How Can You Efficiently Use SQL to Update Multiple Columns in a Single Query?
In the world of databases, SQL (Structured Query Language) stands as the backbone for managing and manipulating data. One of the most powerful features of SQL is its ability to update multiple columns in a single command, streamlining the process of data management and enhancing efficiency. Whether you’re a seasoned database administrator or a budding developer, mastering the art of updating multiple columns can significantly elevate your SQL skills and optimize your workflow. In this article, we will delve into the intricacies of crafting effective SQL update statements, empowering you to handle complex data scenarios with ease.
When it comes to updating records in a database, the ability to modify several columns simultaneously can save time and reduce the risk of errors. This feature is particularly useful in scenarios where related data needs to be synchronized or corrected across multiple fields. Understanding the syntax and structure of SQL update commands not only simplifies the coding process but also ensures that your database remains consistent and accurate.
As we explore this essential aspect of SQL, we will cover various techniques and best practices for updating multiple columns efficiently. From the foundational principles to advanced strategies, you’ll gain insights that will enhance your database management capabilities. Get ready to unlock the potential of SQL and transform the way you interact with your data!
Understanding SQL Update Syntax
To update multiple columns in a SQL database, it is essential to understand the basic syntax used in the `UPDATE` statement. The general structure of the SQL `UPDATE` command allows you to modify existing records in a table. The syntax for updating multiple columns can be defined as follows:
“`sql
UPDATE table_name
SET column1 = value1, column2 = value2, …
WHERE condition;
“`
Here, `table_name` refers to the table that contains the records you want to update. The `SET` clause specifies the columns to be updated and their new values. The `WHERE` clause is crucial, as it determines which records will be updated. Omitting the `WHERE` clause will result in all records being updated, which is often not the desired outcome.
Example of Updating Multiple Columns
Consider a scenario where you have an employee table named `employees` with the following structure:
employee_id | first_name | last_name | job_title | salary |
---|---|---|---|---|
1 | John | Doe | Developer | 60000 |
2 | Jane | Smith | Designer | 65000 |
3 | Mike | Johnson | Manager | 70000 |
To update both the `job_title` and `salary` for the employee with `employee_id` 1, you would execute the following SQL command:
“`sql
UPDATE employees
SET job_title = ‘Senior Developer’, salary = 75000
WHERE employee_id = 1;
“`
After executing this command, the `employees` table would be modified as follows:
employee_id | first_name | last_name | job_title | salary |
---|---|---|---|---|
1 | John | Doe | Senior Developer | 75000 |
2 | Jane | Smith | Designer | 65000 |
3 | Mike | Johnson | Manager | 70000 |
Best Practices for Updating Multiple Columns
When updating multiple columns, consider the following best practices to ensure data integrity and performance:
- Use Transactions: If updating multiple records or performing complex operations, wrap your updates in a transaction to maintain data consistency.
- Backup Data: Always back up your data before performing updates, especially if you are modifying critical information.
- Limit with WHERE Clause: Ensure that your `WHERE` clause is specific enough to avoid unintentional updates to multiple records.
- Test Changes: If possible, test your updates on a small subset of data before applying them to the entire table.
Common Errors to Avoid
While using the `UPDATE` statement, be mindful of these common errors:
Error Type | Description |
---|---|
Missing WHERE Clause | Updates all records if omitted, leading to data loss. |
Incorrect Data Types | Assigning values that do not match the column data type can cause errors. |
Syntax Errors | Typos in SQL commands can prevent successful execution. |
By adhering to these guidelines and understanding the SQL `UPDATE` syntax, you can effectively manage and update multiple columns in your database tables, ensuring both accuracy and reliability in your data management practices.
Understanding SQL Update Syntax
The SQL `UPDATE` statement is essential for modifying existing records in a database. To update multiple columns within a single command, the syntax must be carefully structured to ensure clarity and accuracy.
Basic Syntax:
“`sql
UPDATE table_name
SET column1 = value1,
column2 = value2,
column3 = value3
WHERE condition;
“`
- table_name: The name of the table containing the records to be updated.
- column1, column2, column3: The columns that need to be updated.
- value1, value2, value3: The new values to assign to the respective columns.
- condition: A filter that determines which records should be updated; if omitted, all records in the table will be affected.
Example of Updating Multiple Columns
Consider a scenario where you have a table called `employees` and you need to update both the `salary` and `department` of an employee identified by their `employee_id`.
SQL Query Example:
“`sql
UPDATE employees
SET salary = 75000,
department = ‘Sales’
WHERE employee_id = 101;
“`
This command updates the `salary` to 75,000 and changes the `department` to ‘Sales’ for the employee with an `employee_id` of 101.
