How Can You Effectively Use a Case Statement in a Where Clause?
In the world of SQL, the ability to manipulate and filter data effectively is paramount for any data analyst or database administrator. One powerful tool at your disposal is the case statement, which allows for conditional logic within your queries. While most users are familiar with its application in the SELECT clause, the potential of incorporating a case statement in the WHERE clause opens up a new realm of possibilities. This technique not only enhances the flexibility of your queries but also enables more nuanced data retrieval based on complex conditions.
Overview
Using a case statement within the WHERE clause can transform the way you approach data filtering. By allowing for multiple conditions to be evaluated, it empowers you to create dynamic queries that adapt to varying scenarios. This is particularly useful in situations where you need to filter records based on different criteria without resorting to cumbersome multiple OR statements.
Moreover, the integration of a case statement in the WHERE clause can lead to more readable and maintainable SQL code. Instead of cluttering your queries with nested conditions or repetitive logic, you can consolidate your filtering criteria into a single, coherent structure. This not only streamlines your code but also enhances its clarity, making it easier for others (or your future self) to understand the logic behind your data selection. As we delve deeper
Understanding the Case Statement
The CASE statement is a powerful tool in SQL that allows for conditional logic within queries. It enables the user to perform different actions based on varying conditions, effectively allowing for branching logic similar to if-then-else statements found in programming languages. The syntax of a CASE statement can be structured in two primary ways: simple and searched.
- Simple CASE Statement: Evaluates a single expression against multiple values.
- Searched CASE Statement: Evaluates multiple conditions that can be true or .
An example of a searched CASE statement is illustrated below:
“`sql
SELECT
employee_id,
salary,
CASE
WHEN salary < 30000 THEN 'Low'
WHEN salary BETWEEN 30000 AND 60000 THEN 'Medium'
ELSE 'High'
END AS salary_category
FROM employees;
```
In the example above, the CASE statement categorizes employee salaries into three groups based on their value.
Using CASE in the WHERE Clause
In SQL, the WHERE clause is essential for filtering records. Incorporating a CASE statement within the WHERE clause allows for dynamic filtering based on specific conditions. This feature can significantly enhance query flexibility and readability.
For instance, consider a scenario where you need to filter employee records based on different criteria depending on the department. The following SQL demonstrates this approach:
“`sql
SELECT *
FROM employees
WHERE department_id =
CASE
WHEN job_title = ‘Manager’ THEN 1
WHEN job_title = ‘Staff’ THEN 2
ELSE 3
END;
“`
In this case, the query filters employees based on their job title, assigning department IDs dynamically.
Examples of CASE in WHERE Clauses
To further elaborate on the use of CASE statements in WHERE clauses, here are some practical examples:
– **Example 1: Dynamic Filtering**
This example filters products based on their availability status:
“`sql
SELECT product_id, product_name
FROM products
WHERE availability =
CASE
WHEN stock_quantity > 0 THEN ‘In Stock’
WHEN stock_quantity = 0 THEN ‘Out of Stock’
ELSE ‘Discontinued’
END;
“`
- Example 2: Conditional Date Filtering
The following query selects orders based on the status of the order:
“`sql
SELECT order_id, order_date
FROM orders
WHERE order_status =
CASE
WHEN order_date < '2023-01-01' THEN 'Old'
ELSE 'Recent'
END;
```
Advantages of Using CASE in WHERE Clauses
Utilizing a CASE statement in the WHERE clause provides several advantages:
- Increased Readability: Makes complex filtering conditions clearer and easier to understand.
- Dynamic Filtering: Allows for flexible condition evaluations, adapting to various scenarios without multiple queries.
- Reduced Complexity: Minimizes the need for nested queries or multiple conditions in WHERE clauses.
Considerations When Using CASE in WHERE Clauses
While the CASE statement is a robust feature, there are considerations to keep in mind:
- Performance: Complex CASE statements can impact query performance, especially on large datasets.
- Database Compatibility: Not all SQL databases handle CASE statements identically; always refer to the specific SQL dialect documentation.
Best Practices
To effectively implement CASE statements in WHERE clauses, consider the following best practices:
- Keep conditions simple and straightforward to avoid confusion.
- Limit the number of CASE conditions to enhance performance.
- Test queries for performance impacts, especially on large datasets.
Best Practice | Description |
---|---|
Simplicity | Avoid overly complex conditions in CASE statements. |
Testing | Always test the performance of queries that utilize CASE in WHERE. |
Documentation | Refer to the specific SQL dialect documentation for compatibility. |
Understanding the Case Statement in SQL
The CASE statement in SQL provides a way to implement conditional logic within your queries. While it is typically utilized in the SELECT clause for transforming data, it can also be effectively applied within the WHERE clause to filter records based on specific conditions.
Using CASE in the WHERE Clause
When using a CASE statement in the WHERE clause, it allows for more dynamic filtering. This is particularly useful when you need to evaluate multiple conditions to determine which records to return.
Syntax of CASE in WHERE Clause:
“`sql
SELECT column1, column2
FROM table_name
WHERE condition1 AND
(CASE
WHEN condition_a THEN result1
WHEN condition_b THEN result2
ELSE default_result
END) = some_value;
“`
Example of CASE in WHERE Clause:
Consider a sales database where you want to filter orders based on the status of the order. The SQL query could look like this:
“`sql
SELECT order_id, customer_id, order_status
FROM orders
WHERE (CASE
WHEN order_status = ‘Shipped’ THEN 1
WHEN order_status = ‘Pending’ THEN 2
ELSE 3
END) = 1;
“`
In this example, only orders that are ‘Shipped’ will be selected.
