How Can You Change the Image Source in JavaScript?
Can You Change Img Src In JavaScript?
In the dynamic world of web development, the ability to manipulate elements on a webpage is essential for creating interactive and engaging user experiences. One common task that developers often encounter is changing the source of an image dynamically using JavaScript. Whether you’re building a gallery that showcases different images based on user interaction or simply updating a logo based on a user’s preferences, understanding how to change an image’s `src` attribute can elevate your web applications to the next level.
JavaScript provides a straightforward yet powerful way to modify HTML elements, and images are no exception. By leveraging the Document Object Model (DOM), developers can easily access image elements and alter their properties in real-time. This capability not only enhances the visual appeal of a site but also allows for more responsive designs that can adapt to user input or other events.
In this article, we will explore the various methods to change an image’s source using JavaScript, discuss best practices, and provide practical examples that you can implement in your own projects. Whether you’re a seasoned developer or just starting out, mastering this skill will undoubtedly enrich your toolkit and help you create more dynamic web experiences.
Understanding Image Elements in HTML
To manipulate image sources using JavaScript, it’s essential to first understand how images are represented in HTML. An image is typically embedded using the `` tag, which has a `src` attribute that specifies the path to the image file. Here’s an example of a basic image element:
“`html
“`
The `id` attribute allows for easy selection of the image element when using JavaScript.
Changing the Image Source with JavaScript
JavaScript provides a straightforward way to change the `src` attribute of an image dynamically. This can be particularly useful for creating interactive web applications. To change the image source, follow these steps:
- Select the image element using `document.getElementById()` or a similar method.
- Assign a new value to the `src` property.
Here’s an example of how to implement this:
“`javascript
const imgElement = document.getElementById(“myImage”);
imgElement.src = “image2.jpg”;
“`
This code snippet will change the displayed image from “image1.jpg” to “image2.jpg”.
Dynamic Image Changes Based on User Interaction
Changing an image source can also be triggered by user interactions, such as clicking a button. Here’s a practical example that demonstrates this approach:
“`html
“`
In this example, when the button is clicked, the image displayed in the `` tag changes.
Event Handling for Image Changes
JavaScript allows for various event handling techniques to detect when an image source should change. Some common events include:
- Click: Triggered when an element is clicked.
- Hover: Activated when a user hovers over an element.
- Load: Occurs when an image finishes loading.
Using the `addEventListener` method enhances flexibility. For example:
“`javascript
const imgElement = document.getElementById(“myImage”);
imgElement.addEventListener(“mouseover”, function() {
imgElement.src = “image3.jpg”; // Change on hover
});
“`
Considerations When Changing Image Sources
When dynamically changing image sources, consider the following:
- Loading Times: Ensure images are optimized for web use to minimize loading times.
- Fallbacks: Provide alternative content using the `alt` attribute for accessibility.
- Error Handling: Implement error handling for cases where an image fails to load.
Aspect | Consideration |
---|---|
Loading Times | Optimize images to enhance performance. |
Accessibility | Use descriptive alt text for screen readers. |
Error Handling | Consider fallback options if the image fails to load. |
By understanding these principles, developers can effectively manage image sources in their web applications, enhancing user experience and interactivity.
Changing the Image Source Using JavaScript
To change the `src` attribute of an image in JavaScript, you first need to select the image element from the DOM. This can be accomplished using various methods such as `getElementById`, `getElementsByClassName`, or `querySelector`. Once you have a reference to the image element, you can update its `src` property directly.
Methods for Selecting Image Elements
Here are common methods for selecting an image element in the DOM:
- getElementById: This method selects an element by its unique ID.
- getElementsByClassName: This method selects elements that share a specific class name.
- querySelector: This method selects the first element that matches a specified CSS selector.
Example Code for Changing Image Source
The following example demonstrates how to change the `src` of an image using different selection methods:
“`html
“`
This example changes the image source when a button is clicked.
Using Other Selection Methods
Here’s how to achieve the same result using `getElementsByClassName` and `querySelector`:
“`html
“`
“`html
“`
Dynamic Image Loading Considerations
When changing image sources dynamically, consider the following:
- Image Preloading: To avoid display delays, preload images before assigning them as sources.
