How Can I Run a Cron Job Every Two Days in Spring Boot?
In the fast-paced world of software development, automation is key to enhancing productivity and ensuring reliability. For Java developers utilizing Spring Boot, the ability to schedule tasks efficiently can significantly streamline operations and improve application performance. One common requirement is to run a cron job every two days, a task that can seem daunting at first but is entirely manageable with the right approach. This article will guide you through the intricacies of scheduling such tasks in Spring Boot, empowering you to harness the full potential of automated processes in your applications.
When it comes to scheduling tasks in Spring Boot, the framework offers robust support through its scheduling annotations and configuration options. Understanding how to leverage these features allows developers to create flexible and powerful scheduling solutions tailored to their specific needs. Running a cron job every two days is a practical example that illustrates the broader capabilities of Spring Boot’s scheduling framework, showcasing how you can automate routine tasks with ease.
As we delve deeper into this topic, we will explore the mechanics of cron expressions, the nuances of configuring scheduled tasks in Spring Boot, and best practices for ensuring your jobs run smoothly and efficiently. Whether you’re a seasoned developer or just starting your journey with Spring Boot, this guide will equip you with the knowledge and tools necessary to implement effective scheduling strategies in your applications.
Using Spring Boot’s Scheduling Annotations
In Spring Boot, you can leverage scheduling annotations to execute tasks at specific intervals. The `@Scheduled` annotation enables you to create scheduled tasks effortlessly. To run a cron job every two days, you’ll need to employ the correct cron expression.
Understanding Cron Expressions
Cron expressions are strings that represent a schedule. They consist of six fields: second, minute, hour, day of month, month, and day of week. Each field can contain specific values, including:
- A single number (e.g., `5` for 5 minutes)
- A range (e.g., `1-5` for days 1 to 5)
- A list (e.g., `1,3,5` for specific days)
- An asterisk (`*`) for every possible value
- A question mark (`?`) for “no specific value”
- Special characters like `/` for increments
To run a task every two days, you can use a cron expression like `0 0 0 */2 * ?`. This translates to:
- `0` seconds
- `0` minutes
- `0` hours (midnight)
- `*/2` every two days
- `*` every month
- `?` any day of the week
Configuration in Spring Boot
You must enable scheduling in your Spring Boot application to use the `@Scheduled` annotation. This can be done by adding the `@EnableScheduling` annotation to your main application class.
“`java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
“`
You can then create a scheduled task as follows:
“`java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Component;
@Component
public class MyScheduledTask {
@Scheduled(cron = “0 0 0 */2 * ?”)
public void executeTask() {
// Task implementation here
System.out.println(“Task executed every two days”);
}
}
“`
Task Management Considerations
When implementing scheduled tasks, consider the following best practices:
- Thread Safety: Ensure your task implementation is thread-safe, particularly if it accesses shared resources.
- Error Handling: Incorporate error handling to manage exceptions that may occur during task execution.
- Monitoring: Keep track of scheduled tasks for performance and debugging purposes.
Best Practice | Description |
---|---|
Thread Safety | Use synchronized blocks or other mechanisms to avoid concurrency issues. |
Error Handling | Implement try-catch blocks and logging for exceptions. |
Monitoring | Use tools or frameworks to monitor task execution and performance metrics. |
By following these guidelines and using the appropriate cron expression, you can effectively schedule tasks in your Spring Boot application to run every two days, ensuring that your application performs its necessary background operations efficiently.
Configuring Cron Expression for Every Two Days
In Spring Boot, scheduling tasks can be effectively achieved using the `@Scheduled` annotation. To run a task every two days, a specific cron expression is needed. The cron expression format follows the pattern: `second minute hour dayOfMonth month dayOfWeek`.
For a job that needs to run every two days, you can use the following cron expression:
“`
0 0 0 */2 * *
“`
This expression can be broken down as follows:
- `0` – At second 0
- `0` – At minute 0
- `0` – At hour 0 (midnight)
- `*/2` – Every two days
- `*` – Every month
- `*` – Every day of the week
This setup ensures that the scheduled task will trigger at midnight every second day.
Implementing the Scheduled Task
To implement the task in a Spring Boot application, annotate a method with `@Scheduled` and provide the cron expression as follows:
“`java
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Service;
@Service
public class MyScheduledTask {
@Scheduled(cron = “0 0 0 */2 * *”)
public void executeTask() {
// Task logic here
System.out.println(“Task executed every two days”);
}
}
“`
Ensure that the `@EnableScheduling` annotation is present in your main application class to enable scheduling support:
“`java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.scheduling.annotation.EnableScheduling;
@SpringBootApplication
@EnableScheduling
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
“`
Considerations for Cron Jobs
When setting up cron jobs, consider the following:
- Time Zone: Ensure the application is aware of the time zone settings, as cron jobs are time zone-sensitive. You can specify the time zone in the `@Scheduled` annotation like this:
“`java
@Scheduled(cron = “0 0 0 */2 * *”, zone = “UTC”)
“`
- Overlapping Tasks: If a task takes longer than the scheduled interval, it may overlap. To prevent this, you can control the execution with the `fixedDelay` or `fixedRate` parameters, or implement a locking mechanism.
