Can You Program Arduino Using Python? Exploring the Possibilities!
In the ever-evolving world of electronics and programming, Arduino has emerged as a powerful platform for hobbyists and professionals alike. Traditionally, programming Arduino boards has been synonymous with C and C++, but as the programming landscape expands, many enthusiasts are left wondering: Can Arduino be programmed in Python? This question opens the door to a fascinating intersection of hardware and software, where the simplicity and versatility of Python can enhance the capabilities of Arduino projects. In this article, we will explore the possibilities, tools, and methodologies that allow Python to interface seamlessly with Arduino, empowering creators to build innovative projects with ease.
As we delve into this topic, it’s important to recognize the growing popularity of Python in various fields, including data science, web development, and automation. Its readability and extensive libraries make it an attractive choice for both beginners and experienced programmers. While Arduino’s native programming environment is rooted in C/C++, the integration of Python offers a refreshing alternative that can simplify the development process and broaden the scope of what can be achieved. This article will provide an overview of how Python can be utilized with Arduino, highlighting the benefits and potential challenges that come with this approach.
Whether you are a seasoned Arduino user looking to expand your skillset or a newcomer eager to dive into the world of
Python Programming for Arduino
Arduino can indeed be programmed using Python, which opens up various possibilities for developers and hobbyists alike. While the primary language for Arduino programming is C/C++, the integration of Python allows for more versatile and higher-level programming options. This capability is primarily achieved through several libraries and tools designed to bridge the gap between Python and Arduino.
Popular Methods to Program Arduino in Python
There are several methods to use Python for programming Arduino boards:
- MicroPython: A lean implementation of Python 3 designed to run on microcontrollers. MicroPython is capable of running directly on boards like the ESP8266, ESP32, and some Arduino models.
- Firmata Protocol: An open-source protocol that allows communication between Arduino and software on a host computer. By using the `pyFirmata` library, users can control Arduino pins directly from Python scripts.
- Arduino-Python3: A package that allows direct communication with Arduino boards using Python. It simplifies the process of sending and receiving data between the Arduino and Python applications.
Setting Up Python with Arduino
To start programming your Arduino with Python, follow these steps:
- Install Python: Ensure that Python is installed on your computer. You can download it from the official Python website.
- Install Required Libraries: Depending on the method you choose, you may need to install specific libraries. For example:
- For Firmata: Install the `pyFirmata` library using pip:
“`
pip install pyFirmata
“`
- For MicroPython: Download the firmware and upload it to your microcontroller.
- Upload the Firmata Sketch: Open the Arduino IDE and upload the StandardFirmata sketch to your Arduino board.
- Write Python Code: Use your favorite IDE to write Python scripts that interact with the Arduino.
Example Code Using Firmata
Here is a simple example of how to blink an LED connected to pin 13 using the `pyFirmata` library:
“`python
import pyfirmata
import time
Initialize the board
board = pyfirmata.Arduino(‘/dev/ttyACM0’) Replace with your port
Set up the pin
led = board.get_pin(‘d:13:o’) Digital pin 13 as output
while True:
led.write(1) Turn LED on
time.sleep(1) Wait for 1 second
led.write(0) Turn LED off
time.sleep(1) Wait for 1 second
“`
Comparison of Programming Methods
The following table outlines the key characteristics of the different methods available for programming Arduino using Python:
Method | Use Case | Complexity | Supported Boards |
---|---|---|---|
MicroPython | Run Python natively on microcontrollers | Medium | ESP8266, ESP32, and some Arduino boards |
Firmata | Control Arduino from a host computer | Low | All boards that support Firmata |
Arduino-Python3 | Direct communication with Arduino | Medium | All boards with a serial interface |
By leveraging these methods, developers can effectively utilize Python to interact with Arduino hardware, broadening the scope of projects and enhancing the programming experience.
Understanding Python on Arduino
While Arduino is primarily programmed using its own language, which is based on C/C++, there are several ways to leverage Python for Arduino programming. This can be particularly useful for users who are more familiar with Python or wish to utilize its extensive libraries and frameworks.
Using MicroPython
MicroPython is a lean implementation of Python 3 that is designed to run on microcontrollers. It enables the use of Python to control hardware components.
- Supported Boards: MicroPython is compatible with several Arduino boards, including:
- Arduino Uno
- Arduino Nano
- ESP8266
- ESP32
- Installation Steps:
- Download the MicroPython firmware for your board.
- Use a tool like `esptool.py` to flash the firmware onto the board.
- Connect to the board using a serial terminal or an IDE that supports MicroPython.
- Basic Example:
“`python
from machine import Pin
led = Pin(2, Pin.OUT) Initialize pin 2 as an output
led.on() Turn the LED on
“`
Using CircuitPython
CircuitPython is a derivative of MicroPython tailored for education and ease of use. It simplifies the process of programming microcontrollers with Python.
- Key Features:
- Easy to install and use.
- Built-in support for libraries, making it beginner-friendly.
- Live code editing via USB.
- Supported Hardware:
- Adafruit boards (e.g., Circuit Playground, Feather)
- Some Arduino boards compatible with CircuitPython.
