What Does ‘None’ Mean in Python: Understanding Its Role and Usage?
In the world of Python programming, understanding the nuances of data types is crucial for writing effective code. One such data type that often sparks curiosity among both novice and seasoned developers is `None`. This seemingly simple keyword holds significant importance in Python, serving as a placeholder for the absence of a value or a null reference. Whether you’re debugging your code, managing function returns, or working with data structures, grasping the concept of `None` can elevate your programming skills and enhance your ability to handle various scenarios with confidence.
At its core, `None` represents the absence of a value, making it a unique and versatile element in Python. Unlike other data types, such as integers or strings, `None` is not equivalent to zero or an empty string; instead, it signifies that a variable has no value assigned to it. This distinction is essential for developers as it allows for clearer logic in code, enabling them to manage conditions and states effectively. Understanding how `None` interacts with other data types and functions can lead to more robust and error-free programming practices.
Moreover, `None` plays a pivotal role in function definitions and return values. When a function does not explicitly return a value, it implicitly returns `None`, which can be crucial for maintaining the flow of a program.
Understanding None in Python
In Python, `None` is a special constant that represents the absence of a value or a null value. It is an object of its own datatype, the `NoneType`. This can be particularly useful in various programming situations where you need to signify that a variable does not have a value assigned.
Characteristics of None
- Unique Object: `None` is a singleton, which means there is only one instance of `None` in a Python program. This is important for comparisons and logical checks.
- Falsy Value: In a Boolean context, `None` evaluates to “. This makes it useful in conditional statements where you want to check if a variable has been assigned a meaningful value.
- Type: The type of `None` is `NoneType`, which can be checked using the `type()` function.
Common Uses of None
`None` is commonly used in various scenarios, including:
- Default Function Parameters: You can use `None` as a default parameter value to indicate that no specific value has been provided.
- Return Value for Functions: Functions that do not explicitly return a value will return `None` by default.
- Placeholder for Optional Data: It can be used as a placeholder in data structures, such as lists or dictionaries, when a value is not applicable.
Examples of Using None
Here are some examples that illustrate the use of `None` in Python:
“`python
def example_function(param=None):
if param is None:
print(“No value provided”)
else:
print(f”Value provided: {param}”)
example_function() Output: No value provided
example_function(5) Output: Value provided: 5
“`
In this example, the function `example_function` checks if the parameter `param` is `None` and responds accordingly.
Comparison with Other Values
When comparing `None` to other values, it’s crucial to use the identity operator `is` rather than equality operator `==`. This ensures that you are checking whether a variable is actually `None`, not just equal to it.
Comparison | Result |
---|---|
`None is None` | True |
`None == None` | True |
`None is not None` | |
`None == 0` | |
`None == “”` |
Using `is` provides a clearer and more efficient check for `None`, as it directly compares the identity of the objects.
Conclusion on Usage
In summary, `None` is a fundamental aspect of Python programming that allows developers to handle the absence of values effectively. Understanding its characteristics and appropriate contexts for use can greatly enhance the clarity and functionality of Python code.
Understanding None in Python
In Python, `None` is a special constant that represents the absence of a value or a null value. It is an object of its own datatype, the `NoneType`. Unlike other programming languages that may use `null` or `nil`, Python explicitly uses `None` to denote a lack of value.
Characteristics of None
- Singleton Nature: There is only one instance of `None` in a Python program, making it a singleton. This means that any variable assigned to `None` will point to the same object in memory.
- Boolean Context: In a boolean context, `None` evaluates to “. It is one of the few values in Python that evaluates to “ alongside `0`, `0.0`, `”` (empty string), and `[]` (empty list).
