How to Set Default Current Date for Created_At in Laravel Migrations?
In the world of web development, managing databases efficiently is crucial for building robust applications. One of the most powerful tools at a developer’s disposal is Laravel, a popular PHP framework known for its elegant syntax and rich feature set. Among its many capabilities, Laravel migrations stand out as a vital component for creating and modifying database structures seamlessly. When it comes to tracking changes and timestamps, the `created_at` field plays a pivotal role, providing insights into when records are created. However, setting a default date for this field can sometimes be a challenge for developers.
In this article, we will explore the intricacies of setting a default date for the `created_at` column in Laravel migrations, focusing on how to ensure that this field automatically captures the current date and time when a new record is created. We will delve into the best practices for implementing this functionality, highlighting the importance of maintaining accurate timestamps in your database. By understanding the nuances of Laravel migrations and the `created_at` field, you can enhance your application’s data integrity and streamline your development process.
Whether you’re a seasoned Laravel developer or just starting your journey, mastering the management of default dates in migrations will empower you to create more dynamic and responsive applications. Join us as we unravel the steps and considerations necessary for setting up the
Setting Default Values in Laravel Migrations
In Laravel migrations, setting default values for timestamp fields is a common practice, especially for `created_at` and `updated_at` columns. By default, Laravel uses the `CURRENT_TIMESTAMP` for these timestamp fields when they are created. To explicitly define default values for these fields, developers can utilize the `default()` method in their migration files.
To set the default value for the `created_at` column, you may use:
“`php
$table->timestamp(‘created_at’)->default(DB::raw(‘CURRENT_TIMESTAMP’));
“`
This ensures that when a new record is created, the `created_at` field automatically populates with the current date and time.
Example Migration for Timestamps
The following is an example of a migration that includes default timestamp columns:
“`php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
public function up()
{
Schema::create(‘posts’, function (Blueprint $table) {
$table->id();
$table->string(‘title’);
$table->text(‘content’);
$table->timestamp(‘created_at’)->default(DB::raw(‘CURRENT_TIMESTAMP’));
$table->timestamp(‘updated_at’)->default(DB::raw(‘CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP’));
});
}
public function down()
{
Schema::dropIfExists(‘posts’);
}
}
“`
This migration creates a `posts` table with `created_at` and `updated_at` columns. The `updated_at` column also uses `CURRENT_TIMESTAMP` but updates automatically whenever the row is updated.
Advantages of Using Default Timestamps
Utilizing default timestamps in Laravel migrations offers several benefits:
- Automatic Tracking: Automatically tracks when records are created and last updated.
- Consistency: Ensures consistency across all records regarding date and time.
- Ease of Querying: Simplifies querying for records based on creation or update times.
Creating Tables with Default Timestamps
When designing tables that require automatic date tracking, consider the following table structure:
Column Name | Data Type | Default Value |
---|---|---|
id | Big Integer | Auto Increment |
title | String | N/A |
content | Text | N/A |
created_at | Timestamp | CURRENT_TIMESTAMP |
updated_at | Timestamp | CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP |
This structure provides a clear view of how the `created_at` and `updated_at` fields are defined, making it easier for developers to understand and implement timestamp functionality within their applications.
Setting Default Date for Created_At in Laravel Migrations
In Laravel, when you create a migration for a database table, you often need to set a default value for the `created_at` column. This column is typically used to store the timestamp when a record is created. By default, Laravel uses the `timestamps()` method to handle `created_at` and `updated_at` fields automatically. However, if you want to customize or ensure that the `created_at` column always defaults to the current timestamp, you can specify this in your migration file.
Creating a Migration with Default Current Timestamp
To create a migration with a default current timestamp for the `created_at` column, follow these steps:
- **Generate a Migration**
Use the Artisan command to create a new migration:
“`bash
php artisan make:migration create_example_table
“`
- **Edit the Migration File**
Open the migration file located in the `database/migrations` directory. In the `up` method, define your table structure like this:
“`php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreateExampleTable extends Migration
{
public function up()
{
Schema::create(‘example’, function (Blueprint $table) {
$table->id();
$table->timestamps(); // This will create created_at and updated_at columns
// Or you can define created_at manually
$table->timestamp(‘created_at’)->default(now()); // Set default to current timestamp
});
}
public function down()
{
Schema::dropIfExists(‘example’);
}
}
“`
In this example, the `created_at` column is explicitly defined with a default value of `now()`, which returns the current timestamp.
