How Can You Easily Find a File by Name Using PowerShell?
In today’s fast-paced digital landscape, the ability to efficiently locate files on your computer can save you precious time and enhance your productivity. Whether you are a seasoned IT professional, a developer, or simply a tech-savvy individual, mastering the art of file management is essential. Enter PowerShell, a powerful command-line shell and scripting language that allows users to automate tasks and manage system resources with ease. One of its most valuable capabilities is the ability to find files by name, a feature that can streamline your workflow and keep your projects organized.
PowerShell provides a robust set of tools for searching through directories and locating files based on specific criteria, including their names. With its versatile cmdlets and straightforward syntax, users can execute complex searches that would otherwise be tedious through traditional file exploration methods. Whether you need to pinpoint a single document among thousands or conduct batch searches across multiple drives, PowerShell equips you with the necessary commands to make the process efficient and effective.
As we delve deeper into the world of PowerShell, we will explore various techniques and commands that can help you harness the full potential of file searching. From simple queries to more advanced filtering options, you’ll discover how to navigate your file system like a pro, ensuring that you can always find exactly what you
Using PowerShell to Find Files by Name
To locate files by their names using PowerShell, the `Get-ChildItem` cmdlet is the primary tool. This cmdlet allows users to retrieve files and directories, and it can be combined with other parameters to refine searches effectively.
A basic command structure for finding files by name looks like this:
“`powershell
Get-ChildItem -Path “C:\Path\To\Search” -Filter “filename.ext”
“`
In this command:
- `-Path` specifies the directory where the search will begin.
- `-Filter` allows you to define the filename you are looking for.
You can also use wildcards in the `-Filter` parameter. For instance, if you’re searching for all `.txt` files, you could use:
“`powershell
Get-ChildItem -Path “C:\Path\To\Search” -Filter “*.txt”
“`
Searching Recursively
If you need to search through all subdirectories, the `-Recurse` parameter is essential. Here’s how to use it:
“`powershell
Get-ChildItem -Path “C:\Path\To\Search” -Filter “filename.ext” -Recurse
“`
This command will search for `filename.ext` in the specified directory and all its subdirectories.
Using the Where-Object Cmdlet
For more complex searches, the `Where-Object` cmdlet can be utilized to filter results based on specific conditions. For example, to find all files that contain “report” in their name, you can use:
“`powershell
Get-ChildItem -Path “C:\Path\To\Search” -Recurse | Where-Object { $_.Name -like “*report*” }
“`
This method provides greater flexibility and allows for additional filtering based on file attributes.
Example Table of Commands
Command | Description |
---|---|
Get-ChildItem -Path "C:\Path" -Filter "file.txt" |
Finds a specific file named “file.txt” in the specified path. |
Get-ChildItem -Path "C:\Path" -Filter "*.jpg" -Recurse |
Finds all JPEG files in the specified path and its subdirectories. |
Get-ChildItem -Path "C:\Path" | Where-Object { $_.Length -gt 1MB } |
Lists files larger than 1MB in the specified path. |
Case Sensitivity
It’s important to note that file searches in PowerShell are case-insensitive by default. If you require case-sensitive search, use the `-CaseSensitive` parameter in your command. However, this feature is often more relevant in contexts where the file system itself supports case sensitivity, such as certain Linux file systems accessed via Windows Subsystem for Linux (WSL).
By employing these techniques, users can efficiently locate files by name across directories using PowerShell, enhancing productivity and simplifying file management tasks.
Using PowerShell to Find Files by Name
To locate files by name using PowerShell, various cmdlets can be utilized. The most common cmdlet for this purpose is `Get-ChildItem`, often abbreviated as `gci`. This cmdlet allows users to retrieve files and directories from the file system.
Basic Command Syntax
The basic syntax for using `Get-ChildItem` to find files by name is as follows:
“`powershell
Get-ChildItem -Path
“`
- `
`: Specifies the folder where the search will begin. - `
`: Defines the name of the file or pattern to search for (e.g., `*.txt` for all text files).
Example Commands
Here are some practical examples of how to use PowerShell to find files by their names:
- Find a specific file:
“`powershell
Get-ChildItem -Path “C:\Documents” -Filter “report.docx”
“`
- Find all text files in a directory:
“`powershell
Get-ChildItem -Path “C:\Documents” -Filter “*.txt”
“`
- Find files by a partial name:
“`powershell
Get-ChildItem -Path “C:\Documents” -Filter “*report*”
“`
Searching Recursively
To search for files in all subdirectories, include the `-Recurse` parameter. This allows for a deeper search within the specified directory structure.
“`powershell
Get-ChildItem -Path “C:\Documents” -Filter “*.txt” -Recurse
“`
Using Wildcards
PowerShell supports wildcard characters for flexible searching:
- `*`: Matches zero or more characters.
- `?`: Matches a single character.
