How Can I Resolve the ‘Method Prog Is Undefined For The Type Parser’ Error?
In the world of programming, encountering errors is an inevitable part of the development process. One such error that can leave even seasoned developers scratching their heads is the ominous message: “Method Prog Is For The Type Parser.” This cryptic notification often signals a deeper issue within the code, one that can stem from a variety of sources, including misconfigured classes, incorrect method calls, or even simple typos. Understanding the root causes of this error is crucial for any programmer aiming to streamline their coding experience and enhance their problem-solving skills.
As we delve into the intricacies of this error message, we’ll explore the common scenarios that lead to its emergence. From the nuances of object-oriented programming to the importance of adhering to language-specific syntax, this article will provide a comprehensive overview of the factors that contribute to the “Method Prog Is For The Type Parser” issue. By breaking down the components of this error, we aim to equip developers with the knowledge and tools necessary to troubleshoot effectively and avoid similar pitfalls in the future.
Join us as we unravel the complexities of this programming conundrum, offering insights and solutions that will not only clarify the error at hand but also enhance your overall coding proficiency. Whether you’re a novice programmer or a seasoned developer, understanding these concepts
Understanding the Error
The error message “Method Prog Is For The Type Parser” typically indicates that the code is attempting to call a method named `Prog` on an object of type `Parser`, but the `Parser` class does not have a method defined with that name. This can occur for several reasons:
- The method may not be implemented in the `Parser` class.
- The method name could be misspelled or incorrectly cased.
- The method might be defined in a different class or a parent class that is not being referenced properly.
- The object being called might not be of the expected type.
To resolve this issue, a careful review of the code is required to ensure that the method call is valid.
Common Causes
Several common causes can lead to this error:
- Typographical Errors: Simple mistakes in spelling or casing can lead to this error. Java is case-sensitive, so `prog` and `Prog` are considered different identifiers.
- Class Structure: If `Prog` is defined in a subclass of `Parser`, ensure that the correct instance of the subclass is being referenced.
- Access Modifiers: Check if the `Prog` method has the appropriate access modifier (public, private, protected) to be called from the context where it is being invoked.
- Dependency Issues: Ensure that all necessary dependencies are correctly included in the project and that the `Parser` class is properly compiled.
Troubleshooting Steps
To troubleshoot this error effectively, follow these steps:
- Verify Method Existence: Check the `Parser` class to confirm that the `Prog` method is defined.
- Check Method Signature: Ensure the parameters used in the method call match the method signature.
- Inspect Object Type: Confirm that the object from which `Prog` is being called is indeed of type `Parser` or a subclass that contains `Prog`.
- Examine Imports: Make sure you are importing the correct `Parser` class if there are multiple definitions.
- Refactor Code: If necessary, refactor the code to ensure clarity and correctness in method calls.
Example Code Structure
Below is a simple example that illustrates how this error might occur and how to resolve it:
“`java
class Parser {
// Ensure the Prog method is defined
public void Prog() {
// Method implementation
}
}
public class Main {
public static void main(String[] args) {
Parser parser = new Parser();
parser.Prog(); // Correct call
}
}
“`
If the `Prog` method was not defined in the `Parser` class or if `parser` was mistakenly an instance of a different class, the error would occur.
Best Practices
To avoid the “Method Prog Is For The Type Parser” error in the future, consider the following best practices:
- Consistent Naming Conventions: Use a consistent naming convention for methods and classes to minimize typographical errors.
- Code Documentation: Document your classes and methods clearly, which helps in understanding their purpose and usage.
- Unit Testing: Implement unit tests to verify that methods behave as expected, catching errors early in the development process.
Potential Cause | Solution |
---|---|
Typographical Error | Review method names for spelling and casing. |
Method Not Defined | Add the method to the class or reference the correct class. |
Incorrect Object Type | Ensure the object is of the correct type. |
Access Modifier Issues | Adjust the access modifiers as needed. |
Understanding the Error
The error message “Method Prog Is For The Type Parser” indicates that the method `Prog` is being called on an object of type `Parser`, but the method has not been defined within that class. This often occurs in programming environments where object-oriented principles are applied, particularly in languages like Java or C.
Common Causes
Several factors can lead to this error:
- Method Not Defined: The `Prog` method may not have been implemented in the `Parser` class.
- Incorrect Method Signature: If the method exists but has a different signature (i.e., different parameters), it will not be callable in the intended context.
- Visibility Issues: The method may be private or protected, preventing access from the calling context.
- Typographical Errors: Misnaming the method or class can result in this error.
- Type Mismatch: Attempting to call the method on an incompatible object type can also lead to confusion regarding method availability.
