Can I Program Arduino in Python? Exploring the Possibilities!
Introduction
In the world of electronics and programming, Arduino has emerged as a beloved platform for hobbyists, educators, and professionals alike. Traditionally, programming an Arduino board has meant diving into the C/C++ language, which can be a barrier for those more familiar with Python. However, as the demand for more accessible programming languages grows, many are left wondering: can I program Arduino in Python? This question opens the door to a wealth of possibilities, allowing enthusiasts to leverage their Python skills to create innovative projects and explore the exciting realm of embedded systems.
As we delve into this topic, we will explore the various ways Python can interface with Arduino boards, transforming the way you approach your projects. From libraries that bridge the gap between Python and C/C++ to alternative platforms that allow for direct Python programming, the landscape is rich with options. Whether you are a seasoned programmer looking to expand your toolkit or a beginner eager to jump into the world of Arduino, understanding the potential of Python in this context can significantly enhance your creative endeavors.
Join us as we navigate the intersection of Python and Arduino, uncovering the tools, techniques, and resources that empower you to bring your ideas to life with ease. Whether you’re interested in robotics, IoT, or simply experimenting with electronics, the
Using MicroPython for Arduino Programming
MicroPython is a lean implementation of the Python programming language specifically designed for microcontrollers and embedded systems. It allows users to write Python code to control hardware, making it a suitable alternative for programming Arduino boards, particularly those that support it, such as the ESP8266 and ESP32.
To get started with MicroPython on Arduino, follow these steps:
- Flash MicroPython Firmware: Download the MicroPython firmware for your specific board and flash it using tools like esptool.py.
- Connect to REPL: Use a terminal program such as PuTTY or screen to connect to the board’s REPL (Read-Eval-Print Loop) environment.
- Write and Upload Code: You can write Python scripts using any text editor and upload them to your board using tools like ampy or rshell.
MicroPython provides a range of libraries for various functionalities, including:
- GPIO control
- I2C and SPI communication
- Networking capabilities
Using Python with Firmata
Firmata is a protocol that allows communication between software on a host computer and microcontrollers like Arduino. By uploading the Firmata firmware to an Arduino board, users can control the board using Python scripts on their computer.
To use Firmata with Python, you need to:
- Install pyFirmata: This is a Python library that implements the Firmata protocol.
- Upload Standard Firmata: Upload the StandardFirmata sketch from the Arduino IDE to your Arduino board.
- Write Python Code: Use the pyFirmata library to write code that interacts with the Arduino hardware.
An example setup for using Firmata with Python is as follows:
python
import pyfirmata
board = pyfirmata.Arduino(‘/dev/ttyACM0’) # Adjust the port as needed
it = pyfirmata.util.Iterator(board)
it.start()
# Set pin mode
board.digital[13].mode = pyfirmata.OUTPUT
# Blink LED on pin 13
while True:
board.digital[13].write(1) # Turn LED on
time.sleep(1)
board.digital[13].write(0) # Turn LED off
time.sleep(1)
Limitations and Considerations
While programming Arduino with Python offers flexibility, there are some limitations and considerations to keep in mind:
- Performance: Python is generally slower than C/C++, the native languages for Arduino programming. For time-critical applications, this may affect performance.
- Resource Constraints: Microcontrollers have limited memory and processing power. Ensure that your Python code is optimized for efficiency.
- Library Support: Not all Arduino libraries are available in Python. Check for alternative libraries in MicroPython or pyFirmata.
Method | Pros | Cons |
---|---|---|
MicroPython |
|
|
Firmata |
|
|
By understanding these methods and their implications, users can effectively leverage Python for their Arduino projects, enhancing their development experience while considering the inherent constraints.
Programming Arduino with Python
Using Python to program Arduino boards is possible through several libraries and frameworks designed to facilitate this interaction. While traditional Arduino programming is done in C/C++, Python offers an alternative for those who prefer its syntax and features.
Popular Libraries for Python and Arduino
Several libraries enable Python to communicate with Arduino boards:
- pySerial: A simple library for serial communication with the Arduino.
- Arduino-Python3: This library allows for easy interaction with Arduino hardware using Python.
- Firmata: A protocol that allows you to control Arduino hardware from software on your computer. The `pyFirmata` library is a popular implementation.
Setting Up Your Environment
To program an Arduino using Python, you need to follow these steps:
- **Install Python**: Ensure you have Python installed on your machine. You can download it from the official Python website.
- **Install pySerial**: Use pip to install the pySerial library.
bash
pip install pyserial
- **Upload Firmata to Arduino**:
- Open the Arduino IDE.
- Go to `File` > `Examples` > `Firmata` > `StandardFirmata`.