Using the WHERE Clause Effectively
The `WHERE` clause is crucial to avoid unintentional updates. When updating multiple columns, ensure the condition is specific enough to target the desired records.
Common Patterns:
- Update based on a single condition:
“`sql
WHERE condition1;
“`
- Update based on multiple conditions using AND/OR:
“`sql
WHERE condition1 AND condition2;
WHERE condition1 OR condition2;
“`
Example:
“`sql
UPDATE employees
SET salary = 80000,
department = ‘Marketing’
WHERE employee_id = 102 AND status = ‘active’;
“`
This ensures that only active employees with `employee_id` 102 will have their records updated.
Handling NULL Values
When updating columns, you may need to set values to `NULL`. This is particularly useful for resetting fields or clearing outdated information.
Example:
“`sql
UPDATE employees
SET department = NULL
WHERE employee_id = 103;
“`
This command sets the `department` to `NULL` for the employee with `employee_id` 103.
Batch Updates with Subqueries
You can also perform batch updates using subqueries. This approach allows you to update multiple records based on the results of another query.
Example of a Batch Update:
“`sql
UPDATE employees
SET salary = (SELECT AVG(salary) FROM employees WHERE department = ‘Sales’)
WHERE department = ‘Marketing’;
“`
Here, all employees in the ‘Marketing’ department will have their salaries updated to the average salary of the ‘Sales’ department.
Best Practices for Updating Multiple Columns
- Always back up data before performing bulk updates.
- Test your queries in a safe environment before executing them in production.
- Use transactions when updating multiple rows to ensure data integrity.
- Consider performance implications with large datasets; proper indexing can help.
By following these guidelines, you can effectively manage and update multiple columns in your SQL databases while minimizing risks associated with data manipulation.
Expert Insights on SQL to Update Multiple Columns
“Maria Chen (Database Administrator, Tech Solutions Inc.). Utilizing SQL to update multiple columns efficiently is crucial for maintaining data integrity and performance. It is essential to ensure that the update statement is well-structured to avoid unintended data changes, especially in large datasets.”
“James Patel (Senior Data Analyst, Data Insights Group). When updating multiple columns in SQL, leveraging transactions can be beneficial. This practice not only allows for rollback in case of errors but also ensures that all changes are applied consistently, which is vital for accurate reporting.”
“Linda Gomez (SQL Developer, Innovative Tech Solutions). It is important to use the correct syntax when updating multiple columns in SQL. Using the SET clause properly can enhance readability and maintainability of the code, making it easier for teams to collaborate on database management.”
Frequently Asked Questions (FAQs)
What is the basic syntax for updating multiple columns in SQL?
The basic syntax for updating multiple columns in SQL is:
“`sql
UPDATE table_name
SET column1 = value1, column2 = value2, …
WHERE condition;
“`
Can I use the UPDATE statement to modify all rows in a table?
Yes, you can modify all rows by omitting the WHERE clause. However, this will apply the changes to every row in the table, which may not be desirable.
Is it possible to update multiple columns with different conditions?
Yes, you can use a CASE statement within the SET clause to apply different conditions for each column. This allows for more granular control over the updates.
What happens if I forget to include a WHERE clause in my update?
If you forget to include a WHERE clause, all records in the table will be updated with the specified values, which can lead to unintended data loss or corruption.
Can I update multiple columns in different tables in a single SQL statement?
No, a single SQL UPDATE statement can only modify one table at a time. To update multiple tables, you need to execute separate UPDATE statements for each table.
Are there any performance considerations when updating multiple columns?
Yes, updating multiple columns can impact performance, especially on large tables. It is advisable to ensure that the WHERE clause is as specific as possible to minimize the number of rows affected.
In summary, updating multiple columns in SQL is a fundamental operation that allows database administrators and developers to efficiently modify records within a table. The SQL syntax for updating multiple columns typically involves the `UPDATE` statement, followed by the `SET` clause, where each column to be updated is specified along with its new value. Properly structuring this command is essential to ensure that the intended records are accurately modified without affecting unintended data.
One of the key insights when working with SQL updates is the importance of using the `WHERE` clause. This clause serves as a filter to specify which records should be updated, thereby preventing unintended changes to the entire dataset. Without a `WHERE` clause, all rows in the table would be updated, which could lead to significant data integrity issues. Therefore, always double-check the conditions set in the `WHERE` clause before executing an update operation.
Additionally, it is advisable to perform a backup of the data or use transactions when executing updates on multiple columns, especially in production environments. This precaution allows for recovery in case of errors or unintended consequences. Testing the update command on a smaller dataset or in a development environment can also help identify potential issues before applying changes to the live database.
Overall
Author Profile
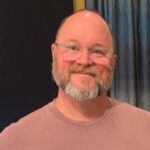
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?