Why Can’t I Reference a Non-Static Variable from a Static Context?
In the world of programming, particularly in object-oriented languages like Java, understanding the distinction between static and non-static variables is crucial for writing efficient, error-free code. One common error that many developers encounter is the infamous “Non-static variable this cannot be referenced from a static context.” This seemingly cryptic message can be a source of frustration for both novice and seasoned programmers alike. However, it serves as a vital reminder of the principles that govern object-oriented design and the importance of context in code execution.
As we delve into this topic, we’ll explore the underlying concepts that lead to this error, shedding light on the differences between static and non-static variables. Static variables belong to the class itself, while non-static variables are tied to individual instances of that class. This fundamental difference can lead to confusion, especially when developers try to access instance-specific data from a static context, resulting in the error message that can halt progress in its tracks.
By examining real-world examples and common scenarios that trigger this error, we can demystify the concept and provide clarity on how to navigate these challenges effectively. Whether you’re debugging a complex codebase or simply looking to enhance your understanding of Java’s object-oriented principles, this article will equip you with the knowledge needed to tackle the ”
Understanding the Error Message
The error message “Non-Static Variable This Cannot Be Referenced From A Static Context” typically arises in programming languages like Java when a static method attempts to access a non-static variable or method directly. This situation occurs because static methods belong to the class itself rather than to any specific instance of the class. Non-static variables, however, are tied to instances of the class.
In simpler terms, static methods do not have access to instance variables (non-static) unless they are associated with an instance of the class. This restriction is crucial for maintaining the integrity of the object-oriented programming model.
Key Concepts
To better understand the error, it’s essential to grasp the following concepts:
- Static Variables and Methods: These belong to the class and can be accessed without creating an instance of the class.
- Non-Static Variables and Methods: These belong to an instance of the class and require an object to be accessed.
Common Scenarios Leading to the Error
The error can occur in various scenarios, including:
- Calling a non-static method from a static method.
- Accessing non-static fields directly within a static context.
Example of the Error
Consider the following Java code snippet:
“`java
public class Example {
private int instanceVariable = 10; // Non-static variable
public static void staticMethod() {
System.out.println(instanceVariable); // Error: Cannot reference non-static variable from static context
}
}
“`
In this example, `staticMethod()` tries to access `instanceVariable` directly, leading to the error.
How to Resolve the Error
To resolve this error, you can use one of the following approaches:
- Create an Instance: Instantiate the class within the static method to access the non-static variable.
“`java
public static void staticMethod() {
Example example = new Example();
System.out.println(example.instanceVariable); // Correctly accesses the non-static variable
}
“`
- Make the Variable Static: If appropriate, you can change the variable to static.
“`java
private static int instanceVariable = 10; // Now static
“`
Best Practices
To avoid encountering this error in the future, consider the following best practices:
- Use static methods for operations that do not require instance data.
- Clearly distinguish between static and instance methods and variables.
- Avoid excessive reliance on static methods to maintain object-oriented principles.
Comparison Table
Aspect | Static | Non-Static |
---|---|---|
Belongs To | Class | Instance |
Access | Can be accessed without an object | Requires an object to access |
Usage | Utility functions or constants | Instance-specific data |
Understanding the Error Message
The error message “Non-static variable this cannot be referenced from a static context” arises in object-oriented programming languages, particularly in Java. This occurs when a static method or block attempts to access an instance variable or method directly.
Key Concepts
- Static Context: Refers to methods or blocks that belong to the class rather than any specific instance. Static methods can be called without creating an object of the class.
- Instance Context: Refers to methods and variables that belong to an instance of the class. They require an object of the class to be accessed.
Why the Error Occurs
- Static methods do not have access to instance variables because they do not operate on an object.
- An attempt to access instance members from a static context leads to ambiguity, as the static context does not maintain a reference to any specific object instance.
Common Scenarios Leading to the Error
Understanding the common scenarios that lead to this error can help developers avoid it effectively.
Scenario 1: Calling Instance Methods from Static Methods
“`java
class Example {
int instanceVariable = 10;
static void staticMethod() {
System.out.println(instanceVariable); // Error
}
}
“`
Scenario 2: Creating Instance Variables in Static Blocks
“`java
class Example {
static {
int instanceVariable = 5; // Error
}
}
“`
Scenario 3: Accessing Instance Variables in a Static Context
“`java
class Example {
int instanceVariable = 20;
static void staticMethod() {
System.out.println(this.instanceVariable); // Error
}
}
“`
How to Resolve the Error
To resolve this error, consider the following strategies:
- Convert Static to Instance: Change the static method to an instance method if you need to access instance variables.