- Upload this sketch to your Arduino board.
Example: Controlling an LED
Here’s a simple example of how to control an LED connected to an Arduino using Python and the pyFirmata library.
- Install pyFirmata:
bash
pip install pyFirmata
- Python Code:
python
import pyfirmata
import time
# Set up the board
board = pyfirmata.Arduino(‘COM3’) # Change ‘COM3’ to your board’s port
# Define the pin where the LED is connected
led_pin = board.get_pin(‘d:9:o’) # Digital pin 9 as output
# Blink the LED
while True:
led_pin.write(1) # Turn on
time.sleep(1) # Wait for 1 second
led_pin.write(0) # Turn off
time.sleep(1) # Wait for 1 second
Common Challenges and Solutions
When programming Arduino with Python, you may encounter some challenges:
Challenge | Solution |
---|---|
Connection issues | Ensure the correct COM port is selected. |
Permissions errors on Linux | Run the script with `sudo` or add your user to the `dialout` group. |
Timeout errors during communication | Check the baud rate settings and ensure the Arduino sketch is properly uploaded. |
Programming Arduino in Python is feasible and can enhance the accessibility of hardware programming. By utilizing libraries like pyFirmata and pySerial, users can leverage Python’s simplicity while still harnessing the capabilities of Arduino. This approach is ideal for rapid prototyping and educational purposes.
Can Python Be Used for Arduino Programming? Insights from Experts
Dr. Emily Carter (Embedded Systems Engineer, Tech Innovations Inc.). “While Arduino is primarily programmed using C/C++, there are libraries such as MicroPython and CircuitPython that enable developers to write Arduino sketches in Python. This flexibility allows for rapid prototyping and can make the platform more accessible to those familiar with Python.”
Mark Thompson (IoT Solutions Architect, Future Tech Labs). “Using Python to program Arduino devices can significantly simplify the development process for beginners. However, it is essential to recognize that performance and compatibility may vary depending on the specific Arduino board and the libraries used.”
Sarah Lee (Educational Technology Specialist, STEM Learning Center). “Integrating Python with Arduino offers an excellent opportunity for educational settings. It allows students to leverage their existing Python skills while learning about hardware interaction, thus bridging the gap between software and hardware education.”
Frequently Asked Questions (FAQs)
Can I program Arduino in Python?
Yes, you can program Arduino in Python using libraries such as PyMata or Firmata, which allow you to communicate with the Arduino board over a serial connection.
What is Firmata?
Firmata is a protocol that allows communication between software on a host computer and microcontrollers like Arduino. It enables you to control Arduino hardware using Python or other programming languages.
Do I need to install additional software to use Python with Arduino?
Yes, you need to install the Firmata firmware on your Arduino board and a Python library such as PyMata or pyFirmata to facilitate the communication between Python and Arduino.
Are there any limitations to programming Arduino with Python?
Yes, programming Arduino with Python may have limitations in terms of performance and real-time processing compared to using the native Arduino IDE with C/C++. Python is generally slower and may not be suitable for time-sensitive applications.
Can I use Python for all Arduino projects?
While you can use Python for many projects, some complex or time-critical applications may require the efficiency of C/C++. Evaluate your project requirements before choosing Python as your programming language.
Is it possible to run Python code directly on Arduino?
No, Arduino boards do not natively support Python. However, you can use MicroPython on compatible boards like the ESP8266 or ESP32, which allows you to run Python code directly on those microcontrollers.
programming Arduino using Python is indeed possible, although it requires specific tools and libraries to bridge the gap between Python and the Arduino platform. The most notable library for this purpose is PyMata, which allows users to communicate with Arduino boards using Python scripts. This approach offers the flexibility of Python’s syntax and extensive libraries, making it appealing for those who prefer Python over the traditional C/C++ programming languages typically used for Arduino development.
Additionally, using Python for Arduino programming can enhance the development process, especially for those familiar with Python’s capabilities in data analysis, machine learning, and automation. The integration of Python can also facilitate rapid prototyping and testing, as it allows for quicker iterations and modifications compared to traditional methods. However, it is essential to note that performance may vary depending on the complexity of the tasks and the specific libraries used.
Ultimately, while the traditional Arduino IDE and C/C++ remain the standard for many projects, the ability to program Arduino in Python opens up new possibilities for developers and hobbyists alike. This versatility can lead to innovative applications and a broader audience for Arduino projects, as it lowers the barrier for those who might be more comfortable with Python than with C/C++. Embracing this method can enrich
Author Profile
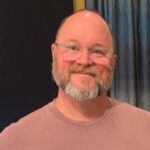
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?