How Can You Resolve a JavaScript Error in the Main Process?
In the ever-evolving landscape of software development, encountering errors is an inevitable part of the journey. Among the myriad of issues that developers face, the cryptic message “A Javascript Error In The Main Process” stands out as a particularly perplexing challenge, especially for those working with frameworks like Electron. This error can halt applications in their tracks, leaving developers scratching their heads and users frustrated. Understanding the nuances of this error not only aids in troubleshooting but also enhances overall coding practices. In this article, we will delve into the underlying causes, implications, and effective solutions to this common yet disruptive issue.
Overview
At its core, “A Javascript Error In The Main Process” signifies a breakdown in the execution of JavaScript code that is critical to the main thread of an application. This error can stem from various sources, including syntax mistakes, improper API usage, or even issues with dependencies. When such an error occurs, it disrupts the flow of the application, often resulting in crashes or unresponsive behavior that can significantly impact user experience.
As we explore this topic further, we will examine the common scenarios that lead to this error, the tools available for diagnosing the underlying problems, and best practices for prevention. By gaining a deeper understanding of this issue, developers
Common Causes of JavaScript Errors in the Main Process
JavaScript errors in the main process can stem from various issues within the application. Understanding these causes is crucial for effective troubleshooting. Common factors include:
- Incorrect Code Syntax: A simple typo or misplacement of brackets can lead to errors.
- Module Loading Issues: If modules fail to load properly due to path errors or missing files, it can halt execution.
- Concurrency Problems: Issues arising from asynchronous code can lead to race conditions and unhandled promise rejections.
- Resource Limitations: Memory or processing constraints can result in crashes or errors, particularly in applications with heavy resource demands.
Troubleshooting Steps
When encountering a JavaScript error in the main process, follow these systematic troubleshooting steps:
- Check the Console: The console often provides detailed error messages and stack traces that can pinpoint the source of the issue.
- Review Recent Changes: If the error appeared after recent updates, reverting those changes may resolve the problem.
- Use Debugging Tools: Utilize built-in debugging tools or third-party software to step through the code and identify problematic areas.
- Test in Isolation: Isolate the code that is suspected to be causing the error to determine if it functions correctly on its own.
Error Handling Best Practices
Implementing robust error handling practices can mitigate the impact of errors and improve the resilience of applications. Consider the following best practices:
- Use Try-Catch Statements: Surround critical code with try-catch blocks to gracefully handle exceptions.
- Log Errors: Implement logging to capture errors for later analysis, aiding in the identification of patterns or frequent issues.
- User Feedback: Provide meaningful error messages to users, guiding them on potential next steps or workarounds.
Best Practice | Description |
---|---|
Try-Catch | Allows developers to handle errors without crashing the application. |
Error Logging | Captures errors for later review, helping to prevent future occurrences. |
User Notifications | Informs users of issues and suggests corrective actions. |
Preventing Future Errors
To reduce the likelihood of encountering JavaScript errors in the main process, adopt proactive measures:
- Code Reviews: Regular peer reviews can catch potential issues before they reach production.
- Automated Testing: Implement unit tests and integration tests to ensure code quality and functionality.
- Continuous Integration: Utilize CI tools to automate testing during the development process, catching errors early.
By following these guidelines and best practices, developers can effectively manage and mitigate JavaScript errors in the main process, leading to smoother application performance and enhanced user experience.
Understanding the Error
The error message “A Javascript Error In The Main Process” typically indicates a failure during the execution of a JavaScript code that is critical to the main process of an application, often seen in frameworks like Electron. This error can arise due to various factors, including:
- Syntax errors in JavaScript code
- Missing dependencies or modules
- Issues in the application’s configuration
- Problems with file paths or resources
Identifying the root cause requires careful inspection of logs and the context in which the error occurs.
Common Causes
Several issues can lead to this error, including:
- Incorrect Code Syntax: Mistakes in the JavaScript code can lead to runtime errors.
- Unresolved Dependencies: If the application depends on external libraries or modules that are not properly installed, it may fail to execute.
- Improper Configuration: Configuration files that are not set correctly can disrupt the application’s operation.
- File Path Issues: Incorrect paths to resources can cause the application to fail to load necessary files.
Troubleshooting Steps
To troubleshoot the “A Javascript Error In The Main Process”, consider the following steps:
- Check the Console Logs: Use the developer tools or any logging mechanism to look for specific error messages.
