Why Am I Getting a ‘Float Object Is Not Subscriptable’ Error in Python?
### Introduction
In the world of programming, encountering errors is an inevitable part of the journey toward mastering a language. One common error that many developers, both novice and experienced, stumble upon is the infamous “float object is not subscriptable” message. This seemingly cryptic phrase can halt your code in its tracks, leaving you scratching your head and wondering where things went awry. Understanding this error is crucial not only for debugging but also for improving your overall coding proficiency. In this article, we will unravel the mystery behind this error, explore its causes, and provide insights into how to avoid it in the future.
The “float object is not subscriptable” error typically arises in Python when a programmer attempts to index or slice a float variable as if it were a list or a string. This error serves as a reminder of the importance of data types in programming. Python, with its dynamic typing, allows for flexibility, but it also requires a keen awareness of how different data types behave. When you treat a float as a subscriptable object, the interpreter raises this error, signaling that you’ve crossed the boundaries of what that data type can do.
To effectively navigate this error, it’s essential to grasp the underlying principles of Python’s data structures and their respective functionalities. By
Understanding the Error
The error message “Float object is not subscriptable” is a common issue encountered in Python when attempting to index or slice a float object. In Python, the term “subscriptable” refers to an object that supports indexing and can be accessed using square brackets. Objects such as lists, tuples, and dictionaries are subscriptable, allowing the retrieval of elements based on their position or key. However, float objects do not support this functionality.
When this error occurs, it typically indicates that the programmer is trying to access a float variable as if it were a list or another subscriptable data type. Understanding the context in which this error arises is crucial for troubleshooting.
Common Causes of the Error
Several scenarios can lead to encountering the “Float object is not subscriptable” error:
- Incorrect Variable Assignment: Assigning a float value to a variable that was intended to hold a list or a similar subscriptable type.
- Misuse of Function Returns: Functions may return float values when lists or tuples are expected.
- Data Processing Errors: During data manipulation or processing, operations might inadvertently convert a list into a float.
Examples of the Error
Here are some examples demonstrating how the error can occur:
python
# Example 1: Incorrect Indexing
num = 5.0
print(num[0]) # Raises TypeError: ‘float’ object is not subscriptable
# Example 2: Function Return Value
def calculate_average(values):
return sum(values) / len(values)
avg = calculate_average([10, 20, 30])
print(avg[0]) # Raises TypeError: ‘float’ object is not subscriptable
Troubleshooting Steps
To resolve the “Float object is not subscriptable” error, follow these steps:
- Check Variable Types: Ensure that the variable you are trying to index is indeed a list or another subscriptable type.
- Examine Function Outputs: Review the return values of functions to confirm they return the expected data types.
- Debugging: Use print statements or debugging tools to inspect variable types during runtime.
Best Practices to Avoid the Error
To prevent this error from occurring in your code, consider the following best practices:
- Use Type Annotations: Implement type hints to clarify the expected types of variables and function returns.
- Implement Type Checks: Utilize `isinstance()` to verify variable types before performing indexing.
- Maintain Clear Naming Conventions: Use descriptive variable names to differentiate between subscriptable and non-subscriptable objects.
Variable Name | Expected Type | Actual Type |
---|---|---|
num | list | float |
avg | list | float |
By adhering to these practices, developers can minimize the risk of encountering the “Float object is not subscriptable” error, leading to more robust and error-free code.
Understanding the Error Message
The error message “float object is not subscriptable” occurs in Python when attempting to index or slice a float, which is an immutable data type representing a numerical value. In Python, only certain data types, such as lists, tuples, strings, and dictionaries, are subscriptable, meaning they can be indexed or sliced to access specific elements.
Common Causes of the Error
This error commonly arises in several scenarios, including:
- Incorrect Variable Assignment: Assigning a float to a variable and then attempting to access it as if it were a list or dictionary.
- Function Returns: A function that is expected to return a list or another subscriptable type may inadvertently return a float.
- Mathematical Operations: Performing operations that yield a float, followed by an attempt to index the result.
