How Do You Initialize a List in Python? A Step-by-Step Guide
How To Initialize List In Python: A Comprehensive Guide
In the world of programming, lists are one of the most versatile and widely used data structures, especially in Python. Whether you’re a seasoned developer or just starting your coding journey, understanding how to initialize a list in Python is fundamental to harnessing the full power of this dynamic language. Lists allow you to store multiple items in a single variable, making it easier to manage collections of data, perform operations, and manipulate information seamlessly.
Initializing a list in Python is not just about creating a simple collection of items; it opens the door to a myriad of possibilities in data handling and algorithm development. From storing user inputs to managing complex datasets, the way you initialize a list can significantly impact your code’s efficiency and clarity. Python offers several methods to create lists, each with its own unique advantages and use cases, allowing for flexibility in how you approach problem-solving.
As we delve deeper into the intricacies of list initialization, we will explore various techniques, best practices, and common pitfalls to avoid. Whether you’re looking to create an empty list, populate it with predefined values, or dynamically generate its contents, this guide will equip you with the knowledge you need to master list initialization in Python. Get ready to enhance your coding skills and
Creating an Empty List
To initialize an empty list in Python, you can use either of the following methods:
- Using square brackets:
“`python
my_list = []
“`
- Using the `list()` constructor:
“`python
my_list = list()
“`
Both methods yield the same result, creating an empty list that can be populated later with data.
Initializing a List with Elements
You can initialize a list with predefined elements by specifying them within the square brackets, separated by commas. For example:
“`python
my_list = [1, 2, 3, 4, 5]
“`
This creates a list containing the integers 1 through 5. You can also initialize a list with mixed data types, such as strings, integers, and floats:
“`python
mixed_list = [1, “apple”, 3.14, True]
“`
Using List Comprehensions
List comprehensions provide a concise way to create lists. They consist of brackets containing an expression followed by a `for` clause, optionally including `if` clauses. For example:
“`python
squared_numbers = [x**2 for x in range(10)]
“`
This initializes a list of squared values for numbers from 0 to 9.
Initializing a List with Repeated Elements
If you need to create a list with repeated elements, you can use the multiplication operator. For instance, to create a list containing five zeros:
“`python
zero_list = [0] * 5
“`
This results in:
“`python
[0, 0, 0, 0, 0]
“`
Initializing a List from a String
You can also initialize a list by converting a string into a list of its characters. This can be accomplished using the `list()` function:
“`python
char_list = list(“hello”)
“`
This will create a list containing:
“`python
[‘h’, ‘e’, ‘l’, ‘l’, ‘o’]
“`
Common Use Cases for List Initialization
Here are some common scenarios where you might need to initialize lists:
- Storing user input: Collecting a series of inputs from users during runtime.
- Data processing: Storing results from calculations or transformations.
- Dynamic lists: Building lists based on conditions or loops.
Example Table of List Initialization Methods
Method | Example | Description |
---|---|---|
Empty List | my_list = [] | Creates an empty list. |
Predefined Elements | my_list = [1, 2, 3] | Creates a list with specified elements. |
List Comprehension | my_list = [x for x in range(5)] | Creates a list based on a condition or expression. |
Repeated Elements | my_list = [0] * 5 | Creates a list with repeated elements. |
From String | my_list = list(“abc”) | Converts a string into a list of characters. |
By utilizing these various methods, you can effectively initialize lists in Python to suit your programming needs.
Creating Lists in Python
Python provides multiple ways to initialize a list. Below are some common methods along with examples.
Using Square Brackets
The simplest way to create a list is by using square brackets. This method allows you to directly define the list elements.
“`python
my_list = [1, 2, 3, 4, 5]
“`
You can also create an empty list this way:
“`python
empty_list = []
“`
Using the `list()` Constructor
Another approach is to use the `list()` constructor. This method is particularly useful for converting other iterable types into a list.
“`python
Creating a list from a string
string_list = list(“Hello”) [‘H’, ‘e’, ‘l’, ‘l’, ‘o’]
Creating a list from a tuple
tuple_list = list((1, 2, 3)) [1, 2, 3]
“`
Initializing Lists with Repeated Elements
You can initialize a list with repeated elements using the multiplication operator. This is especially useful for creating lists with default values.
“`python
A list of ten zeros
zero_list = [0] * 10 [0, 0, 0, 0, 0, 0, 0, 0, 0, 0]
A list of five ‘Hello’
hello_list = [‘Hello’] * 5 [‘Hello’, ‘Hello’, ‘Hello’, ‘Hello’, ‘Hello’]
“`
Using List Comprehensions
List comprehensions provide a concise way to create lists. This method is not only efficient but also allows for complex initialization.
