How Can You Easily Append to a Dictionary in Python?
In the world of Python programming, dictionaries stand out as one of the most versatile and powerful data structures. They allow you to store and manage data in a key-value format, making it easy to access and manipulate information. Whether you’re building a simple application or tackling complex data processing tasks, knowing how to effectively append to a dictionary can significantly enhance your coding efficiency. In this article, we will explore the various methods and best practices for adding new entries to dictionaries, empowering you to harness their full potential.
Appending to a dictionary in Python is not just about adding new keys and values; it’s about understanding the nuances of this dynamic data structure. As you delve deeper, you’ll discover that there are multiple approaches to achieve this, each suited for different scenarios. From straightforward assignments to more advanced techniques involving methods like `update()`, the possibilities are vast.
Moreover, we will discuss the implications of appending to dictionaries, including how it affects data integrity and performance. By the end of this exploration, you’ll not only be equipped with practical skills but also gain insights into when and why to choose specific methods for appending to dictionaries. So, let’s embark on this journey to master one of Python’s foundational elements!
Appending Items to a Dictionary
In Python, appending items to a dictionary is a straightforward process. A dictionary is a mutable, unordered collection of items that store data in key-value pairs. To add or update an item in a dictionary, you simply assign a value to a key. If the key already exists, the existing value will be updated; if it does not, a new key-value pair will be created.
To append to a dictionary, you can use the following methods:
- Using the assignment operator: This is the most common method for adding items.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict[‘c’] = 3 Adding a new key ‘c’ with value 3
“`
- Using the `update()` method: This method can add multiple key-value pairs at once.
“`python
my_dict.update({‘d’: 4, ‘e’: 5}) Adding multiple keys
“`
- Using the `setdefault()` method: This method adds a new key with a default value only if the key does not already exist.
“`python
my_dict.setdefault(‘f’, 6) Adds ‘f’ with value 6 if ‘f’ is not present
“`
Appending Multiple Items
When you need to append multiple items to a dictionary, the `update()` method is particularly useful. It allows you to merge another dictionary or an iterable of key-value pairs into your existing dictionary.
Here is an example of using `update()`:
“`python
my_dict = {‘a’: 1, ‘b’: 2}
new_items = {‘c’: 3, ‘d’: 4}
my_dict.update(new_items)
“`
After executing this code, `my_dict` will contain:
Key | Value |
---|---|
a | 1 |
b | 2 |
c | 3 |
d | 4 |
Using Dictionary Comprehensions
Another efficient way to append items to a dictionary is through dictionary comprehensions. This method is particularly useful when you want to create a new dictionary based on existing data.
Example of using a dictionary comprehension:
“`python
existing_dict = {‘a’: 1, ‘b’: 2}
additional_items = {‘c’: 3, ‘d’: 4}
combined_dict = {key: value for d in (existing_dict, additional_items) for key, value in d.items()}
“`
This will result in a new dictionary `combined_dict` that contains all keys and values from both dictionaries.
Performance Considerations
When appending items to a dictionary, performance can vary based on the method used. Here’s a quick comparison:
Method | Time Complexity | Description |
---|---|---|
Assignment | O(1) | Directly adds or updates a key |
`update()` | O(k) | Adds k items from another dictionary |
`setdefault()` | O(1) | Adds a key only if it doesn’t exist |
Comprehension | O(n) | Creates a new dictionary, iterating through all items |
In general, using the assignment operator is the most efficient for single items, while `update()` is best for batch operations. Understanding these distinctions can help optimize the performance of your code.
Appending to a Dictionary in Python
Appending to a dictionary in Python can be accomplished in several ways, depending on the desired outcome. This section outlines the most common methods for adding new key-value pairs or updating existing ones.
Using the Assignment Operator
One of the simplest ways to append to a dictionary is by using the assignment operator. This method allows you to add a new key-value pair or update the value of an existing key.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict[‘c’] = 3 Adding a new key-value pair
my_dict[‘a’] = 10 Updating an existing key
“`
After executing the above code, `my_dict` will be `{‘a’: 10, ‘b’: 2, ‘c’: 3}`.
Using the `update()` Method
The `update()` method provides a convenient way to append multiple key-value pairs to a dictionary at once. This method can accept another dictionary or an iterable of key-value pairs.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict.update({‘c’: 3, ‘d’: 4}) Adding multiple key-value pairs
“`
Alternatively, using an iterable:
“`python
my_dict.update([(‘e’, 5), (‘f’, 6)]) Adding key-value pairs from a list of tuples
“`
After these operations, `my_dict` will be `{‘a’: 1, ‘b’: 2, ‘c’: 3, ‘d’: 4, ‘e’: 5, ‘f’: 6}`.
Using the `setdefault()` Method
The `setdefault()` method is useful when you want to append a key with a default value only if the key does not already exist in the dictionary.
“`python
my_dict = {‘a’: 1, ‘b’: 2}
my_dict.setdefault(‘c’, 3) Adds ‘c’ with value 3 if ‘c’ is not in the dictionary
my_dict.setdefault(‘a’, 10) Does not change ‘a’ since it already exists
“`
The resulting dictionary will be `{‘a’: 1, ‘b’: 2, ‘c’: 3}`.
Appending to Nested Dictionaries
When dealing with nested dictionaries, you can append entries by specifying the key of the outer dictionary first.
“`python
nested_dict = {‘outer_key’: {‘inner_key1’: ‘value1’}}
nested_dict[‘outer_key’][‘inner_key2’] = ‘value2’ Adding to the inner dictionary
“`
After this operation, `nested_dict` becomes `{‘outer_key’: {‘inner_key1’: ‘value1’, ‘inner_key2’: ‘value2’}}`.
