What Are the Scope and Lifetime of Variables in Python?
In the world of programming, understanding the nuances of variable management is crucial for writing efficient and effective code. One of the fundamental concepts that every Python developer must grasp is the scope and lifetime of a variable. These two elements dictate how and when variables can be accessed and modified within a program, influencing everything from memory management to code readability. Whether you’re a novice coder or a seasoned developer, mastering these concepts will empower you to create cleaner, more reliable applications.
At its core, the scope of a variable refers to the context in which it is defined and can be accessed. In Python, variables can have different scopes depending on where they are declared—be it within a function, a class, or at the module level. This distinction is essential for managing variable visibility and avoiding potential conflicts in larger codebases. On the other hand, the lifetime of a variable pertains to the duration for which a variable exists in memory. Understanding how long a variable remains accessible after its creation can help developers optimize resource usage and prevent memory leaks.
As we delve deeper into the intricacies of variable scope and lifetime in Python, we will explore the various types of scopes, including local, global, and nonlocal, and how they interact with each other. We will also examine the implications of variable lifetime
Scope of a Variable
The scope of a variable in Python defines the region of the program where the variable is accessible. It determines the visibility of the variable and is governed by the location of the variable’s declaration. In Python, there are four primary types of scopes:
- Local Scope: Variables defined within a function are local to that function. They cannot be accessed outside of it.
- Enclosing Scope: This applies to nested functions. The inner function can access variables from the outer function.
- Global Scope: Variables declared at the top level of a script or module have global scope and can be accessed from anywhere within that module.
- Built-in Scope: This includes names that are pre-defined in Python, such as keywords and built-in functions (e.g., `print()`, `len()`).
The scope hierarchy can be summarized in the following table:
Scope Type | Accessibility |
---|---|
Local | Accessible only within the function where it is defined |
Enclosing | Accessible within nested functions |
Global | Accessible throughout the module |
Built-in | Accessible from any part of the program |
Understanding scope is crucial for avoiding variable name conflicts and ensuring that functions operate on the intended data.
Lifetime of a Variable
The lifetime of a variable refers to the duration for which the variable exists in memory during the execution of a program. In Python, the lifetime is closely related to its scope and can be categorized as follows:
- Local Variables: The lifetime of a local variable is limited to the execution of the function in which it is defined. Once the function exits, the variable is destroyed.
- Global Variables: Global variables exist for the duration of the program. They can be accessed and modified by any function that declares them as global.
- Temporary Variables: These are typically created during the execution of expressions or loops and are deleted once the execution context is exited.
The timing of variable creation and destruction can be summarized in the following points:
- Local variables are created when a function starts and destroyed when it ends.
- Global variables are created when the script starts and remain until the program terminates.
- Variables within a loop or a conditional block are created anew in each iteration or execution and are discarded afterward.
Understanding both scope and lifetime is essential for effective memory management and avoiding unintended side effects in Python programming.
Scope of a Variable in Python
The scope of a variable refers to the region of the program where the variable is accessible. In Python, variable scope can be classified into four main categories: local, enclosing, global, and built-in.
- Local Scope: Variables declared within a function have local scope. They are only accessible within that function.
“`python
def my_function():
local_var = 10
print(local_var) Accessible here
my_function()
print(local_var) Raises NameError
“`
- Enclosing Scope: This refers to the scope of variables in nested functions. If a function is defined inside another function, the inner function can access variables from the outer function’s scope.
“`python
def outer_function():
enclosing_var = 20
def inner_function():
print(enclosing_var) Accessible here
inner_function()
outer_function()
“`
- Global Scope: Variables defined at the top level of a script or module have global scope and can be accessed from any function within the same module.
“`python
global_var = 30
def my_function():
print(global_var) Accessible here
my_function()
“`
- Built-in Scope: This includes names that are pre-defined in Python, such as `print()` and `len()`. These names are available in any Python script.
Lifetime of a Variable in Python
The lifetime of a variable refers to the duration for which the variable exists in memory. In Python, the lifetime is closely tied to the scope.