“`python
Creating a list of squares
squares = [x**2 for x in range(10)] [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
Creating a list of even numbers
evens = [x for x in range(20) if x % 2 == 0] [0, 2, 4, 6, 8, 10, 12, 14, 16, 18]
“`
Using the `append()` Method
You can also initialize a list by starting with an empty list and using the `append()` method to add elements one at a time.
“`python
dynamic_list = []
for i in range(5):
dynamic_list.append(i) [0, 1, 2, 3, 4]
“`
Example: Initializing a List of Objects
When dealing with custom objects, you can initialize a list to hold multiple instances.
“`python
class Item:
def __init__(self, name, value):
self.name = name
self.value = value
item_list = [Item(“Item1”, 10), Item(“Item2”, 20)]
“`
Summary of List Initialization Methods
Method | Example | Description |
---|---|---|
Square Brackets | `my_list = [1, 2, 3]` | Directly defines a list with elements. |
`list()` Constructor | `my_list = list((1, 2, 3))` | Converts iterable into a list. |
Repeated Elements | `my_list = [0] * 10` | Creates a list with repeated elements. |
List Comprehensions | `squares = [x**2 for x in range(10)]` | Efficiently generates lists using expressions. |
`append()` Method | `my_list.append(4)` | Adds elements to an initially empty list. |
Expert Insights on Initializing Lists in Python
Dr. Emily Chen (Senior Python Developer, Tech Innovations Inc.). “When initializing lists in Python, it is crucial to choose the method that best fits your use case. For instance, using list comprehensions can enhance performance and readability, especially when generating lists based on existing data.”
Michael Thompson (Lead Software Engineer, CodeCraft Solutions). “The most common methods for initializing lists in Python include using square brackets for empty lists or the `list()` constructor. However, understanding the implications of each method on memory and performance is essential for efficient coding.”
Sarah Patel (Data Scientist, Analytics Pro). “In data science applications, initializing lists with specific data types can be beneficial. Utilizing libraries like NumPy can provide more efficient data structures compared to traditional Python lists, especially for large datasets.”
Frequently Asked Questions (FAQs)
How do I initialize an empty list in Python?
You can initialize an empty list in Python by using empty square brackets: `my_list = []`.
What are the different ways to initialize a list with values in Python?
You can initialize a list with values by using square brackets with comma-separated values, e.g., `my_list = [1, 2, 3]`, or by using the `list()` constructor, e.g., `my_list = list((1, 2, 3))`.
Can I initialize a list with a specific number of identical elements?
Yes, you can initialize a list with identical elements using multiplication, e.g., `my_list = [0] * 5` creates a list with five zeros: `[0, 0, 0, 0, 0]`.
How do I initialize a list using a loop in Python?
You can initialize a list using a loop by appending elements within a loop, e.g., `my_list = []` followed by `for i in range(5): my_list.append(i)` results in `[0, 1, 2, 3, 4]`.
Is it possible to initialize a list with elements from another iterable?
Yes, you can initialize a list from another iterable using the `list()` constructor, e.g., `my_list = list(range(5))` creates a list with elements `[0, 1, 2, 3, 4]`.
What is the difference between a list and a tuple in terms of initialization?
A list is initialized using square brackets `[]` and is mutable, while a tuple is initialized using parentheses `()` and is immutable. For example, `my_list = [1, 2, 3]` and `my_tuple = (1, 2, 3)`.
In Python, initializing a list can be accomplished in several straightforward ways, each suited to different needs and scenarios. The most common method is to use square brackets, which allows for the creation of an empty list or a list pre-populated with elements. Additionally, the `list()` constructor provides an alternative approach, particularly useful when converting other iterable objects into a list format. Understanding these methods is essential for effective data manipulation and storage in Python programming.
Another important aspect of list initialization is the ability to create lists with specific values or patterns. For instance, list comprehensions offer a concise way to generate lists based on existing data, while the multiplication of lists can be used to create a list containing repeated elements. These techniques enhance the flexibility and efficiency of list creation, making it easier for developers to manage collections of data.
In summary, initializing lists in Python is a fundamental skill that opens the door to more advanced programming concepts. By mastering the various methods of list initialization, programmers can improve their code’s readability and functionality. As Python continues to evolve, staying informed about best practices for list management will contribute to more efficient and effective coding strategies.
Author Profile
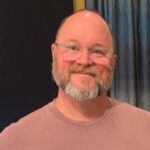
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?