Examples:
- To find all `.jpg` files:
“`powershell
Get-ChildItem -Path “C:\Images” -Filter “*.jpg”
“`
- To find files starting with “img” followed by any character:
“`powershell
Get-ChildItem -Path “C:\Images” -Filter “img?.jpg”
“`
Filtering and Formatting Output
To further refine search results, the output can be filtered and formatted using additional cmdlets like `Where-Object` and `Select-Object`.
- Example of filtering by file size:
“`powershell
Get-ChildItem -Path “C:\Documents” -Recurse | Where-Object { $_.Length -gt 1MB }
“`
- Example of selecting specific properties:
“`powershell
Get-ChildItem -Path “C:\Documents” -Recurse | Select-Object Name, Length, LastWriteTime
“`
Saving Results to a File
To save the search results to a text file, use the `Out-File` cmdlet:
“`powershell
Get-ChildItem -Path “C:\Documents” -Filter “*.txt” -Recurse | Out-File “C:\Output\TextFiles.txt”
“`
This command captures all `.txt` files found in the specified directory and its subdirectories and saves them in a file named `TextFiles.txt`.
Conclusion of Commands
Utilizing these commands and techniques within PowerShell will enhance your file search capabilities, making it easier to manage and locate files efficiently. PowerShell’s flexibility with patterns, recursion, and output formatting allows for tailored file searches to meet specific needs.
Expert Insights on Using PowerShell to Find Files by Name
Jessica Tran (Senior Systems Administrator, TechSolutions Inc.). “Utilizing PowerShell to find files by name is not only efficient but also enhances productivity. The command ‘Get-ChildItem -Recurse -Filter
‘ allows users to search through directories and subdirectories seamlessly, making it an invaluable tool for system administrators.”
Mark Chen (IT Security Consultant, CyberGuard Corp.). “When searching for files by name in PowerShell, it is crucial to consider the implications of using wildcard characters. They can significantly broaden your search results, but they may also lead to retrieving sensitive files inadvertently. Always review the results carefully to maintain security compliance.”
Aisha Patel (DevOps Engineer, Cloud Innovations). “PowerShell’s ability to combine file searching with other commands, such as ‘Where-Object’, allows for more refined searches based on additional criteria, such as file size or modification date. This flexibility makes it a powerful tool for developers and IT professionals alike.”
Frequently Asked Questions (FAQs)
How can I find a file by name using PowerShell?
You can use the `Get-ChildItem` cmdlet combined with the `-Filter` parameter to find a file by name. For example, `Get-ChildItem -Path “C:\Path\To\Directory” -Filter “filename.txt”` will search for “filename.txt” in the specified directory.
Is it possible to search for files recursively in PowerShell?
Yes, you can search recursively by using the `-Recurse` parameter with `Get-ChildItem`. For example, `Get-ChildItem -Path “C:\Path\To\Directory” -Filter “filename.txt” -Recurse` will search for the file in all subdirectories.
Can I search for files by partial name in PowerShell?
Yes, you can use wildcards in the `-Filter` parameter. For instance, `Get-ChildItem -Path “C:\Path\To\Directory” -Filter “*partOfFileName*”` will return all files containing “partOfFileName” in their names.
What if I want to search for files with a specific extension?
You can specify the extension in the `-Filter` parameter. For example, `Get-ChildItem -Path “C:\Path\To\Directory” -Filter “*.txt”` will list all text files in the specified directory.
How can I output the search results to a text file?
You can redirect the output to a text file using the `>` operator. For example, `Get-ChildItem -Path “C:\Path\To\Directory” -Filter “filename.txt” > C:\Path\To\Output\results.txt` will save the results to “results.txt”.
What command can I use to find files by name and display their full path?
You can use the `Select-Object` cmdlet to display the full path. For example, `Get-ChildItem -Path “C:\Path\To\Directory” -Filter “filename.txt” | Select-Object FullName` will show the full path of the found file.
In summary, utilizing PowerShell to find files by name is an efficient and powerful method for managing files within the Windows operating system. PowerShell provides a variety of cmdlets, such as `Get-ChildItem`, which can be employed to search for files based on specific criteria, including name patterns. This capability is particularly useful for system administrators and users who need to locate files quickly across extensive directory structures.
Moreover, PowerShell’s flexibility allows for advanced search functionalities, such as filtering by file type, date modified, and size. By using wildcards and regular expressions, users can refine their searches to pinpoint exact matches or broader categories, enhancing the search process’s effectiveness. This level of customization is a significant advantage over traditional search methods.
Lastly, integrating PowerShell scripts into routine tasks can streamline workflows and reduce the time spent on file management. By automating the search process, users can focus on more critical tasks while ensuring that they can access the necessary files promptly. Overall, mastering PowerShell’s file search capabilities can lead to improved productivity and efficiency in file management tasks.
Author Profile
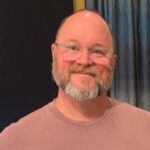
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?