Example Scenarios
Here are some examples that illustrate the occurrence of this error:
python
# Example 1: Incorrect Variable Assignment
my_float = 3.14
print(my_float[0]) # Raises TypeError: ‘float’ object is not subscriptable
# Example 2: Function Returns
def calculate_average(numbers):
return sum(numbers) / len(numbers) # Returns a float
avg = calculate_average([10, 20, 30])
print(avg[0]) # Raises TypeError: ‘float’ object is not subscriptable
# Example 3: Mathematical Operations
result = 5.0 + 3.0
print(result[0]) # Raises TypeError: ‘float’ object is not subscriptable
Debugging Steps
To resolve this error, follow these debugging steps:
- Identify the Variable:
- Check the type of the variable causing the issue using `type(variable)`.
- Trace Function Returns:
- Ensure that functions return the expected data type. Use print statements or logging to verify the return values.
- Review Mathematical Operations:
- Confirm that the operations performed on variables yield the intended types before attempting to index them.
- Use Conditionals:
- Implement conditionals to check types before indexing:
python
if isinstance(my_var, (list, tuple, str, dict)):
print(my_var[0])
else:
print(“Variable is not subscriptable.”)
Preventive Measures
To prevent encountering the “float object is not subscriptable” error in the future, consider the following practices:
- Type Annotations: Use type hints in function definitions to clarify expected input and output types.
- Testing: Write unit tests to verify that functions return the correct types.
- Code Reviews: Conduct regular code reviews to catch type-related issues early.
Understanding the contexts in which the “float object is not subscriptable” error arises is crucial for effective debugging. By following structured debugging steps and implementing preventive measures, you can minimize the occurrence of this error in your Python code.
Understanding the ‘Float Object Is Not Subscriptable’ Error in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “The error ‘Float Object Is Not Subscriptable’ typically arises when a programmer attempts to access an index of a float variable as if it were a list or a dictionary. This misunderstanding often stems from a lack of clarity regarding data types in Python.”
Michael Chen (Lead Software Engineer, CodeMaster Solutions). “To resolve the ‘Float Object Is Not Subscriptable’ error, developers should first ensure that they are not mistakenly treating float values as collections. It is crucial to check the data type of variables when performing indexing operations.”
Sarah Lopez (Python Educator and Author, Learn Python Today). “This error serves as a reminder of the importance of understanding Python’s type system. New programmers should familiarize themselves with the properties of different data types to avoid common pitfalls like trying to subscript non-iterable objects.”
Frequently Asked Questions (FAQs)
What does the error “float object is not subscriptable” mean?
The error indicates that you are trying to access an index or key on a float object, which is not allowed in Python. Float objects represent numerical values and do not support indexing.
How can I reproduce the “float object is not subscriptable” error?
You can reproduce this error by attempting to use square brackets to access an element of a float variable, such as `my_float = 3.14; my_float[0]`.
What are common scenarios that lead to this error?
Common scenarios include mistakenly treating a float variable as a list or dictionary, often due to variable name conflicts or incorrect data type assumptions during calculations or data processing.
How can I fix the “float object is not subscriptable” error?
To fix the error, ensure that you are not attempting to index a float variable. Verify your variable types and use appropriate data structures, such as lists or dictionaries, when you need to access elements by index or key.
Can this error occur when using functions that return float values?
Yes, this error can occur if you attempt to index the result of a function that returns a float. Always check the return type of functions and ensure you handle the data appropriately.
What debugging steps can I take to resolve this issue?
To debug, check the variable types using the `type()` function, review your code for any unintended variable assignments, and ensure that you are using the correct data structures for your intended operations.
The error message “float object is not subscriptable” typically arises in Python programming when an attempt is made to access elements of a float variable using indexing, similar to how one would access elements in a list or a dictionary. This indicates a fundamental misunderstanding of data types, as floats are single numeric values and do not support indexing. Consequently, this error serves as a reminder of the importance of recognizing and correctly utilizing data types within Python to avoid runtime errors.
To resolve this issue, it is crucial to review the code to identify where the float variable is being incorrectly indexed. Common scenarios include mistakenly treating a float as a list or attempting to access elements from a function that returns a float value. By ensuring that only appropriate data types are being indexed, programmers can effectively eliminate this error and enhance the robustness of their code.
the “float object is not subscriptable” error highlights the significance of understanding Python’s data structures. It encourages developers to develop a clear mental model of how different data types function and interact. By doing so, they can write more efficient and error-free code, ultimately leading to improved programming practices and better software development outcomes.
Author Profile
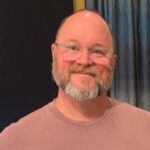
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?