Considerations When Using Current Timestamp
When setting the default value for the `created_at` column, keep the following points in mind:
– **Database Compatibility**: Ensure that your database supports the `now()` function. Most databases like MySQL and PostgreSQL support it.
– **Time Zone Handling**: If your application is timezone-sensitive, make sure to handle timezone conversions appropriately.
– **Automatic Management**: If you use `$table->timestamps()`, Laravel will automatically manage `created_at` and `updated_at`, making explicit definitions unnecessary unless you want specific behaviors.
Example Migration with Custom Defaults
Here’s a complete example of a migration that uses both `timestamps()` and a custom default for `created_at`:
“`php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
public function up()
{
Schema::create(‘posts’, function (Blueprint $table) {
$table->id();
$table->string(‘title’);
$table->text(‘content’);
$table->timestamp(‘created_at’)->default(now());
$table->timestamps(); // This will create updated_at with default functionality
});
}
public function down()
{
Schema::dropIfExists(‘posts’);
}
}
“`
In this example, the `created_at` field is set to default to the current timestamp while allowing Laravel to manage the `updated_at` field automatically.
Testing the Migration
After defining your migration, run the following command to execute it:
“`bash
php artisan migrate
“`
To verify that the `created_at` field is set correctly, you can use Tinker or check directly in your database. You may create a record and observe that the `created_at` field is populated with the current timestamp upon creation.
By following these steps, you can effectively manage default timestamps in your Laravel migrations, ensuring consistent behavior across your application’s database interactions.
Expert Insights on Setting Default Date in Laravel Migrations
Maria Chen (Senior Software Engineer, Tech Innovations Inc.). “Setting the default date for the created_at field in Laravel migrations is crucial for maintaining data integrity. By using the current timestamp, developers ensure that every record is accurately time-stamped upon creation, which is essential for tracking changes over time.”
James Patel (Database Architect, Cloud Solutions Group). “When defining the created_at column in Laravel migrations, utilizing the default value of now() is a best practice. This approach not only simplifies the code but also enhances the readability and consistency of the database records, making it easier for future developers to understand the data lifecycle.”
Linda Torres (Lead Laravel Developer, WebCraft Agency). “Incorporating a default date for the created_at field is more than just a technical detail; it plays a vital role in application analytics. By ensuring that every entry has a precise creation date, teams can perform better data analysis and reporting, which ultimately drives informed decision-making.”
Frequently Asked Questions (FAQs)
What is a Laravel migration?
A Laravel migration is a version control system for your database schema, allowing you to define and modify database tables and columns using PHP code.
How can I set a default value for the created_at column in Laravel migrations?
You can set a default value for the created_at column by using the `default()` method in your migration file, such as `->default(DB::raw(‘CURRENT_TIMESTAMP’))`.
What is the purpose of the created_at column in Laravel?
The created_at column automatically records the timestamp when a record is created, providing a way to track when data was inserted into the database.
Can I customize the name of the created_at column in Laravel?
Yes, you can customize the name of the created_at column by overriding the default timestamps in your model using the `$createdAt` property.
How do I ensure the created_at column updates automatically on record creation?
Laravel automatically manages the created_at column when you use Eloquent ORM, ensuring it updates to the current timestamp upon record creation.
Is it possible to change the default date format for timestamps in Laravel migrations?
Yes, you can change the default date format for timestamps by modifying the `dateFormat` method in your model or by configuring it in the database connection settings.
In Laravel, managing database migrations effectively is crucial for maintaining the integrity and functionality of applications. One common requirement is to set a default value for the `created_at` timestamp in migrations. By utilizing the `current()` method in the migration file, developers can ensure that the `created_at` field automatically captures the current date and time when a record is created. This approach not only simplifies the process of tracking record creation but also enhances data consistency across the application.
Moreover, Laravel provides built-in support for handling timestamps, which includes both `created_at` and `updated_at` fields. By leveraging the `timestamps()` method in the migration schema, developers can easily incorporate these fields into their database tables. This method automatically manages the population of these timestamps, reducing the need for manual intervention and potential errors during record creation and updates.
In summary, setting a default date for the `created_at` field in Laravel migrations is a straightforward yet powerful feature that enhances the overall data management process. By utilizing Laravel’s built-in functionalities, developers can ensure accurate and automatic timestamping, which is essential for tracking the lifecycle of database records. This practice not only improves application performance but also aids in maintaining a clear audit trail of data changes.
Author Profile
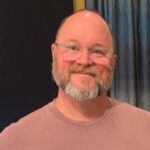
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?