How Can You Remove Non-Alphanumeric Characters in Python?
In the world of programming, data cleanliness is paramount. Whether you’re processing user input, cleaning up text data for analysis, or preparing strings for display, the presence of non-alphanumeric characters can be a significant hurdle. These characters, which include punctuation marks, symbols, and whitespace, can introduce noise into your datasets, leading to inaccurate results or unexpected behavior in your applications. If you’ve ever found yourself wrestling with messy strings in Python, you’re not alone. Fortunately, there are effective methods to remove these unwanted characters and restore order to your data.
Removing non-alphanumeric characters in Python is a straightforward task that can greatly enhance the quality of your data. By leveraging Python’s powerful string manipulation capabilities, you can easily filter out characters that do not fall within the alphanumeric range. This process not only simplifies your strings but also prepares them for further processing or analysis. Whether you’re working with text from user inputs, web scraping, or data cleaning, understanding how to efficiently handle these characters is an essential skill for any Python programmer.
In this article, we will explore various techniques to strip away non-alphanumeric characters, ranging from simple string methods to more advanced regular expressions. You’ll learn how to implement these techniques in practical scenarios, ensuring that your data is clean, consistent, and ready for whatever tasks
Using Regular Expressions
Regular expressions provide a powerful way to identify and remove non-alphanumeric characters in strings. The `re` module in Python is the standard library that allows you to use regular expressions.
Here’s how to use regular expressions to remove non-alphanumeric characters:
“`python
import re
def remove_non_alphanumeric(input_string):
return re.sub(r’\W+’, ”, input_string)
sample_text = “Hello, World! 123 Python”
cleaned_text = remove_non_alphanumeric(sample_text)
print(cleaned_text) Output: HelloWorld123Python
“`
In this example, the `re.sub()` function replaces all occurrences of non-alphanumeric characters with an empty string. The pattern `\W` matches any character that is not a word character (equivalent to `[a-zA-Z0-9_]`).
Using String Methods
Another approach to remove non-alphanumeric characters is by using Python’s built-in string methods. This method is generally more straightforward but may require more code than regular expressions.
The following code demonstrates how to achieve this using a list comprehension:
“`python
def remove_non_alphanumeric(input_string):
return ”.join(char for char in input_string if char.isalnum())
sample_text = “Welcome to Python! 2023.”
cleaned_text = remove_non_alphanumeric(sample_text)
print(cleaned_text) Output: WelcometoPython2023
“`
In this implementation, the `isalnum()` method checks whether each character is alphanumeric, and only those characters are joined together to form the cleaned string.
Performance Considerations
When choosing between regular expressions and string methods for removing non-alphanumeric characters, performance can be a factor, especially with large datasets.
Method | Speed | Readability | Flexibility |
---|---|---|---|
Regular Expressions | Moderate | Moderate | High |
String Methods | Fast | High | Low |
- Regular Expressions: Best for complex patterns and flexibility in matching.
- String Methods: Optimal for simpler tasks where performance is critical.
Both methods have their advantages and can be selected based on the specific needs of your application. Regular expressions offer more power and flexibility, while string methods provide faster performance for simpler tasks.
Using Regular Expressions
To effectively remove non-alphanumeric characters from strings in Python, the `re` module provides a powerful tool through regular expressions. This method allows for complex pattern matching and substitution.
“`python
import re
def remove_non_alphanumeric(input_string):
return re.sub(r'[^a-zA-Z0-9]’, ”, input_string)
Example usage
cleaned_string = remove_non_alphanumeric(“Hello, World! 123.”)
print(cleaned_string) Output: HelloWorld123
“`
In this example, the regular expression `[^a-zA-Z0-9]` matches any character that is not a letter or digit, and replaces it with an empty string.
Using String Methods
An alternative approach to removing non-alphanumeric characters is by utilizing Python’s built-in string methods. This method is straightforward but may require additional logic to filter out unwanted characters.
“`python
def remove_non_alphanumeric(input_string):
return ”.join(char for char in input_string if char.isalnum())
Example usage
cleaned_string = remove_non_alphanumeric(“Hello, World! 123.”)
print(cleaned_string) Output: HelloWorld123
“`
Here, the `isalnum()` method checks if each character is alphanumeric, and `join()` concatenates the filtered characters back into a string.
Using List Comprehensions
List comprehensions offer a concise way to remove non-alphanumeric characters. This approach is both readable and efficient.
