How Can You Find a Key from a Value in Python?
In the world of programming, particularly in Python, dictionaries serve as one of the most versatile and powerful data structures. They allow you to store data in key-value pairs, making it easy to retrieve information based on a unique identifier. However, what happens when you find yourself needing to locate a key based on its corresponding value? This situation can be a bit tricky, especially when dealing with large datasets or when values are not unique. In this article, we will explore various methods to efficiently find a key from a value in Python, equipping you with the tools to navigate this common challenge with ease.
Understanding how to reverse the typical key-to-value lookup can significantly enhance your coding efficiency and problem-solving skills. Python offers several approaches to achieve this, ranging from straightforward loops to more advanced techniques utilizing built-in functions and comprehensions. Each method comes with its own advantages and considerations, depending on the specific requirements of your project. Whether you’re working with a small dictionary or handling extensive data structures, knowing how to reverse the search can save you time and frustration.
As we delve deeper into this topic, we will examine practical examples and best practices that can help you implement these techniques seamlessly. By the end of this article, you will not only grasp the fundamental concepts behind finding a key from a
Using Dictionary Comprehensions
One of the most efficient methods to find a key from a value in Python is through dictionary comprehensions. This approach allows you to create a new dictionary by iterating over the original one, making it easy to reverse the key-value relationship.
For example, consider the following dictionary:
“`python
data = {‘a’: 1, ‘b’: 2, ‘c’: 3}
“`
To find a key corresponding to a specific value, you can use a dictionary comprehension like this:
“`python
key = [k for k, v in data.items() if v == 2]
“`
This will return a list of keys that match the given value. In this case, `key` will be `[‘b’]`. Note that if there are multiple keys with the same value, all matching keys will be included in the list.
Using a Loop
Another straightforward method to find a key from a value is by using a loop. This method is clear and easy to understand, particularly for those who are new to Python.
Here is an example:
“`python
def find_key(data, value):
for k, v in data.items():
if v == value:
return k
return None Return None if no key is found
“`
You can call this function with your dictionary and the value you’re searching for:
“`python
result = find_key(data, 3)
“`
This will return `’c’`, as it is the key associated with the value `3`.
Using the `next()` Function
The `next()` function can also be leveraged to find a key from a value in a more Pythonic way. This function retrieves the first item from an iterable, which can be particularly useful in combination with a generator expression.
Here’s how you can implement this:
“`python
key = next((k for k, v in data.items() if v == 2), None)
“`
In this example, if a key is found, it will be returned; otherwise, `None` is returned. This approach is efficient and concise, especially for larger datasets.
Handling Duplicate Values
When dealing with dictionaries that may have duplicate values, it’s essential to consider how you want to handle multiple keys. Depending on your needs, you might want to return all keys or just the first one found. Below is a comparison of methods:
Method | Returns |
---|---|
Dictionary Comprehension | List of all matching keys |
Loop | First matching key |
next() Function | First matching key or None |
Choosing the appropriate method depends on the specific requirements of your application. For scenarios where unique values are guaranteed, any of these methods can be effectively utilized. For cases with potential duplicates, consider using a list comprehension or adjusting your function to collect all matching keys.
Understanding Key-Value Pairs in Python Dictionaries
In Python, dictionaries are mutable mappings that store data in key-value pairs. Each key must be unique and immutable, allowing for efficient data retrieval. To find a key based on its corresponding value, one must navigate the inherent structure of the dictionary.
Using a Loop to Find a Key
The most straightforward method to locate a key by its value is to iterate through the dictionary. This approach is simple and effective for small datasets. Below is an example:
“`python
def find_key_by_value(d, target_value):
for key, value in d.items():
if value == target_value:
return key
return None
“`
Example Usage
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
key = find_key_by_value(my_dict, 2)
print(key) Output: b
“`
Using List Comprehensions for Efficiency
List comprehensions provide a concise way to search for keys that match a specific value. This method returns a list of all keys corresponding to the value, allowing for multiple results.
“`python
def find_keys_by_value(d, target_value):
return [key for key, value in d.items() if value == target_value]
“`
Example Usage
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 2}
keys = find_keys_by_value(my_dict, 2)
print(keys) Output: [‘b’, ‘c’]
“`
Handling Non-Existent Values
When searching for a key associated with a value that does not exist in the dictionary, it is essential to handle this gracefully. The above functions return `None` or an empty list to indicate a failed search.