- Error Handling: Implement error handling within the scheduled task to manage any exceptions that might occur during execution.
Testing the Cron Job
Testing cron jobs can be challenging due to their scheduling nature. To facilitate testing, consider:
- Shortening the Interval: Temporarily change the cron expression to a shorter interval, such as every minute (`0 * * * * *`), during testing.
- Logging: Utilize logging within the task to track execution and errors.
- Unit Tests: Use JUnit and Mockito to create unit tests that validate the scheduled task logic without depending on the actual scheduling.
“`java
import static org.mockito.Mockito.*;
public class MyScheduledTaskTest {
@Test
public void testExecuteTask() {
MyScheduledTask task = new MyScheduledTask();
task.executeTask(); // Invoke the method directly for testing
// Add assertions or verifications as necessary
}
}
“`
These practices help ensure that your scheduled tasks in Spring Boot are robust, reliable, and maintainable.
Strategies for Scheduling Cron Jobs in Spring Boot
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “To effectively run a cron job every two days in Spring Boot, it is essential to utilize the @Scheduled annotation with a fixedRate or fixedDelay configuration. However, for a more precise schedule, leveraging a cron expression like ‘0 0 0 */2 * *’ can ensure that your task executes at midnight every second day.”
Michael Thompson (DevOps Specialist, Cloud Solutions Inc.). “When implementing a cron job in Spring Boot, consider the implications of server time zones and daylight saving changes. Using UTC for scheduling can help maintain consistency across environments, especially when setting up jobs to run every two days.”
Jessica Lin (Spring Framework Contributor, Open Source Advocate). “For running a cron job every two days, it is crucial to test the job’s execution thoroughly. Utilizing Spring’s testing framework can help simulate the cron job’s behavior and ensure that it operates correctly without unintended side effects.”
Frequently Asked Questions (FAQs)
How can I schedule a Cron job to run every two days in Spring Boot?
To schedule a Cron job to run every two days in Spring Boot, you can use the `@Scheduled` annotation with the appropriate Cron expression. The expression `0 0 0 */2 * *` will execute the task at midnight every two days.
What does the Cron expression `0 0 0 */2 * *` mean?
The Cron expression `0 0 0 */2 * *` specifies that the task will run at 00:00 (midnight) every two days. The `*/2` in the day-of-month field indicates that the task should trigger on every second day.
Can I run multiple Cron jobs in Spring Boot?
Yes, you can run multiple Cron jobs in Spring Boot by defining multiple methods annotated with `@Scheduled` in your service class, each with its own unique Cron expression.
How do I enable scheduling in a Spring Boot application?
To enable scheduling in a Spring Boot application, you need to add the `@EnableScheduling` annotation to one of your configuration classes. This allows Spring to detect and execute scheduled tasks.
What should I do if my Cron job is not executing as expected?
If your Cron job is not executing as expected, check the following: ensure that scheduling is enabled, verify the Cron expression for correctness, and check the application logs for any errors or exceptions that may indicate issues with the scheduled task.
Is there a way to dynamically change the Cron expression at runtime?
Yes, you can dynamically change the Cron expression at runtime by using a `ScheduledTaskRegistrar` and implementing a custom scheduling configuration. This allows you to modify the Cron expression based on application logic or external configuration.
In summary, scheduling a cron job to run every two days in a Spring Boot application can be effectively achieved using the `@Scheduled` annotation. This annotation allows developers to define the frequency of task execution using a cron expression. A cron expression for running a task every two days can be specified as “0 0 0 */2 * *”, which indicates that the task will execute at midnight every two days.
Implementing this functionality not only streamlines background processing but also enhances the application’s ability to manage periodic tasks without manual intervention. The integration of Spring’s scheduling capabilities simplifies the management of such tasks, allowing developers to focus on core business logic while ensuring that necessary operations are performed consistently.
Furthermore, it is essential to consider the implications of running tasks at regular intervals, such as potential resource consumption and the need for monitoring task execution. Proper logging and error handling mechanisms should be in place to ensure that any issues can be promptly addressed. Overall, leveraging Spring Boot’s scheduling features provides a robust solution for automating tasks effectively.
Author Profile
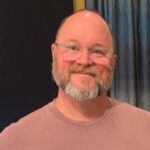
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?