How Can You Call a Function Stored in a Dictionary in Python?
In the dynamic world of Python programming, dictionaries serve as one of the most versatile and powerful data structures. They allow developers to store and manage data in key-value pairs, making data retrieval efficient and intuitive. But did you know that you can also store functions within these dictionaries? This capability opens up a realm of possibilities, enabling you to create more organized, modular, and dynamic code. If you’ve ever wondered how to call a function stored in a dictionary, you’re in the right place!
In this article, we’ll explore the fascinating intersection of dictionaries and functions in Python. We’ll discuss how to define functions, store them in a dictionary, and invoke them seamlessly. This technique not only enhances code readability but also allows for the implementation of more complex logic without cluttering your codebase. Whether you’re a beginner looking to expand your Python toolkit or an experienced developer seeking to refine your skills, understanding how to leverage functions within dictionaries can significantly elevate your programming prowess.
Join us as we delve into practical examples and best practices that will empower you to harness the full potential of this powerful combination. By the end of this article, you’ll be equipped with the knowledge to effectively call functions stored in dictionaries, paving the way for more efficient and elegant code.
Accessing Functions in a Dictionary
In Python, dictionaries are versatile data structures that can store key-value pairs. When using dictionaries to store functions, you can call these functions dynamically by referencing their keys. This allows for a clean and modular approach to function management. Here’s how to effectively access and call functions stored in a dictionary.
To call a function in a dictionary, use the following syntax:
“`python
function_dict[key]()
“`
Where `function_dict` is your dictionary containing functions, and `key` is the specific key for the function you want to call.
Example of Function Storage in a Dictionary
Consider the following example where we define a few simple functions and store them in a dictionary:
“`python
def greet():
return “Hello!”
def farewell():
return “Goodbye!”
function_dict = {
‘greet’: greet,
‘farewell’: farewell
}
“`
To call the `greet` function, you would use:
“`python
print(function_dict[‘greet’]()) Output: Hello!
“`
Similarly, calling the `farewell` function:
“`python
print(function_dict[‘farewell’]()) Output: Goodbye!
“`
Dynamic Function Calling
Using a dictionary to call functions dynamically can be particularly useful in scenarios where the function to be called is determined at runtime. For instance, you might take user input or process data that dictates which function to execute.
“`python
action = ‘greet’ This could be user input
if action in function_dict:
print(function_dict[action]()) Calls the greet function
else:
print(“Invalid action”)
“`
Advantages of Using Functions in Dictionaries
Utilizing dictionaries to manage functions provides several advantages:
- Modularity: Functions can be added or removed easily without changing the overall structure of the code.
- Dynamic Behavior: Functions can be called based on runtime conditions, improving flexibility.
- Readability: Organizing functions within a dictionary can enhance code readability and maintenance.
Table of Example Functions
Here’s a simple table summarizing the example functions used:
Function Name | Description |
---|---|
greet | Returns a greeting message. |
farewell | Returns a farewell message. |
Handling Arguments in Functions
If your functions require arguments, you can still store and call them from a dictionary. Ensure to pass the required arguments when calling the function.
“`python
def add(a, b):
return a + b
function_dict = {
‘add’: add
}
result = function_dict[‘add’](5, 3) Output: 8
print(result)
“`
In this example, the `add` function takes two parameters, demonstrating how to handle functions with arguments within a dictionary structure.
By using dictionaries to manage functions, Python developers can create more dynamic and organized code, enhancing both functionality and maintainability.
Understanding Functions in a Dictionary
In Python, dictionaries can store functions as values. This allows for flexible and dynamic execution of functions based on keys. Here’s how to effectively call a function stored in a dictionary.
Defining Functions and Creating a Dictionary
First, define some functions that you want to store in a dictionary. Then, create a dictionary where these functions are the values associated with specific keys.
“`python
def greet():
return “Hello, World!”
def farewell():
return “Goodbye, World!”
Creating a dictionary with functions
function_dict = {
‘greet’: greet,
‘farewell’: farewell
}
“`
Calling a Function from a Dictionary
To call a function stored in a dictionary, access it using its key and then invoke it using parentheses. Here’s how to do it:
“`python
Calling the ‘greet’ function
result_greet = function_dict[‘greet’]()
print(result_greet) Output: Hello, World!
Calling the ‘farewell’ function
result_farewell = function_dict[‘farewell’]()
print(result_farewell) Output: Goodbye, World!