- Example Code:
“`python
import board
import digitalio
led = digitalio.DigitalInOut(board.D13)
led.direction = digitalio.Direction.OUTPUT
led.value = True Turn the LED on
“`
Using Firmata with Python
Firmata is a protocol for communication between microcontrollers and software on computers. It allows Python scripts to control Arduino boards using the Firmata protocol.
- Installation Requirements:
- Install the Firmata library on the Arduino IDE.
- Use Python libraries such as `pyFirmata` to communicate with the board.
- Setup Steps:
- Upload the StandardFirmata sketch to your Arduino.
- Install the `pyFirmata` library using pip:
“`bash
pip install pyFirmata
“`
- Write a Python script to control the Arduino.
- Basic Example:
“`python
from pyfirmata import Arduino, util
board = Arduino(‘COM3’) Replace with the correct port
it = util.Iterator(board)
it.start()
led = board.get_pin(‘d:13:o’) Digital pin 13 as output
led.write(1) Turn the LED on
“`
Considerations When Using Python with Arduino
When opting to program Arduino with Python, several factors should be taken into account:
- Performance: Python may introduce latency compared to native C/C++ code.
- Library Support: Some libraries available in C/C++ may not have direct equivalents in Python.
- Learning Curve: While Python is user-friendly, adapting to microcontroller programming may still present challenges.
Approach | Pros | Cons |
---|---|---|
MicroPython | Lightweight, Python syntax | Limited hardware compatibility |
CircuitPython | Beginner-friendly, easy setup | Mostly for Adafruit boards |
Firmata | Leverages existing libraries | Requires additional setup |
By utilizing these methods, programmers can effectively use Python to work with Arduino, opening up new possibilities for rapid prototyping and development.
Expert Insights on Programming Arduino with Python
Dr. Emily Carter (Embedded Systems Specialist, Tech Innovations Inc.). “While Arduino is primarily designed to be programmed in C/C++, Python can indeed be used through libraries such as MicroPython and CircuitPython. These adaptations allow developers to leverage Python’s simplicity and readability, making it accessible for beginners and rapid prototyping.”
James Liu (Senior Software Engineer, Robotics Research Lab). “Using Python with Arduino opens up new possibilities for educational projects and hobbyist applications. However, it is essential to understand the limitations in terms of performance and hardware capabilities, as Python is generally slower than C/C++. For tasks requiring real-time processing, traditional languages may still be preferable.”
Maria Gonzalez (IoT Solutions Architect, Future Tech Solutions). “The integration of Python into Arduino programming is a game changer for IoT projects. It allows for easier integration with web services and cloud platforms, enhancing the functionality of Arduino devices. However, developers should be mindful of the specific Python variant they choose, as compatibility can vary based on the hardware in use.”
Frequently Asked Questions (FAQs)
Can Arduino be programmed in Python?
Yes, Arduino can be programmed in Python using libraries such as MicroPython and CircuitPython, which allow you to write Python code that runs on compatible microcontrollers.
What is MicroPython?
MicroPython is a lean implementation of Python 3 designed to run on microcontrollers and in constrained environments, enabling developers to use Python for hardware programming.
What is CircuitPython?
CircuitPython is a version of MicroPython designed specifically for education and beginners, providing an easy-to-use interface for programming Adafruit’s hardware and other compatible boards.
Do I need to install special software to program Arduino in Python?
Yes, you need to install MicroPython or CircuitPython firmware on your Arduino-compatible board, along with a suitable development environment like Thonny or Mu Editor.
Can I use standard Python libraries with Arduino?
Standard Python libraries may not be directly usable due to hardware limitations, but many libraries are specifically designed for MicroPython and CircuitPython to provide similar functionality.
What are the advantages of using Python for Arduino programming?
Using Python simplifies the coding process, enhances readability, and allows for rapid prototyping, making it accessible for beginners and those familiar with Python.
while Arduino boards are primarily programmed using C/C++ through the Arduino IDE, it is indeed possible to program them using Python. This can be achieved through various libraries and frameworks, such as MicroPython and CircuitPython, which allow developers to write Python code that can be executed on compatible microcontrollers. These alternatives provide a more accessible entry point for those familiar with Python, expanding the potential user base for Arduino projects.
Furthermore, Python can also be utilized in conjunction with Arduino through serial communication. This method allows users to write Python scripts on a computer that communicate with an Arduino board, enabling the execution of complex tasks and data processing. This flexibility highlights the versatility of Arduino platforms and the growing trend of integrating different programming languages in embedded systems development.
Key takeaways from this discussion include the recognition that while traditional Arduino programming relies on C/C++, the incorporation of Python opens new avenues for creativity and ease of use. Developers can leverage Python’s simplicity to prototype and develop applications more rapidly. As the ecosystem continues to evolve, the integration of Python with Arduino is likely to expand, fostering innovation and collaboration within the maker community.
Author Profile
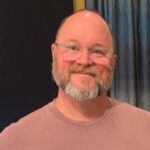
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?