- Type: The type of `None` can be checked using the built-in `type()` function:
“`python
type(None) Output:
“`
Common Use Cases for None
`None` is utilized in various scenarios:
- Default Function Arguments: Functions often use `None` as a default value for parameters to indicate that no value has been provided:
“`python
def my_function(param=None):
if param is None:
param = []
Function logic here
“`
- Return Value: When a function does not explicitly return a value, it implicitly returns `None`. This is useful for functions that perform actions rather than calculations:
“`python
def print_message():
print(“Hello, World!”)
result = print_message() result will be None
“`
- Placeholders: `None` can serve as a placeholder for variables that will be assigned later:
“`python
data = None
Some processing occurs
data = fetch_data() Now data holds the result from fetch_data
“`
Comparison with Other Values
When comparing `None` with other values, it is important to use the `is` operator rather than `==` to check for identity:
Comparison | Result | Explanation |
---|---|---|
`None == None` | `True` | Both are the same object. |
`None is None` | `True` | Confirms that both refer to the same singleton. |
`None == 0` | “ | `None` is not equal to zero. |
`None is ` | “ | `None` is distinct from “. |
Checking for None
To check if a variable is `None`, the most efficient and pythonic way is to use the `is` operator:
“`python
if variable is None:
Execute code for None case
“`
Using `==` could lead to unexpected behavior if the variable is an object that implements a custom equality method.
`None` plays a critical role in Python programming, serving as an indicator of no value. Understanding its functionality, usage, and behavior is essential for effective programming in Python.
Understanding the Significance of None in Python
Dr. Emily Carter (Senior Python Developer, Tech Innovations Inc.). “In Python, None is a special constant that represents the absence of a value or a null value. It is crucial for functions that do not explicitly return a value, as they return None by default, allowing developers to check for the absence of a return value in a clean and readable manner.”
Mark Thompson (Lead Software Engineer, CodeCraft Solutions). “Understanding None is essential for effective error handling in Python. It allows programmers to differentiate between a variable that has been initialized but holds no value and one that has not been initialized at all, thereby preventing potential runtime errors and improving code robustness.”
Sarah Lee (Python Educator and Author, LearnPythonNow). “Many beginners often confuse None with other data types like 0 or an empty string. However, None is its own unique type, which can be particularly useful for signaling that a variable is intentionally left empty, making the code more expressive and easier to understand.”
Frequently Asked Questions (FAQs)
What does None mean in Python?
None is a special constant in Python that represents the absence of a value or a null value. It is an object of its own datatype, the NoneType.
How is None different from other data types in Python?
None is unique as it signifies no value or a null state, whereas other data types like integers, strings, and lists represent actual data. None is often used to indicate that a variable has not been assigned a meaningful value.
Can None be used in conditional statements?
Yes, None can be used in conditional statements. It evaluates to in boolean contexts, meaning that if a variable is set to None, it will not execute the block of code under an if statement.
How do you check if a variable is None?
You can check if a variable is None using the equality operator (==) or the identity operator (is). The preferred method is using `is`, as it checks for identity rather than equality.
What are common use cases for None in Python?
Common use cases for None include default function arguments, representing the absence of a return value in functions, and initializing variables that may later hold a value.
Can None be assigned to a variable?
Yes, None can be assigned to a variable. This is often done to initialize a variable when the actual value is not yet known or to reset a variable to indicate it no longer holds a meaningful value.
In Python, the keyword ‘None’ represents a special constant that signifies the absence of a value or a null value. It is an object of its own datatype, the NoneType, and is commonly used to indicate that a variable has no assigned value or to denote the end of a function that does not explicitly return a value. Understanding the role of None is crucial for effective programming in Python, as it helps developers manage cases where a value is not applicable or when a function should not return anything.
One of the key takeaways regarding None is its use in conditional statements. Since None evaluates to in a boolean context, it can be effectively used to check for the existence of a value. This characteristic is particularly useful when dealing with optional parameters in functions or when initializing variables that may later hold data. Additionally, None can be utilized as a default argument in functions, allowing for flexible function signatures and behavior.
Furthermore, it is important to note that None is not the same as , 0, or an empty string. Each of these represents different concepts in Python, and confusing them with None can lead to logical errors in code. As such, developers should be mindful of the distinctions between these values and use None judicious
Author Profile
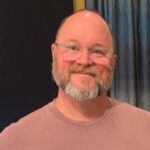
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?