- Validate JavaScript Syntax: Use tools like ESLint to analyze and correct syntax errors.
- Verify Dependencies: Ensure all required modules are installed and up to date. Use the following command:
“`bash
npm install
“`
- Review Configuration Files: Double-check configuration files for any potential misconfigurations.
- Test File Paths: Make sure all file paths used in the application are correct and accessible.
Preventive Measures
To prevent the occurrence of this error in the future, implement these best practices:
- Code Reviews: Regularly conduct code reviews to catch potential issues early.
- Use Linting Tools: Integrate linting tools into the development process to catch syntax errors automatically.
- Automated Testing: Implement unit and integration tests to ensure code changes do not introduce new errors.
- Dependency Management: Keep dependencies updated and regularly check for deprecated packages.
Example Error Log
An example of a typical error log entry might look like this:
Timestamp | Error Type | Description |
---|---|---|
2023-10-01 12:00:00 | Error: SyntaxError | Unexpected token in JSON at position 10 |
2023-10-01 12:00:01 | Error: ModuleNotFoundError | Cannot find module ‘express’ |
2023-10-01 12:00:02 | Error: ReferenceError | foo is not defined |
Reviewing logs like these can help pinpoint issues more effectively.
While this section does not contain a conclusion, it is important to remember that addressing JavaScript errors in the main process requires thorough investigation and adherence to best practices in coding and application management.
Understanding JavaScript Errors in the Main Process
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “JavaScript errors in the main process often stem from improper handling of asynchronous operations. Developers must ensure that promises are correctly resolved and that error handling is implemented to avoid crashing the application.”
Mark Thompson (Lead Developer, Web Solutions Group). “When encountering a JavaScript error in the main process, it is crucial to analyze the stack trace for clues. Many times, these errors arise from dependencies that are not properly loaded or initialized, leading to runtime exceptions.”
Linda Zhang (Technical Architect, FutureTech Labs). “To mitigate the risk of JavaScript errors in the main process, implementing a robust error logging system is essential. This allows developers to capture and analyze errors in real-time, facilitating quicker resolutions and improving application stability.”
Frequently Asked Questions (FAQs)
What does “A Javascript Error In The Main Process” mean?
This error typically indicates that there is an issue with the JavaScript code executing in the main thread of an application, often related to Electron applications. It suggests that the application has encountered an unexpected problem that prevents it from running properly.
What are common causes of this error?
Common causes include syntax errors in JavaScript code, missing dependencies, incorrect configurations, or issues with the application’s environment. It may also arise from improper handling of asynchronous operations.
How can I troubleshoot this error?
To troubleshoot, check the application logs for detailed error messages, review the JavaScript code for syntax issues, ensure all dependencies are correctly installed, and verify that the application is running in the appropriate environment.
Can this error affect my application’s performance?
Yes, this error can significantly impact application performance by causing crashes or unresponsiveness. It prevents the application from executing its intended functions, leading to a poor user experience.
Is there a way to prevent this error from occurring?
Preventing this error involves writing clean, well-structured code, conducting thorough testing, and using error handling mechanisms to catch exceptions. Regularly updating dependencies and maintaining the application environment can also help mitigate risks.
What should I do if I cannot resolve the error?
If the error persists, consider seeking assistance from developer communities or forums, reviewing official documentation, or consulting with a software development professional for expert guidance.
In summary, encountering a “Javascript Error In The Main Process” typically indicates an issue within the Electron framework, which is commonly used for building cross-platform desktop applications. This error can arise from various underlying causes, including problems with the application’s code, issues with dependencies, or conflicts with the operating system. Identifying the specific source of the error is crucial for effective troubleshooting and resolution.
Key takeaways from the discussion include the importance of thorough debugging practices. Developers should utilize tools such as console logs and error tracking to pinpoint the exact line of code or module causing the issue. Additionally, ensuring that all dependencies are up to date and compatible with the application can significantly reduce the likelihood of encountering such errors.
Furthermore, it is advisable to consult the Electron documentation and community forums for insights and solutions shared by other developers who may have faced similar challenges. Implementing best practices in error handling and maintaining a clean codebase can also mitigate the risk of future errors, leading to a more stable application experience for users.
Author Profile
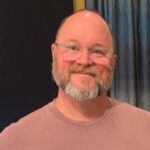
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?