- Error Handling: Implement error handling to manage cases where the new image fails to load. You can use the `onerror` event.
Example of error handling:
“`javascript
document.getElementById(‘myImage’).onerror = function() {
this.src = ‘fallbackImage.jpg’; // Fallback image if the new source fails
};
“`
Performance Optimization Tips
- Use Efficient Selectors: Choose selectors that minimize DOM traversal time.
- Cache References: Store references to frequently accessed elements to reduce the number of DOM lookups.
- Batch DOM Updates: If changing multiple image sources, consider batching updates to minimize reflows.
Changing the `src` attribute of an image in JavaScript is straightforward and can enhance user interactivity on a webpage. By using efficient DOM selection methods and considering performance optimization, developers can create seamless experiences that respond dynamically to user actions.
Expert Insights on Changing Image Sources with JavaScript
Dr. Emily Carter (Web Development Specialist, Tech Innovations Inc.). “Changing the `src` attribute of an image using JavaScript is a straightforward process that can enhance user experience. By manipulating the DOM, developers can dynamically update images based on user interactions, such as clicks or form submissions, which is essential for creating responsive web applications.”
Michael Chen (Front-End Engineer, Creative Solutions Group). “Using JavaScript to change the `src` of an image is not just about functionality; it’s also about performance. Developers should ensure that the new image is optimized for web use to avoid slowing down page load times. Techniques such as preloading images can be beneficial when implementing dynamic image changes.”
Sarah Thompson (UX/UI Designer, Digital Experience Agency). “From a design perspective, changing image sources dynamically can significantly impact user engagement. It is crucial to maintain visual consistency and ensure that transitions between images are smooth. Implementing fade effects or other animations can improve the overall aesthetic and user satisfaction.”
Frequently Asked Questions (FAQs)
Can you change the `src` attribute of an image using JavaScript?
Yes, you can change the `src` attribute of an image element in JavaScript by selecting the image element and setting its `src` property to a new URL.
How do you select an image element in JavaScript?
You can select an image element using methods such as `document.getElementById()`, `document.querySelector()`, or `document.getElementsByClassName()`, depending on your HTML structure.
What is the syntax to change the `src` of an image in JavaScript?
The syntax is as follows: `document.getElementById(‘imageId’).src = ‘newImageURL.jpg’;` This changes the source of the image with the specified ID.
Can you change the image `src` dynamically based on user actions?
Yes, you can change the image `src` dynamically in response to user actions, such as clicking a button or hovering over an element, by using event listeners.
What happens if the new image URL is invalid?
If the new image URL is invalid, the browser will not display the image, and it may show a broken image icon or an error message, depending on the browser’s handling of image loading errors.
Is it possible to preload images in JavaScript before changing the `src`?
Yes, you can preload images by creating a new `Image` object in JavaScript and setting its `src` property. This allows the image to load in the background before it is displayed.
In summary, changing the `src` attribute of an image element in JavaScript is a straightforward process that enhances the interactivity and dynamism of web applications. By utilizing the Document Object Model (DOM), developers can easily manipulate image sources to display different images based on user actions or other events. This capability is essential for creating responsive designs and engaging user experiences.
One of the primary methods for altering an image’s source is through direct manipulation of the `src` property of the image element. This can be accomplished using various techniques, such as selecting the image element via its ID or class and then assigning a new URL to the `src` attribute. Additionally, event listeners can be employed to trigger these changes in response to user interactions, such as clicks or hover events, further enhancing the functionality of the web page.
It is also important to consider best practices when changing image sources. For instance, preloading images can improve performance and user experience, ensuring that images are displayed without delay. Furthermore, implementing error handling can prevent broken images from appearing if the new source is invalid or inaccessible. Overall, understanding how to effectively change image sources in JavaScript is a valuable skill for web developers aiming to create modern, interactive websites.
Author Profile
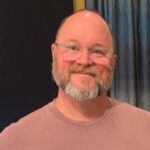
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?