What Is Sequencing in Python and Why Is It Important for Your Coding Journey?
In the world of programming, the ability to organize and manipulate data is paramount, and Python excels in this regard. One of the fundamental concepts that every Python programmer encounters is sequencing. But what exactly does sequencing mean in the context of Python? As you dive into the realm of coding, understanding sequencing will not only enhance your programming skills but also elevate your ability to solve complex problems with elegance and efficiency. This article will unravel the intricacies of sequencing in Python, providing you with the foundational knowledge to harness its power in your projects.
Sequencing in Python refers to the ordered arrangement of elements, which can be manipulated and accessed through various data structures. At its core, sequencing allows programmers to work with collections of items, such as lists, tuples, and strings, in a systematic way. These sequences enable developers to perform operations like iteration, slicing, and indexing, all of which are essential for effective data management and manipulation. As you explore the concept further, you’ll discover how sequencing not only simplifies coding but also opens up a world of possibilities for data analysis and application development.
Moreover, understanding the nuances of sequencing is crucial for optimizing performance and ensuring code readability. By mastering this fundamental concept, you will be better equipped to tackle challenges ranging from simple data retrieval to complex algorithm
Understanding Sequencing in Python
Sequencing in Python refers to the order in which items are arranged in data structures such as lists, tuples, and strings. It plays a crucial role in how data is manipulated and accessed in programming. Python supports several built-in data types that maintain the order of elements, allowing developers to perform various operations systematically.
Types of Sequences
Python features several types of sequences, each offering unique properties and functionalities. The most commonly used sequences include:
- Lists: Mutable sequences that allow modification of elements. Lists can contain mixed data types and support dynamic resizing.
- Tuples: Immutable sequences that cannot be altered after creation. Tuples are typically used to store related pieces of data.
- Strings: Immutable sequences of characters, widely used for handling text.
- Bytes and Bytearrays: Used for binary data, where bytes are immutable, and bytearrays are mutable.
Characteristics of Sequences
Different sequences in Python share some common characteristics, which include:
- Ordered: Elements maintain a specific order, which can be accessed via indexing.
- Indexing: Sequences are zero-indexed, meaning the first element is accessed with index 0.
- Slicing: Portions of sequences can be extracted using a technique known as slicing, allowing for the retrieval of sub-sequences.
Operations on Sequences
Python provides a variety of operations that can be performed on sequences. Below is a summary of some common operations:
Operation | Description |
---|---|
Indexing | Accessing an element using its index. |
Slicing | Retrieving a sub-sequence using a range of indices. |
Concatenation | Combining two sequences into one. |
Repetition | Repeating a sequence multiple times. |
Membership | Checking for the presence of an element in a sequence. |
Example Usage
To illustrate the concept of sequencing, consider the following examples:
- Creating a List:
“`python
my_list = [1, 2, 3, 4, 5]
“`
- Accessing Elements:
“`python
first_element = my_list[0] Accesses 1
“`
- Slicing a List:
“`python
sub_list = my_list[1:4] Retrieves [2, 3, 4]
“`
- Concatenating Lists:
“`python
another_list = [6, 7, 8]
combined_list = my_list + another_list Results in [1, 2, 3, 4, 5, 6, 7, 8]
“`
- Checking Membership:
“`python
is_present = 3 in my_list Returns True
“`
Conclusion on Sequence Handling
Effectively managing sequences is vital for data manipulation in Python. Understanding how to utilize and interact with different types of sequences can enhance programming efficiency and data handling capabilities. Each sequence type offers distinct advantages, and leveraging them appropriately can lead to cleaner and more maintainable code.
Understanding Sequencing in Python
In Python, sequencing refers to the arrangement of items in a specific order. This concept is fundamental as it allows developers to store, access, and manipulate collections of data systematically. The primary data structures that exhibit sequencing properties in Python include lists, tuples, strings, and ranges.
Types of Sequential Data Structures
Python offers several built-in sequential data structures, each with distinct characteristics:
Data Structure | Mutability | Usage |
---|---|---|
List | Mutable | Ordered collection of items, allowing duplicates |
Tuple | Immutable | Ordered collection of items, cannot be changed after creation |
String | Immutable | Sequence of characters, used for text manipulation |
Range | Immutable | Represents a sequence of numbers, commonly used in loops |
Key Features of Python Sequences
- Indexing: Each item in a sequence can be accessed via an index, starting from zero. For example, in a list `my_list = [10, 20, 30]`, `my_list[0]` returns `10`.