Using Dictionary Comprehensions
In some cases, you might want to create a new dictionary by appending values from an existing one. Dictionary comprehensions can be a powerful tool for this.
“`python
original_dict = {‘a’: 1, ‘b’: 2}
appended_dict = {k: v for k, v in original_dict.items()}
appended_dict[‘c’] = 3 Appending a new key-value pair
“`
This results in `appended_dict` being `{‘a’: 1, ‘b’: 2, ‘c’: 3}`.
Performance Considerations
When appending to dictionaries, it is essential to consider performance:
Operation | Time Complexity |
---|---|
Assignment | O(1) |
`update()` | O(k) |
`setdefault()` | O(1) |
Nested append | O(1) |
In general, appending a single key-value pair or updating an existing key is efficient, while adding multiple pairs at once with `update()` may have a higher overhead depending on the number of pairs being added.
Expert Insights on Appending to Dictionaries in Python
Dr. Emily Chen (Senior Software Engineer, Tech Innovations Inc.). “Appending to a dictionary in Python is straightforward and efficient. The key is to understand that dictionaries are mutable, allowing you to add new key-value pairs dynamically using simple syntax. This flexibility is essential for developers who need to manage data structures in real-time applications.”
Michael Thompson (Data Scientist, Analytics Hub). “When working with dictionaries in Python, it is crucial to remember that keys must be unique. If you attempt to append a key that already exists, the value will be overwritten. This behavior can be leveraged to update existing records seamlessly, making dictionaries a powerful tool for data manipulation.”
Sarah Patel (Python Developer and Educator, Code Academy). “Using the `update()` method is one of the most effective ways to append multiple key-value pairs to a dictionary. This method not only enhances readability but also improves performance when compared to adding items one at a time. Mastering this technique is vital for any Python programmer aiming to work with complex data structures.”
Frequently Asked Questions (FAQs)
How do I append a single key-value pair to a dictionary in Python?
You can append a single key-value pair to a dictionary by using the assignment operator. For example, `my_dict[‘new_key’] = ‘new_value’` adds the key `’new_key’` with the value `’new_value’` to `my_dict`.
Can I append multiple key-value pairs to a dictionary at once?
Yes, you can append multiple key-value pairs using the `update()` method. For instance, `my_dict.update({‘key1’: ‘value1’, ‘key2’: ‘value2’})` adds both pairs to `my_dict`.
What happens if I append a key that already exists in the dictionary?
If you append a key that already exists, the existing value will be overwritten with the new value. For example, `my_dict[‘existing_key’] = ‘new_value’` will replace the old value associated with `’existing_key’`.
Is there a way to append key-value pairs from another dictionary?
Yes, you can use the `update()` method to append key-value pairs from another dictionary. For example, `my_dict.update(another_dict)` will add all key-value pairs from `another_dict` to `my_dict`.
Can I append to a nested dictionary in Python?
Yes, you can append to a nested dictionary by first accessing the inner dictionary and then adding the key-value pair. For example, `my_dict[‘outer_key’][‘inner_key’] = ‘value’` adds a value to the nested dictionary.
What is the best practice for appending to a dictionary in Python?
The best practice is to use the `update()` method for multiple additions and direct assignment for single key-value pairs. This approach ensures clarity and maintains the integrity of the dictionary structure.
In Python, appending to a dictionary is a straightforward process that involves adding new key-value pairs or updating existing ones. Dictionaries in Python are mutable data structures, allowing for dynamic modifications. To append to a dictionary, one can simply assign a value to a new key using the syntax `dict[key] = value`. If the key already exists, this operation will update the existing value associated with that key rather than creating a duplicate.
Another method to append multiple items at once is by using the `update()` method. This method takes another dictionary or an iterable of key-value pairs and merges them into the existing dictionary. This functionality is particularly useful when dealing with bulk data updates or when integrating data from different sources.
It is also important to consider the implications of appending to a dictionary, such as potential key collisions and the need for unique keys. When appending items, one should ensure that the keys remain distinct to avoid unintended overwrites. Additionally, understanding the performance characteristics of dictionaries in Python can help in optimizing operations, especially when dealing with large datasets.
In summary, appending to a dictionary in Python is an essential skill that enhances data manipulation capabilities. By mastering the techniques of adding and updating key-value pairs
Author Profile
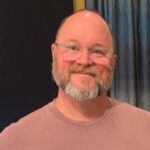
-
I’m Leonard a developer by trade, a problem solver by nature, and the person behind every line and post on Freak Learn.
I didn’t start out in tech with a clear path. Like many self taught developers, I pieced together my skills from late-night sessions, half documented errors, and an internet full of conflicting advice. What stuck with me wasn’t just the code it was how hard it was to find clear, grounded explanations for everyday problems. That’s the gap I set out to close.
Freak Learn is where I unpack the kind of problems most of us Google at 2 a.m. not just the “how,” but the “why.” Whether it's container errors, OS quirks, broken queries, or code that makes no sense until it suddenly does I try to explain it like a real person would, without the jargon or ego.
Latest entries
- May 11, 2025Stack Overflow QueriesHow Can I Print a Bash Array with Each Element on a Separate Line?
- May 11, 2025PythonHow Can You Run Python on Linux? A Step-by-Step Guide
- May 11, 2025PythonHow Can You Effectively Stake Python for Your Projects?
- May 11, 2025Hardware Issues And RecommendationsHow Can You Configure an Existing RAID 0 Setup on a New Motherboard?