To use a `while` loop, the syntax is:
“`python
while condition:
code to execute
“`
The code block will repeat as long as the condition evaluates to true.
Can I repeat code a specific number of times in Python?
Yes, you can repeat code a specific number of times using the `range()` function within a `for` loop. For example:
“`python
for i in range(n): where n is the number of repetitions
code to execute
“`
What is the difference between a for loop and a while loop?
A `for` loop is typically used when the number of iterations is known beforehand, such as iterating over a list. A `while` loop is used when the number of iterations is not predetermined and depends on a condition being met.
How can I repeat code indefinitely in Python?
To repeat code indefinitely, you can use a `while True:` loop. This will continue executing the code block until a break condition is met or an exception occurs. For example:
“`python
while True:
code to execute
if some_condition:
break
“`
In summary, repeating Python code can be achieved through various methods, each suited to different scenarios and coding styles. The most common techniques include using loops, such as `for` and `while` loops, which allow for the execution of a block of code multiple times based on specified conditions. Functions are another powerful way to encapsulate code that can be reused throughout a program, providing a clean and organized approach to repetition.
Additionally, list comprehensions and generator expressions offer concise alternatives for repeating operations over iterable data structures, promoting both efficiency and readability. The use of recursion is also a noteworthy method for repeating tasks, although it requires careful management of base cases to avoid infinite loops. Understanding these various techniques enables developers to select the most appropriate method for their specific coding needs.
Key takeaways include the importance of choosing the right repetition method based on the context and requirements of the task at hand. Utilizing loops and functions enhances code modularity and maintainability, while comprehensions can streamline operations on collections. Ultimately, mastering these techniques not only improves coding efficiency but also contributes to writing cleaner and more effective Python code.
Author Profile
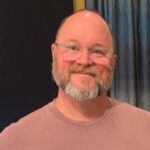
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?