- Local Variables: The lifetime of local variables begins when the function is called and ends when the function exits. They are created when the function starts executing and destroyed when it finishes.
- Enclosing Variables: The lifetime of enclosing variables persists as long as the outer function is executing. Once the outer function completes, the inner function can no longer access those variables.
- Global Variables: The lifetime of global variables spans the entire runtime of the program. They are created when the module is loaded and remain until the program exits.
- Built-in Variables: Built-in names exist for the duration of the Python session and are always available.
Variable Type | Scope | Lifetime |
---|---|---|
Local | Local | Exists only during function call |
Enclosing | Enclosing | Exists during outer function call |
Global | Global | Exists throughout program runtime |
Built-in | Built-in | Exists for the duration of session |
Understanding the scope and lifetime of variables is crucial for managing memory effectively and avoiding unintended side effects in your code. Properly managing these aspects helps maintain clean, efficient, and functional code in Python.
Understanding Variable Scope and Lifetime in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “The scope of a variable in Python determines where that variable can be accessed within the code. It is crucial for developers to understand the difference between local and global scope to avoid unintended side effects in their programs.”
Michael Chen (Lead Instructor, Python Programming Academy). “The lifetime of a variable refers to the duration for which the variable exists in memory. In Python, a variable’s lifetime is tied to its scope, and understanding this relationship is essential for effective memory management and debugging.”
Sarah Thompson (Technical Writer, Code Insights Magazine). “In Python, variables defined within a function have local scope and are destroyed once the function exits, while global variables persist throughout the program’s execution. This distinction is fundamental for writing clean and maintainable code.”
Frequently Asked Questions (FAQs)
What is the scope of a variable in Python?
The scope of a variable in Python refers to the region of the program where the variable is accessible. Variables can have local scope, where they are only accessible within the function or block they are defined, or global scope, where they can be accessed throughout the entire module.
What is the lifetime of a variable in Python?
The lifetime of a variable in Python refers to the duration for which the variable exists in memory. A variable’s lifetime begins when it is created and ends when it is deleted or goes out of scope, typically when the function or block in which it was defined finishes execution.
How does local scope affect variable lifetime?
In Python, a variable defined within a function has local scope and its lifetime is limited to the duration of that function’s execution. Once the function completes, the variable is destroyed and cannot be accessed outside of that function.
Can a variable have both local and global scope in Python?
Yes, a variable can have both local and global scope. If a variable is defined globally and is redefined within a function, the function will use the local version, while the global version remains accessible outside the function unless explicitly declared as global within the function.
What are the rules for variable scope in nested functions?
In nested functions, the inner function can access variables from its own local scope, the enclosing function’s scope, and the global scope. However, it cannot modify variables in the enclosing function’s scope unless they are declared as nonlocal.
How can I check the scope of a variable in Python?
You can check the scope of a variable in Python using the `locals()` and `globals()` functions. `locals()` returns a dictionary of the current local symbol table, while `globals()` provides a dictionary of the global symbol table, allowing you to inspect the variables and their scopes.
In Python, the scope of a variable refers to the region of the program where the variable is accessible. Variables can have different scopes, including local, enclosing, global, and built-in. Local variables are defined within a function and can only be accessed inside that function. Enclosing variables are found in nested functions, while global variables are defined at the top level of a module and can be accessed throughout the module. Built-in variables are predefined in Python and can be accessed anywhere in the code.
The lifetime of a variable, on the other hand, pertains to the duration for which the variable exists in memory during the execution of a program. Local variables are created when a function is called and are destroyed once the function exits, leading to a short lifetime. Global variables, however, persist for the entire duration of the program, remaining in memory until the program terminates. Understanding both scope and lifetime is crucial for effective memory management and avoiding potential errors in code.
Key takeaways from the discussion on variable scope and lifetime in Python include the importance of using the correct variable scope to prevent naming conflicts and ensure code clarity. Additionally, awareness of variable lifetime can help developers optimize performance by managing memory usage more effectively. By mastering these concepts, programmers
Author Profile
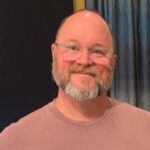
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?