Practical Scenarios for CASE in WHERE Clause
There are various scenarios where applying a CASE statement in the WHERE clause is beneficial:
- Dynamic Filtering: Adjusting filter criteria based on user input or other variables.
- Complex Business Logic: Implementing multi-condition filtering that is not straightforward with simple AND/OR logic.
- Conditional Aggregation: Using in conjunction with aggregate functions to filter based on results of calculations.
Performance Considerations
Using a CASE statement in the WHERE clause can have implications on query performance.
Key Points:
- Index Utilization: If the CASE statement leads to a full table scan, it may negate the benefits of existing indexes.
- Complexity: Overly complex CASE statements can slow down query execution time.
- Testing: Always test performance impacts through execution plans to ensure efficiency.
Alternative Approaches
In some cases, there may be simpler alternatives to using a CASE statement in the WHERE clause:
- Simple WHERE Conditions: Using straightforward AND/OR conditions can often suffice.
- Subqueries: For complex logic, consider using subqueries that may simplify the overall query structure.
- Common Table Expressions (CTEs): CTEs can enhance readability and maintainability while providing similar functionality.
Example of Alternative Approach:
“`sql
SELECT order_id, customer_id, order_status
FROM orders
WHERE order_status = ‘Shipped’;
“`
This alternative is more readable and may perform better than a CASE statement for simple conditions.
Conclusion on CASE in WHERE Clause
The CASE statement in the WHERE clause is a powerful tool for implementing conditional logic in SQL queries. By understanding its syntax, practical applications, performance implications, and alternatives, SQL practitioners can effectively leverage this feature to meet complex querying needs.
Expert Insights on Using Case Statements in Where Clauses
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “Incorporating a CASE statement within a WHERE clause can significantly enhance query flexibility. It allows for conditional logic directly in filtering criteria, which can simplify complex queries and improve readability.”
James Liu (Senior Data Analyst, Insight Analytics Group). “Using a CASE statement in a WHERE clause is particularly beneficial when dealing with multiple conditions that need to be evaluated dynamically. This approach can reduce the need for multiple queries and optimize performance.”
Maria Gonzalez (SQL Consultant, Data Solutions Firm). “While a CASE statement can provide powerful conditional logic, it is crucial to use it judiciously within WHERE clauses. Overusing it can lead to complex queries that are hard to maintain and may impact performance negatively.”
Frequently Asked Questions (FAQs)
What is a CASE statement in SQL?
A CASE statement in SQL is a conditional expression that allows you to execute different expressions based on specific conditions. It can be used in SELECT, UPDATE, and WHERE clauses to provide dynamic results based on the data.
Can a CASE statement be used in a WHERE clause?
Yes, a CASE statement can be used in a WHERE clause to determine which rows to include in the result set based on conditional logic. It evaluates conditions and returns a value that can be compared to filter records.
What is the syntax for using a CASE statement in a WHERE clause?
The syntax involves using the CASE statement to evaluate conditions and returning a value. For example:
“`sql
WHERE column_name = CASE
WHEN condition1 THEN value1
WHEN condition2 THEN value2
ELSE default_value
END;
“`
Are there any performance considerations when using CASE statements in WHERE clauses?
Yes, using CASE statements can impact performance, especially if they involve complex calculations or are applied to large datasets. It is advisable to evaluate the execution plan and optimize queries as needed.
Can I use multiple conditions in a CASE statement within a WHERE clause?
Yes, you can include multiple conditions in a CASE statement within a WHERE clause. Each condition can lead to different outcomes, allowing for complex filtering based on various criteria.
What are some common use cases for CASE statements in WHERE clauses?
Common use cases include filtering records based on user roles, status codes, or any scenario where dynamic conditions determine which records to retrieve. This enhances query flexibility and allows for tailored data retrieval.
The use of a CASE statement in a WHERE clause is a powerful technique in SQL that allows for conditional logic within queries. By incorporating a CASE statement, developers can create more dynamic and flexible filtering criteria. This capability enables the execution of complex queries that can adapt based on varying conditions, ultimately leading to more efficient data retrieval and analysis.
One of the key advantages of using a CASE statement in the WHERE clause is the ability to simplify complex logical expressions. Instead of nesting multiple AND/OR conditions, a CASE statement can consolidate these conditions into a single, readable structure. This not only enhances the clarity of the SQL code but also improves maintainability, making it easier for developers to understand and modify queries as necessary.
Moreover, leveraging a CASE statement can lead to performance optimizations in certain scenarios. By reducing the number of logical checks required in the WHERE clause, the database engine can execute queries more efficiently. However, it is essential to use this feature judiciously, as overly complex CASE statements can sometimes lead to performance degradation if not implemented thoughtfully.
incorporating a CASE statement in the WHERE clause is a valuable skill for SQL practitioners. It allows for enhanced query flexibility, improved readability, and potential performance benefits. By
Author Profile
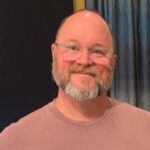
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?