“`java
class Example {
int instanceVariable = 10;
void instanceMethod() {
System.out.println(instanceVariable); // No error
}
}
“`
- Create an Instance: If you need to keep the method static, create an instance of the class within the static method.
“`java
class Example {
int instanceVariable = 10;
static void staticMethod() {
Example example = new Example();
System.out.println(example.instanceVariable); // No error
}
}
“`
- Use Static Variables: If applicable, consider using static variables for values that do not require an instance context.
“`java
class Example {
static int staticVariable = 10;
static void staticMethod() {
System.out.println(staticVariable); // No error
}
}
“`
Best Practices to Avoid the Error
Implementing best practices can minimize the occurrence of this error in your code.
- Understand Class Structure: Clearly differentiate between static and instance members in your classes.
- Use Clear Naming Conventions: Distinguish static variables and methods from instance variables and methods through naming conventions.
- Limit Static Usage: Use static members judiciously, reserving them for utility functions or constants that don’t require object state.
- Regular Code Reviews: Conduct code reviews focusing on the correct use of static and instance contexts.
The “Non-static variable this cannot be referenced from a static context” error serves as a reminder of the distinctions between static and instance members in object-oriented programming. Understanding and adhering to these principles will lead to more robust and error-free code.
Understanding Non-Static Variables in Static Contexts
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). Non-static variables are tied to a specific instance of a class, which means they require an object to be referenced. In contrast, static methods and variables belong to the class itself, making it impossible to directly access non-static members without creating an instance of the class.
Michael Thompson (Lead Developer, CodeCraft Solutions). The error message ‘Non-Static Variable This Cannot Be Referenced From A Static’ serves as a reminder of the fundamental principles of object-oriented programming. It emphasizes the distinction between class-level and instance-level data, which is crucial for maintaining encapsulation and data integrity.
Sarah Lin (Software Architect, FutureTech Labs). To resolve this issue, developers should ensure that they are invoking non-static variables from an instance of the class. This practice not only aligns with the object-oriented paradigm but also enhances code readability and maintainability.
Frequently Asked Questions (FAQs)
What does the error “Non-Static Variable This Cannot Be Referenced From A Static Context” mean?
This error indicates that you are trying to access an instance variable (non-static) from a static method or context. Static methods do not have access to instance variables unless an instance of the class is created.
How can I resolve the “Non-Static Variable This Cannot Be Referenced From A Static” error?
To resolve this error, either change the static method to an instance method or create an instance of the class within the static method to access the non-static variables.
Can I access non-static variables directly from a static method?
No, non-static variables belong to instances of the class, while static methods belong to the class itself. You must create an instance of the class to access non-static variables from a static method.
What is the difference between static and non-static variables in Java?
Static variables belong to the class and are shared among all instances, while non-static variables are specific to each instance of the class. Static variables can be accessed without creating an instance of the class.
Are there scenarios where accessing non-static variables from static methods is necessary?
Yes, there may be scenarios where static methods need to interact with instance data. In such cases, you should pass the instance as a parameter or create an instance within the static method.
What are the implications of using static methods in object-oriented programming?
Using static methods can lead to less flexible code, as they do not operate on instance data. This can hinder polymorphism and the benefits of encapsulation, making it important to use static methods judiciously.
The error message “Non-static variable cannot be referenced from a static context” typically arises in object-oriented programming languages such as Java. This error indicates an attempt to access an instance variable or method from a static context, which is inherently linked to the class itself rather than to any specific instance of that class. Understanding the distinction between static and non-static members is crucial for effective programming, as it directly impacts the organization and functionality of the code.
Static members belong to the class and can be accessed without creating an instance of the class. In contrast, non-static members are associated with specific instances and require an object to be referenced. This fundamental difference is essential for managing memory and ensuring that the program operates as intended. Developers must be mindful of this distinction to avoid common pitfalls that can lead to runtime errors and hinder code maintainability.
Key takeaways from this discussion include the importance of recognizing the context in which variables and methods are accessed. To resolve the error, developers can either change the static method to an instance method or create an instance of the class to access the non-static variable. Additionally, adopting best practices such as clear documentation and consistent naming conventions can help prevent confusion regarding static and non-static members, ultimately leading to more robust and error
Author Profile
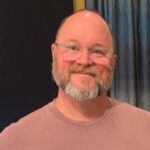
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?