“`
Passing Arguments to Functions in a Dictionary
If your functions require parameters, you can pass them while calling the function from the dictionary. Here’s an example:
“`python
def personalized_greet(name):
return f”Hello, {name}!”
function_dict[‘personalized_greet’] = personalized_greet
Calling the function with an argument
result_personalized = function_dict[‘personalized_greet’](‘Alice’)
print(result_personalized) Output: Hello, Alice!
“`
Handling Non-Existent Keys
When accessing a function in a dictionary, it’s essential to handle potential errors that may arise from non-existent keys. Using `dict.get()` can be helpful:
“`python
Attempting to call a function with a safe approach
function_name = ‘unknown_function’
func = function_dict.get(function_name)
if func:
print(func())
else:
print(f”Function ‘{function_name}’ not found.”)
“`
Practical Use Cases
Using functions in a dictionary can be beneficial in various scenarios, such as:
- Command Pattern: Implementing commands for user interaction.
- Event Handling: Managing events with different handlers based on event types.
- Dynamic Function Execution: Allowing dynamic execution of functions based on user input or program state.
Example Use Case: Command Execution
Consider a simple command execution scenario where user inputs dictate which function to call:
“`python
def add(a, b):
return a + b
def subtract(a, b):
return a – b
command_dict = {
‘add’: add,
‘subtract’: subtract
}
Simulating user input
user_command = ‘add’
result = command_dict[user_command](5, 3)
print(result) Output: 8
“`
This structure promotes clean code and enhances maintainability by centralizing function management.
Expert Insights on Calling Functions in Python Dictionaries
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, functions can be stored as values in dictionaries, allowing for dynamic function calls. This is particularly useful in scenarios where behavior needs to be determined at runtime, enabling more flexible and maintainable code.”
James Liu (Software Engineer, Data Solutions Corp.). “Utilizing dictionaries to map strings to functions is a powerful technique in Python. It allows developers to create command patterns or event handlers that can be easily extended without modifying existing code, promoting better design practices.”
Linda Martinez (Python Educator, Code Academy). “When calling functions stored in a dictionary, it is essential to ensure that the keys are unique and descriptive. This not only aids in readability but also prevents potential errors during function calls, making the code more robust and user-friendly.”
Frequently Asked Questions (FAQs)
How can I store functions in a dictionary in Python?
You can store functions in a dictionary by assigning them as values to keys. For example:
“`python
def greet():
return “Hello!”
functions_dict = {‘greet’: greet}
“`
How do I call a function stored in a dictionary?
To call a function stored in a dictionary, access it using its key and then use parentheses. For example:
“`python
result = functions_dict[‘greet’]()
“`
Can I pass arguments to functions stored in a dictionary?
Yes, you can pass arguments to functions stored in a dictionary. Access the function and include the arguments in parentheses. For example:
“`python
def add(a, b):
return a + b
functions_dict = {‘add’: add}
result = functions_dict[‘add’](5, 3)
“`
What happens if I try to call a non-existent function in a dictionary?
If you attempt to call a non-existent function using a key that does not exist in the dictionary, Python will raise a `KeyError`. Always ensure the key exists before calling the function.
Is it possible to store lambda functions in a dictionary?
Yes, lambda functions can be stored in a dictionary just like regular functions. For example:
“`python
functions_dict = {‘square’: lambda x: x ** 2}
result = functions_dict[‘square’](4)
“`
How can I iterate over functions in a dictionary and call them?
You can iterate over the dictionary using a loop and call each function. For example:
“`python
for func in functions_dict.values():
print(func())
“`
In Python, dictionaries are versatile data structures that can store key-value pairs, where the values can be functions. Calling a function stored in a dictionary involves accessing the function using its corresponding key and then invoking it with parentheses. This method allows for dynamic function calls and can be particularly useful in scenarios such as implementing command patterns, callbacks, or event-driven programming.
One of the significant advantages of using dictionaries to hold functions is the ability to easily manage and organize multiple functions under a single variable. This approach enhances code readability and maintainability, as it allows developers to reference functions by descriptive keys rather than cumbersome function names. Additionally, it facilitates the implementation of more complex logic, such as selecting functions based on user input or other runtime conditions.
calling a function in a dictionary in Python is a straightforward yet powerful technique that promotes clean coding practices. By leveraging this functionality, developers can create more flexible and dynamic applications. Ultimately, understanding this concept can significantly enhance a programmer’s ability to write efficient and organized code.
Author Profile
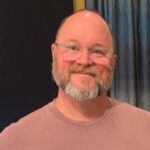
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?