How Can You Effectively Drop a Column in Python?
In the world of data analysis and manipulation, the ability to efficiently manage your datasets is paramount. Whether you’re working with large-scale databases or simple spreadsheets, knowing how to drop a column in Python can significantly streamline your workflow and enhance your data preprocessing skills. Python, with its powerful libraries like Pandas, offers intuitive methods to handle data structures, allowing you to focus on extracting insights rather than getting bogged down by unnecessary information.
Dropping a column in Python is a fundamental operation that can help you clean your data by removing irrelevant or redundant features. This process is crucial for improving the performance of your models and ensuring that your analyses are based on the most pertinent information. With just a few lines of code, you can easily eliminate columns that do not contribute to your objectives, thereby simplifying your datasets and making them more manageable.
As you delve deeper into this topic, you’ll discover various techniques and best practices for dropping columns in Python. From understanding the syntax to exploring different scenarios where column removal is beneficial, this guide will equip you with the knowledge to enhance your data manipulation capabilities. So, whether you’re a novice looking to learn the basics or an experienced analyst seeking to refine your skills, you’ll find valuable insights that will empower you to handle your data with confidence.
Using Pandas to Drop a Column
Pandas is a powerful data manipulation library in Python that provides an efficient way to handle data structures, including DataFrames. Dropping a column in a DataFrame can be accomplished using the `drop()` method, which allows for flexibility in specifying which columns to remove.
To drop a column, you can use the following syntax:
“`python
import pandas as pd
Sample DataFrame
data = {
‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]
}
df = pd.DataFrame(data)
Dropping a column
df = df.drop(‘B’, axis=1)
“`
Key parameters of the `drop()` method include:
- labels: The names of the columns to drop.
- axis: The axis along which to drop, with `0` for rows and `1` for columns.
- inplace: If set to `True`, the DataFrame will be modified in place. If “, a new DataFrame will be returned.
Dropping Multiple Columns
To drop multiple columns simultaneously, you can pass a list of column names to the `labels` parameter. Here is an example:
“`python
Dropping multiple columns
df = df.drop([‘A’, ‘C’], axis=1)
“`
This will remove both columns ‘A’ and ‘C’ from the DataFrame.
Handling Errors While Dropping Columns
When attempting to drop a column that does not exist in the DataFrame, it is advisable to handle potential errors. You can do this using a `try` and `except` block. Alternatively, you can set the `errors` parameter of the `drop()` method to `’ignore’`, which will suppress the error if the column is not found.
“`python
Safely dropping a column
df = df.drop(‘D’, axis=1, errors=’ignore’)
“`
Example DataFrame Before and After Dropping Columns
To illustrate the effect of dropping columns, consider the following example DataFrame:
A | B | C |
---|---|---|
1 | 4 | 7 |
2 | 5 | 8 |
3 | 6 | 9 |
After executing the command `df = df.drop(‘B’, axis=1)`, the DataFrame will be transformed as follows:
A | C |
---|---|
1 | 7 |
2 | 8 |
3 | 9 |
This example demonstrates how easily columns can be managed within a DataFrame using Pandas.
Using Pandas to Drop a Column
One of the most common libraries for data manipulation in Python is Pandas. Dropping a column in a DataFrame is straightforward and can be accomplished with the `drop()` method. Below is a detailed approach to doing this.
Syntax:
“`python
DataFrame.drop(labels, axis=1, inplace=)
“`
- labels: The name(s) of the column(s) you want to drop.
- axis: Set to `1` to indicate that you are dropping a column.
- inplace: If `True`, the operation is performed in place and the DataFrame is modified. If “, a new DataFrame is returned.
Example:
“`python
import pandas as pd
Create a sample DataFrame
data = {
‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]
}
df = pd.DataFrame(data)
Drop column ‘B’
df_dropped = df.drop(‘B’, axis=1)
print(df_dropped)
“`
Output:
“`
A C
0 1 7
1 2 8
2 3 9
“`
Dropping Multiple Columns
To drop multiple columns simultaneously, you can provide a list of column names to the `labels` parameter.
Example:
“`python
Drop columns ‘A’ and ‘C’
df_dropped_multiple = df.drop([‘A’, ‘C’], axis=1)
print(df_dropped_multiple)
“`
Output:
“`
B
0 4
1 5
2 6
“`
Dropping Columns with Conditions
Sometimes, you may want to drop columns based on certain conditions. For instance, if you want to drop columns that contain null values, you can use the `dropna()` method.