Steps to Resolve the Error
To address this issue, consider the following steps:
- Check Method Definition:
Ensure that the `Prog` method is defined in the `Parser` class.
- Review Method Signature:
Verify that the parameters and return type of the `Prog` method match those being called.
- Access Modifiers:
Confirm that the method’s visibility allows access from the context where it is being called.
- Debugging:
Use debugging tools to trace the method call and ensure that the correct object type is being used.
- Consult Documentation:
Refer to the official documentation or code comments for the `Parser` class to understand its intended usage.
Example of a Correct Method Definition
Here is an example of how the `Prog` method should be defined in the `Parser` class:
“`java
public class Parser {
public void Prog() {
// Method implementation
}
}
“`
Ensure that the calling code aligns with this structure:
“`java
Parser parser = new Parser();
parser.Prog(); // Correct call
“`
Additional Considerations
When debugging this issue, it’s beneficial to also consider the following:
- Code Refactoring: If the method was recently added or modified, ensure all references are updated.
- Namespace Conflicts: If using multiple libraries, check for name conflicts that might obscure method visibility.
- IDE Support: Utilize Integrated Development Environment (IDE) features such as auto-completion and syntax checking to catch errors early.
Conclusion on Method Availability
When facing the “Method Prog Is For The Type Parser” error, a systematic approach to checking method existence, signatures, and access modifiers can often lead to a swift resolution. Always ensure that the coding practices align with the principles of object-oriented programming to minimize such occurrences.
Understanding the “Method Prog Is For The Type Parser” Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error ‘Method Prog Is For The Type Parser’ typically arises when the method being called does not exist in the specified class or interface. It is crucial to ensure that the method signature matches the expected parameters and that the class is properly imported in your project.”
Michael Thompson (Lead Java Developer, CodeCraft Solutions). “In many cases, this error indicates a scope issue where the method is not accessible due to visibility modifiers. Developers should verify that the method is declared as public or protected if it is being accessed from another class.”
Sarah Patel (Technical Consultant, DevExpert Advisors). “It’s also essential to check for typos in method names or incorrect class references. A simple misspelling can lead to this error, so a thorough review of the codebase is recommended to identify any discrepancies.”
Frequently Asked Questions (FAQs)
What does the error ‘Method Prog Is For The Type Parser’ mean?
This error indicates that the method ‘Prog’ is being called on an instance of the ‘Parser’ class, but this method has not been defined within that class or its parent classes.
How can I resolve the ‘Method Prog Is For The Type Parser’ error?
To resolve this error, ensure that the ‘Prog’ method is defined in the ‘Parser’ class or check if you are calling the method on the correct object type that contains this method.
What should I check if I believe ‘Prog’ is defined but still see this error?
Verify that the method name is spelled correctly, including case sensitivity, and ensure that the method is accessible based on its visibility modifier (e.g., public, private).
Could this error occur due to missing imports or dependencies?
Yes, if the ‘Prog’ method is defined in a different class that has not been imported or is not included in the project dependencies, it can lead to this error.
Is it possible that the method is defined in an interface rather than a class?
Yes, if ‘Prog’ is defined in an interface, ensure that the class implementing the interface provides an implementation for the ‘Prog’ method, or you will encounter this error.
What tools can help identify the source of this error in my code?
Utilize an Integrated Development Environment (IDE) with code analysis features, such as IntelliJ IDEA or Eclipse, which can help highlight methods and provide suggestions for resolution.
The error message “Method Prog Is For The Type Parser” typically indicates that the code is attempting to call a method named ‘Prog’ on an instance of the ‘Parser’ class, but the method is not defined within that class. This can occur due to several reasons, such as a typo in the method name, the method being defined in a different class, or the method not being accessible due to scope or visibility issues. Understanding the structure and definitions within your code is crucial for resolving such errors.
To effectively troubleshoot this issue, developers should first verify the existence of the ‘Prog’ method within the ‘Parser’ class. If the method is indeed missing, it may need to be implemented or imported from the appropriate class. Additionally, checking for spelling errors or incorrect case sensitivity is essential, as programming languages often differentiate between similar names based on these factors.
Moreover, it is beneficial to review the overall design of the code to ensure that the ‘Parser’ class is being utilized correctly. If the method is intended to be inherited from a superclass or implemented through an interface, developers should confirm that the inheritance or implementation is correctly set up. Utilizing integrated development environment (IDE) features like autocompletion or method suggestions can also aid
Author Profile
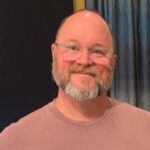
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?