- Slicing: This allows extraction of a subset of the sequence. For instance, `my_list[1:3]` returns `[20, 30]`, which includes elements from index 1 to 2.
- Concatenation: Sequences can be combined using the `+` operator. For example, `[1, 2] + [3, 4]` results in `[1, 2, 3, 4]`.
- Repetition: Using the `*` operator, sequences can be repeated. For instance, `[1, 2] * 3` produces `[1, 2, 1, 2, 1, 2]`.
Common Operations on Sequences
Python provides a variety of built-in functions and methods to manipulate sequences:
- Length: Use `len(sequence)` to determine the number of elements.
- Membership Testing: The `in` keyword checks if an element exists within the sequence.
- Iteration: Sequences can be traversed using loops. For instance:
“`python
for item in my_list:
print(item)
“`
Performance Considerations
The performance of operations on sequences can vary significantly:
- Lists:
- Access time is O(1).
- Append operations are O(1) on average; however, insertion and deletion can be O(n) in the worst case.
- Tuples:
- Similar access time as lists, but they are slightly faster due to immutability.
- Strings:
- Access time is O(1), but modifications involve creating new strings, leading to O(n) complexity.
- Ranges:
- Efficiently represent sequences without storing all values, with O(1) access time.
Conclusion on Sequencing in Python
Understanding sequencing in Python is essential for effective programming. Mastering these data structures enhances the ability to handle data efficiently and perform complex operations with ease. The flexibility and ease of use of Python’s sequential data types make them powerful tools for developers.
Understanding Sequencing in Python: Perspectives from Experts
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “Sequencing in Python refers to the ordered arrangement of data structures such as lists, tuples, and strings. It is essential for data manipulation and algorithm implementation, allowing developers to access and process data in a predictable manner.”
Michael Chen (Lead Software Engineer, CodeCraft Solutions). “In Python, sequencing is fundamental to control flow and data organization. Understanding how sequences work enables programmers to leverage Python’s powerful features, such as list comprehensions and slicing, to write more efficient and readable code.”
Sarah Patel (Python Instructor, LearnPython Academy). “The concept of sequencing in Python is crucial for beginners. It introduces them to the idea of ordered collections, which is a building block for more complex programming concepts. Mastering sequences can significantly enhance one’s coding skills and problem-solving abilities.”
Frequently Asked Questions (FAQs)
What is sequencing in Python?
Sequencing in Python refers to the arrangement of elements in a specific order, typically within data structures such as lists, tuples, and strings. It allows for the organization and manipulation of collections of data.
How do you create a sequence in Python?
A sequence in Python can be created using built-in data types like lists, tuples, and strings. For example, a list can be created using square brackets: `my_list = [1, 2, 3]`.
What are the main types of sequences in Python?
The main types of sequences in Python include lists, tuples, strings, and ranges. Each type has its own characteristics, such as mutability and methods available for manipulation.
How can you access elements in a sequence?
Elements in a sequence can be accessed using indexing. In Python, indexing starts at 0, so the first element can be accessed with `sequence[0]`. Negative indexing can also be used to access elements from the end of the sequence.
What operations can be performed on sequences in Python?
Common operations on sequences include indexing, slicing, concatenation, repetition, and membership testing. These operations allow for efficient data retrieval and manipulation.
How does Python handle sequence immutability?
In Python, immutable sequences like tuples and strings cannot be modified after their creation. This means that operations that would alter the sequence, such as item assignment, will raise an error.
Sequencing in Python refers to the arrangement of elements in a specific order within data structures, such as lists, tuples, and strings. These sequences allow for the organization and manipulation of data, enabling developers to access, modify, and iterate through elements efficiently. Understanding how sequencing works is fundamental to leveraging Python’s capabilities in data handling and algorithm implementation.
Key takeaways from the discussion on sequencing include the various types of sequences available in Python, such as mutable and immutable sequences. Lists are mutable, allowing for modifications, while tuples are immutable, providing a fixed structure. Strings, though also immutable, are essential for handling text data. Each type of sequence has its own set of methods and functionalities, which can enhance the programming experience and improve code efficiency.
Moreover, Python’s built-in functions, such as indexing, slicing, and concatenation, play a crucial role in working with sequences. Mastery of these functions enables developers to perform complex data manipulations with ease. Additionally, understanding the implications of sequence types on performance and memory usage can lead to more optimized code, which is especially important in larger applications.
Author Profile
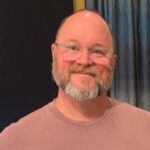
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?