Example:
“`python
Create a DataFrame with NaN values
data_with_nan = {
‘A’: [1, 2, None],
‘B’: [4, None, 6],
‘C’: [7, 8, 9]
}
df_nan = pd.DataFrame(data_with_nan)
Drop columns with any NaN values
df_no_nan = df_nan.dropna(axis=1)
print(df_no_nan)
“`
Output:
“`
C
0 7
1 8
2 9
“`
Dropping Columns in Place
If you prefer to modify the original DataFrame directly without creating a new one, set the `inplace` parameter to `True`.
Example:
“`python
Drop column ‘A’ in place
df_nan.drop(‘A’, axis=1, inplace=True)
print(df_nan)
“`
Output:
“`
B C
0 4.0 7.0
1 NaN 8.0
2 6.0 9.0
“`
Using Del Keyword to Drop Columns
An alternative method to remove a column is by using the `del` statement. This method is less flexible than `drop()` since it cannot handle multiple columns at once, but it is quite effective for a single column.
Example:
“`python
Delete column ‘B’
del df[‘B’]
print(df)
“`
Output:
“`
A C
0 1 7
1 2 8
2 3 9
“`
Dropping columns in Python using Pandas is a versatile task that can be accomplished in various ways depending on specific requirements. Whether using the `drop()` method for flexibility or the `del` keyword for simplicity, the ability to manage your DataFrame’s structure is crucial for effective data analysis.
Expert Insights on Dropping Columns in Python
Dr. Emily Carter (Data Scientist, Analytics Innovations). “When it comes to data manipulation in Python, using the Pandas library is essential. Dropping a column can be efficiently achieved with the `drop()` method, which allows for both in-place modifications and the option to specify whether to drop by column name or index.”
James Liu (Software Engineer, DataTech Solutions). “Understanding the implications of dropping a column is crucial. It is not merely about removing data; one must consider how this action affects the integrity of the dataset and any subsequent analyses. Always ensure to back up your data before performing such operations.”
Maria Gonzalez (Machine Learning Engineer, AI Research Group). “In machine learning workflows, dropping irrelevant or redundant features can significantly improve model performance. Utilizing the `drop()` function in Pandas is a straightforward approach, but I recommend conducting exploratory data analysis to identify which columns should be removed.”
Frequently Asked Questions (FAQs)
How can I drop a column from a Pandas DataFrame in Python?
You can drop a column from a Pandas DataFrame using the `drop()` method. For example, `df.drop(‘column_name’, axis=1, inplace=True)` will remove the specified column from the DataFrame.
What does the `axis` parameter in the `drop()` method signify?
The `axis` parameter specifies whether to drop a row or a column. Setting `axis=0` indicates rows, while `axis=1` indicates columns.
Is it possible to drop multiple columns at once in a DataFrame?
Yes, you can drop multiple columns by passing a list of column names. For example, `df.drop([‘column1’, ‘column2’], axis=1, inplace=True)` will remove both specified columns.
What happens if I try to drop a column that does not exist?
If you attempt to drop a non-existent column, Pandas will raise a `KeyError`. To avoid this, you can set the `errors` parameter to `’ignore’`, which will suppress the error.
Can I drop a column without modifying the original DataFrame?
Yes, you can drop a column without modifying the original DataFrame by setting `inplace=`. This will return a new DataFrame with the specified column removed, while the original remains unchanged.
How do I verify if a column has been successfully dropped?
You can verify if a column has been dropped by checking the DataFrame’s columns using `df.columns`. If the column is no longer listed, it has been successfully removed.
In Python, dropping a column from a DataFrame is a common operation, particularly when using the pandas library. This process can be accomplished using the `drop()` method, which allows users to specify the column(s) they wish to remove by providing the column name(s) and the appropriate axis parameter. It is essential to understand that this operation can be performed in place or can return a new DataFrame, depending on the user’s requirements.
Additionally, when dropping columns, it is crucial to handle potential errors gracefully. For instance, attempting to drop a column that does not exist will raise a KeyError. To mitigate this, users can utilize the `errors` parameter in the `drop()` method, setting it to ‘ignore’ to avoid disruptions in the workflow. This flexibility makes pandas a powerful tool for data manipulation in Python.
In summary, mastering the technique of dropping columns in Python using pandas is vital for effective data cleaning and preparation. By leveraging the `drop()` method, users can efficiently manage their DataFrames, ensuring that only relevant data is retained for analysis. Understanding the nuances of this operation, including error handling, enhances the user’s ability to work with data in a robust and efficient manner.
Author Profile
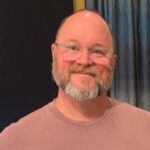
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?