“`python
def remove_non_alphanumeric(input_string):
return ”.join([c for c in input_string if c.isalnum()])
Example usage
cleaned_string = remove_non_alphanumeric(“[email protected]”)
print(cleaned_string) Output: Python39
“`
The comprehension iterates over each character, retaining only those that meet the alphanumeric criteria.
Performance Considerations
When choosing a method for removing non-alphanumeric characters, consider the following factors:
Method | Complexity | Readability | Performance |
---|---|---|---|
Regular Expressions | O(n) | Moderate | Moderate |
String Methods | O(n) | High | High |
List Comprehensions | O(n) | High | High |
- Regular Expressions: Best for complex patterns but may introduce overhead.
- String Methods: Highly readable and efficient for simple tasks.
- List Comprehensions: Combines readability and performance effectively.
Selecting the appropriate method for removing non-alphanumeric characters depends on the specific needs of your application, such as performance requirements and code clarity. Each approach has its strengths and can be implemented based on the context of use.
Expert Insights on Removing Non-Alphanumeric Characters in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “When dealing with data preprocessing in Python, removing non-alphanumeric characters is crucial for ensuring data integrity. Utilizing regular expressions via the `re` module is an effective approach, as it allows for precise control over which characters to eliminate.”
Mark Thompson (Software Engineer, CodeCraft Solutions). “In my experience, using the `str.isalnum()` method in a list comprehension is a straightforward way to filter out unwanted characters. This method provides a clean and efficient solution, especially when working with large datasets.”
Linda Zhao (Python Developer, DataSecure Corp.). “For projects requiring a robust solution, I recommend combining the `re.sub()` function with a well-defined pattern to remove non-alphanumeric characters. This method not only enhances readability but also improves performance in data cleaning tasks.”
Frequently Asked Questions (FAQs)
How can I remove non-alphanumeric characters from a string in Python?
You can use the `re` module with a regular expression to remove non-alphanumeric characters. For example:
“`python
import re
cleaned_string = re.sub(r’\W+’, ”, original_string)
“`
What does the `\W` character class represent in regular expressions?
The `\W` character class matches any character that is not a word character, which includes alphanumeric characters and underscores. Thus, it effectively targets non-alphanumeric characters.
Is there a way to remove non-alphanumeric characters without using regular expressions?
Yes, you can use a list comprehension combined with the `str.isalnum()` method to filter out non-alphanumeric characters. For example:
“`python
cleaned_string = ”.join(char for char in original_string if char.isalnum())
“`
Can I remove specific non-alphanumeric characters instead of all of them?
Yes, you can specify which characters to remove by using the `str.replace()` method or a custom filtering approach. For instance:
“`python
for char in [‘!’, ‘@’, ”]:
original_string = original_string.replace(char, ”)
“`
What is the difference between `str.isalnum()` and `str.isalpha()`?
`str.isalnum()` checks if all characters in a string are alphanumeric (letters and numbers), while `str.isalpha()` checks if all characters are alphabetic (only letters). Use `str.isalnum()` to retain numbers as well.
Are there any performance considerations when removing non-alphanumeric characters in large strings?
Yes, using regular expressions can be more efficient for large strings compared to iterative methods. However, the choice depends on the specific use case and the complexity of the operations involved.
In summary, removing non-alphanumeric characters in Python can be efficiently achieved using various methods, including regular expressions, string methods, and list comprehensions. Regular expressions, particularly with the `re` module, provide a powerful and flexible way to identify and eliminate unwanted characters from strings. This approach allows for precise control over which characters to retain or discard, making it suitable for complex string manipulation tasks.
Additionally, the `str.isalnum()` method can be utilized in conjunction with list comprehensions to filter out non-alphanumeric characters. This method is straightforward and enhances code readability, making it a preferred choice for simpler applications. The choice of method often depends on the specific requirements of the task, such as performance considerations and the complexity of the input data.
Key takeaways include the importance of understanding the context in which the string manipulation is being performed. For example, when dealing with user input or data cleaning, ensuring that only valid characters remain can significantly improve data integrity. Furthermore, leveraging Python’s built-in capabilities alongside libraries like `re` can streamline the process and enhance code maintainability.
Author Profile
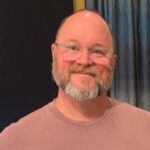
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?