How Can You Use JavaScript to Effectively Remove Onload Webpage Popups?
In the fast-paced world of web development, user experience reigns supreme. One of the most disruptive elements for visitors is the dreaded onload popup. These intrusive windows can interrupt the flow of information, detract from the overall aesthetic of a webpage, and often lead to frustration. As developers, it’s crucial to strike a balance between engaging users and respecting their browsing experience. In this article, we will explore effective JavaScript techniques to remove onload popups from your webpages, ensuring a smoother, more enjoyable interaction for your audience.
Overview
Onload popups have long been a staple for many websites, often used for promotions, subscriptions, or important announcements. However, their effectiveness is increasingly questioned as users grow weary of unsolicited interruptions. Understanding how to manage these popups is essential for developers who want to create a more seamless browsing experience. By leveraging JavaScript, you can implement strategies to either eliminate these popups entirely or control their behavior, allowing for a more user-friendly approach.
In the following sections, we will delve into various methods and best practices for removing onload popups using JavaScript. We will cover the underlying principles of event handling, the importance of user engagement, and the potential impact on your site’s performance and SEO. Whether you’re looking to
Understanding Onload Popups
Onload popups are typically triggered when a webpage finishes loading. These popups can be used for various purposes, including advertisements, user notifications, or prompts to sign up for newsletters. However, they can also hinder user experience by interrupting navigation or obscuring content. Understanding how these popups function is essential for effective removal or modification.
Common Causes of Onload Popups
Onload popups can arise from various sources:
- JavaScript Functions: Often, popups are generated by JavaScript functions that are executed when the window’s load event occurs.
- Third-party Libraries: Many websites use third-party libraries or plugins that include popup features by default.
- Inline HTML Attributes: Developers may use inline `onload` attributes in HTML to trigger popups directly when the page loads.
Removing Onload Popups with JavaScript
To effectively remove or prevent onload popups, one can manipulate the JavaScript code responsible for triggering them. Below are several strategies to achieve this.
Disabling Popup Functions
You can override the popup function defined in the JavaScript code. For example:
“`javascript
window.onload = function() {
// Disable the popup
if (typeof originalPopupFunction === ‘function’) {
originalPopupFunction = function() {};
}
};
“`
This snippet checks if the original popup function exists and reassigns it to an empty function, effectively disabling it.
Using a Mutation Observer
A more robust solution involves using a Mutation Observer to monitor the DOM for any changes that may introduce a popup. Here’s a basic implementation:
“`javascript
const observer = new MutationObserver((mutations) => {
mutations.forEach((mutation) => {
if (mutation.type === ‘childList’) {
const popups = document.querySelectorAll(‘.popup-class’); // Adjust selector as needed
popups.forEach((popup) => popup.remove());
}
});
});
observer.observe(document.body, { childList: true, subtree: true });
“`
This code will actively monitor the body for any added elements and remove them if they match the specified popup class.
Preventing Future Popups
To ensure that popups do not reappear, you can implement several best practices within your JavaScript code:
- Use Event Listeners: Attach event listeners that prevent default behavior for known popup triggers.
- CSS Display Property: Set the CSS display property of popups to `none` on page load.
- Content Security Policy (CSP): Implement CSP headers to restrict the sources of scripts that can run on your site.
Example Table: Popup Management Techniques
Technique | Description | Use Case |
---|---|---|
Function Override | Reassigns popup functions to prevent execution. | Ideal for simple popups controlled by known functions. |
Mutation Observer | Monitors DOM changes and removes popups dynamically. | Useful for popups added by third-party scripts. |
CSS Manipulation | Sets display properties to hide unwanted elements. | Good for consistent styling and visual control. |
CSP Headers | Restricts script sources to enhance security. | Best practice for preventing unauthorized scripts. |
By employing these techniques, developers can effectively manage and remove unwanted onload popups, thereby enhancing user experience and maintaining control over webpage behavior.
Understanding Onload Popups
Onload popups are often implemented using JavaScript to create interactive experiences. However, they can disrupt user experience if not managed properly. These popups can be triggered when a webpage fully loads, which may lead to unwanted interruptions for users.
Common Methods of Triggering Onload Popups
Several methods can be employed to create onload popups, including:
- JavaScript Alerts: Simple alert boxes that notify users.
- Modal Dialogs: More complex popups that overlay content on the page.
- Custom HTML/CSS Popups: Fully styled elements that can be controlled through JavaScript.
Removing Onload Popups Using JavaScript
To effectively remove onload popups, you can implement the following JavaScript strategies:
“`javascript
window.onload = function() {
// Find the popup element
var popup = document.getElementById(‘popupElementId’);
if (popup) {
popup.style.display = ‘none’; // Hides the popup
}
};
“`
This snippet will execute once the window has fully loaded, searching for a popup element by its ID and setting its display property to ‘none’, effectively removing it from view.