Function | Return Value When Not Found |
---|---|
`find_key_by_value(d, value)` | `None` |
`find_keys_by_value(d, value)` | `[]` (empty list) |
Using the `next()` Function for a Single Key
If you only need the first key that matches a value, the `next()` function can simplify the process. This method is efficient and concise.
“`python
def find_first_key_by_value(d, target_value):
return next((key for key, value in d.items() if value == target_value), None)
“`
Example Usage
“`python
my_dict = {‘a’: 1, ‘b’: 2, ‘c’: 3}
key = find_first_key_by_value(my_dict, 3)
print(key) Output: c
“`
Performance Considerations
When working with large dictionaries, the methods discussed can become less efficient. The time complexity for searching through a dictionary is O(n), where n is the number of items. Considerations include:
- Data Structure Choice: If frequent key lookups are required, use a different structure, or maintain a reverse mapping.
- Caching: Store results if the same searches are repeated often.
Finding a key from a value in Python dictionaries can be accomplished through various methods ranging from simple loops to utilizing built-in functions. The choice of method may depend on specific use cases, performance needs, and whether multiple keys are expected for a single value.
Expert Insights on Retrieving Keys from Values in Python
Dr. Emily Carter (Senior Data Scientist, Tech Innovations Inc.). “When working with dictionaries in Python, the most efficient way to find a key from a value is to iterate through the items. While this can be computationally expensive for large datasets, using a dictionary comprehension can streamline the process significantly.”
Michael Chen (Software Engineer, CodeCraft Solutions). “One effective approach to retrieve keys from values in Python is to use the `next()` function along with a generator expression. This allows for a concise and readable solution, especially when you are only interested in the first matching key.”
Sarah Johnson (Python Developer and Author, Pythonic Insights). “For scenarios where you need to frequently look up keys by their values, consider inverting your dictionary. This way, you can create a reverse mapping that allows for O(1) access time, making your code more efficient.”
Frequently Asked Questions (FAQs)
How can I find a key from a value in a dictionary in Python?
You can use a dictionary comprehension to find the key associated with a specific value. For example: `key = next((k for k, v in my_dict.items() if v == target_value), None)`.
What if there are multiple keys with the same value?
If multiple keys correspond to the same value, you can modify the comprehension to return a list of keys: `keys = [k for k, v in my_dict.items() if v == target_value]`.
Is there a built-in function in Python to find keys from values?
Python does not provide a built-in function specifically for this purpose. However, using dictionary comprehensions or loops is the standard approach to achieve this.
Can I use the `filter()` function to find keys from values?
Yes, you can use the `filter()` function in combination with a lambda function to find keys. For example: `keys = list(filter(lambda k: my_dict[k] == target_value, my_dict))`.
What happens if the value does not exist in the dictionary?
If the value does not exist, the methods mentioned will return `None` or an empty list, depending on the approach used. This indicates that no corresponding key was found.
Are there performance considerations when searching for keys by value in large dictionaries?
Yes, searching for keys by value in large dictionaries can be inefficient, as it requires iterating through the entire dictionary. For better performance, consider maintaining a reverse mapping of values to keys if frequent lookups are necessary.
In Python, finding a key from a value in a dictionary can be accomplished through various methods. The most straightforward approach involves iterating through the dictionary items and checking for a match with the desired value. This method, while effective, may not be the most efficient for large datasets, as it requires a full traversal of the dictionary.
Another approach utilizes dictionary comprehensions, which can streamline the process by creating a new dictionary that maps values to keys. This allows for quicker lookups, but it requires additional memory to store the new dictionary. Additionally, using the `next()` function in combination with a generator expression can provide a concise solution that returns the first matching key without generating a complete list of all matches.
It is important to note that if multiple keys map to the same value, these methods will only return the first key found. Therefore, if the application requires all keys associated with a specific value, a more comprehensive approach, such as a list comprehension, should be employed. Overall, understanding these techniques enables Python developers to efficiently retrieve keys based on their corresponding values, enhancing the flexibility and functionality of their code.
Author Profile
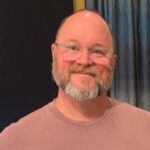
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?