Preventing Onload Popups from Displaying
To prevent onload popups from appearing altogether, you can modify the JavaScript code responsible for their display. Here are common techniques:
- Comment Out Popup Code: Locate the code responsible for the popup and comment it out or remove it.
“`javascript
// function showPopup() {
// // Popup logic here
// }
“`
- Use Flags to Control Popup Display: Implement a flag that determines whether the popup should display based on user interactions.
“`javascript
var showPopup = ; // Set to to prevent popup
if (showPopup) {
// Show popup logic
}
“`
CSS Solutions for Hiding Popups
CSS can also be utilized to hide popups by setting specific styles. This can be a quick fix if you cannot modify JavaScript.
“`css
popupElementId {
display: none; /* Hides the popup */
}
“`
This method will effectively prevent the popup from appearing visually but may still exist in the DOM.
Testing and Validation
After implementing changes to remove or prevent onload popups, thorough testing is essential. Consider the following methods:
- Browser Developer Tools: Use tools such as the console and inspector to verify that the popup element is not being displayed.
- User Testing: Gather feedback from users to ensure that their experience is unaffected by the changes.
Conclusion on Managing Onload Popups
Effective management of onload popups requires a blend of JavaScript and CSS strategies. By understanding how these popups operate and implementing solutions to mitigate their impact, you can significantly enhance user experience on your website.
Expert Insights on Removing Onload Webpage Popups in JavaScript
Jessica Tran (Web Development Specialist, CodeCraft Inc.). “To effectively remove onload popups in JavaScript, developers should consider utilizing event listeners that can intercept and prevent default actions. This approach not only enhances user experience but also maintains the integrity of the webpage’s functionality.”
Michael Chen (User Experience Researcher, UX Innovations). “From a user experience perspective, it is crucial to minimize intrusive popups that appear on page load. Implementing a delay or using user-triggered events for displaying popups can significantly improve engagement and reduce bounce rates.”
Sarah Patel (Frontend Engineer, Tech Solutions Group). “Utilizing JavaScript’s `removeEventListener` method can be an effective strategy for managing onload popups. By removing the event listener after the popup is displayed, developers can prevent it from reappearing unnecessarily, thus streamlining the user journey.”
Frequently Asked Questions (FAQs)
What are webpage popups triggered by onload events?
Webpage popups triggered by onload events are modal windows or alerts that appear automatically when a webpage finishes loading. They are often used for advertisements, notifications, or user prompts.
How can I remove onload popups using JavaScript?
To remove onload popups using JavaScript, you can modify the onload event handler of the window object. You can either prevent the popup from displaying by commenting out or removing the code that triggers it or by overriding the onload function to include your custom logic.
Are there any browser extensions that can help block onload popups?
Yes, there are several browser extensions available that can block or manage popups, such as AdBlock Plus, uBlock Origin, and Popup Blocker. These tools can help prevent unwanted popups from appearing on webpages.
Can I disable all popups on a specific website?
Yes, you can disable all popups on a specific website by adjusting your browser settings. Most modern browsers allow users to block popups on a site-by-site basis, ensuring a more controlled browsing experience.
What are the potential drawbacks of removing onload popups?
Removing onload popups can lead to missing important notifications or updates from the website. Additionally, some websites may rely on these popups for essential functionality, and disabling them may affect user experience.
Is it possible to programmatically close an onload popup?
Yes, it is possible to programmatically close an onload popup using JavaScript. You can target the popup window using its reference and call the `close()` method, provided that the popup was opened by a script and not blocked by browser settings.
In summary, removing onload webpage popups using JavaScript involves understanding the structure of the webpage and the specific methods employed to trigger these popups. Developers can utilize various techniques, such as modifying the DOM, disabling event listeners, or overriding functions that create popups. These methods can enhance user experience by eliminating intrusive popups that may disrupt navigation or engagement with the content.
Furthermore, it is essential to consider the ethical implications of removing popups. While it can improve usability, developers must balance this with the need for legitimate notifications and advertisements that may provide value to users. Therefore, implementing a solution that respects user preferences and promotes a positive browsing experience is crucial.
Key takeaways include the importance of thorough testing after implementing changes to ensure that the removal of popups does not inadvertently affect other functionalities of the website. Additionally, developers should remain updated on best practices and evolving standards in web development to effectively manage popups while maintaining compliance with user consent regulations.
Author Profile
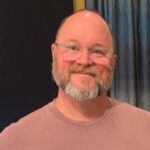
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?